svcore
1.9
|
CSVStreamWriter.h
Go to the documentation of this file.
const SelectionList & getSelections() const
Definition: Selection.cpp:111
Definition: ProgressReporter.h:22
A selection object simply represents a range in time, via start and end frame.
Definition: Selection.h:40
Definition: CSVStreamWriter.h:30
Definition: DataExportOptions.h:20
Definition: Selection.h:63
sv_frame_t getEndFrame() const
Return the audio frame at the end of the model, i.e.
Definition: Model.h:87
void addSelection(const Selection &selection)
Definition: Selection.cpp:124
virtual QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const =0
Emit events starting within the given range as string rows ready for conversion to an e...
Model is the base class for all data models that represent any sort of data on a time scale based on ...
Definition: Model.h:51
virtual sv_frame_t getStartFrame() const =0
Return the first audio frame spanned by the model.
static QString joinDelimited(QVector< QString > row, QString delimiter)
Join a vector of strings into a single string, with the delimiter as the joining string.
Definition: StringBits.cpp:172
bool writeInChunks(OutStream &oss, const Model &model, const MultiSelection ®ions, ProgressReporter *reporter=nullptr, QString delimiter=",", DataExportOptions options=DataExportDefaults, const sv_frame_t blockSize=16384)
Definition: CSVStreamWriter.h:35
Generated by
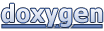