svgui
1.9
|
#include <TimeInstantLayer.h>
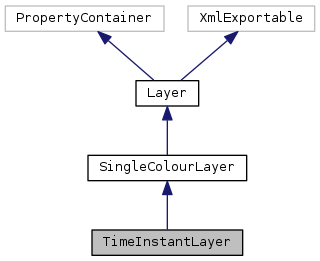
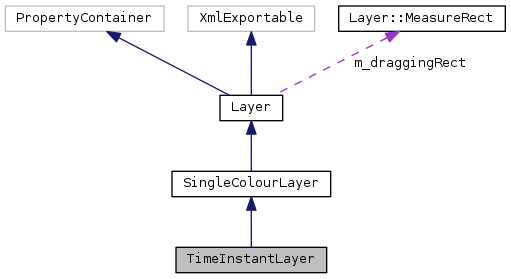
Public Types | |
enum | PlotStyle { PlotInstants, PlotSegmentation } |
enum | VerticalPosition { PositionTop, PositionMiddle, PositionBottom } |
enum | SnapType { SnapLeft, SnapRight, SnapNeighbouring } |
enum | ColourSignificance { ColourAbsent, ColourIrrelevant, ColourDistinguishes, ColourAndBackgroundSignificant, ColourHasMeaningfulValue } |
Public Slots | |
void | showLayer (LayerGeometryProvider *, bool show) |
Change the visibility status (dormancy) of the layer in the given view. More... | |
Signals | |
void | modelChanged (ModelId) |
void | modelCompletionChanged (ModelId) |
void | modelAlignmentCompletionChanged (ModelId) |
void | modelChangedWithin (ModelId, sv_frame_t startFrame, sv_frame_t endFrame) |
void | modelReplaced () |
void | layerParametersChanged () |
void | layerParameterRangesChanged () |
void | layerMeasurementRectsChanged () |
void | layerNameChanged () |
void | verticalZoomChanged () |
Public Member Functions | |
TimeInstantLayer () | |
virtual | ~TimeInstantLayer () |
void | paint (LayerGeometryProvider *v, QPainter &paint, QRect rect) const override |
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view. More... | |
QString | getLabelPreceding (sv_frame_t) const override |
QString | getFeatureDescription (LayerGeometryProvider *v, QPoint &) const override |
bool | snapToFeatureFrame (LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override |
Adjust the given frame to snap to the nearest feature, if possible. More... | |
void | drawStart (LayerGeometryProvider *v, QMouseEvent *) override |
void | drawDrag (LayerGeometryProvider *v, QMouseEvent *) override |
void | drawEnd (LayerGeometryProvider *v, QMouseEvent *) override |
void | eraseStart (LayerGeometryProvider *v, QMouseEvent *) override |
void | eraseDrag (LayerGeometryProvider *v, QMouseEvent *) override |
void | eraseEnd (LayerGeometryProvider *v, QMouseEvent *) override |
void | editStart (LayerGeometryProvider *v, QMouseEvent *) override |
void | editDrag (LayerGeometryProvider *v, QMouseEvent *) override |
void | editEnd (LayerGeometryProvider *v, QMouseEvent *) override |
bool | editOpen (LayerGeometryProvider *, QMouseEvent *) override |
Open an editor on the item under the mouse (e.g. More... | |
void | moveSelection (Selection s, sv_frame_t newStartFrame) override |
void | resizeSelection (Selection s, Selection newSize) override |
void | deleteSelection (Selection s) override |
void | copy (LayerGeometryProvider *v, Selection s, Clipboard &to) override |
bool | paste (LayerGeometryProvider *v, const Clipboard &from, sv_frame_t frameOffset, bool interactive) override |
Paste from the given clipboard onto the layer at the given frame offset. More... | |
ModelId | getModel () const override |
Return the ID of the model represented in this layer. More... | |
void | setModel (ModelId model) |
PropertyList | getProperties () const override |
QString | getPropertyLabel (const PropertyName &) const override |
PropertyType | getPropertyType (const PropertyName &) const override |
int | getPropertyRangeAndValue (const PropertyName &, int *min, int *max, int *deflt) const override |
QString | getPropertyValueLabel (const PropertyName &, int value) const override |
void | setProperty (const PropertyName &, int value) override |
void | setPlotStyle (PlotStyle style) |
PlotStyle | getPlotStyle () const |
bool | isLayerScrollable (const LayerGeometryProvider *v) const override |
This should return true if the layer can safely be scrolled automatically by a given view (simply copying the existing data and then refreshing the exposed area) without altering its meaning. More... | |
bool | isLayerEditable () const override |
This should return true if the layer can be edited by the user. More... | |
int | getCompletion (LayerGeometryProvider *) const override |
Return the proportion of background work complete in drawing this view, as a percentage – in most cases this will be the value returned by pointer from a call to the underlying model's isReady(int *) call. More... | |
bool | needsTextLabelHeight () const override |
True if this layer will need to place text labels when it is painted. More... | |
bool | getValueExtents (double &, double &, bool &, QString &) const override |
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether the layer is configured to use a logarithmic y axis display. More... | |
void | toXml (QTextStream &stream, QString indent="", QString extraAttributes="") const override |
void | setProperties (const QXmlAttributes &attributes) override |
Set the particular properties of a layer (those specific to the subclass) from a set of XML attributes. More... | |
ColourSignificance | getLayerColourSignificance () const override |
Implements Layer::getLayerColourSignificance() More... | |
int | getVerticalScaleWidth (LayerGeometryProvider *, bool, QPainter &) const override |
virtual void | setBaseColour (int) |
Set the colour used to draw primary items in the layer. More... | |
virtual int | getBaseColour () const |
Retrieve the current primary drawing colour, as a ColourDatabase index value. More... | |
bool | hasLightBackground () const override |
Return true if the layer currently has a dark colour on a light background, false if it has a light colour on a dark background. More... | |
QPixmap | getLayerPresentationPixmap (QSize size) const override |
QString | getPropertyGroupName (const PropertyName &) const override |
RangeMapper * | getNewPropertyRangeMapper (const PropertyName &) const override |
virtual void | setDefaultColourFor (LayerGeometryProvider *v) |
ModelId | getSourceModel () const |
Return the ID of the source model for the model represented in this layer. More... | |
virtual ModelId | getExportModel (LayerGeometryProvider *) const |
Return the ID of a model representing the contents of this layer in a form suitable for export to a tabular file format such as CSV. More... | |
virtual const ZoomConstraint * | getZoomConstraint () const |
Return a zoom constraint object defining the supported zoom levels for this layer. More... | |
virtual bool | supportsOtherZoomLevels () const |
Return true if this layer can handle zoom levels other than those supported by its zoom constraint (presumably less efficiently or accurately than the officially supported zoom levels). More... | |
virtual void | setSynchronousPainting (bool) |
Enable or disable synchronous painting. More... | |
virtual VerticalPosition | getPreferredTimeRulerPosition () const |
virtual VerticalPosition | getPreferredFrameCountPosition () const |
QString | getPropertyContainerIconName () const override |
QString | getPropertyContainerName () const override |
virtual void | setPresentationName (QString name) |
virtual bool | isPresentationNameSet () const |
virtual QString | getLayerPresentationName () const |
virtual void | paintVerticalScale (LayerGeometryProvider *, bool, QPainter &, QRect) const |
virtual int | getHorizontalScaleHeight (LayerGeometryProvider *, QPainter &) const |
virtual bool | getCrosshairExtents (LayerGeometryProvider *, QPainter &, QPoint, std::vector< QRect > &) const |
virtual void | paintCrosshairs (LayerGeometryProvider *, QPainter &, QPoint) const |
virtual void | paintMeasurementRects (LayerGeometryProvider *, QPainter &, bool showFocus, QPoint focusPoint) const |
virtual bool | nearestMeasurementRectChanged (LayerGeometryProvider *, QPoint prev, QPoint now) const |
virtual bool | snapToSimilarFeature (LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType) const |
