svgui
1.9
|
LayerGeometryProvider.h
Go to the documentation of this file.
virtual int scalePixelSize(int size) const =0
virtual int getXForViewX(int viewx) const =0
Return the closest pixel x-coordinate corresponding to a given view x-coordinate. ...
virtual QColor getForeground() const =0
virtual QColor getBackground() const =0
virtual QSize getPaintSize() const
Definition: LayerGeometryProvider.h:186
virtual bool shouldIlluminateLocalFeatures(const Layer *, QPoint &) const =0
virtual ZoomLevel getZoomLevel() const =0
Return the zoom level, i.e.
virtual double getFrequencyForY(double y, double minFreq, double maxFreq, bool logarithmic) const =0
Return the closest frequency to the given (maybe fractional) pixel y-coordinate, if the frequency ran...
virtual sv_frame_t getFrameForX(int x) const =0
Return the closest frame to the given pixel x-coordinate.
LayerGeometryProvider()
Definition: LayerGeometryProvider.h:67
virtual double scaleSize(double size) const =0
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
virtual double getYForFrequency(double frequency, double minFreq, double maxFreq, bool logarithmic) const =0
Return the (maybe fractional) pixel y-coordinate corresponding to a given frequency, if the frequency range is as specified.
virtual QRect getPaintRect() const =0
To be called from a layer, to obtain the extent of the surface that the layer is currently painting t...
virtual sv_frame_t getStartFrame() const =0
Retrieve the first visible sample frame on the widget.
virtual double scalePenWidth(double width) const =0
virtual bool shouldShowFeatureLabels() const =0
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
virtual sv_frame_t getModelsStartFrame() const =0
virtual sv_frame_t getModelsEndFrame() const =0
virtual QPen scalePen(QPen pen) const =0
virtual int getViewXForX(int x) const =0
Return the closest view x-coordinate corresponding to a given pixel x-coordinate. ...
virtual void updatePaintRect(QRect r)=0
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
virtual void drawMeasurementRect(QPainter &p, const Layer *, QRect rect, bool focus) const =0
virtual ViewManager * getViewManager() const =0
The ViewManager manages properties that may need to be synchronised between separate Views...
Definition: ViewManager.h:78
virtual int getTextLabelYCoord(const Layer *layer, QPainter &) const =0
Return a y-coordinate at which text labels for individual items in a layer may be drawn...
virtual bool getVisibleExtentsForUnit(QString unit, double &min, double &max, bool &log) const =0
Return the visible vertical extents for the given unit, if any.
virtual sv_frame_t getCentreFrame() const =0
Return the centre frame of the visible widget.
virtual sv_frame_t getEndFrame() const =0
Retrieve the last visible sample frame on the widget.
virtual bool hasLightBackground() const =0
virtual int getXForFrame(sv_frame_t frame) const =0
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative)...
virtual int getPaintWidth() const
Definition: LayerGeometryProvider.h:187
virtual View * getView()=0
Generated by
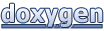