svgui
1.9
|
RegionLayer.cpp
Go to the documentation of this file.
288 // SVDEBUG << "RegionLayer::recalcSpacing: value found: " << e.getValue() << " (now have " << m_distributionMap[e.getValue()] << " of this value)" << endl;
296 // SVDEBUG << "RegionLayer::recalcSpacing: " << i->first << " -> " << m_spacingMap[i->first] << endl;
597 RegionLayer::getScaleExtents(LayerGeometryProvider *v, double &min, double &max, bool &log) const
616 // cerr << "RegionLayer[" << this << "]::getScaleExtents: min = " << min << ", max = " << max << ", log = " << log << endl;
622 // cerr << "RegionLayer[" << this << "]::getScaleExtents: min = " << min << ", max = " << max << ", log = " << log << endl;
634 // cerr << "RegionLayer[" << this << "]::getScaleExtents: equal spaced; min = " << min << ", max = " << max << ", log = " << log << endl;
689 // SVDEBUG << "RegionLayer::getYForValue: value " << val << " -> i->second " << i->second << " -> y " << y << endl;
697 // cerr << "RegionLayer[" << this << "]::getYForValue(" << val << "): min = " << min << ", max = " << max << ", log = " << logarithmic << endl;
748 // cerr << "getValueForY: y = " << y << ", vh = " << vh << ", ivh = " << ivh << " of " << n << ", iy = " << iy << ", dist = " << dist << ", gap = " << gap << endl;
1474 tr("The items you are pasting came from a layer with different source material from this one. Do you want to re-align them in time, to match the source material for this layer?"),
virtual int scalePixelSize(int size) const =0
int getCompletion(LayerGeometryProvider *) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: RegionLayer.cpp:66
double getValueForY(LayerGeometryProvider *v, int y) const override
Definition: RegionLayer.cpp:709
void drawEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1173
virtual bool snapToFeatureFrame(LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType, int) const
Adjust the given frame to snap to the nearest feature, if possible.
Definition: Layer.h:226
void drawDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1141
Definition: RegionLayer.h:97
void setFrameDuration(sv_frame_t frame)
Definition: ItemEditDialog.cpp:267
virtual QColor getForeground() const =0
QString getPropertyGroupName(const PropertyName &) const override
Definition: RegionLayer.cpp:136
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: RegionLayer.cpp:395
void getScaleExtents(LayerGeometryProvider *, double &min, double &max, bool &log) const
Definition: RegionLayer.cpp:597
PropertyList getProperties() const override
Definition: SingleColourLayer.cpp:60
virtual bool shouldIlluminateLocalFeatures(const Layer *, QPoint &) const =0
Definition: SingleColourLayer.h:24
void copy(LayerGeometryProvider *v, Selection s, Clipboard &to) override
Definition: RegionLayer.cpp:1446
static int scalePixelSize(int pixels)
Take a "design pixel" size and scale it for the actual display.
Definition: ViewManager.cpp:857
void paintVerticalScale(LayerGeometryProvider *v, bool, QPainter &paint, QRect rect) const override
Definition: RegionLayer.cpp:1073
void editStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1231
void modelReplaced()
static QString abbreviate(QString text, int maxLength, Policy policy=ElideEnd, bool fuzzy=true, QString ellipsis="")
Abbreviate the given text to the given maximum length (including ellipsis), using the given abbreviat...
Definition: TextAbbrev.cpp:79
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: RegionLayer.cpp:145
QColor getColourForValue(LayerGeometryProvider *v, double value) const override
Definition: RegionLayer.cpp:812
Definition: ItemEditDialog.h:34
int spacingIndexToY(LayerGeometryProvider *v, int i) const
Definition: RegionLayer.cpp:652
virtual QColor getForegroundQColor(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:253
bool getPointToDrag(LayerGeometryProvider *v, int x, int y, Event &) const
Definition: RegionLayer.cpp:354
void paintVertical(LayerGeometryProvider *v, const VerticalScaleLayer *layer, QPainter &paint, int x0, double minlog, double maxlog)
Definition: LogNumericalScale.cpp:40
bool isLayerScrollable(const LayerGeometryProvider *v) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: RegionLayer.cpp:268
QString getPropertyLabel(const PropertyName &) const override
Definition: RegionLayer.cpp:117
virtual sv_frame_t getFrameForX(int x) const =0
Return the closest frame to the given pixel x-coordinate.
PropertyType getPropertyType(const PropertyName &) const override
Definition: RegionLayer.cpp:126
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: RegionLayer.cpp:1566
void setVerticalScale(VerticalScale scale)
Definition: RegionLayer.cpp:260
Definition: Layer.h:198
static int getColourMapCount()
Return the number of known colour maps.
Definition: ColourMapper.cpp:142
void moveSelection(Selection s, sv_frame_t newStartFrame) override
Definition: RegionLayer.cpp:1365
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: RegionLayer.cpp:842
int getWidth(LayerGeometryProvider *v, QPainter &paint)
Definition: LinearNumericalScale.cpp:27
Definition: ItemEditDialog.h:28
void setProperty(const PropertyName &, int value) override
Definition: RegionLayer.cpp:218
virtual QColor getBaseQColor() const
Definition: SingleColourLayer.cpp:241
void layerParameterRangesChanged()
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SingleColourLayer.cpp:274
int getWidth(LayerGeometryProvider *v, QPainter &paint)
Definition: LogNumericalScale.cpp:28
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: RegionLayer.cpp:192
virtual bool snapToSimilarFeature(LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType) const
Adjust the given frame to snap to the next feature that has "effectively" the same value as the featu...
Definition: Layer.h:251
static int getBackwardCompatibilityColourMap(int n)
Older versions of colour-handling code save and reload colour maps by numerical index and can't prope...
