svgui
1.9
|
PropertyStack.h
Go to the documentation of this file.
void tabBarContextMenuRequested(const QPoint &)
Definition: PropertyStack.cpp:172
void propertyContainerPropertyChanged(PropertyContainer *)
Definition: PropertyStack.cpp:245
void propertyContainerAdded(PropertyContainer *)
Definition: PropertyStack.cpp:217
Definition: PropertyBox.h:34
int getContainerIndex(PropertyContainer *container) const
Definition: PropertyStack.cpp:196
void viewSelected(View *client)
void selectedContainerChanged(int)
Definition: PropertyStack.cpp:295
bool containsContainer(PropertyContainer *container) const
Definition: PropertyStack.cpp:185
PropertyStack(QWidget *parent, View *client)
Definition: PropertyStack.cpp:37
void updateValues(PropertyContainer *)
void contextHelpChanged(const QString &)
void propertyContainerRemoved(PropertyContainer *)
Definition: PropertyStack.cpp:231
void propertyContainerPropertyRangeChanged(PropertyContainer *)
Definition: PropertyStack.cpp:260
void propertyContainerNameChanged(PropertyContainer *)
Definition: PropertyStack.cpp:270
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
Definition: PropertyStack.h:28
void propertyContainerContextMenuRequested(View *client, PropertyContainer *container, QPoint pos)
void propertyContainerSelected(View *client, PropertyContainer *container)
Generated by
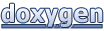