svcore
1.9
|
CSVFileWriter.cpp
Go to the documentation of this file.
A selection object simply represents a range in time, via start and end frame.
Definition: Selection.h:40
CSVFileWriter(QString path, Model *model, QString delimiter=",", DataExportOptions options=DataExportDefaults)
Definition: CSVFileWriter.cpp:33
Definition: Selection.h:63
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
virtual QVector< QString > getStringExportHeaders(DataExportOptions options) const =0
Return a label for each column that would be written by toStringExportRows.
void addSelection(const Selection &selection)
Definition: Selection.cpp:124
Definition: Exceptions.h:69
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
A class that manages the creation of a temporary file with a given prefix and the renaming of that fi...
Definition: TempWriteFile.h:26
void moveToTarget()
Rename the temporary file to the target filename.
Definition: TempWriteFile.cpp:53
virtual sv_frame_t getStartFrame() const =0
Return the first audio frame spanned by the model.
virtual void writeSelection(MultiSelection selection)
Definition: CSVFileWriter.cpp:74
void getExtents(sv_frame_t &startFrame, sv_frame_t &endFrame) const
Definition: Selection.cpp:177
QString getTemporaryFilename()
Return the name of the temporary file.
Definition: TempWriteFile.cpp:47
static QString joinDelimited(QVector< QString > row, QString delimiter)
Join a vector of strings into a single string, with the delimiter as the joining string.
Definition: StringBits.cpp:172
bool writeInChunks(OutStream &oss, const Model &model, const MultiSelection ®ions, ProgressReporter *reporter=nullptr, QString delimiter=",", DataExportOptions options=DataExportDefaults, const sv_frame_t blockSize=16384)
Definition: CSVStreamWriter.h:35
Generated by
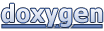