Adjust the given frame to snap to the next feature that has "effectively" the same value as the feature prior to the given frame, if possible. More... | |
virtual void | splitStart (LayerGeometryProvider *, QMouseEvent *) |
virtual void | splitEnd (LayerGeometryProvider *, QMouseEvent *) |
virtual void | addNote (LayerGeometryProvider *, QMouseEvent *) |
virtual void | measureStart (LayerGeometryProvider *, QMouseEvent *) |
virtual void | measureDrag (LayerGeometryProvider *, QMouseEvent *) |
virtual void | measureEnd (LayerGeometryProvider *, QMouseEvent *) |
virtual void | measureDoubleClick (LayerGeometryProvider *, QMouseEvent *) |
virtual bool | haveCurrentMeasureRect () const |
virtual void | deleteCurrentMeasureRect () |
virtual bool | isLayerOpaque () const |
This should return true if the layer completely obscures any underlying layers. More... | |
virtual QString | getError (LayerGeometryProvider *) const |
Return an error string if any errors have occurred while loading or processing data for the given view. More... | |
virtual void | setObjectName (const QString &name) |
virtual void | toBriefXml (QTextStream &stream, QString indent="", QString extraAttributes="") const |
Produce XML containing the layer's ID and type. More... | |
virtual void | addMeasurementRect (const QXmlAttributes &) |
Add a measurement rectangle from the given XML attributes (presumably taken from a measurement element). More... | |
virtual void | setLayerDormant (const LayerGeometryProvider *v, bool dormant) |
Indicate that a layer is not currently visible in the given view and is not expected to become visible in the near future (for example because the user has explicitly removed or hidden it). More... | |
virtual bool | isLayerDormant (const LayerGeometryProvider *v) const |
Return whether the layer is dormant (i.e. More... | |
std::shared_ptr< PlayParameters > | getPlayParameters () override |
Return the play parameters for this layer, if any. More... | |
virtual bool | hasTimeXAxis () const |
Return true if the X axis on the layer is time proportional to audio frames, false otherwise. More... | |
virtual void | zoomToRegion (const LayerGeometryProvider *, QRect) |
Update the X and Y axis scales, where appropriate, to focus on the given rectangular region. More... | |
virtual bool | getDisplayExtents (double &, double &) const |
Return the minimum and maximum values within the visible area for the y axis of this layer. More... | |
virtual bool | setDisplayExtents (double, double) |
Set the displayed minimum and maximum values for the y axis to the given range, if supported. More... | |
virtual bool | adoptExtents (double, double, QString) |
Consider using the given value extents and units for this layer. More... | |
virtual bool | getXScaleValue (const LayerGeometryProvider *v, int x, double &value, QString &unit) const |
Return the value and unit at the given x coordinate in the given view. More... | |
virtual bool | getYScaleValue (const LayerGeometryProvider *, int, double &, QString &) const |
Return the value and unit at the given y coordinate in the given view. More... | |
virtual bool | getYScaleDifference (const LayerGeometryProvider *v, int y0, int y1, double &diff, QString &unit) const |
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference. More... | |
virtual int | getVerticalZoomSteps (int &) const |
Get the number of vertical zoom steps available for this layer. More... | |
virtual int | getCurrentVerticalZoomStep () const |
Get the current vertical zoom step. More... | |
virtual void | setVerticalZoomStep (int) |
Set the vertical zoom step. More... | |
virtual RangeMapper * | getNewVerticalZoomRangeMapper () const |
Create and return a range mapper for vertical zoom step values. More... | |
virtual bool | canExistWithoutModel () const |
Return true if this layer type can function without a model being set. More... | |
Protected Types | |
typedef std::map< int, int > | ColourRefCount |
typedef std::set< MeasureRect > | MeasureRectSet |
Protected Attributes | |
ModelId | m_model |
bool | m_editing |
Event | m_editingPoint |
ChangeEventsCommand * | m_editingCommand |
PlotStyle | m_plotStyle |
int | m_colour |
bool | m_colourExplicitlySet |
bool | m_defaultColourSet |
MeasureRectSet | m_measureRects |
MeasureRect | m_draggingRect |
bool | m_haveDraggingRect |
bool | m_haveCurrentMeasureRect |
QPoint | m_currentMeasureRectPoint |
QString | m_presentationName |
Static Protected Attributes | |
static ColourRefCount | m_colourRefCount |
Detailed Description
Definition at line 28 of file TimeInstantLayer.h.
Member Typedef Documentation
|
protectedinherited |
Definition at line 89 of file SingleColourLayer.h.
|
protectedinherited |
Member Enumeration Documentation
Enumerator | |
---|---|
PlotInstants | |
PlotSegmentation |
Definition at line 79 of file TimeInstantLayer.h.
|
inherited |
|
inherited |
|
inherited |
Constructor & Destructor Documentation
TimeInstantLayer::TimeInstantLayer | ( | ) |
Definition at line 42 of file TimeInstantLayer.cpp.
|
virtual |
Definition at line 51 of file TimeInstantLayer.cpp.
Member Function Documentation
|
overridevirtual |
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
The LayerGeometryProvider (an interface implemented by View) is provided here because it is possible for one layer to exist in more than one view, so the dimensions of the view may vary from one paint call to another (without any view having been resized).
Implements Layer.
Definition at line 317 of file TimeInstantLayer.cpp.
References PaintAssistant::drawVisibleText(), SingleColourLayer::getBaseQColor(), SingleColourLayer::getForegroundQColor(), LayerGeometryProvider::getFrameForX(), getLocalPoints(), getModel(), LayerGeometryProvider::getPaintHeight(), LayerGeometryProvider::getPaintWidth(), LayerGeometryProvider::getTextLabelYCoord(), LayerGeometryProvider::getView(), LayerGeometryProvider::getXForFrame(), m_model, m_plotStyle, PaintAssistant::OutlinedText, PlotInstants, PlotSegmentation, and LayerGeometryProvider::shouldIlluminateLocalFeatures().
|
overridevirtual |
|
overridevirtual |
Reimplemented from Layer.
Definition at line 241 of file TimeInstantLayer.cpp.
References getLocalPoints(), LayerGeometryProvider::getXForFrame(), and m_model.
|
overridevirtual |
Adjust the given frame to snap to the nearest feature, if possible.
If snap is SnapLeft or SnapRight, adjust the frame to match that of the nearest feature in the given direction regardless of how far away it is. If snap is SnapNeighbouring, adjust the frame to that of the nearest feature in either direction if it is close, and leave it alone (returning false) otherwise. SnapNeighbouring should always choose the same feature that would be used in an editing operation through calls to editStart etc.
If ycoord is non-negative, it contains the y coordinate at which the interaction that prompts this snap is taking place (e.g. of the mouse press used for a selection action). Layers that have objects at multiple different heights may choose to use this information. If the current action has no particular y coordinate associated with it, ycoord will be passed as -1.
Return true if a suitable feature was found and frame adjusted accordingly. Return false if no suitable feature was available (and leave frame unmodified). If returning true, also return the resolution of the model in this layer in sample frames.
Reimplemented from Layer.
Definition at line 278 of file TimeInstantLayer.cpp.
References getLocalPoints(), LayerGeometryProvider::getXForFrame(), m_model, Layer::SnapLeft, Layer::SnapNeighbouring, and Layer::snapToFeatureFrame().
|
overridevirtual |
Reimplemented from Layer.
Definition at line 504 of file TimeInstantLayer.cpp.