Definition: ColourMapper.cpp:232
void modelChanged(ModelId)
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
static QString getColourMapLabel(int n)
Return a human-readable label for the colour map with the given index.
Definition: ColourMapper.cpp:148
double yToSpacingIndex(LayerGeometryProvider *v, int y) const
Definition: RegionLayer.cpp:663
EventVector getLocalPoints(LayerGeometryProvider *v, int x) const
Definition: RegionLayer.cpp:330
void deleteSelection(Selection s) override
Definition: RegionLayer.cpp:1422
int getColourIndex(QString name) const
Return the index of the colour with the given name, if found in the database.
Definition: ColourDatabase.cpp:67
virtual sv_frame_t alignFromReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:198
QString getPropertyGroupName(const PropertyName &) const override
Definition: SingleColourLayer.cpp:82
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: RegionLayer.cpp:1541
Definition: RegionLayer.h:107
static int getColourMapById(QString id)
Return the index for the colour map with the given machine-readable id string, or -1 if the id is not...
Definition: ColourMapper.cpp:204
PropertyList getProperties() const override
Definition: RegionLayer.cpp:107
void layerParametersChanged()
Definition: ItemEditDialog.h:37
bool snapToFeatureFrame(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override
Adjust the given frame to snap to the nearest feature, if possible.
Definition: RegionLayer.cpp:467
bool clipboardHasDifferentAlignment(LayerGeometryProvider *v, const Clipboard &clip) const
Definition: Layer.cpp:209
int getWidth(LayerGeometryProvider *v, QPainter &paint)
Definition: LinearColourScale.cpp:26
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SingleColourLayer.cpp:88
int getVerticalScaleWidth(LayerGeometryProvider *v, bool, QPainter &) const override
Definition: RegionLayer.cpp:1050
bool editOpen(LayerGeometryProvider *v, QMouseEvent *) override
Open an editor on the item under the mouse (e.g.
Definition: RegionLayer.cpp:1323
virtual sv_frame_t alignToReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:187
bool getDisplayExtents(double &min, double &max) const override
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: RegionLayer.cpp:316
void paintVertical(LayerGeometryProvider *v, const VerticalScaleLayer *layer, QPainter &paint, int x0, double minf, double maxf)
Definition: LinearNumericalScale.cpp:39
A class for mapping intensity values onto various colour maps.
Definition: ColourMapper.h:27
int getWidth(LayerGeometryProvider *v, QPainter &paint)
Definition: LogColourScale.cpp:28
Definition: LogColourScale.h:25
void editEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1294
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
Definition: ItemEditDialog.h:36
PropertyType getPropertyType(const PropertyName &) const override
Definition: SingleColourLayer.cpp:75
Definition: ItemEditDialog.h:35
void paintVertical(LayerGeometryProvider *v, const ColourScaleLayer *layer, QPainter &paint, int x0, double minf, double maxf)
Definition: LinearColourScale.cpp:38
Definition: RegionLayer.h:99
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SingleColourLayer.cpp:294
Definition: Layer.h:196
void resizeSelection(Selection s, Selection newSize) override
Definition: RegionLayer.cpp:1390
Definition: LinearNumericalScale.h:25
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SingleColourLayer.cpp:113
Definition: PaintAssistant.h:43
static QString getColourMapId(int n)
Return a machine-readable id string for the colour map with the given index.
Definition: ColourMapper.cpp:177
virtual int getTextLabelYCoord(const Layer *layer, QPainter &) const =0
Return a y-coordinate at which text labels for individual items in a layer may be drawn...
static void drawVisibleText(const LayerGeometryProvider *, QPainter &p, int x, int y, QString text, TextStyle style)
Definition: PaintAssistant.cpp:199
Definition: LinearColourScale.h:25
virtual bool getVisibleExtentsForUnit(QString unit, double &min, double &max, bool &log) const =0
Return the visible vertical extents for the given unit, if any.
void eraseStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1185
void eraseEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1207
int getYForValue(LayerGeometryProvider *v, double value) const override
VerticalScaleLayer and ColourScaleLayer methods.
Definition: RegionLayer.cpp:674
void editDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1257
void drawStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1117
void setProperty(const PropertyName &, int value) override
Definition: SingleColourLayer.cpp:132
QString getLabelPreceding(sv_frame_t) const override
Definition: RegionLayer.cpp:378
bool snapToSimilarFeature(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap) const override
Adjust the given frame to snap to the next feature that has "effectively" the same value as the featu...
Definition: RegionLayer.cpp:542
virtual int getXForFrame(sv_frame_t frame) const =0
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative)...
virtual int getPaintWidth() const
Definition: LayerGeometryProvider.h:187
void eraseDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: RegionLayer.cpp:1202
sv_frame_t getFrameDuration() const
Definition: ItemEditDialog.cpp:280
int getDefaultColourHint(bool dark, bool &impose) override
Definition: RegionLayer.cpp:834
QString getPropertyLabel(const PropertyName &) const override
Definition: SingleColourLayer.cpp:68
Definition: RegionLayer.h:96
void paintVertical(LayerGeometryProvider *v, const ColourScaleLayer *layer, QPainter &paint, int x0, double minf, double maxf)
Definition: LogColourScale.cpp:40
virtual View * getView()=0
bool getValueExtents(double &min, double &max, bool &log, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: RegionLayer.cpp:301
Definition: LogNumericalScale.h:25
bool paste(LayerGeometryProvider *v, const Clipboard &from, sv_frame_t frameOffset, bool interactive) override
Paste from the given clipboard onto the layer at the given frame offset.
Definition: RegionLayer.cpp:1460
Generated by
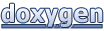