svcore
1.9
|
RecentFiles manages a list of recently-used identifier strings, saving and restoring that list via QSettings. More...
#include <RecentFiles.h>
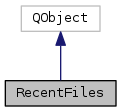
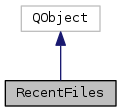
Signals | |
void | recentChanged () |
Public Member Functions | |
RecentFiles (QString settingsGroup="RecentFiles", int maxCount=10) | |
Construct a RecentFiles object that saves and restores in the given QSettings group and truncates when the given count of identifiers is reached. More... | |
virtual | ~RecentFiles () |
QString | getSettingsGroup () const |
Return the settingsGroup as passed to the constructor. More... | |
int | getMaxCount () const |
Return the maxCount as passed to the constructor. More... | |
std::vector< QString > | getRecentIdentifiers () const |
Return the list of recent identifiers, without labels. More... | |
std::vector< QString > | getRecent () const |
Return the list of recent identifiers, without labels. More... | |
std::vector< std::pair< QString, QString > > | getRecentEntries () const |
Return the list of recent identifiers, with labels. More... | |
void | add (QString identifier, QString label="") |
Add a literal identifier, optionally with a label. More... | |
void | addFile (QString filepath, QString label="") |
Add a name that is known to be either a file path or a URL, optionally with a label. More... | |
Private Member Functions | |
void | read () |
void | write () |
void | truncateAndWrite () |
Private Attributes | |
QMutex | m_mutex |
const QString | m_settingsGroup |
const int | m_maxCount |
std::deque< std::pair< QString, QString > > | m_entries |
Detailed Description
RecentFiles manages a list of recently-used identifier strings, saving and restoring that list via QSettings.
The identifiers do not actually have to refer to files.
Each entry must have a non-empty identifier, which is typically a filename, path, URI, or internal id, and may optionally also have a label, which is typically a user-visible convenience.
RecentFiles is thread-safe - all access is serialised.
Definition at line 36 of file RecentFiles.h.
Constructor & Destructor Documentation
RecentFiles::RecentFiles | ( | QString | settingsGroup = "RecentFiles" , |
int | maxCount = 10 |
||
) |
Construct a RecentFiles object that saves and restores in the given QSettings group and truncates when the given count of identifiers is reached.
Definition at line 25 of file RecentFiles.cpp.
References read().
|
virtual |
Definition at line 32 of file RecentFiles.cpp.
Member Function Documentation
|
inline |
Return the settingsGroup as passed to the constructor.
Definition at line 54 of file RecentFiles.h.
References m_settingsGroup.
|
inline |
Return the maxCount as passed to the constructor.
Definition at line 61 of file RecentFiles.h.
References getRecentIdentifiers(), and m_maxCount.
std::vector< QString > RecentFiles::getRecentIdentifiers | ( | ) | const |
Return the list of recent identifiers, without labels.
Definition at line 101 of file RecentFiles.cpp.
References m_entries, m_maxCount, and m_mutex.
Referenced by getMaxCount(), and getRecent().
|
inline |
Return the list of recent identifiers, without labels.
This is an alias for getRecentIdentifiers included for backward compatibility.
Definition at line 75 of file RecentFiles.h.
References add(), addFile(), getRecentEntries(), getRecentIdentifiers(), and recentChanged().
std::vector< std::pair< QString, QString > > RecentFiles::getRecentEntries | ( | ) | const |
Return the list of recent identifiers, with labels.
Each returned entry is a pair of identifier and label in that order.
Definition at line 116 of file RecentFiles.cpp.
References m_entries, m_maxCount, and m_mutex.
Referenced by getRecent().
void RecentFiles::add | ( | QString | identifier, |
QString | label = "" |
||
) |
Add a literal identifier, optionally with a label.
If the identifier already exists in the recent entries list, it is moved to the front of the list and its label is replaced with the given one.
Definition at line 131 of file RecentFiles.cpp.
References in_range_for(), m_entries, m_mutex, recentChanged(), and truncateAndWrite().
Referenced by addFile(), and getRecent().
void RecentFiles::addFile | ( | QString | filepath, |
QString | label = "" |
||
) |
Add a name that is known to be either a file path or a URL, optionally with a label.
If it looks like a URL, add it literally; otherwise treat it as a file path and canonicalise it appropriately. Also take into account the user preference for whether to include temporary files in the recent files menu: the file will not be added if the preference is set and the file appears to be a temporary one.
If the identifier derived from the file path already exists in the recent entries list, it is moved to the front of the list and its label is replaced with the given one.
Definition at line 163 of file RecentFiles.cpp.
References add(), Preferences::getInstance(), and Preferences::getOmitTempsFromRecentFiles().
Referenced by getRecent().
|
signal |
Referenced by add(), and getRecent().
|
private |
Definition at line 38 of file RecentFiles.cpp.
References m_entries, m_maxCount, and m_settingsGroup.
Referenced by RecentFiles().
|
private |
Definition at line 66 of file RecentFiles.cpp.
References in_range_for(), m_entries, m_maxCount, and m_settingsGroup.
Referenced by truncateAndWrite().
|
private |
Definition at line 90 of file RecentFiles.cpp.
References m_entries, m_maxCount, and write().
Referenced by add().
Member Data Documentation
|
mutableprivate |
Definition at line 113 of file RecentFiles.h.
Referenced by add(), getRecentEntries(), and getRecentIdentifiers().
|
private |
Definition at line 115 of file RecentFiles.h.
Referenced by getSettingsGroup(), read(), and write().
|
private |
Definition at line 116 of file RecentFiles.h.
Referenced by getMaxCount(), getRecentEntries(), getRecentIdentifiers(), read(), truncateAndWrite(), and write().
|
private |
Definition at line 118 of file RecentFiles.h.
Referenced by add(), getRecentEntries(), getRecentIdentifiers(), read(), truncateAndWrite(), and write().
The documentation for this class was generated from the following files:
Generated by
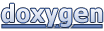