References finish(), LayerGeometryProvider::getFrameForX(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 527 of file TimeInstantLayer.cpp.
References LayerGeometryProvider::getFrameForX(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 545 of file TimeInstantLayer.cpp.
References finish(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 567 of file TimeInstantLayer.cpp.
References finish(), getLocalPoints(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 586 of file TimeInstantLayer.cpp.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 591 of file TimeInstantLayer.cpp.
References finish(), getLocalPoints(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 610 of file TimeInstantLayer.cpp.
References finish(), getLocalPoints(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 633 of file TimeInstantLayer.cpp.
References LayerGeometryProvider::getFrameForX(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 656 of file TimeInstantLayer.cpp.
References finish(), m_editing, m_editingCommand, m_editingPoint, and m_model.
|
overridevirtual |
Open an editor on the item under the mouse (e.g.
on double-click). If there is no item or editing is not supported, return false.
Reimplemented from Layer.
Definition at line 680 of file TimeInstantLayer.cpp.
References finish(), ItemEditDialog::getFrameTime(), getLocalPoints(), ItemEditDialog::getText(), m_model, ItemEditDialog::setFrameTime(), ItemEditDialog::setText(), ItemEditDialog::ShowText, and ItemEditDialog::ShowTime.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 716 of file TimeInstantLayer.cpp.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 738 of file TimeInstantLayer.cpp.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 767 of file TimeInstantLayer.cpp.
|
overridevirtual |
Reimplemented from Layer.
Definition at line 786 of file TimeInstantLayer.cpp.
References Layer::alignToReference(), and m_model.
|
overridevirtual |
Paste from the given clipboard onto the layer at the given frame offset.
If interactive is true, the layer may ask the user about paste options through a dialog if desired, and may return false if the user cancelled the paste operation. This function should return true if a paste actually occurred.
Reimplemented from Layer.
Definition at line 800 of file TimeInstantLayer.cpp.
References Layer::alignFromReference(), Layer::clipboardHasDifferentAlignment(), finish(), LayerGeometryProvider::getView(), and m_model.
|
inlineoverridevirtual |
Return the ID of the model represented in this layer.
Implements Layer.
Definition at line 67 of file TimeInstantLayer.h.
References getProperties(), getPropertyLabel(), getPropertyRangeAndValue(), getPropertyType(), getPropertyValueLabel(), m_model, setModel(), and setProperty().
Referenced by paint().
void TimeInstantLayer::setModel | ( | ModelId | model | ) |
Definition at line 64 of file TimeInstantLayer.cpp.
References Layer::connectSignals(), m_model, Layer::modelReplaced(), PlotSegmentation, and setPlotStyle().
Referenced by getModel().
|
override |
Definition at line 85 of file TimeInstantLayer.cpp.
References SingleColourLayer::getProperties().
Referenced by getModel().
|
override |
Definition at line 93 of file TimeInstantLayer.cpp.
References SingleColourLayer::getPropertyLabel().
Referenced by getModel().
|
override |
Definition at line 100 of file TimeInstantLayer.cpp.
References SingleColourLayer::getPropertyType().
Referenced by getModel().
|
override |
Definition at line 107 of file TimeInstantLayer.cpp.
References SingleColourLayer::getPropertyRangeAndValue(), and m_plotStyle.
Referenced by getModel().
|
override |
Definition at line 129 of file TimeInstantLayer.cpp.
References SingleColourLayer::getPropertyValueLabel().
Referenced by getModel().
|
override |
Definition at line 143 of file TimeInstantLayer.cpp.
References setPlotStyle(), and SingleColourLayer::setProperty().
Referenced by getModel().
void TimeInstantLayer::setPlotStyle | ( | PlotStyle | style | ) |
Definition at line 153 of file TimeInstantLayer.cpp.
References Layer::layerParametersChanged(), and m_plotStyle.
Referenced by setModel(), setProperties(), and setProperty().
|
inline |
Definition at line 85 of file TimeInstantLayer.h.
References isLayerScrollable(), and m_plotStyle.
|
overridevirtual |
This should return true if the layer can safely be scrolled automatically by a given view (simply copying the existing data and then refreshing the exposed area) without altering its meaning.
For the view widget as a whole this is usually not possible because of invariant (non-scrolling) material displayed over the top, but the widget may be able to optimise scrolling better if it is known that individual views can be scrolled safely in this way.
Reimplemented from Layer.
Definition at line 169 of file TimeInstantLayer.cpp.
References LayerGeometryProvider::shouldIlluminateLocalFeatures().
Referenced by getPlotStyle().
|
inlineoverridevirtual |
This should return true if the layer can be edited by the user.
If this is the case, the appropriate edit tools may be made available by the application and the layer's drawStart/Drag/End and editStart/Drag/End methods should be implemented.
Reimplemented from Layer.
Definition at line 89 of file TimeInstantLayer.h.
References getCompletion(), and needsTextLabelHeight().
|
overridevirtual |
Return the proportion of background work complete in drawing this view, as a percentage – in most cases this will be the value returned by pointer from a call to the underlying model's isReady(int *) call.
The view may choose to show a progress meter if it finds that this returns < 100 at any given moment.
Reimplemented from Layer.
Definition at line 56 of file TimeInstantLayer.cpp.
References m_model.
Referenced by isLayerEditable().
|
overridevirtual |
True if this layer will need to place text labels when it is painted.
The view will take into account how many layers are requesting this, and will provide a distinct y-coord to each layer on request via View::getTextLabelHeight().
Reimplemented from Layer.
Definition at line 161 of file TimeInstantLayer.cpp.
References m_model.
Referenced by isLayerEditable().
|
inlineoverridevirtual |
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether the layer is configured to use a logarithmic y axis display.
Also return the unit for these values if known.
This function returns the "normal" extents for the layer, not necessarily the extents actually in use in the display (see getDisplayExtents).
Implements Layer.
Definition at line 95 of file TimeInstantLayer.h.
References setProperties(), and toXml().
|
override |
Definition at line 877 of file TimeInstantLayer.cpp.
References m_plotStyle, and SingleColourLayer::toXml().
Referenced by getValueExtents().
|
overridevirtual |
Set the particular properties of a layer (those specific to the subclass) from a set of XML attributes.
This is the effective inverse of the toXml method.
Reimplemented from SingleColourLayer.
Definition at line 887 of file TimeInstantLayer.cpp.
References setPlotStyle(), and SingleColourLayer::setProperties().
Referenced by getValueExtents().
|
inlineoverridevirtual |
Implements Layer::getLayerColourSignificance()
Reimplemented from SingleColourLayer.
Definition at line 104 of file TimeInstantLayer.h.
References Layer::ColourDistinguishes, Layer::ColourHasMeaningfulValue, m_plotStyle, and PlotSegmentation.
|
inlineoverridevirtual |
Implements Layer.
Definition at line 112 of file TimeInstantLayer.h.
References clipboardAlignmentDiffers(), getDefaultColourHint(), and getLocalPoints().
|
protected |
Definition at line 176 of file TimeInstantLayer.cpp.
References LayerGeometryProvider::getEndFrame(), LayerGeometryProvider::getFrameForX(), LayerGeometryProvider::getXForFrame(), m_model, and LayerGeometryProvider::scaleSize().
Referenced by editOpen(), editStart(), eraseEnd(), eraseStart(), getFeatureDescription(), getVerticalScaleWidth(), paint(), and snapToFeatureFrame().
|
overrideprotectedvirtual |
Reimplemented from SingleColourLayer.
Definition at line 869 of file TimeInstantLayer.cpp.
References ColourDatabase::getColourIndex(), and ColourDatabase::getInstance().
