svcore
1.9
|
Pitch.h
Go to the documentation of this file.
static int getPitchForNoteAndOctave(int note, int octave)
Return the MIDI pitch for the given note number (0-12 where 0 is C) and octave number.
Definition: Pitch.cpp:104
static double getFrequencyForPitch(int midiPitch, double centsOffset=0, double concertA=0.0)
Return the frequency at the given MIDI pitch plus centsOffset cents (1/100ths of a semitone)...
Definition: Pitch.cpp:23
static QString getLabelForPitchRange(int semis, double cents=0)
Return a string describing the given pitch range in octaves, semitones and cents. ...
Definition: Pitch.cpp:164
static QString getPitchLabelForFrequency(double frequency, double concertA=0.0, bool useFlats=false)
Return a string describing the nearest MIDI pitch to the given frequency, with cents offset...
Definition: Pitch.cpp:151
static int getPitchForFrequency(double frequency, float *centsOffsetReturn, double concertA=0.0)
Compatibility version of getPitchForFrequency accepting float pointer argument.
Definition: Pitch.h:57
static void getNoteAndOctaveForPitch(int midiPitch, int ¬e, int &octave)
Return the note number (0-12 where 0 is C) and octave number for the given MIDI pitch.
Definition: Pitch.cpp:111
static int getPitchForFrequencyDifference(double frequencyA, double frequencyB, float *centsOffsetReturn, double concertA=0.0)
Compatibility version of getPitchForFrequencyDifference accepting float pointer argument.
Definition: Pitch.h:89
Definition: Pitch.h:21
static bool isFrequencyInMidiRange(double frequency, double concertA=0.0)
Return true if the given frequency falls within the range of MIDI note pitches, plus or minus half a ...
Definition: Pitch.cpp:205
static int getPitchForFrequencyDifference(double frequencyA, double frequencyB, double *centsOffsetReturn=0, double concertA=0.0)
Return the nearest MIDI pitch range to the given frequency range, that is, the difference in MIDI pit...
Definition: Pitch.cpp:61
static int getPitchForFrequency(double frequency, double *centsOffsetReturn=0, double concertA=0.0)
Return the nearest MIDI pitch to the given frequency.
Definition: Pitch.cpp:35
static QString getPitchLabel(int midiPitch, double centsOffset=0, bool useFlats=false)
Return a string describing the given MIDI pitch, with optional cents offset.
Definition: Pitch.cpp:135
Generated by
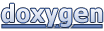