svcore
1.9
|
AggregateWaveModel.cpp
Go to the documentation of this file.
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: Model.cpp:204
void componentModelChanged(ModelId)
Definition: AggregateWaveModel.cpp:238
static PowerOfSqrtTwoZoomConstraint m_zoomConstraint
Definition: AggregateWaveModel.h:89
std::vector< Range > RangeBlock
Definition: RangeSummarisableTimeValueModel.h:76
ModelChannelSpec getComponent(int c) const
Definition: AggregateWaveModel.cpp:232
int getChannelCount() const override
Return the number of distinct channels for this model.
Definition: AggregateWaveModel.cpp:132
floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
Definition: AggregateWaveModel.cpp:147
sv_frame_t getFrameCount() const
Definition: AggregateWaveModel.cpp:119
AggregateWaveModel(ChannelSpecList channelSpecs)
Definition: AggregateWaveModel.cpp:29
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: AggregateWaveModel.cpp:138
Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const override
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Definition: AggregateWaveModel.cpp:219
int getSummaryBlockSize(int desired) const override
Definition: AggregateWaveModel.cpp:205
void componentModelChangedWithin(ModelId, sv_frame_t, sv_frame_t)
Definition: AggregateWaveModel.cpp:244
void completionChanged(ModelId myId)
Emitted when some internal processing has advanced a stage, but the model has not changed externally...
std::vector< ModelChannelSpec > ChannelSpecList
Definition: AggregateWaveModel.h:36
bool isReady(int *) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: AggregateWaveModel.cpp:93
bool in_range_for(const C &container, T i)
Check whether an integer index is in range for a container, avoiding overflows and signed/unsigned co...
Definition: BaseTypes.h:37
void modelChangedWithin(ModelId myId, sv_frame_t startFrame, sv_frame_t endFrame)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: AggregateWaveModel.cpp:256
void componentModelCompletionChanged(ModelId)
Definition: AggregateWaveModel.cpp:250
bool isOK() const override
Return true if the model was constructed successfully.
Definition: AggregateWaveModel.cpp:78
int getComponentCount() const
Definition: AggregateWaveModel.cpp:226
Definition: ById.h:115
void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const override
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
Definition: AggregateWaveModel.cpp:212
void modelChanged(ModelId myId)
Emitted when a model has been edited (or more data retrieved from cache, in the case of a cached mode...
std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
Definition: AggregateWaveModel.cpp:182
Generated by
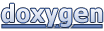