svgui
1.9
|
The ViewManager manages properties that may need to be synchronised between separate Views. More...
#include <ViewManager.h>
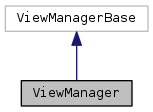
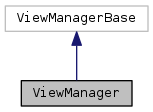
Classes | |
class | SetSelectionCommand |
Public Types | |
enum | ToolMode { NavigateMode, SelectMode, EditMode, DrawMode, EraseMode, MeasureMode, NoteEditMode } |
enum | OverlayMode { NoOverlays, GlobalOverlays, StandardOverlays, AllOverlays } |
Public Slots | |
void | viewCentreFrameChanged (sv_frame_t, bool, PlaybackFollowMode) |
void | viewZoomLevelChanged (ZoomLevel, bool) |
void | setGlobalCentreFrame (sv_frame_t) |
void | setPlaybackFrame (sv_frame_t) |
void | playStatusChanged (bool playing) |
void | recordStatusChanged (bool recording) |
Signals | |
void | globalCentreFrameChanged (sv_frame_t frame) |
Emitted when user causes the global centre frame to change. More... | |
void | viewCentreFrameChanged (View *v, sv_frame_t frame) |
Emitted when user scrolls a view, but doesn't affect global centre. More... | |
void | viewZoomLevelChanged (View *v, ZoomLevel zoom, bool locked) |
Emitted when a view zooms. More... | |
void | playbackFrameChanged (sv_frame_t frame) |
Emitted when the playback frame changes. More... | |
void | monitoringLevelsChanged (float left, float right) |
Emitted when the output or record levels change. More... | |
void | selectionChanged () |
Emitted whenever the selection has changed. More... | |
void | selectionChangedByUser () |
Emitted when the selection has been changed through an explicit selection-editing action. More... | |
void | inProgressSelectionChanged () |
Emitted when the in-progress (rubberbanding) selection has changed. More... | |
void | toolModeChanged () |
Emitted when the tool mode has been changed. More... | |
void | playLoopModeChanged () |
Emitted when the play loop mode has been changed. More... | |
void | playLoopModeChanged (bool) |
void | playSelectionModeChanged () |
Emitted when the play selection mode has been changed. More... | |
void | playSelectionModeChanged (bool) |
void | playSoloModeChanged () |
Emitted when the play solo mode has been changed. More... | |
void | playSoloModeChanged (bool) |
void | alignModeChanged () |
Emitted when the alignment mode has been changed. More... | |
void | alignModeChanged (bool) |
void | overlayModeChanged () |
Emitted when the overlay mode has been changed. More... | |
void | showCentreLineChanged () |
Emitted when the centre line visibility has been changed. More... | |
void | zoomWheelsEnabledChanged () |
Emitted when the zoom wheels have been toggled. More... | |
void | opportunisticEditingEnabledChanged () |
Emitted when editing-enabled has been toggled. More... | |
void | activity (QString) |
Emitted when any loggable activity has occurred. More... | |
Public Member Functions | |
ViewManager () | |
virtual | ~ViewManager () |
void | setAudioPlaySource (AudioPlaySource *source) override |
void | setAudioRecordTarget (AudioRecordTarget *target) override |
bool | isPlaying () const |
bool | isRecording () const |
sv_frame_t | getGlobalCentreFrame () const |
ZoomLevel | getGlobalZoom () const |
sv_frame_t | getPlaybackFrame () const |
ModelId | getPlaybackModel () const |
void | setPlaybackModel (ModelId) |
sv_frame_t | alignPlaybackFrameToReference (sv_frame_t) const override |
sv_frame_t | alignReferenceToPlaybackFrame (sv_frame_t) const override |
bool | haveInProgressSelection () const |
const Selection & | getInProgressSelection (bool &exclusive) const |
void | setInProgressSelection (const Selection &selection, bool exclusive) |
void | clearInProgressSelection () |
const MultiSelection & | getSelection () const override |
const MultiSelection::SelectionList & | getSelections () const override |
void | setSelection (const Selection &selection) |
void | addSelection (const Selection &selection) |
void | removeSelection (const Selection &selection) |
void | clearSelections () |
sv_frame_t | constrainFrameToSelection (sv_frame_t frame) const override |
void | addSelectionQuietly (const Selection &selection) |
Adding a selection normally emits the selectionChangedByUser signal. More... | |
Selection | getContainingSelection (sv_frame_t frame, bool defaultToFollowing) const override |
Return the selection that contains a given frame. More... | |
