svcore
1.9
|
Dense3DModelPeakCache.h
Go to the documentation of this file.
int getResolution() const override
Return the number of sample frames covered by each column of bins.
Definition: Dense3DModelPeakCache.h:60
A DenseThreeDimensionalModel that represents a reduction in the time dimension of another DenseThreeD...
Definition: Dense3DModelPeakCache.h:31
QString getTypeName() const override
Return the type of the model.
Definition: Dense3DModelPeakCache.h:115
QVector< QString > getStringExportHeaders(DataExportOptions) const override
Return a label for each column that would be written by toStringExportRows.
Definition: Dense3DModelPeakCache.h:123
int getHeight() const override
Return the number of bins in each column.
Definition: Dense3DModelPeakCache.h:80
QString getBinName(int n) const override
Get the name of a given bin (i.e.
Definition: Dense3DModelPeakCache.h:105
float getValueAt(int col, int n) const override
Get the single data point from the n'th bin of the given column.
Definition: Dense3DModelPeakCache.cpp:51
int getWidth() const override
Return the number of columns of bins in the model.
Definition: Dense3DModelPeakCache.h:69
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: Dense3DModelPeakCache.h:50
Dense3DModelPeakCache(ModelId source, int columnsPerPeak)
Definition: Dense3DModelPeakCache.cpp:22
bool shouldUseLogValueScale() const override
Estimate whether a logarithmic scale might be appropriate for the value scale.
Definition: Dense3DModelPeakCache.h:110
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: Dense3DModelPeakCache.h:45
float getMinimumLevel() const override
Return the minimum permissible value in each bin.
Definition: Dense3DModelPeakCache.h:85
Column getColumn(int col) const override
Retrieve the peaks column at peak-cache column number col.
Definition: Dense3DModelPeakCache.cpp:44
void sourceModelChanged(ModelId)
Definition: Dense3DModelPeakCache.cpp:58
QVector< QVector< QString > > toStringExportRows(DataExportOptions, sv_frame_t, sv_frame_t) const override
Emit events starting within the given range as string rows ready for conversion to an e...
Definition: Dense3DModelPeakCache.h:128
~Dense3DModelPeakCache()
Definition: Dense3DModelPeakCache.cpp:39
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: Dense3DModelPeakCache.h:55
std::vector< bool > m_coverage
Definition: Dense3DModelPeakCache.h:140
virtual int getColumnsPerPeak() const
Definition: Dense3DModelPeakCache.h:65
bool haveColumn(int column) const
Definition: Dense3DModelPeakCache.cpp:69
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: Dense3DModelPeakCache.h:117
ColumnOp::Column Column
Definition: DenseThreeDimensionalModel.h:59
void fillColumn(int column) const
Definition: Dense3DModelPeakCache.cpp:82
bool m_finalColumnIncomplete
Definition: Dense3DModelPeakCache.h:142
float getMaximumLevel() const override
Return the maximum permissible value in each bin.
Definition: Dense3DModelPeakCache.h:90
Definition: ById.h:115
bool isOK() const override
Return true if the model was constructed successfully.
Definition: Dense3DModelPeakCache.h:40
Generated by
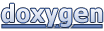