svgui
1.9
|
#include <LEDButton.h>
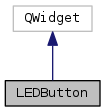
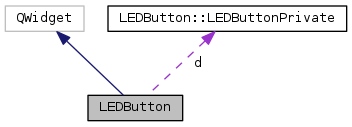
Classes | |
class | LEDButtonPrivate |
Public Slots | |
void | toggle () |
void | on () |
void | off () |
void | setState (bool) |
void | toggleState () |
void | setColor (const QColor &color) |
void | setDarkFactor (int darkfactor) |
Signals | |
void | stateChanged (bool) |
void | mouseEntered () |
void | mouseLeft () |
Public Member Functions | |
LEDButton (QWidget *parent=0) | |
LEDButton (const QColor &col, QWidget *parent=0) | |
LEDButton (const QColor &col, bool state, QWidget *parent=0) | |
~LEDButton () | |
bool | state () const |
QColor | color () const |
int | darkFactor () const |
QSize | sizeHint () const override |
QSize | minimumSizeHint () const override |
Protected Member Functions | |
void | paintEvent (QPaintEvent *) override |
void | mousePressEvent (QMouseEvent *) override |
void | enterEvent (QEvent *) override |
void | leaveEvent (QEvent *) override |
Protected Attributes | |
bool | led_state |
QColor | led_color |
LEDButtonPrivate * | d |
Properties | |
QColor | color |
int | darkFactor |
Detailed Description
Definition at line 36 of file LEDButton.h.
Constructor & Destructor Documentation
LEDButton::LEDButton | ( | QWidget * | parent = 0 | ) |
Definition at line 45 of file LEDButton.cpp.
References d, LEDButton::LEDButtonPrivate::dark_factor, LEDButton::LEDButtonPrivate::offcolor, and setColor().
LEDButton::LEDButton | ( | const QColor & | col, |
QWidget * | parent = 0 |
||
) |
Definition at line 58 of file LEDButton.cpp.
References d, LEDButton::LEDButtonPrivate::dark_factor, LEDButton::LEDButtonPrivate::offcolor, and setColor().
LEDButton::LEDButton | ( | const QColor & | col, |
bool | state, | ||
QWidget * | parent = 0 |
||
) |
Definition at line 69 of file LEDButton.cpp.
References d, LEDButton::LEDButtonPrivate::dark_factor, LEDButton::LEDButtonPrivate::offcolor, and setColor().
LEDButton::~LEDButton | ( | ) |
Definition at line 80 of file LEDButton.cpp.
References d.
Member Function Documentation
bool LEDButton::state | ( | ) | const |
Definition at line 207 of file LEDButton.cpp.
References color(), led_color, and led_state.
Referenced by mousePressEvent(), and setState().
QColor LEDButton::color | ( | ) | const |
Referenced by paintEvent(), and state().
int LEDButton::darkFactor | ( | ) | const |
Referenced by setDarkFactor().
|
override |
Definition at line 281 of file LEDButton.cpp.
References WidgetScale::scaleQSize().
|
override |
Definition at line 287 of file LEDButton.cpp.
References WidgetScale::scaleQSize().
|
signal |
Referenced by mousePressEvent().
|
signal |
Referenced by enterEvent().
|
signal |
Referenced by leaveEvent().
|
slot |
Definition at line 263 of file LEDButton.cpp.
References toggleState().
Referenced by mousePressEvent().
|
slot |
Definition at line 269 of file LEDButton.cpp.
References setState().
|
slot |
Definition at line 275 of file LEDButton.cpp.
References setState().
|
slot |
Definition at line 219 of file LEDButton.cpp.
References led_state, and state().
Referenced by PropertyBox::layerVisibilityChanged(), off(), and on().
|
slot |
|
slot |
Definition at line 237 of file LEDButton.cpp.
References d, LEDButton::LEDButtonPrivate::dark_factor, led_color, and LEDButton::LEDButtonPrivate::offcolor.
Referenced by LEDButton().
|
slot |
Definition at line 247 of file LEDButton.cpp.
References d, LEDButton::LEDButtonPrivate::dark_factor, darkFactor(), led_color, and LEDButton::LEDButtonPrivate::offcolor.
|
overrideprotected |
Definition at line 111 of file LEDButton.cpp.
References color(), d, led_color, led_state, and LEDButton::LEDButtonPrivate::offcolor.
|
overrideprotected |
Definition at line 86 of file LEDButton.cpp.
References state(), stateChanged(), and toggle().
|
overrideprotected |
Definition at line 99 of file LEDButton.cpp.
References mouseEntered().
|
overrideprotected |
Definition at line 105 of file LEDButton.cpp.
References mouseLeft().
Member Data Documentation
|
protected |
Definition at line 77 of file LEDButton.h.
Referenced by paintEvent(), setState(), state(), and toggleState().
|
protected |
Definition at line 78 of file LEDButton.h.
Referenced by paintEvent(), setColor(), setDarkFactor(), and state().
|
protected |
Definition at line 80 of file LEDButton.h.
Referenced by LEDButton(), paintEvent(), setColor(), setDarkFactor(), and ~LEDButton().
Property Documentation
|
readwrite |
Definition at line 39 of file LEDButton.h.
|
readwrite |
Definition at line 40 of file LEDButton.h.
The documentation for this class was generated from the following files:
Generated by
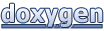