svgui
1.9
|
Layer.h
Go to the documentation of this file.
705 virtual void setMeasureRectYCoord(LayerGeometryProvider *v, MeasureRect &r, bool start, int y) const;
706 virtual void setMeasureRectFromPixrect(LayerGeometryProvider *v, MeasureRect &r, QRect pixrect) const;
virtual void setSynchronousPainting(bool)
Enable or disable synchronous painting.
Definition: Layer.h:138
QString getPropertyContainerName() const override
Definition: Layer.h:155
virtual bool isLayerDormant(const LayerGeometryProvider *v) const
Return whether the layer is dormant (i.e.
Definition: Layer.cpp:144
virtual void paintVerticalScale(LayerGeometryProvider *, bool, QPainter &, QRect) const
Definition: Layer.h:170
virtual bool snapToFeatureFrame(LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType, int) const
Adjust the given frame to snap to the nearest feature, if possible.
Definition: Layer.h:226
void deleteMeasureRectFromSet(const MeasureRect &r)
Definition: Layer.h:687
void modelCompletionChanged(ModelId)
virtual void addMeasurementRect(const QXmlAttributes &)
Add a measurement rectangle from the given XML attributes (presumably taken from a measurement elemen...
Definition: Layer.cpp:333
virtual bool getCrosshairExtents(LayerGeometryProvider *, QPainter &, QPoint, std::vector< QRect > &) const
Definition: Layer.h:175
Definition: Layer.h:350
virtual void setProperties(const QXmlAttributes &)=0
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: Layer.h:351
virtual bool getXScaleValue(const LayerGeometryProvider *v, int x, double &value, QString &unit) const
Return the value and unit at the given x coordinate in the given view.
Definition: Layer.cpp:160
virtual void eraseDrag(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.h:269
AddMeasurementRectCommand(Layer *layer, MeasureRect rect)
Definition: Layer.h:655
virtual ModelId getExportModel(LayerGeometryProvider *) const
Return the ID of a model representing the contents of this layer in a form suitable for export to a t...
Definition: Layer.h:95
virtual VerticalPosition getPreferredFrameCountPosition() const
Definition: Layer.h:146
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Convert the layer's data (though not those of the model it refers to) into XML for file output...
Definition: Layer.cpp:653
virtual void eraseStart(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.h:268
Definition: Layer.h:352
void modelReplaced()
DeleteMeasurementRectCommand(Layer *layer, MeasureRect rect)
Definition: Layer.h:670
virtual QPixmap getLayerPresentationPixmap(QSize) const
Definition: Layer.h:165
virtual void updateMeasureRectYCoords(LayerGeometryProvider *v, const MeasureRect &r) const
Definition: Layer.cpp:546
Definition: Layer.h:141
virtual QString getFeatureDescription(LayerGeometryProvider *, QPoint &) const
Definition: Layer.h:187
virtual bool needsTextLabelHeight() const
True if this layer will need to place text labels when it is painted.
Definition: Layer.h:468
void layerMeasurementRectsChanged()
virtual VerticalPosition getPreferredTimeRulerPosition() const
Definition: Layer.h:143
virtual bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const =0
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: Layer.h:141
virtual void setLayerDormant(const LayerGeometryProvider *v, bool dormant)
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: Layer.cpp:136
Definition: Layer.h:353
Definition: Layer.h:667
ModelId getSourceModel() const
Return the ID of the source model for the model represented in this layer.
Definition: Layer.cpp:68
virtual void paint(LayerGeometryProvider *, QPainter &, QRect) const =0
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: Layer.h:198
virtual RangeMapper * getNewVerticalZoomRangeMapper() const
Create and return a range mapper for vertical zoom step values.
Definition: Layer.h:602
void toXml(QTextStream &stream, QString indent) const
Definition: Layer.cpp:315
virtual ColourSignificance getLayerColourSignificance() const =0
This should return the degree of meaning associated with colour in this layer.
virtual void measureDoubleClick(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:444
void layerParameterRangesChanged()
virtual void measureDrag(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:414
virtual bool canExistWithoutModel() const
Return true if this layer type can function without a model being set.
Definition: Layer.h:609
virtual bool snapToSimilarFeature(LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType) const
Adjust the given frame to snap to the next feature that has "effectively" the same value as the featu...
Definition: Layer.h:251
void modelChanged(ModelId)
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
virtual bool paste(LayerGeometryProvider *, const Clipboard &, sv_frame_t, bool)
Paste from the given clipboard onto the layer at the given frame offset.
Definition: Layer.h:314
virtual void zoomToRegion(const LayerGeometryProvider *, QRect)
Update the X and Y axis scales, where appropriate, to focus on the given rectangular region...
Definition: Layer.h:483
virtual sv_frame_t alignFromReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:198
std::shared_ptr< PlayParameters > getPlayParameters() override
Return the play parameters for this layer, if any.