Clipboard & | getClipboard () |
ToolMode | getToolMode () const |
void | setToolMode (ToolMode mode) |
void | setToolModeFor (const View *v, ToolMode mode) |
Override the tool mode for a specific view. More... | |
ToolMode | getToolModeFor (const View *v) const |
Return override mode if it exists for this view or global mode otherwise. More... | |
void | clearToolModeOverrides () |
Clear all current view-specific overrides. More... | |
bool | getPlayLoopMode () const override |
void | setPlayLoopMode (bool on) |
bool | getPlaySelectionMode () const override |
void | setPlaySelectionMode (bool on) |
bool | getPlaySoloMode () const override |
void | setPlaySoloMode (bool on) |
bool | getAlignMode () const override |
void | setAlignMode (bool on) |
void | setIlluminateLocalFeatures (bool i) |
void | setShowWorkTitle (bool show) |
void | setShowDuration (bool show) |
sv_samplerate_t | getPlaybackSampleRate () const |
The sample rate that is used for playback. More... | |
sv_samplerate_t | getDeviceSampleRate () const |
The sample rate of the audio output device. More... | |
sv_samplerate_t | getMainModelSampleRate () const |
The sample rate of the current main model. More... | |
void | setMainModelSampleRate (sv_samplerate_t sr) |
void | setOverlayMode (OverlayMode mode) |
OverlayMode | getOverlayMode () const |
void | setShowCentreLine (bool show) |
bool | shouldShowCentreLine () const |
bool | shouldShowDuration () const |
bool | shouldShowFrameCount () const |
bool | shouldShowVerticalScale () const |
bool | shouldShowVerticalColourScale () const |
bool | shouldShowHorizontalValueScale () const |
bool | shouldShowSelectionExtents () const |
bool | shouldShowLayerNames () const |
bool | shouldShowScaleGuides () const |
bool | shouldShowWorkTitle () const |
bool | shouldIlluminateLocalFeatures () const |
bool | shouldShowFeatureLabels () const |
void | setZoomWheelsEnabled (bool enable) |
bool | getZoomWheelsEnabled () const |
void | setOpportunisticEditingEnabled (bool enable) |
Enable or disable opportunistic editing. More... | |
bool | getOpportunisticEditingEnabled () const |
void | setGlobalDarkBackground (bool dark) |
bool | getGlobalDarkBackground () const |
Static Public Member Functions | |
static int | scalePixelSize (int pixels) |
Take a "design pixel" size and scale it for the actual display. More... | |
Protected Slots | |
void | checkPlayStatus () |
void | seek (sv_frame_t) |
Protected Member Functions | |
void | setSelections (const MultiSelection &ms, bool quietly=false) |
void | signalSelectionChange () |
Protected Attributes | |
AudioPlaySource * | m_playSource |
!! void considerZoomChange(void *, int, bool); More... | |
AudioRecordTarget * | m_recordTarget |
sv_frame_t | m_globalCentreFrame |
ZoomLevel | m_globalZoom |
sv_frame_t | m_playbackFrame |
ModelId | m_playbackModel |
sv_samplerate_t | m_mainModelSampleRate |
float | m_lastLeft |
float | m_lastRight |
MultiSelection | m_selections |
Selection | m_inProgressSelection |
bool | m_inProgressExclusive |
Clipboard | m_clipboard |
ToolMode | m_toolMode |
std::map< const View *, ToolMode > | m_toolModeOverrides |
bool | m_playLoopMode |
bool | m_playSelectionMode |
bool | m_playSoloMode |
bool | m_alignMode |
OverlayMode | m_overlayMode |
bool | m_zoomWheelsEnabled |
bool | m_opportunisticEditingEnabled |
bool | m_showCentreLine |
bool | m_illuminateLocalFeatures |
bool | m_showWorkTitle |
bool | m_showDuration |
QPalette | m_lightPalette |
QPalette | m_darkPalette |
Detailed Description
The ViewManager manages properties that may need to be synchronised between separate Views.
For example, it handles signals associated with changes to the global pan and zoom, and it handles selections.
Views should be implemented in such a way as to work correctly whether they are supplied with a ViewManager or not.
Definition at line 78 of file ViewManager.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NavigateMode | |
SelectMode | |
EditMode | |
DrawMode | |
EraseMode | |
MeasureMode | |
NoteEditMode |
Definition at line 135 of file ViewManager.h.
Enumerator | |
---|---|
NoOverlays | |
GlobalOverlays | |
StandardOverlays | |
AllOverlays |
Definition at line 201 of file ViewManager.h.
Constructor & Destructor Documentation
ViewManager::ViewManager | ( | ) |
Definition at line 35 of file ViewManager.cpp.
