svcore
1.9
|
Labeller.h
Go to the documentation of this file.
209 std::cerr << "ERROR: Labeller::setValue: Previous point required but not provided" << std::endl;
404 std::cerr << "ERROR: Labeller::getValueFor: Real-time conversion required, but no sample rate set" << std::endl;
415 std::cerr << "ERROR: Labeller::getValueFor: Real-time conversion required, but no sample rate set" << std::endl;
417 std::cerr << "ERROR: Labeller::getValueFor: Time difference required, but only one point provided" << std::endl;
Definition: Labeller.h:158
Definition: Labeller.h:37
A selection object simply represents a range in time, via start and end frame.
Definition: Selection.h:40
Definition: Labeller.h:42
int getSecondLevelCounterValue() const
Definition: Labeller.h:124
Relabelling label(Event e, const Event *prev=nullptr)
Return a labelled event based on the given event, previous event if supplied, and internal labeller s...
Definition: Labeller.h:171
Definition: Labeller.h:46
Definition: Labeller.h:39
Definition: Selection.h:63
Command * labelAll(int editableId, MultiSelection *ms, const EventVector &allEvents)
Relabel all events in the given event vector that lie within the given multi-selection, according to the labelling properties of this labeller.
Definition: Labeller.h:232
Selection getContainingSelection(sv_frame_t frame, bool defaultToFollowing) const
Return the selection that contains a given frame.
Definition: Selection.cpp:196
Definition: Labeller.h:44
Definition: Labeller.h:159
Command * subdivide(int editableId, MultiSelection *ms, const EventVector &allEvents, int n)
For each event in the given event vector (except the last), if that event lies within the given multi...
Definition: Labeller.h:279
Command * winnow(int editableId, MultiSelection *ms, const EventVector &allEvents, int n)
The opposite of subdivide.
Definition: Labeller.h:330
An immutable(-ish) type used for point and event representation in sparse models, as well as for inte...
Definition: Event.h:55
Definition: Command.h:26
Definition: Labeller.h:36
Definition: Labeller.h:40
Definition: Labeller.h:29
Definition: Labeller.h:38
Revaluing revalue(Event e, const Event *prev=nullptr)
Return an event with a value following the labelling scheme, based on the given event, previous event if supplied, and internal labeller state.
Definition: Labeller.h:203
void setSecondLevelCounterValue(int v)
Definition: Labeller.h:125
Command to add or remove a series of events to or from an editable, with undo.
Definition: EventCommands.h:115
Definition: Labeller.h:35
Generated by
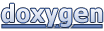