Referenced by getVerticalScaleWidth().
|
protected |
Referenced by getVerticalScaleWidth().
|
inlineprotected |
Definition at line 127 of file TimeInstantLayer.h.
References CommandHistory::addCommand(), and CommandHistory::getInstance().
Referenced by deleteSelection(), drawEnd(), drawStart(), editEnd(), editOpen(), editStart(), eraseEnd(), eraseStart(), moveSelection(), paste(), and resizeSelection().
|
virtualinherited |
Set the colour used to draw primary items in the layer.
The colour value is a colour database index as returned by ColourDatabase::getColourIndex().
Definition at line 220 of file SingleColourLayer.cpp.
References SingleColourLayer::flagBaseColourChanged(), Layer::layerParametersChanged(), SingleColourLayer::m_colour, SingleColourLayer::m_colourExplicitlySet, SingleColourLayer::refColor(), and SingleColourLayer::unrefColor().
Referenced by SingleColourLayer::setDefaultColourFor(), and SingleColourLayer::setProperty().
|
virtualinherited |
Retrieve the current primary drawing colour, as a ColourDatabase index value.
Definition at line 235 of file SingleColourLayer.cpp.
References SingleColourLayer::m_colour.
|
overridevirtualinherited |
Return true if the layer currently has a dark colour on a light background, false if it has a light colour on a dark background.
Reimplemented from Layer.
Reimplemented in TimeValueLayer, and SliceLayer.
Definition at line 53 of file SingleColourLayer.cpp.
References ColourDatabase::getInstance(), SingleColourLayer::m_colour, and ColourDatabase::useDarkBackground().
Referenced by SliceLayer::hasLightBackground(), and TimeValueLayer::hasLightBackground().
|
overridevirtualinherited |
Reimplemented from Layer.
Definition at line 47 of file SingleColourLayer.cpp.
References ColourDatabase::getExamplePixmap(), ColourDatabase::getInstance(), and SingleColourLayer::m_colour.
Referenced by SingleColourLayer::getLayerColourSignificance().
|
overrideinherited |
Definition at line 82 of file SingleColourLayer.cpp.
Referenced by SingleColourLayer::getLayerColourSignificance(), SliceLayer::getPropertyGroupName(), BoxLayer::getPropertyGroupName(), TimeValueLayer::getPropertyGroupName(), RegionLayer::getPropertyGroupName(), NoteLayer::getPropertyGroupName(), and FlexiNoteLayer::getPropertyGroupName().
|
overrideinherited |
Definition at line 126 of file SingleColourLayer.cpp.
Referenced by SingleColourLayer::getLayerColourSignificance(), and SliceLayer::getNewPropertyRangeMapper().
|
virtualinherited |
Definition at line 140 of file SingleColourLayer.cpp.
References ColourDatabase::getColourCount(), SingleColourLayer::getDefaultColourHint(), ColourDatabase::getInstance(), LayerGeometryProvider::hasLightBackground(), SingleColourLayer::m_colour, SingleColourLayer::m_colourExplicitlySet, SingleColourLayer::m_colourRefCount, SingleColourLayer::m_defaultColourSet, SingleColourLayer::refColor(), SingleColourLayer::setBaseColour(), SingleColourLayer::unrefColor(), and ColourDatabase::useDarkBackground().
Referenced by View::addLayer(), SingleColourLayer::getLayerColourSignificance(), and SingleColourLayer::SingleColourLayer().
|
protectedvirtualinherited |
Definition at line 241 of file SingleColourLayer.cpp.
References ColourDatabase::getColour(), ColourDatabase::getInstance(), and SingleColourLayer::m_colour.
Referenced by SingleColourLayer::getLayerColourSignificance(), SingleColourLayer::getPartialShades(), TextLayer::paint(), TimeRulerLayer::paint(), paint(), BoxLayer::paint(), FlexiNoteLayer::paint(), SliceLayer::paint(), TimeValueLayer::paint(), RegionLayer::paint(), NoteLayer::paint(), and WaveformLayer::paintChannel().
|
protectedvirtualinherited |
Definition at line 247 of file SingleColourLayer.cpp.
References LayerGeometryProvider::getBackground().
Referenced by SingleColourLayer::getLayerColourSignificance(), SingleColourLayer::getPartialShades(), and WaveformLayer::paint().
|
protectedvirtualinherited |
Definition at line 253 of file SingleColourLayer.cpp.
References LayerGeometryProvider::getForeground().
Referenced by SingleColourLayer::getLayerColourSignificance(), paint(), TimeValueLayer::paint(), WaveformLayer::paint(), and RegionLayer::paint().
|
protectedinherited |
Definition at line 259 of file SingleColourLayer.cpp.
References SingleColourLayer::getBackgroundQColor(), and SingleColourLayer::getBaseQColor().
Referenced by SingleColourLayer::getLayerColourSignificance(), TimeRulerLayer::paint(), and TimeValueLayer::paint().
|
inlineprotectedvirtualinherited |
Reimplemented in WaveformLayer.
Definition at line 85 of file SingleColourLayer.h.
Referenced by SingleColourLayer::setBaseColour(), and SingleColourLayer::setProperties().
|
inherited |
Return the ID of the source model for the model represented in this layer.
If the model has no other source, or there is no model here, return None.
Definition at line 68 of file Layer.cpp.
References Layer::getModel().
Referenced by View::paintEvent().
|
inlinevirtualinherited |
Return the ID of a model representing the contents of this layer in a form suitable for export to a tabular file format such as CSV.
In most cases this will be the same as returned by getModel(). The exceptions are those layers such as SpectrogramLayer, that are "only" alternative views of time-domain sample data. For such layers, getModel() will return the backing time-domain data, for example as a ReadOnlyWaveFileModel; but getExportModel() will return a model, possibly "local to" the layer, which adapts this into the form shown in the layer for a given view so that the export matches the layer's visible contents.
Because this is supposed to match the contents of the view rather than the backing model, it's necessary to pass in a view (or LayerGeometryProvider) so that the layer can retrieve its vertical extents for export.
Reimplemented in SpectrogramLayer, and Colour3DPlotLayer.
Definition at line 95 of file Layer.h.
References Layer::getModel().
|
inlinevirtualinherited |
Return a zoom constraint object defining the supported zoom levels for this layer.
If this returns zero, the layer will support any integer zoom level.
Reimplemented in SpectrogramLayer, Colour3DPlotLayer, and WaveformLayer.
|
inlinevirtualinherited |
Return true if this layer can handle zoom levels other than those supported by its zoom constraint (presumably less efficiently or accurately than the officially supported zoom levels).
If true, the layer will unenthusistically accept any integer zoom level from 1 to the maximum returned by its zoom constraint.
Definition at line 114 of file Layer.h.
References Layer::paint().
|
inlinevirtualinherited |
Enable or disable synchronous painting.
If synchronous painting is enabled, a call to paint() must complete painting the entire rectangle before it returns. If synchronous painting is disabled (which should be the default), the paint() call may defer painting some regions if data is not yet available, by calling back on its view to schedule another update. Synchronous painting is necessary when rendering to an image. Simple layer types will always paint synchronously, and so may ignore this.
Reimplemented in SpectrogramLayer, and Colour3DPlotLayer.
|
inlinevirtualinherited |
Definition at line 143 of file Layer.h.
References Layer::PositionMiddle.
|
inlinevirtualinherited |
Reimplemented in SpectrogramLayer, and SpectrumLayer.
Definition at line 146 of file Layer.h.
References Layer::PositionBottom.
|
overrideinherited |
Definition at line 80 of file Layer.cpp.
References LayerFactory::getInstance(), and LayerFactory::getLayerIconName().
Referenced by LayerTreeModel::data(), and Layer::hasLightBackground().
|
inlineoverrideinherited |
Definition at line 155 of file Layer.h.