References AllOverlays, getGlobalDarkBackground(), m_darkPalette, m_lightPalette, m_overlayMode, m_showCentreLine, m_zoomWheelsEnabled, NoOverlays, and StandardOverlays.
|
virtual |
Definition at line 137 of file ViewManager.cpp.
Member Function Documentation
|
override |
Definition at line 536 of file ViewManager.cpp.
References checkPlayStatus(), and m_playSource.
|
override |
Definition at line 545 of file ViewManager.cpp.
References checkPlayStatus(), and m_recordTarget.
bool ViewManager::isPlaying | ( | ) | const |
Definition at line 631 of file ViewManager.cpp.
References m_playSource.
Referenced by checkPlayStatus(), Pane::dragTopLayer(), View::drawPlayPointer(), Pane::edgeScrollMaybe(), getPlaybackFrame(), Pane::mouseMoveEvent(), View::movePlayPointer(), Pane::paintEvent(), seek(), and setPlaybackFrame().
bool ViewManager::isRecording | ( | ) | const |
Definition at line 637 of file ViewManager.cpp.
References m_recordTarget.
Referenced by checkPlayStatus(), getPlaybackFrame(), and seek().
sv_frame_t ViewManager::getGlobalCentreFrame | ( | ) | const |
Definition at line 142 of file ViewManager.cpp.
References m_globalCentreFrame.
Referenced by View::setViewManager().
ZoomLevel ViewManager::getGlobalZoom | ( | ) | const |
Definition at line 161 of file ViewManager.cpp.
References m_globalZoom.
Referenced by View::setViewManager().
sv_frame_t ViewManager::getPlaybackFrame | ( | ) | const |
Definition at line 170 of file ViewManager.cpp.
References isPlaying(), isRecording(), m_playbackFrame, m_playSource, and m_recordTarget.
Referenced by View::getAlignedPlaybackFrame(), and View::setViewManager().
ModelId ViewManager::getPlaybackModel | ( | ) | const |
Definition at line 207 of file ViewManager.cpp.
References m_playbackModel.
Referenced by View::getAligningAndReferenceModels().
void ViewManager::setPlaybackModel | ( | ModelId | model | ) |
Definition at line 213 of file ViewManager.cpp.
References m_playbackModel.
|
override |
Definition at line 219 of file ViewManager.cpp.
References m_alignMode, and m_playbackModel.
|
override |
Definition at line 240 of file ViewManager.cpp.
References m_alignMode, and m_playbackModel.
bool ViewManager::haveInProgressSelection | ( | ) | const |
Definition at line 261 of file ViewManager.cpp.
References m_inProgressSelection.
Referenced by Pane::dragExtendSelection(), View::drawSelections(), and Pane::mouseReleaseEvent().
const Selection & ViewManager::getInProgressSelection | ( | bool & | exclusive | ) | const |
Definition at line 267 of file ViewManager.cpp.
References m_inProgressExclusive, and m_inProgressSelection.
Referenced by Pane::dragExtendSelection(), View::drawSelections(), and Pane::mouseReleaseEvent().
void ViewManager::setInProgressSelection | ( | const Selection & | selection, |
bool | exclusive | ||
) |
Definition at line 274 of file ViewManager.cpp.
References clearSelections(), inProgressSelectionChanged(), m_inProgressExclusive, and m_inProgressSelection.
Referenced by Pane::dragExtendSelection(), and Pane::mousePressEvent().
void ViewManager::clearInProgressSelection | ( | ) |
Definition at line 283 of file ViewManager.cpp.
References inProgressSelectionChanged(), and m_inProgressSelection.
Referenced by Pane::mouseDoubleClickEvent(), and Pane::mouseReleaseEvent().
|
override |
Definition at line 290 of file ViewManager.cpp.
References m_selections.
|
override |
Definition at line 296 of file ViewManager.cpp.
References m_selections.
Referenced by constrainFrameToSelection(), View::drawSelections(), View::movePlayPointer(), Pane::shouldIlluminateLocalSelection(), and Pane::updateContextHelp().
void ViewManager::setSelection | ( | const Selection & | selection | ) |
Definition at line 302 of file ViewManager.cpp.
References m_selections, and setSelections().
Referenced by Pane::mouseReleaseEvent().
void ViewManager::addSelection | ( | const Selection & | selection | ) |
Definition at line 310 of file ViewManager.cpp.
References m_selections, and setSelections().
Referenced by Pane::editSelectionEnd(), and Pane::mouseReleaseEvent().
void ViewManager::removeSelection | ( | const Selection & | selection | ) |
Definition at line 326 of file ViewManager.cpp.
