svcore
1.9
|
#include <Labeller.h>
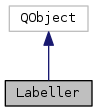
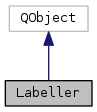
Public Types | |
enum | ValueType { ValueNone, ValueFromSimpleCounter, ValueFromCyclicalCounter, ValueFromTwoLevelCounter, ValueFromFrameNumber, ValueFromRealTime, ValueFromDurationFromPrevious, ValueFromDurationToNext, ValueFromTempoFromPrevious, ValueFromTempoToNext, ValueFromExistingNeighbour, ValueFromLabel } |
enum | Application { AppliesToThisEvent, AppliesToPreviousEvent } |
typedef std::map< ValueType, QString > | TypeNameMap |
typedef std::pair< Application, Event > | Relabelling |
typedef std::pair< Application, Event > | Revaluing |
Public Member Functions | |
Labeller (ValueType type=ValueNone) | |
Labeller (const Labeller &l) | |
virtual | ~Labeller () |
TypeNameMap | getTypeNames () const |
ValueType | getType () const |
void | setType (ValueType type) |
int | getCounterValue () const |
void | setCounterValue (int v) |
int | getSecondLevelCounterValue () const |
void | setSecondLevelCounterValue (int v) |
int | getCounterCycleSize () const |
void | setCounterCycleSize (int s) |
void | setSampleRate (sv_samplerate_t rate) |
void | resetCounters () |
void | incrementCounter () |
Relabelling | label (Event e, const Event *prev=nullptr) |
Return a labelled event based on the given event, previous event if supplied, and internal labeller state. More... | |
Revaluing | revalue (Event e, const Event *prev=nullptr) |
Return an event with a value following the labelling scheme, based on the given event, previous event if supplied, and internal labeller state. More... | |
Command * | labelAll (int editableId, MultiSelection *ms, const EventVector &allEvents) |
Relabel all events in the given event vector that lie within the given multi-selection, according to the labelling properties of this labeller. More... | |
Command * | subdivide (int editableId, MultiSelection *ms, const EventVector &allEvents, int n) |
For each event in the given event vector (except the last), if that event lies within the given multi-selection, add n-1 new events at equally spaced intervals between it and the following event. More... | |
Command * | winnow (int editableId, MultiSelection *ms, const EventVector &allEvents, int n) |
The opposite of subdivide. More... | |
bool | requiresPrevPoint () const |
bool | actingOnPrevEvent () const |
Protected Member Functions | |
float | getValueFor (Event p, const Event *prev) |
Protected Attributes | |
ValueType | m_type |
int | m_counter |
int | m_counter2 |
int | m_cycle |
int | m_dp |
sv_samplerate_t | m_rate |
Detailed Description
Definition at line 29 of file Labeller.h.
Member Typedef Documentation
typedef std::map<ValueType, QString> Labeller::TypeNameMap |
Definition at line 88 of file Labeller.h.
typedef std::pair<Application, Event> Labeller::Relabelling |
Definition at line 161 of file Labeller.h.
typedef std::pair<Application, Event> Labeller::Revaluing |
Definition at line 162 of file Labeller.h.
Member Enumeration Documentation
enum Labeller::ValueType |
Definition at line 34 of file Labeller.h.
Enumerator | |
---|---|
AppliesToThisEvent | |
AppliesToPreviousEvent |
Definition at line 157 of file Labeller.h.
Constructor & Destructor Documentation
Definition at line 69 of file Labeller.h.
|
inline |
Definition at line 77 of file Labeller.h.
|
inlinevirtual |
Definition at line 86 of file Labeller.h.
Member Function Documentation
|
inline |
Definition at line 89 of file Labeller.h.
References ValueFromCyclicalCounter, ValueFromDurationFromPrevious, ValueFromDurationToNext, ValueFromExistingNeighbour, ValueFromFrameNumber, ValueFromLabel, ValueFromRealTime, ValueFromSimpleCounter, ValueFromTempoFromPrevious, ValueFromTempoToNext, ValueFromTwoLevelCounter, and ValueNone.
|
inline |
Definition at line 118 of file Labeller.h.
References m_type.
|
inline |
Definition at line 119 of file Labeller.h.
References m_type.
|
inline |
Definition at line 121 of file Labeller.h.
References m_counter.
|
inline |
Definition at line 122 of file Labeller.h.
References m_counter.
|
inline |
Definition at line 124 of file Labeller.h.
References m_counter2.
|
inline |
Definition at line 125 of file Labeller.h.
References m_counter2.
|
inline |
Definition at line 127 of file Labeller.h.
References m_cycle.
|
inline |
Definition at line 128 of file Labeller.h.
|
inline |
Definition at line 138 of file Labeller.h.