References Layer::getLayerPresentationName(), Layer::isPresentationNameSet(), Layer::m_presentationName, and Layer::setPresentationName().
|
virtualinherited |
Definition at line 87 of file Layer.cpp.
References Layer::m_presentationName.
Referenced by Layer::getPropertyContainerName().
|
virtualinherited |
Definition at line 93 of file Layer.cpp.
References Layer::m_presentationName.
Referenced by Layer::getPropertyContainerName().
|
virtualinherited |
Reimplemented in TimeRulerLayer.
Definition at line 99 of file Layer.cpp.
References LayerFactory::getInstance(), LayerFactory::getLayerPresentationName(), LayerFactory::getLayerType(), Layer::getModel(), and Layer::m_presentationName.
Referenced by Pane::drawVerticalScale(), FlexiNoteLayer::getAssociatedPitchModel(), Layer::getPropertyContainerName(), View::getScaleProvidingLayerForUnit(), and PropertyStack::repopulate().
|
inlinevirtualinherited |
Reimplemented in SpectrogramLayer, Colour3DPlotLayer, NoteLayer, WaveformLayer, RegionLayer, SliceLayer, TimeValueLayer, BoxLayer, and FlexiNoteLayer.
Definition at line 170 of file Layer.h.
Referenced by Pane::drawVerticalScale(), and Pane::render().
|
inlinevirtualinherited |
Reimplemented in SpectrumLayer.
Definition at line 173 of file Layer.h.
Referenced by SliceLayer::paint(), and SliceLayer::paintVerticalScale().
|
inlinevirtualinherited |
Reimplemented in SpectrogramLayer, and SpectrumLayer.
|
inlinevirtualinherited |
Reimplemented in SpectrogramLayer, and SpectrumLayer.
Definition at line 179 of file Layer.h.
References Layer::nearestMeasurementRectChanged(), and Layer::paintMeasurementRects().
|
virtualinherited |
Definition at line 464 of file Layer.cpp.
References Layer::findFocusedMeasureRect(), Layer::m_currentMeasureRectPoint, Layer::m_draggingRect, Layer::m_haveCurrentMeasureRect, Layer::m_haveDraggingRect, Layer::m_measureRects, Layer::paintMeasurementRect(), and Layer::updateMeasurePixrects().
Referenced by Layer::paintCrosshairs(), and Pane::paintEvent().
|
virtualinherited |
Definition at line 496 of file Layer.cpp.
References Layer::findFocusedMeasureRect(), and Layer::updateMeasurePixrects().
Referenced by Pane::mouseMoveEvent(), and Layer::paintCrosshairs().
|
inlinevirtualinherited |
Adjust the given frame to snap to the next feature that has "effectively" the same value as the feature prior to the given frame, if possible.
The snap type must be SnapLeft (snap to the time of the next feature prior to the one preceding the given frame that has a similar value to it) or SnapRight (snap to the time of the next feature following the given frame that has a similar value to the feature preceding it). Other values are not permitted.
Return true if a suitable feature was found and frame adjusted accordingly. Return false if no suitable feature was available (and leave frame unmodified). If returning true, also return the resolution of the model in this layer in sample frames.
Reimplemented in RegionLayer, and TimeValueLayer.
Definition at line 251 of file Layer.h.
Referenced by TimeValueLayer::snapToSimilarFeature(), and RegionLayer::snapToSimilarFeature().
|
inlinevirtualinherited |
Reimplemented in FlexiNoteLayer.
Definition at line 276 of file Layer.h.
Referenced by Pane::mousePressEvent().
|
inlinevirtualinherited |
Reimplemented in FlexiNoteLayer.
Definition at line 277 of file Layer.h.
Referenced by Pane::mouseReleaseEvent().
|
inlinevirtualinherited |
Reimplemented in FlexiNoteLayer.
Definition at line 278 of file Layer.h.
References Layer::measureDoubleClick(), Layer::measureDrag(), Layer::measureEnd(), and Layer::measureStart().
Referenced by Pane::mouseDoubleClickEvent().
|
virtualinherited |
Definition at line 390 of file Layer.cpp.
References Layer::MeasureRect::endFrame, LayerGeometryProvider::getFrameForX(), Layer::hasTimeXAxis(), Layer::MeasureRect::haveFrames, Layer::m_draggingRect, Layer::m_haveDraggingRect, Layer::MeasureRect::pixrect, Layer::setMeasureRectYCoord(), and Layer::MeasureRect::startFrame.
Referenced by Layer::addNote(), and Pane::mousePressEvent().
|
virtualinherited |
Definition at line 414 of file Layer.cpp.
References Layer::MeasureRect::endFrame, LayerGeometryProvider::getFrameForX(), Layer::MeasureRect::haveFrames, Layer::m_draggingRect, Layer::m_haveDraggingRect, Layer::MeasureRect::pixrect, and Layer::setMeasureRectYCoord().
Referenced by Layer::addNote(), Layer::measureEnd(), and Pane::mouseMoveEvent().
|
virtualinherited |
Definition at line 430 of file Layer.cpp.
References CommandHistory::addCommand(), CommandHistory::getInstance(), Layer::m_draggingRect, Layer::m_haveDraggingRect, Layer::measureDrag(), and Layer::MeasureRect::pixrect.
Referenced by Layer::addNote(), and Pane::mouseReleaseEvent().
|
virtualinherited |
Reimplemented in SpectrogramLayer.
Definition at line 444 of file Layer.cpp.
Referenced by Layer::addNote(), and Pane::mouseDoubleClickEvent().
|
inlinevirtualinherited |
Definition at line 289 of file Layer.h.
References Layer::deleteCurrentMeasureRect(), and Layer::m_haveCurrentMeasureRect.
|
virtualinherited |
Definition at line 450 of file Layer.cpp.
References CommandHistory::addCommand(), Layer::findFocusedMeasureRect(), CommandHistory::getInstance(), Layer::m_currentMeasureRectPoint, Layer::m_haveCurrentMeasureRect, and Layer::m_measureRects.
Referenced by Layer::haveCurrentMeasureRect().
|
inlinevirtualinherited |
This should return true if the layer completely obscures any underlying layers.
It's used to determine whether the view can safely draw any selection rectangles under the layer instead of over it, in the case where the layer is not scrollable and therefore needs to be redrawn each time (so that the selection rectangle can be cached).
Reimplemented in SpectrogramLayer.
Definition at line 346 of file Layer.h.
Referenced by Pane::drawVerticalScale().
|
inlinevirtualinherited |
Return an error string if any errors have occurred while loading or processing data for the given view.
Return the empty string if no error has occurred.
Reimplemented in SpectrogramLayer.
Definition at line 398 of file Layer.h.
References Layer::addMeasurementRect(), Layer::getPlayParameters(), Layer::isLayerDormant(), Layer::setLayerDormant(), Layer::setObjectName(), Layer::setProperties(), Layer::toBriefXml(), and Layer::toXml().
|
virtualinherited |
Definition at line 122 of file Layer.cpp.
References Layer::layerNameChanged().
Referenced by LayerFactory::createLayer(), and Layer::getError().
|
virtualinherited |
Produce XML containing the layer's ID and type.
This is used to refer to the layer in the display section of the SV session file, for a layer that has already been described in the data section.
Definition at line 691 of file Layer.cpp.
References LayerFactory::getInstance(), Layer::getModel(), and Layer::m_presentationName.
Referenced by Layer::getError().
|
virtualinherited |
Add a measurement rectangle from the given XML attributes (presumably taken from a measurement element).
Does not use a command.
Definition at line 333 of file Layer.cpp.
