svcore
1.9
|
AggregateWaveModel.h
Go to the documentation of this file.
68 std::vector<floatvec_t> getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override;
void componentModelChanged(ModelId)
Definition: AggregateWaveModel.cpp:238
float getValueMinimum() const override
Return the minimum possible value found in this model type.
Definition: AggregateWaveModel.h:60
static PowerOfSqrtTwoZoomConstraint m_zoomConstraint
Definition: AggregateWaveModel.h:89
std::vector< Range > RangeBlock
Definition: RangeSummarisableTimeValueModel.h:76
ModelChannelSpec getComponent(int c) const
Definition: AggregateWaveModel.cpp:232
int getChannelCount() const override
Return the number of distinct channels for this model.
Definition: AggregateWaveModel.cpp:132
floatvec_t getData(int channel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from the given channel of the model in single-precision floating-poi...
Definition: AggregateWaveModel.cpp:147
sv_frame_t getFrameCount() const
Definition: AggregateWaveModel.cpp:119
AggregateWaveModel(ChannelSpecList channelSpecs)
Definition: AggregateWaveModel.cpp:29
ModelChannelSpec(ModelId m, int c)
Definition: AggregateWaveModel.h:31
Definition: AggregateWaveModel.h:24
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: AggregateWaveModel.h:64
std::vector< float, breakfastquay::StlAllocator< float > > floatvec_t
Definition: BaseTypes.h:53
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: AggregateWaveModel.cpp:138
float getValueMaximum() const override
Return the minimum possible value found in this model type.
Definition: AggregateWaveModel.h:61
QString getTypeName() const override
Return the type of the model.
Definition: AggregateWaveModel.h:49
Range getSummary(int channel, sv_frame_t start, sv_frame_t count) const override
Return the range from the given start frame, corresponding to the given number of underlying sample f...
Definition: AggregateWaveModel.cpp:219
int getSummaryBlockSize(int desired) const override
Definition: AggregateWaveModel.cpp:205
void componentModelChangedWithin(ModelId, sv_frame_t, sv_frame_t)
Definition: AggregateWaveModel.cpp:244
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: AggregateWaveModel.h:63
std::vector< ModelChannelSpec > ChannelSpecList
Definition: AggregateWaveModel.h:36
bool isReady(int *) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: AggregateWaveModel.cpp:93
Base class for models containing dense two-dimensional data (value against time) that may be meaningf...
Definition: RangeSummarisableTimeValueModel.h:32
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
Definition: AggregateWaveModel.cpp:256
ZoomConstraint is a simple interface that describes a limitation on the available zoom sizes for a vi...
Definition: ZoomConstraint.h:32
const ZoomConstraint * getZoomConstraint() const override
If this model imposes a zoom constraint, i.e.
Definition: AggregateWaveModel.h:54
void componentModelCompletionChanged(ModelId)
Definition: AggregateWaveModel.cpp:250
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
Definition: AggregateWaveModel.h:43
bool isOK() const override
Return true if the model was constructed successfully.
Definition: AggregateWaveModel.cpp:78
int getComponentCount() const
Definition: AggregateWaveModel.cpp:226
Definition: ById.h:115
void getSummaries(int channel, sv_frame_t start, sv_frame_t count, RangeBlock &ranges, int &blockSize) const override
Return ranges from the given start frame, corresponding to the given number of underlying sample fram...
Definition: AggregateWaveModel.cpp:212
std::vector< floatvec_t > getMultiChannelData(int fromchannel, int tochannel, sv_frame_t start, sv_frame_t count) const override
Get the specified set of samples from given contiguous range of channels of the model in single-preci...
Definition: AggregateWaveModel.cpp:182
Generated by
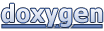