References m_rate.
|
inline |
Definition at line 140 of file Labeller.h.
References m_counter, m_counter2, and m_cycle.
|
inline |
Definition at line 146 of file Labeller.h.
References m_counter, m_counter2, m_cycle, m_type, ValueFromCyclicalCounter, and ValueFromTwoLevelCounter.
Referenced by getValueFor(), and label().
|
inline |
Return a labelled event based on the given event, previous event if supplied, and internal labeller state.
The returned event replaces either the event provided or the previous event, depending on the Application value in the returned pair.
Definition at line 171 of file Labeller.h.
References actingOnPrevEvent(), AppliesToPreviousEvent, AppliesToThisEvent, Event::getFrame(), Event::getLabel(), getValueFor(), incrementCounter(), m_counter, m_counter2, m_type, ValueFromFrameNumber, ValueFromTwoLevelCounter, ValueNone, and Event::withLabel().
Referenced by labelAll().
Return an event with a value following the labelling scheme, based on the given event, previous event if supplied, and internal labeller state.
The returned event replaces either the event provided or the previous event, depending on the Application value in the returned pair.
Definition at line 203 of file Labeller.h.
References actingOnPrevEvent(), AppliesToPreviousEvent, AppliesToThisEvent, Event::getValue(), getValueFor(), m_type, ValueFromExistingNeighbour, and Event::withValue().
|
inline |
Relabel all events in the given event vector that lie within the given multi-selection, according to the labelling properties of this labeller.
Return a command that has been executed but not yet added to the history. The id must be that of a type that can be retrieved from the AnyById store and dynamic_cast to EventEditable.
Definition at line 232 of file Labeller.h.
References AppliesToThisEvent, Selection::contains(), MultiSelection::getContainingSelection(), and label().
|
inline |
For each event in the given event vector (except the last), if that event lies within the given multi-selection, add n-1 new events at equally spaced intervals between it and the following event.
Return a command that has been executed but not yet added to the history. The id must be that of a type that can be retrieved from the AnyById store and dynamic_cast to EventEditable.
Definition at line 279 of file Labeller.h.
References Selection::contains(), MultiSelection::getContainingSelection(), Event::getFrame(), Event::getLabel(), Event::withFrame(), and Event::withLabel().
|
inline |
The opposite of subdivide.
Given an event vector, a multi-selection, and a number n, remove all but every nth event from the vector within the extents of the multi-selection. Return a command that has been executed but not yet added to the history. The id must be that of a type that can be retrieved from the AnyById store and dynamic_cast to EventEditable.
Definition at line 330 of file Labeller.h.
References Selection::contains(), and MultiSelection::getContainingSelection().
|
inline |
Definition at line 364 of file Labeller.h.
References m_type, ValueFromDurationFromPrevious, ValueFromDurationToNext, and ValueFromTempoFromPrevious.
|
inline |
Definition at line 371 of file Labeller.h.
References m_type, ValueFromDurationToNext, and ValueFromTempoToNext.
Definition at line 377 of file Labeller.h.
References Event::getFrame(), Event::getLabel(), incrementCounter(), m_counter, m_counter2, m_dp, m_rate, m_type, ValueFromCyclicalCounter, ValueFromDurationFromPrevious, ValueFromDurationToNext, ValueFromExistingNeighbour, ValueFromFrameNumber, ValueFromLabel, ValueFromRealTime, ValueFromSimpleCounter, ValueFromTempoFromPrevious, ValueFromTempoToNext, ValueFromTwoLevelCounter, and ValueNone.
Member Data Documentation
|
protected |
Definition at line 450 of file Labeller.h.
Referenced by actingOnPrevEvent(), getType(), getValueFor(), incrementCounter(), label(), requiresPrevPoint(), revalue(), and setType().
|
protected |
Definition at line 451 of file Labeller.h.
Referenced by getCounterValue(), getValueFor(), incrementCounter(), label(), resetCounters(), setCounterCycleSize(), and setCounterValue().
|
protected |
Definition at line 452 of file Labeller.h.
Referenced by getSecondLevelCounterValue(), getValueFor(), incrementCounter(), label(), resetCounters(), and setSecondLevelCounterValue().
|
protected |
Definition at line 453 of file Labeller.h.
Referenced by getCounterCycleSize(), incrementCounter(), resetCounters(), and setCounterCycleSize().
|
protected |
Definition at line 454 of file Labeller.h.
Referenced by getValueFor(), and setCounterCycleSize().
|
protected |
Definition at line 455 of file Labeller.h.
Referenced by getValueFor(), and setSampleRate().
The documentation for this class was generated from the following file:
Generated by
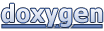