References Layer::addMeasureRectToSet(), Layer::MeasureRect::endFrame, Layer::MeasureRect::endY, Layer::MeasureRect::haveFrames, Layer::MeasureRect::pixrect, Layer::MeasureRect::startFrame, and Layer::MeasureRect::startY.
Referenced by Layer::getError().
|
virtualinherited |
Indicate that a layer is not currently visible in the given view and is not expected to become visible in the near future (for example because the user has explicitly removed or hidden it).
The layer may respond by (for example) freeing any cache memory it is using, until next time its paint method is called, when it should set itself un-dormant again.
A layer class that overrides this function must also call this class's implementation.
Reimplemented in SpectrogramLayer, ImageLayer, and Colour3DPlotLayer.
Definition at line 136 of file Layer.cpp.
References Layer::m_dormancy, and Layer::m_dormancyMutex.
Referenced by Layer::getError(), Colour3DPlotLayer::setLayerDormant(), SpectrogramLayer::setLayerDormant(), and Layer::showLayer().
|
virtualinherited |
Return whether the layer is dormant (i.e.
hidden) in the given view.
Definition at line 144 of file Layer.cpp.
References Layer::m_dormancy, and Layer::m_dormancyMutex.
Referenced by LayerTreeModel::data(), Pane::drawVerticalScale(), Layer::getError(), View::getInteractionLayer(), View::getScaleProvidingLayerForUnit(), View::getVisibleExtentsForAnyUnit(), PropertyStack::propertyContainerPropertyChanged(), PropertyStack::repopulate(), Colour3DPlotLayer::setLayerDormant(), and SpectrogramLayer::setLayerDormant().
|
overrideinherited |
Return the play parameters for this layer, if any.
The return value is a shared_ptr that can be passed to (e.g.) PlayParameterRepository::EditCommand to change the parameters.
Definition at line 129 of file Layer.cpp.
References Layer::getModel().
Referenced by LayerTreeModel::data(), Layer::getError(), LayerTreeModel::LayerTreeModel(), LayerTreeModel::playParametersAudibilityChanged(), and LayerTreeModel::setData().
|
inlinevirtualinherited |
Return true if the X axis on the layer is time proportional to audio frames, false otherwise.
Almost all layer types return true here: the exceptions are spectrum and slice layers.
Reimplemented in SliceLayer.
Definition at line 475 of file Layer.h.
Referenced by Layer::getXScaleValue(), Layer::measureStart(), Pane::mouseMoveEvent(), Layer::setMeasureRectFromPixrect(), and Pane::zoomToRegion().
|
inlinevirtualinherited |
Update the X and Y axis scales, where appropriate, to focus on the given rectangular region.
This should only be overridden by layers whose hasTimeXAxis() returns false - the pane handles zooming appropriately in every "normal" case.
Reimplemented in SliceLayer.
Definition at line 483 of file Layer.h.
References Layer::getValueExtents().
Referenced by Pane::zoomToRegion().
|
inlinevirtualinherited |
Return the minimum and maximum values within the visible area for the y axis of this layer.
Return false if the layer has no display extents of its own. This could be because the layer is "auto-aligning" against another layer with the same units elsewhere in the view, or because the layer has no concept of a vertical scale at all.
Reimplemented in SpectrogramLayer, WaveformLayer, Colour3DPlotLayer, TimeValueLayer, FlexiNoteLayer, RegionLayer, NoteLayer, BoxLayer, and SliceLayer.
Definition at line 509 of file Layer.h.
Referenced by View::getScaleProvidingLayerForUnit(), Pane::getTopLayerDisplayExtents(), View::getVisibleExtentsForAnyUnit(), and View::getVisibleExtentsForUnit().
|
inlinevirtualinherited |
Set the displayed minimum and maximum values for the y axis to the given range, if supported.
Return false if not supported on this layer (and set nothing). In most cases, layers that return false for getDisplayExtents should also return false for this function.
Reimplemented in SpectrogramLayer, Colour3DPlotLayer, TimeValueLayer, FlexiNoteLayer, NoteLayer, SpectrumLayer, and SliceLayer.
Definition at line 521 of file Layer.h.
Referenced by Pane::setTopLayerDisplayExtents(), FlexiNoteLayer::setVerticalRangeToNoteRange(), and Pane::zoomToRegion().
|
inlinevirtualinherited |
Consider using the given value extents and units for this layer.
This may be called on a new layer when added, to prepare it for editing, and the extents are those of the layer underneath it. May not be appropriate for most layer types.
Reimplemented in BoxLayer.
Definition at line 532 of file Layer.h.
References Layer::getXScaleValue().
|
virtualinherited |
Return the value and unit at the given x coordinate in the given view.
This is for descriptive purposes using the measurement tool. The default implementation works correctly if the layer hasTimeXAxis().
Reimplemented in SpectrumLayer.
Definition at line 160 of file Layer.cpp.
References LayerGeometryProvider::getFrameForX(), Layer::getModel(), and Layer::hasTimeXAxis().
Referenced by Layer::adoptExtents(), and View::drawMeasurementRect().
|
inlinevirtualinherited |
Return the value and unit at the given y coordinate in the given view.
Reimplemented in SpectrogramLayer, WaveformLayer, Colour3DPlotLayer, and SpectrumLayer.
Definition at line 550 of file Layer.h.
References Layer::getYScaleDifference().
Referenced by View::drawMeasurementRect(), and Layer::getYScaleDifference().
|
virtualinherited |
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
The default implementation just calls getYScaleValue twice and returns the difference, with the same unit.
Reimplemented in WaveformLayer, and SpectrumLayer.
Definition at line 173 of file Layer.cpp.
References Layer::getYScaleValue().
Referenced by View::drawMeasurementRect(), SpectrumLayer::getYScaleDifference(), and Layer::getYScaleValue().
|
inlinevirtualinherited |
Get the number of vertical zoom steps available for this layer.
If vertical zooming is not available, return 0. The meaning of "zooming" is entirely up to the layer – changing the zoom level may cause the layer to reset its display extents or change another property such as display gain. However, layers are advised for consistency to treat smaller zoom steps as "more distant" or "zoomed out" and larger ones as "closer" or "zoomed in".
Layers that provide this facility should also emit the verticalZoomChanged signal if their vertical zoom changes due to factors other than setVerticalZoomStep being called.
Reimplemented in SpectrogramLayer, WaveformLayer, Colour3DPlotLayer, TimeValueLayer, FlexiNoteLayer, NoteLayer, and SliceLayer.
Definition at line 578 of file Layer.h.
Referenced by Pane::updateHeadsUpDisplay(), Pane::updateVerticalPanner(), and Pane::verticalThumbwheelMoved().
|
inlinevirtualinherited |
Get the current vertical zoom step.
A layer may support finer control over ranges etc than is available through the integer zoom step mechanism; if this one does, it should just return the nearest of the available zoom steps to the current settings.
Reimplemented in SpectrogramLayer, WaveformLayer, Colour3DPlotLayer, TimeValueLayer, FlexiNoteLayer, NoteLayer, and SliceLayer.
Definition at line 586 of file Layer.h.
Referenced by Pane::updateHeadsUpDisplay(), and Pane::verticalZoomChanged().
|
inlinevirtualinherited |
Set the vertical zoom step.
The meaning of "zooming" is entirely up to the layer – changing the zoom level may cause the layer to reset its display extents or change another property such as display gain.
Reimplemented in SpectrogramLayer, WaveformLayer, Colour3DPlotLayer, TimeValueLayer, FlexiNoteLayer, NoteLayer, and SliceLayer.
Definition at line 594 of file Layer.h.
Referenced by Pane::verticalThumbwheelMoved().
|
inlinevirtualinherited |
Create and return a range mapper for vertical zoom step values.