References m_selections, and setSelections().
Referenced by Pane::editSelectionEnd(), and Pane::mousePressEvent().
void ViewManager::clearSelections | ( | ) |
Definition at line 334 of file ViewManager.cpp.
References m_selections, and setSelections().
Referenced by setInProgressSelection().
|
override |
Definition at line 353 of file ViewManager.cpp.
References getSelections().
void ViewManager::addSelectionQuietly | ( | const Selection & | selection | ) |
Adding a selection normally emits the selectionChangedByUser signal.
Call this to add a selection without emitting that signal. This is used in session file load, for example.
Definition at line 318 of file ViewManager.cpp.
References m_selections, and setSelections().
|
override |
Return the selection that contains a given frame.
If defaultToFollowing is true, and if the frame is not in a selected area, return the next selection after the given frame. Return the empty selection if no appropriate selection is found.
Definition at line 412 of file ViewManager.cpp.
References m_selections.
Referenced by Pane::getSelectionAt().
|
inline |
Definition at line 133 of file ViewManager.h.
References m_clipboard.
|
inline |
Definition at line 144 of file ViewManager.h.
References clearToolModeOverrides(), getToolModeFor(), m_toolMode, setToolMode(), and setToolModeFor().
Referenced by getToolModeFor().
void ViewManager::setToolMode | ( | ToolMode | mode | ) |
Definition at line 418 of file ViewManager.cpp.
References activity(), DrawMode, EditMode, EraseMode, m_toolMode, MeasureMode, NavigateMode, NoteEditMode, SelectMode, and toolModeChanged().
Referenced by getToolMode().
Override the tool mode for a specific view.
Definition at line 446 of file ViewManager.cpp.
References m_toolModeOverrides.
Referenced by getToolMode().
ViewManager::ToolMode ViewManager::getToolModeFor | ( | const View * | v | ) | const |
Return override mode if it exists for this view or global mode otherwise.
Definition at line 436 of file ViewManager.cpp.
References getToolMode(), and m_toolModeOverrides.
Referenced by Pane::editSelectionStart(), getToolMode(), Pane::mouseDoubleClickEvent(), Pane::mouseMoveEvent(), Pane::mousePressEvent(), Pane::mouseReleaseEvent(), Pane::paintEvent(), Pane::shouldIlluminateLocalFeatures(), Pane::shouldIlluminateLocalSelection(), Pane::toolModeChanged(), and Pane::updateContextHelp().
void ViewManager::clearToolModeOverrides | ( | ) |
Clear all current view-specific overrides.
Definition at line 452 of file ViewManager.cpp.
References m_toolModeOverrides.
Referenced by getToolMode().
|
inlineoverride |
Definition at line 154 of file ViewManager.h.
References m_playLoopMode, and setPlayLoopMode().
void ViewManager::setPlayLoopMode | ( | bool | on | ) |
Definition at line 458 of file ViewManager.cpp.
References activity(), m_playLoopMode, and playLoopModeChanged().
Referenced by getPlayLoopMode().
|
inlineoverride |
Definition at line 157 of file ViewManager.h.
References m_playSelectionMode, and setPlaySelectionMode().
Referenced by View::movePlayPointer().
void ViewManager::setPlaySelectionMode | ( | bool | on | ) |
Definition at line 473 of file ViewManager.cpp.
References activity(), m_playSelectionMode, and playSelectionModeChanged().
Referenced by getPlaySelectionMode().
|
inlineoverride |
Definition at line 160 of file ViewManager.h.
References m_playSoloMode, and setPlaySoloMode().
void ViewManager::setPlaySoloMode | ( | bool | on | ) |
Definition at line 488 of file ViewManager.cpp.
References activity(), m_playSoloMode, and playSoloModeChanged().
Referenced by getPlaySoloMode().
|
inlineoverride |
Definition at line 163 of file ViewManager.h.
References m_alignMode, and setAlignMode().
Referenced by View::alignFromReference(), View::alignToReference(), View::getAlignedPlaybackFrame(), View::getAligningAndReferenceModels(), and Pane::paintEvent().
void ViewManager::setAlignMode | ( | bool | on | ) |
Definition at line 503 of file ViewManager.cpp.
References activity(), alignModeChanged(), and m_alignMode.
Referenced by getAlignMode().
|
inline |
Definition at line 166 of file ViewManager.h.
References m_illuminateLocalFeatures.
|
inline |
Definition at line 167 of file ViewManager.h.
