svcore
1.9
|
Dense3DModelPeakCache.cpp
Go to the documentation of this file.
30 SVCERR << "WARNING: Dense3DModelPeakCache constructed for unknown or wrong-type source model id " << m_source << endl;
float getValueAt(int col, int n) const override
Get the single data point from the n'th bin of the given column.
Definition: Dense3DModelPeakCache.cpp:51
Dense3DModelPeakCache(ModelId source, int columnsPerPeak)
Definition: Dense3DModelPeakCache.cpp:22
Column getColumn(int col) const override
Retrieve the peaks column at peak-cache column number col.
Definition: Dense3DModelPeakCache.cpp:44
void sourceModelChanged(ModelId)
Definition: Dense3DModelPeakCache.cpp:58
~Dense3DModelPeakCache()
Definition: Dense3DModelPeakCache.cpp:39
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
std::vector< bool > m_coverage
Definition: Dense3DModelPeakCache.h:140
bool haveColumn(int column) const
Definition: Dense3DModelPeakCache.cpp:69
ColumnOp::Column Column
Definition: DenseThreeDimensionalModel.h:59
void fillColumn(int column) const
Definition: Dense3DModelPeakCache.cpp:82
bool m_finalColumnIncomplete
Definition: Dense3DModelPeakCache.h:142
Definition: ById.h:115
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
Generated by
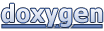