See the RangeMapper documentation for more details. The returned value is allocated on the heap and will be deleted by the caller.
Reimplemented in SpectrogramLayer, Colour3DPlotLayer, TimeValueLayer, FlexiNoteLayer, NoteLayer, and SliceLayer.
Definition at line 602 of file Layer.h.
Referenced by Pane::propertyContainerSelected(), and Pane::updateHeadsUpDisplay().
|
inlinevirtualinherited |
Return true if this layer type can function without a model being set.
If false (the default), the layer will not be loaded from a session if its model cannot be found.
Reimplemented in WaveformLayer, and TimeRulerLayer.
Definition at line 609 of file Layer.h.
References Layer::alignFromReference(), Layer::alignToReference(), Layer::clipboardHasDifferentAlignment(), Layer::connectSignals(), Layer::layerMeasurementRectsChanged(), Layer::layerNameChanged(), Layer::layerParameterRangesChanged(), Layer::layerParametersChanged(), Layer::modelAlignmentCompletionChanged(), Layer::modelChanged(), Layer::modelChangedWithin(), Layer::modelCompletionChanged(), Layer::modelReplaced(), Layer::showLayer(), and Layer::verticalZoomChanged().
|
slotinherited |
Change the visibility status (dormancy) of the layer in the given view.
Definition at line 153 of file Layer.cpp.
References Layer::layerParametersChanged(), and Layer::setLayerDormant().
Referenced by Layer::canExistWithoutModel(), ShowLayerCommand::execute(), LayerTreeModel::setData(), and ShowLayerCommand::unexecute().
|
signalinherited |
Referenced by Layer::canExistWithoutModel(), Layer::connectSignals(), ImageLayer::fileSourceReady(), Colour3DPlotLayer::handleModelChanged(), RegionLayer::setModel(), Colour3DPlotLayer::setModel(), SpectrogramLayer::setModel(), BoxLayer::setProperty(), TimeValueLayer::setProperty(), RegionLayer::setProperty(), NoteLayer::setProperty(), and FlexiNoteLayer::setProperty().
|
signalinherited |
Referenced by Layer::canExistWithoutModel(), and Layer::connectSignals().
|
signalinherited |
Referenced by Layer::canExistWithoutModel(), and Layer::connectSignals().
|
signalinherited |
|
signalinherited |
Referenced by Layer::canExistWithoutModel(), TimeRulerLayer::setModel(), WaveformLayer::setModel(), TextLayer::setModel(), ImageLayer::setModel(), setModel(), BoxLayer::setModel(), TimeValueLayer::setModel(), Colour3DPlotLayer::setModel(), RegionLayer::setModel(), NoteLayer::setModel(), FlexiNoteLayer::setModel(), SpectrogramLayer::setModel(), and SliceLayer::setSliceableModel().
|
signalinherited |
Referenced by Layer::canExistWithoutModel(), SpectrogramLayer::preferenceChanged(), WaveformLayer::setAggressiveCacheing(), WaveformLayer::setAutoNormalize(), SingleColourLayer::setBaseColour(), SpectrogramLayer::setBinDisplay(), SliceLayer::setBinScale(), Colour3DPlotLayer::setBinScale(), SpectrogramLayer::setBinScale(), SpectrumLayer::setChannel(), SpectrogramLayer::setChannel(), WaveformLayer::setChannel(), WaveformLayer::setChannelMode(), Colour3DPlotLayer::setColourMap(), SpectrogramLayer::setColourMap(), SpectrogramLayer::setColourRotation(), Colour3DPlotLayer::setColourScale(), SpectrogramLayer::setColourScale(), SpectrogramLayer::setColourScaleMultiple(), SliceLayer::setDisplayExtents(), NoteLayer::setDisplayExtents(), FlexiNoteLayer::setDisplayExtents(), TimeValueLayer::setDisplayExtents(), Colour3DPlotLayer::setDisplayExtents(), SpectrogramLayer::setDisplayExtents(), TimeValueLayer::setDrawSegmentDivisions(), SliceLayer::setEnergyScale(), TimeValueLayer::setFillColourMap(), RegionLayer::setFillColourMap(), SliceLayer::setFillColourMap(), WaveformLayer::setGain(), Colour3DPlotLayer::setGain(), SliceLayer::setGain(), SpectrogramLayer::setGain(), Colour3DPlotLayer::setInvertVertical(), SpectrogramLayer::setMaxFrequency(), WaveformLayer::setMiddleLineHeight(), SpectrogramLayer::setMinFrequency(), SpectrumLayer::setModel(), WaveformLayer::setModel(), Colour3DPlotLayer::setNormalization(), SpectrogramLayer::setNormalization(), SliceLayer::setNormalize(), Colour3DPlotLayer::setNormalizeVisibleArea(), SpectrogramLayer::setNormalizeVisibleArea(), Colour3DPlotLayer::setOpaque(), SpectrumLayer::setOversampling(), SpectrogramLayer::setOversampling(), setPlotStyle(), SliceLayer::setPlotStyle(), TimeValueLayer::setPlotStyle(), RegionLayer::setPlotStyle(), SliceLayer::setSamplingMode(), WaveformLayer::setScale(), TimeValueLayer::setShowDerivative(), WaveformLayer::setShowMeans(), SpectrumLayer::setShowPeaks(), SliceLayer::setSliceableModel(), Colour3DPlotLayer::setSmooth(), SliceLayer::setThreshold(), SpectrogramLayer::setThreshold(), BoxLayer::setVerticalScale(), NoteLayer::setVerticalScale(), RegionLayer::setVerticalScale(), FlexiNoteLayer::setVerticalScale(), TimeValueLayer::setVerticalScale(), Colour3DPlotLayer::setVerticalZoomStep(), SpectrumLayer::setWindowHopLevel(), SpectrogramLayer::setWindowHopLevel(), SpectrumLayer::setWindowSize(), SpectrogramLayer::setWindowSize(), SpectrumLayer::setWindowType(), SpectrogramLayer::setWindowType(), and Layer::showLayer().
|
signalinherited |
|
signalinherited |
Referenced by Layer::addMeasureRectToSet(), Layer::canExistWithoutModel(), and Layer::deleteMeasureRectFromSet().
|
signalinherited |
Referenced by Layer::canExistWithoutModel(), and Layer::setObjectName().
|
signalinherited |
|
protectedinherited |
Definition at line 49 of file Layer.cpp.
References Layer::modelAlignmentCompletionChanged(), Layer::modelChanged(), Layer::modelChangedWithin(), and Layer::modelCompletionChanged().
Referenced by Layer::canExistWithoutModel(), WaveformLayer::setModel(), TextLayer::setModel(), ImageLayer::setModel(), setModel(), BoxLayer::setModel(), TimeValueLayer::setModel(), Colour3DPlotLayer::setModel(), RegionLayer::setModel(), NoteLayer::setModel(), FlexiNoteLayer::setModel(), SpectrogramLayer::setModel(), and SliceLayer::setSliceableModel().
|
protectedvirtualinherited |
Definition at line 187 of file Layer.cpp.
References View::alignToReference(), Layer::getModel(), and LayerGeometryProvider::getView().
Referenced by Layer::canExistWithoutModel(), Layer::clipboardHasDifferentAlignment(), TextLayer::copy(), ImageLayer::copy(), copy(), BoxLayer::copy(), TimeValueLayer::copy(), RegionLayer::copy(), FlexiNoteLayer::copy(), and NoteLayer::copy().
|
protectedvirtualinherited |
Definition at line 198 of file Layer.cpp.
References View::alignFromReference(), Layer::getModel(), and LayerGeometryProvider::getView().