References m_showWorkTitle.
|
inline |
Definition at line 168 of file ViewManager.h.
References getDeviceSampleRate(), getPlaybackSampleRate(), and m_showDuration.
sv_samplerate_t ViewManager::getPlaybackSampleRate | ( | ) | const |
The sample rate that is used for playback.
This is usually the rate of the main model, but not always. Models whose rates differ from this will play back at the wrong speed – there is no per-model resampler.
Definition at line 518 of file ViewManager.cpp.
References m_playSource.
Referenced by Pane::drawDurationAndRate(), and setShowDuration().
sv_samplerate_t ViewManager::getDeviceSampleRate | ( | ) | const |
The sample rate of the audio output device.
If the playback sample rate differs from this, everything will be resampled at the output stage (but not before).
Definition at line 527 of file ViewManager.cpp.
References m_playSource.
Referenced by setShowDuration().
|
inline |
The sample rate of the current main model.
This may in theory differ from the playback sample rate, in which case even the main model will play at the wrong speed.
Definition at line 190 of file ViewManager.h.
References m_mainModelSampleRate.
Referenced by Colour3DPlotRenderer::decideRenderType(), Pane::drawCentreLine(), AlignmentView::getDefaultKeyFrames(), Colour3DPlotLayer::getFeatureDescription(), and Colour3DPlotRenderer::renderDirectTranslucent().
|
inline |
Definition at line 192 of file ViewManager.h.
References m_mainModelSampleRate, and scalePixelSize().
|
static |
Take a "design pixel" size and scale it for the actual display.
This is relevant to hi-dpi systems that do not do pixel doubling (i.e. Windows and Linux rather than OS/X).
Definition at line 857 of file ViewManager.cpp.
Referenced by PaneStack::addPane(), MenuTitle::addTitle(), PaneStack::adjustAlignmentViewHeights(), CSVExportDialog::CSVExportDialog(), Pane::drawLayerNames(), TextLayer::getLocalPoints(), RegionLayer::getLocalPoints(), NoteLayer::getLocalPoints(), FlexiNoteLayer::getLocalPoints(), TextLayer::getPointToDrag(), TextLayer::paint(), SpectrumLayer::paint(), setMainModelSampleRate(), TimeRulerLayer::snapToFeatureFrame(), Pane::updateDragMode(), and Pane::updateHeadsUpDisplay().
void ViewManager::setOverlayMode | ( | OverlayMode | mode | ) |
Definition at line 739 of file ViewManager.cpp.
References activity(), m_overlayMode, and overlayModeChanged().
|
inline |
Definition at line 208 of file ViewManager.h.
References m_overlayMode, and setShowCentreLine().
Referenced by TimeRulerLayer::paint().
void ViewManager::setShowCentreLine | ( | bool | show | ) |
Definition at line 779 of file ViewManager.cpp.
References activity(), m_showCentreLine, and showCentreLineChanged().
Referenced by getOverlayMode().
|
inline |
Definition at line 211 of file ViewManager.h.
References m_showCentreLine.
Referenced by View::drawPlayPointer(), and Pane::paintEvent().
|
inline |
Definition at line 213 of file ViewManager.h.
References m_overlayMode, m_showDuration, and NoOverlays.
Referenced by Pane::paintEvent(), and shouldShowFrameCount().
|
inline |
Definition at line 216 of file ViewManager.h.
References m_showCentreLine, and shouldShowDuration().
Referenced by Pane::drawCentreLine().
|
inline |
Definition at line 219 of file ViewManager.h.
References m_overlayMode, and NoOverlays.
Referenced by Pane::getRenderedPartImageSize(), Pane::paintEvent(), and Pane::renderPartToNewImage().
|
inline |
Definition at line 222 of file ViewManager.h.
References AllOverlays, and m_overlayMode.
Referenced by Pane::drawVerticalScale(), Pane::getRenderedPartImageSize(), Pane::render(), and Pane::renderPartToNewImage().
|
inline |
Definition at line 225 of file ViewManager.h.
References m_overlayMode, and NoOverlays.
Referenced by SpectrumLayer::paintHorizontalScale().
|
inline |
Definition at line 228 of file ViewManager.h.
References GlobalOverlays, m_overlayMode, and NoOverlays.
Referenced by View::drawSelections().
|
inline |
Definition at line 231 of file ViewManager.h.
References AllOverlays, and m_overlayMode.
Referenced by Pane::paintEvent().
|
inline |
Definition at line 234 of file ViewManager.h.
References m_overlayMode, and NoOverlays.
