svgui
1.9
|
PropertyStack.cpp
Go to the documentation of this file.
107 SVDEBUG << "(" << i << " of " << m_boxes.size() << ": " << m_boxes[i]->getContainer()->getPropertyContainerName() << ")" << endl;
225 SVDEBUG << "PropertyStack::propertyContainerAdded(" << (c ? c->getPropertyContainerName() : "(none)") << ")" << endl;
239 SVDEBUG << "PropertyStack::propertyContainerAdded(" << (c ? c->getPropertyContainerName() : "(none)") << ")" << endl;
virtual bool isLayerDormant(const LayerGeometryProvider *v) const
Return whether the layer is dormant (i.e.
Definition: Layer.cpp:144
void tabBarContextMenuRequested(const QPoint &)
Definition: PropertyStack.cpp:172
void propertyContainerPropertyChanged(PropertyContainer *)
Definition: PropertyStack.cpp:245
virtual const PropertyContainer * getPropertyContainer(int i) const
Definition: View.cpp:183
void propertyContainerAdded(PropertyContainer *)
Definition: PropertyStack.cpp:217
Definition: PropertyBox.h:34
int getContainerIndex(PropertyContainer *container) const
Definition: PropertyStack.cpp:196
void viewSelected(View *client)
void addCommand(Command *command)
Add a command to the command history.
Definition: CommandHistory.cpp:135
void selectedContainerChanged(int)
Definition: PropertyStack.cpp:295
Definition: ShowLayerCommand.h:21
bool containsContainer(PropertyContainer *container) const
Definition: PropertyStack.cpp:185
PropertyStack(QWidget *parent, View *client)
Definition: PropertyStack.cpp:37
Definition: IconLoader.h:21
QString getLayerPresentationName(LayerType type)
Definition: LayerFactory.cpp:70
virtual QString getLayerPresentationName() const
Definition: Layer.cpp:99
void contextHelpChanged(const QString &)
void propertyContainerRemoved(PropertyContainer *)
Definition: PropertyStack.cpp:231
void propertyContainerPropertyRangeChanged(PropertyContainer *)
Definition: PropertyStack.cpp:260
virtual int getPropertyContainerCount() const
Definition: View.cpp:177
void propertyContainerNameChanged(PropertyContainer *)
Definition: PropertyStack.cpp:270
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
void propertyContainerContextMenuRequested(View *client, PropertyContainer *container, QPoint pos)
void propertyContainerSelected(View *client, PropertyContainer *container)
Definition: NotifyingTabBar.h:21
Generated by
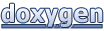