Referenced by Layer::canExistWithoutModel(), TextLayer::paste(), ImageLayer::paste(), paste(), BoxLayer::paste(), TimeValueLayer::paste(), RegionLayer::paste(), FlexiNoteLayer::paste(), and NoteLayer::paste().
|
protectedinherited |
Definition at line 209 of file Layer.cpp.
References Layer::alignToReference().
Referenced by Layer::canExistWithoutModel(), TextLayer::paste(), ImageLayer::paste(), paste(), BoxLayer::paste(), TimeValueLayer::paste(), RegionLayer::paste(), FlexiNoteLayer::paste(), and NoteLayer::paste().
|
inlineprotectedinherited |
Definition at line 682 of file Layer.h.
References Layer::layerMeasurementRectsChanged(), and Layer::m_measureRects.
Referenced by Layer::addMeasurementRect().
|
inlineprotectedinherited |
Definition at line 687 of file Layer.h.
References Layer::layerMeasurementRectsChanged(), and Layer::m_measureRects.
|
protectedinherited |
Definition at line 507 of file Layer.cpp.
References LayerGeometryProvider::getEndFrame(), LayerGeometryProvider::getStartFrame(), LayerGeometryProvider::getXForFrame(), Layer::m_measureRects, and Layer::updateMeasureRectYCoords().
Referenced by Layer::nearestMeasurementRectChanged(), and Layer::paintMeasurementRects().
|
protectedvirtualinherited |
Reimplemented in SpectrogramLayer.
Definition at line 546 of file Layer.cpp.
References Layer::MeasureRect::endY, LayerGeometryProvider::getPaintHeight(), Layer::MeasureRect::pixrect, and Layer::MeasureRect::startY.
Referenced by Layer::updateMeasurePixrects().
|
protectedvirtualinherited |
Reimplemented in SpectrogramLayer.
Definition at line 554 of file Layer.cpp.
References Layer::MeasureRect::endY, LayerGeometryProvider::getPaintHeight(), and Layer::MeasureRect::startY.
Referenced by Layer::measureDrag(), Layer::measureStart(), and Layer::setMeasureRectFromPixrect().
|
protectedvirtualinherited |
Definition at line 565 of file Layer.cpp.
References Layer::MeasureRect::endFrame, LayerGeometryProvider::getFrameForX(), Layer::hasTimeXAxis(), Layer::MeasureRect::haveFrames, Layer::MeasureRect::pixrect, Layer::setMeasureRectYCoord(), and Layer::MeasureRect::startFrame.
Referenced by SpectrogramLayer::measureDoubleClick().
|
protectedinherited |
Definition at line 578 of file Layer.cpp.
References Layer::m_measureRects.
Referenced by Layer::deleteCurrentMeasureRect(), Layer::nearestMeasurementRectChanged(), and Layer::paintMeasurementRects().
|
protectedinherited |
Definition at line 605 of file Layer.cpp.
References LayerGeometryProvider::drawMeasurementRect(), Layer::MeasureRect::endFrame, LayerGeometryProvider::getEndFrame(), LayerGeometryProvider::getPaintWidth(), LayerGeometryProvider::getStartFrame(), LayerGeometryProvider::getXForFrame(), Layer::MeasureRect::haveFrames, Layer::MeasureRect::pixrect, and Layer::MeasureRect::startFrame.
Referenced by Layer::paintMeasurementRects().
|
protectedinherited |
Definition at line 628 of file Layer.cpp.
References Layer::getValueExtents(), and LayerGeometryProvider::getVisibleExtentsForUnit().
Referenced by TimeValueLayer::getVerticalScaleWidth(), and NoteLayer::getVerticalScaleWidth().
Member Data Documentation
|
protected |
Definition at line 121 of file TimeInstantLayer.h.
Referenced by copy(), deleteSelection(), drawDrag(), drawEnd(), drawStart(), editDrag(), editEnd(), editOpen(), editStart(), eraseEnd(), eraseStart(), getCompletion(), getFeatureDescription(), getLabelPreceding(), getLocalPoints(), getModel(), moveSelection(), needsTextLabelHeight(), paint(), paste(), resizeSelection(), setModel(), and snapToFeatureFrame().
|
protected |
Definition at line 122 of file TimeInstantLayer.h.
Referenced by drawDrag(), drawEnd(), drawStart(), editDrag(), editEnd(), editStart(), eraseEnd(), and eraseStart().
|
protected |
Definition at line 123 of file TimeInstantLayer.h.
Referenced by drawDrag(), drawEnd(), drawStart(), editDrag(), editEnd(), editStart(), eraseEnd(), and eraseStart().
|
protected |
Definition at line 124 of file TimeInstantLayer.h.
Referenced by drawDrag(), drawEnd(), drawStart(), editDrag(), editEnd(), editStart(), eraseEnd(), and eraseStart().
|
protected |
Definition at line 125 of file TimeInstantLayer.h.
Referenced by getLayerColourSignificance(), getPlotStyle(), getPropertyRangeAndValue(), paint(), setPlotStyle(), and toXml().
|
staticprotectedinherited |
Definition at line 90 of file SingleColourLayer.h.
Referenced by SingleColourLayer::refColor(), SingleColourLayer::setDefaultColourFor(), and SingleColourLayer::unrefColor().
|
protectedinherited |
Definition at line 92 of file SingleColourLayer.h.
Referenced by SingleColourLayer::getBaseColour(), SingleColourLayer::getBaseQColor(), SingleColourLayer::getLayerPresentationPixmap(), SingleColourLayer::getPropertyRangeAndValue(), SingleColourLayer::hasLightBackground(), WaveformLayer::paintChannel(), SingleColourLayer::refColor(), SingleColourLayer::setBaseColour(), SingleColourLayer::setDefaultColourFor(), SingleColourLayer::setProperties(), SingleColourLayer::toXml(), WaveformLayer::toXml(), and SingleColourLayer::unrefColor().
|
protectedinherited |
Definition at line 93 of file SingleColourLayer.h.
Referenced by SingleColourLayer::setBaseColour(), SingleColourLayer::setDefaultColourFor(), and SingleColourLayer::setProperties().
|
protectedinherited |
Definition at line 94 of file SingleColourLayer.h.
Referenced by SingleColourLayer::setDefaultColourFor().
|
protectedinherited |
Definition at line 693 of file Layer.h.
Referenced by Layer::addMeasureRectToSet(), Layer::deleteCurrentMeasureRect(), Layer::deleteMeasureRectFromSet(), Layer::findFocusedMeasureRect(), Layer::paintMeasurementRects(), Layer::toXml(), and Layer::updateMeasurePixrects().
|
protectedinherited |
Definition at line 694 of file Layer.h.
Referenced by Layer::measureDrag(), Layer::measureEnd(), Layer::measureStart(), and Layer::paintMeasurementRects().
|
protectedinherited |
Definition at line 695 of file Layer.h.
Referenced by Layer::measureDrag(), Layer::measureEnd(), Layer::measureStart(), and Layer::paintMeasurementRects().
|
mutableprotectedinherited |
Definition at line 696 of file Layer.h.
Referenced by Layer::deleteCurrentMeasureRect(), Layer::haveCurrentMeasureRect(), and Layer::paintMeasurementRects().
|
mutableprotectedinherited |
Definition at line 697 of file Layer.h.
Referenced by Layer::deleteCurrentMeasureRect(), and Layer::paintMeasurementRects().
|
protectedinherited |
Definition at line 716 of file Layer.h.
Referenced by Layer::getLayerPresentationName(), Layer::getPropertyContainerName(), Layer::isPresentationNameSet(), Layer::setPresentationName(), Layer::toBriefXml(), and Layer::toXml().
The documentation for this class was generated from the following files:
Generated by
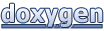