Referenced by SliceLayer::paint(), and WaveformLayer::paintChannelScaleGuides().
|
inline |
Definition at line 237 of file ViewManager.h.
References m_showWorkTitle.
Referenced by Pane::paintEvent().
|
inline |
Definition at line 240 of file ViewManager.h.
References m_illuminateLocalFeatures.
Referenced by Pane::mouseMoveEvent(), Pane::paintEvent(), and Pane::shouldIlluminateLocalFeatures().
|
inline |
Definition at line 243 of file ViewManager.h.
References GlobalOverlays, m_overlayMode, NoOverlays, and setZoomWheelsEnabled().
Referenced by View::shouldShowFeatureLabels().
void ViewManager::setZoomWheelsEnabled | ( | bool | enable | ) |
Definition at line 754 of file ViewManager.cpp.
References activity(), m_zoomWheelsEnabled, and zoomWheelsEnabledChanged().
Referenced by shouldShowFeatureLabels().
|
inline |
Definition at line 248 of file ViewManager.h.
References m_zoomWheelsEnabled, and setOpportunisticEditingEnabled().
Referenced by Pane::drawLayerNames(), Pane::editVerticalPannerExtents(), Pane::updateHeadsUpDisplay(), Pane::updateVerticalPanner(), and Pane::viewZoomLevelChanged().
void ViewManager::setOpportunisticEditingEnabled | ( | bool | enable | ) |
Enable or disable opportunistic editing.
This allows certain edits while not in edit modes - e.g. double-click on an item while in navigate mode to open an edit dialog. It is enabled by default, but it may be undesirable if the application is intended to be "read-only".
This setting makes no difference to behaviour when actually in editing modes.
Unlike some other options, this is considered to be application-build-specific and is not restored from settings.
Definition at line 770 of file ViewManager.cpp.
References m_opportunisticEditingEnabled, and opportunisticEditingEnabledChanged().
Referenced by getZoomWheelsEnabled().
|
inline |
Definition at line 264 of file ViewManager.h.
References activity(), alignModeChanged(), checkPlayStatus(), getGlobalDarkBackground(), globalCentreFrameChanged(), inProgressSelectionChanged(), m_opportunisticEditingEnabled, monitoringLevelsChanged(), opportunisticEditingEnabledChanged(), overlayModeChanged(), playbackFrameChanged(), playLoopModeChanged(), playSelectionModeChanged(), playSoloModeChanged(), playStatusChanged(), recordStatusChanged(), seek(), selectionChanged(), selectionChangedByUser(), setGlobalCentreFrame(), setGlobalDarkBackground(), setPlaybackFrame(), showCentreLineChanged(), toolModeChanged(), viewCentreFrameChanged(), viewZoomLevelChanged(), and zoomWheelsEnabledChanged().
Referenced by Pane::mouseDoubleClickEvent().
void ViewManager::setGlobalDarkBackground | ( | bool | dark | ) |
Definition at line 795 of file ViewManager.cpp.
References getGlobalDarkBackground(), m_darkPalette, and m_lightPalette.
Referenced by getOpportunisticEditingEnabled().
bool ViewManager::getGlobalDarkBackground | ( | ) | const |
Definition at line 846 of file ViewManager.cpp.
Referenced by getOpportunisticEditingEnabled(), View::hasLightBackground(), AlignmentView::paintEvent(), setGlobalDarkBackground(), and ViewManager().
|
signal |
Emitted when user causes the global centre frame to change.
Referenced by getOpportunisticEditingEnabled(), setGlobalCentreFrame(), and viewCentreFrameChanged().
|
signal |
Emitted when user scrolls a view, but doesn't affect global centre.
Referenced by getOpportunisticEditingEnabled(), and viewCentreFrameChanged().
|
signal |
Emitted when a view zooms.
Referenced by getOpportunisticEditingEnabled(), and viewZoomLevelChanged().
|
signal |
Emitted when the playback frame changes.
Referenced by checkPlayStatus(), getOpportunisticEditingEnabled(), seek(), and setPlaybackFrame().
|
signal |
Emitted when the output or record levels change.
Values in range 0.0 -> 1.0.
Referenced by checkPlayStatus(), and getOpportunisticEditingEnabled().
|
signal |
Emitted whenever the selection has changed.
Referenced by getOpportunisticEditingEnabled(), and signalSelectionChange().
|
signal |
Emitted when the selection has been changed through an explicit selection-editing action.
Not emitted when the selection has been changed through undo or redo.
Referenced by getOpportunisticEditingEnabled(), and setSelections().
|
signal |
Emitted when the in-progress (rubberbanding) selection has changed.