Definition: Layer.cpp:129
virtual void setMeasureRectYCoord(LayerGeometryProvider *v, MeasureRect &r, bool start, int y) const
Definition: Layer.cpp:554
virtual int getHorizontalScaleHeight(LayerGeometryProvider *, QPainter &) const
Definition: Layer.h:173
virtual bool adoptExtents(double, double, QString)
Consider using the given value extents and units for this layer.
Definition: Layer.h:532
virtual QString getLayerPresentationName() const
Definition: Layer.cpp:99
virtual bool isLayerOpaque() const
This should return true if the layer completely obscures any underlying layers.
Definition: Layer.h:346
virtual bool getYScaleValue(const LayerGeometryProvider *, int, double &, QString &) const
Return the value and unit at the given y coordinate in the given view.
Definition: Layer.h:550
void layerParametersChanged()
void verticalZoomChanged()
bool clipboardHasDifferentAlignment(LayerGeometryProvider *v, const Clipboard &clip) const
Definition: Layer.cpp:209
virtual sv_frame_t alignToReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:187
Definition: Layer.h:197
Definition: Layer.h:349
virtual void measureEnd(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:430
virtual void toBriefXml(QTextStream &stream, QString indent="", QString extraAttributes="") const
Produce XML containing the layer's ID and type.
Definition: Layer.cpp:691
Definition: Layer.h:639
bool valueExtentsMatchMine(LayerGeometryProvider *v) const
Definition: Layer.cpp:628
virtual void paintCrosshairs(LayerGeometryProvider *, QPainter &, QPoint) const
Definition: Layer.h:179
MeasureRectSet::const_iterator findFocusedMeasureRect(QPoint) const
Definition: Layer.cpp:578
virtual bool supportsOtherZoomLevels() const
Return true if this layer can handle zoom levels other than those supported by its zoom constraint (p...
Definition: Layer.h:114
Definition: Layer.h:652
virtual bool isLayerScrollable(const LayerGeometryProvider *) const
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: Layer.h:336
Definition: Layer.h:196
virtual bool setDisplayExtents(double, double)
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: Layer.h:521
void updateMeasurePixrects(LayerGeometryProvider *v) const
Definition: Layer.cpp:507
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
virtual void editStart(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.h:272
virtual void splitStart(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.h:276
virtual int getCurrentVerticalZoomStep() const
Get the current vertical zoom step.
Definition: Layer.h:586
void showLayer(LayerGeometryProvider *, bool show)
Change the visibility status (dormancy) of the layer in the given view.
Definition: Layer.cpp:153
virtual bool isLayerEditable() const
This should return true if the layer can be edited by the user.
Definition: Layer.h:382
void modelAlignmentCompletionChanged(ModelId)
virtual void copy(LayerGeometryProvider *, Selection, Clipboard &)
Definition: Layer.h:305
virtual const ZoomConstraint * getZoomConstraint() const
Return a zoom constraint object defining the supported zoom levels for this layer.
Definition: Layer.h:104
virtual int getVerticalZoomSteps(int &) const
Get the number of vertical zoom steps available for this layer.
Definition: Layer.h:578
virtual bool hasTimeXAxis() const
Return true if the X axis on the layer is time proportional to audio frames, false otherwise...
Definition: Layer.h:475
virtual void setMeasureRectFromPixrect(LayerGeometryProvider *v, MeasureRect &r, QRect pixrect) const
Definition: Layer.cpp:565
virtual bool getDisplayExtents(double &, double &) const
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: Layer.h:509
virtual ModelId getModel() const =0
Return the ID of the model represented in this layer.
virtual void measureStart(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:390
virtual QString getError(LayerGeometryProvider *) const
Return an error string if any errors have occurred while loading or processing data for the given vie...
Definition: Layer.h:398
void modelChangedWithin(ModelId, sv_frame_t startFrame, sv_frame_t endFrame)
QString getPropertyContainerIconName() const override
Definition: Layer.cpp:80
virtual int getVerticalScaleWidth(LayerGeometryProvider *, bool detailed, QPainter &) const =0
virtual int getCompletion(LayerGeometryProvider *) const
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: Layer.h:391
virtual void paintMeasurementRects(LayerGeometryProvider *, QPainter &, bool showFocus, QPoint focusPoint) const
Definition: Layer.cpp:464
virtual bool getYScaleDifference(const LayerGeometryProvider *v, int y0, int y1, double &diff, QString &unit) const
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
Definition: Layer.cpp:173
Definition: Layer.h:141
virtual bool nearestMeasurementRectChanged(LayerGeometryProvider *, QPoint prev, QPoint now) const
Definition: Layer.cpp:496
void layerNameChanged()
virtual bool editOpen(LayerGeometryProvider *, QMouseEvent *)
Open an editor on the item under the mouse (e.g.
Definition: Layer.h:299
void paintMeasurementRect(LayerGeometryProvider *v, QPainter &paint, const MeasureRect &r, bool focus) const
Definition: Layer.cpp:605
virtual void drawStart(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.h:264
Generated by
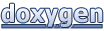