svgui
1.9
|
#include <TextAbbrev.h>
Public Types | |
enum | Policy { ElideEnd, ElideEndAndCommonPrefixes, ElideStart, ElideMiddle } |
Static Public Member Functions | |
static QString | abbreviate (QString text, int maxLength, Policy policy=ElideEnd, bool fuzzy=true, QString ellipsis="") |
Abbreviate the given text to the given maximum length (including ellipsis), using the given abbreviation policy. More... | |
static QString | abbreviate (QString text, const QFontMetrics &metrics, int &maxWidth, Policy policy=ElideEnd, QString ellipsis="") |
Abbreviate the given text to the given maximum painted width, using the given abbreviation policy. More... | |
static QStringList | abbreviate (const QStringList &texts, int maxLength, Policy policy=ElideEndAndCommonPrefixes, bool fuzzy=true, QString ellipsis="") |
Abbreviate all of the given texts to the given maximum length, using the given abbreviation policy. More... | |
static QStringList | abbreviate (const QStringList &texts, const QFontMetrics &metrics, int &maxWidth, Policy policy=ElideEndAndCommonPrefixes, QString ellipsis="") |
Abbreviate all of the given texts to the given maximum painted width, using the given abbreviation policy. More... | |
Static Protected Member Functions | |
static QString | getDefaultEllipsis () |
static int | getFuzzLength (QString ellipsis) |
static int | getFuzzWidth (const QFontMetrics &metrics, QString ellipsis) |
static QString | abbreviateTo (QString text, int characters, Policy policy, QString ellipsis) |
static QStringList | elidePrefixes (const QStringList &texts, int targetReduction, QString ellipsis) |
static QStringList | elidePrefixes (const QStringList &texts, const QFontMetrics &metrics, int targetWidthReduction, QString ellipsis) |
static int | getPrefixLength (const QStringList &texts) |
Detailed Description
Definition at line 24 of file TextAbbrev.h.
Member Enumeration Documentation
enum TextAbbrev::Policy |
Enumerator | |
---|---|
ElideEnd | |
ElideEndAndCommonPrefixes | |
ElideStart | |
ElideMiddle |
Definition at line 27 of file TextAbbrev.h.
Member Function Documentation
|
static |
Abbreviate the given text to the given maximum length (including ellipsis), using the given abbreviation policy.
If fuzzy is true, the text will be left alone if it is "not much more than" the maximum length.
If ellipsis is non-empty, it will be used to show elisions in preference to the default (which is "...").
Definition at line 79 of file TextAbbrev.cpp.
References abbreviateTo(), getDefaultEllipsis(), and getFuzzLength().
Referenced by abbreviate(), CSVAudioFormatDialog::CSVAudioFormatDialog(), Pane::drawLayerNames(), elidePrefixes(), FlexiNoteLayer::paintVerticalScale(), BoxLayer::paintVerticalScale(), TimeValueLayer::paintVerticalScale(), RegionLayer::paintVerticalScale(), NoteLayer::paintVerticalScale(), CSVFormatDialog::repopulate(), and PluginParameterDialog::setCandidateInputModels().
|
static |
Abbreviate the given text to the given maximum painted width, using the given abbreviation policy.
maxWidth is also modified so as to return the painted width of the abbreviated text.
If ellipsis is non-empty, it will be used to show elisions in preference to the default (which is tr("...")).
Definition at line 92 of file TextAbbrev.cpp.
References abbreviateTo(), and getDefaultEllipsis().
|
static |
Abbreviate all of the given texts to the given maximum length, using the given abbreviation policy.
If fuzzy is true, texts that are "not much more than" the maximum length will be left alone.
If ellipsis is non-empty, it will be used to show elisions in preference to the default (which is tr("...")).
Definition at line 126 of file TextAbbrev.cpp.
References abbreviate(), ElideEnd, ElideEndAndCommonPrefixes, elidePrefixes(), getDefaultEllipsis(), and getFuzzLength().
|
static |
Abbreviate all of the given texts to the given maximum painted width, using the given abbreviation policy.
maxWidth is also modified so as to return the maximum painted width of the abbreviated texts.
If ellipsis is non-empty, it will be used to show elisions in preference to the default (which is tr("...")).
Definition at line 157 of file TextAbbrev.cpp.
References abbreviate(), ElideEnd, ElideEndAndCommonPrefixes, elidePrefixes(), and getDefaultEllipsis().
|
staticprotected |
Definition at line 24 of file TextAbbrev.cpp.
Referenced by abbreviate().
|
staticprotected |
Definition at line 30 of file TextAbbrev.cpp.
Referenced by abbreviate(), and elidePrefixes().
|
staticprotected |
Definition at line 39 of file TextAbbrev.cpp.
|
staticprotected |
Definition at line 51 of file TextAbbrev.cpp.
References ElideEnd, ElideEndAndCommonPrefixes, ElideMiddle, and ElideStart.
Referenced by abbreviate().
|
staticprotected |
Definition at line 189 of file TextAbbrev.cpp.
References abbreviate(), ElideEnd, getFuzzLength(), and getPrefixLength().
Referenced by abbreviate().
|
staticprotected |
Definition at line 216 of file TextAbbrev.cpp.
References abbreviate(), ElideEnd, getFuzzLength(), and getPrefixLength().
|
staticprotected |
Definition at line 250 of file TextAbbrev.cpp.
References havePrefix().
Referenced by elidePrefixes().
The documentation for this class was generated from the following files:
Generated by
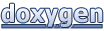