Referenced by clearInProgressSelection(), getOpportunisticEditingEnabled(), and setInProgressSelection().
|
signal |
Emitted when the tool mode has been changed.
Referenced by getOpportunisticEditingEnabled(), and setToolMode().
|
signal |
Emitted when the play loop mode has been changed.
Referenced by getOpportunisticEditingEnabled(), and setPlayLoopMode().
|
signal |
|
signal |
Emitted when the play selection mode has been changed.
Referenced by getOpportunisticEditingEnabled(), and setPlaySelectionMode().
|
signal |
|
signal |
Emitted when the play solo mode has been changed.
Referenced by getOpportunisticEditingEnabled(), and setPlaySoloMode().
|
signal |
|
signal |
Emitted when the alignment mode has been changed.
Referenced by getOpportunisticEditingEnabled(), and setAlignMode().
|
signal |
|
signal |
Emitted when the overlay mode has been changed.
Referenced by getOpportunisticEditingEnabled(), and setOverlayMode().
|
signal |
Emitted when the centre line visibility has been changed.
Referenced by getOpportunisticEditingEnabled(), and setShowCentreLine().
|
signal |
Emitted when the zoom wheels have been toggled.
Referenced by getOpportunisticEditingEnabled(), and setZoomWheelsEnabled().
|
signal |
Emitted when editing-enabled has been toggled.
Referenced by getOpportunisticEditingEnabled(), and setOpportunisticEditingEnabled().
|
signal |
Emitted when any loggable activity has occurred.
Referenced by getOpportunisticEditingEnabled(), setAlignMode(), setOverlayMode(), setPlayLoopMode(), setPlaySelectionMode(), setPlaySoloMode(), setShowCentreLine(), setToolMode(), setZoomWheelsEnabled(), viewCentreFrameChanged(), and viewZoomLevelChanged().
|
slot |
Definition at line 643 of file ViewManager.cpp.
References activity(), globalCentreFrameChanged(), m_globalCentreFrame, m_mainModelSampleRate, PlaybackIgnore, PlaybackScrollContinuous, PlaybackScrollPageWithCentre, seek(), and viewCentreFrameChanged().
|
slot |
!! emit zoomLevelChanged();
Definition at line 708 of file ViewManager.cpp.
References activity(), m_globalZoom, and viewZoomLevelChanged().
|
slot |
Definition at line 151 of file ViewManager.cpp.
References globalCentreFrameChanged(), and m_globalCentreFrame.
Referenced by getOpportunisticEditingEnabled().
|
slot |
Definition at line 191 of file ViewManager.cpp.
References isPlaying(), m_playbackFrame, m_playSource, and playbackFrameChanged().
Referenced by getOpportunisticEditingEnabled(), and Pane::playbackScheduleTimerElapsed().
|
slot |
Definition at line 554 of file ViewManager.cpp.
References checkPlayStatus().
Referenced by getOpportunisticEditingEnabled().
|
slot |
Definition at line 563 of file ViewManager.cpp.
References checkPlayStatus().
Referenced by getOpportunisticEditingEnabled().
|
protectedslot |
Definition at line 572 of file ViewManager.cpp.
References isPlaying(), isRecording(), m_lastLeft, m_lastRight, m_playbackFrame, m_playSource, m_recordTarget, monitoringLevelsChanged(), and playbackFrameChanged().
Referenced by getOpportunisticEditingEnabled(), playStatusChanged(), recordStatusChanged(), setAudioPlaySource(), and setAudioRecordTarget().
|
protectedslot |
Definition at line 674 of file ViewManager.cpp.
References isPlaying(), isRecording(), m_playbackFrame, m_playSource, and playbackFrameChanged().
Referenced by getOpportunisticEditingEnabled(), and viewCentreFrameChanged().
|
protected |
Definition at line 342 of file ViewManager.cpp.
References CommandHistory::addCommand(), CommandHistory::getInstance(), m_selections, and selectionChangedByUser().
Referenced by addSelection(), addSelectionQuietly(), clearSelections(), removeSelection(), and setSelection().
|
protected |
Definition at line 374 of file ViewManager.cpp.
References selectionChanged().
Referenced by ViewManager::SetSelectionCommand::execute(), and ViewManager::SetSelectionCommand::unexecute().
Member Data Documentation
|
protected |
!! void considerZoomChange(void *, int, bool);
Definition at line 346 of file ViewManager.h.
Referenced by checkPlayStatus(), getDeviceSampleRate(), getPlaybackFrame(), getPlaybackSampleRate(), isPlaying(), seek(), setAudioPlaySource(), and setPlaybackFrame().
|
protected |
Definition at line 347 of file ViewManager.h.
