svgui
1.9
|
PropertyBox.h
Go to the documentation of this file.
void propertyContainerPropertyChanged(PropertyContainer *)
Definition: PropertyBox.cpp:591
Very trivial enhancement to QPushButton to make it emit signals when the mouse enters and leaves (for...
Definition: NotifyingPushButton.h:27
std::map< QString, QWidget * > m_propertyControllers
Definition: PropertyBox.h:91
Definition: PropertyBox.h:34
void propertyControllerChanged(int)
Definition: PropertyBox.cpp:654
void playGainControlChanged(float)
Definition: PropertyBox.cpp:764
void updatePropertyEditor(PropertyContainer::PropertyName, bool rangeChanged=false)
Definition: PropertyBox.cpp:239
void contextHelpChanged(const QString &)
void showLayer(bool)
void propertyContainerPropertyRangeChanged(PropertyContainer *)
Definition: PropertyBox.cpp:612
void propertyControllerResetRequested()
Definition: PropertyBox.cpp:726
std::map< QString, QGridLayout * > m_groupLayouts
Definition: PropertyBox.h:90
void contextMenuRequested(const QPoint &)
Definition: PropertyBox.cpp:688
Definition: LEDButton.h:36
void playAudibleButtonChanged(bool)
Definition: PropertyBox.cpp:750
Generated by
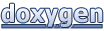