Referenced by checkPlayStatus(), getPlaybackFrame(), isRecording(), and setAudioRecordTarget().
|
protected |
Definition at line 349 of file ViewManager.h.
Referenced by getGlobalCentreFrame(), setGlobalCentreFrame(), and viewCentreFrameChanged().
|
protected |
Definition at line 350 of file ViewManager.h.
Referenced by getGlobalZoom(), and viewZoomLevelChanged().
|
mutableprotected |
Definition at line 351 of file ViewManager.h.
Referenced by checkPlayStatus(), getPlaybackFrame(), seek(), and setPlaybackFrame().
|
protected |
Definition at line 352 of file ViewManager.h.
Referenced by alignPlaybackFrameToReference(), alignReferenceToPlaybackFrame(), getPlaybackModel(), and setPlaybackModel().
|
protected |
Definition at line 353 of file ViewManager.h.
Referenced by getMainModelSampleRate(), setMainModelSampleRate(), and viewCentreFrameChanged().
|
protected |
Definition at line 355 of file ViewManager.h.
Referenced by checkPlayStatus().
|
protected |
Definition at line 356 of file ViewManager.h.
Referenced by checkPlayStatus().
|
protected |
Definition at line 358 of file ViewManager.h.
Referenced by addSelection(), addSelectionQuietly(), clearSelections(), ViewManager::SetSelectionCommand::execute(), getContainingSelection(), getSelection(), getSelections(), removeSelection(), setSelection(), setSelections(), and ViewManager::SetSelectionCommand::unexecute().
|
protected |
Definition at line 359 of file ViewManager.h.
Referenced by clearInProgressSelection(), getInProgressSelection(), haveInProgressSelection(), and setInProgressSelection().
|
protected |
Definition at line 360 of file ViewManager.h.
Referenced by getInProgressSelection(), and setInProgressSelection().
|
protected |
Definition at line 362 of file ViewManager.h.
Referenced by getClipboard().
|
protected |
Definition at line 364 of file ViewManager.h.
Referenced by getToolMode(), and setToolMode().
Definition at line 365 of file ViewManager.h.
Referenced by clearToolModeOverrides(), getToolModeFor(), and setToolModeFor().
|
protected |
Definition at line 367 of file ViewManager.h.
Referenced by getPlayLoopMode(), and setPlayLoopMode().
|
protected |
Definition at line 368 of file ViewManager.h.
Referenced by getPlaySelectionMode(), and setPlaySelectionMode().
|
protected |
Definition at line 369 of file ViewManager.h.
Referenced by getPlaySoloMode(), and setPlaySoloMode().
|
protected |
Definition at line 370 of file ViewManager.h.
Referenced by alignPlaybackFrameToReference(), alignReferenceToPlaybackFrame(), getAlignMode(), and setAlignMode().
|
protected |
Definition at line 390 of file ViewManager.h.
Referenced by getOverlayMode(), setOverlayMode(), shouldShowDuration(), shouldShowFeatureLabels(), shouldShowHorizontalValueScale(), shouldShowLayerNames(), shouldShowScaleGuides(), shouldShowSelectionExtents(), shouldShowVerticalColourScale(), shouldShowVerticalScale(), and ViewManager().
|
protected |
Definition at line 391 of file ViewManager.h.
Referenced by getZoomWheelsEnabled(), setZoomWheelsEnabled(), and ViewManager().
|
protected |
Definition at line 392 of file ViewManager.h.
Referenced by getOpportunisticEditingEnabled(), and setOpportunisticEditingEnabled().
|
protected |
Definition at line 393 of file ViewManager.h.
Referenced by setShowCentreLine(), shouldShowCentreLine(), shouldShowFrameCount(), and ViewManager().
|
protected |
Definition at line 394 of file ViewManager.h.
Referenced by setIlluminateLocalFeatures(), and shouldIlluminateLocalFeatures().
|
protected |
Definition at line 395 of file ViewManager.h.
Referenced by setShowWorkTitle(), and shouldShowWorkTitle().
|
protected |
Definition at line 396 of file ViewManager.h.
Referenced by setShowDuration(), and shouldShowDuration().
|
protected |
Definition at line 398 of file ViewManager.h.
Referenced by setGlobalDarkBackground(), and ViewManager().
|
protected |
Definition at line 399 of file ViewManager.h.
Referenced by setGlobalDarkBackground(), and ViewManager().
The documentation for this class was generated from the following files:
Generated by
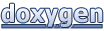