svgui
1.9
|
SingleColourLayer.h
Go to the documentation of this file.
ColourSignificance getLayerColourSignificance() const override
Implements Layer::getLayerColourSignificance()
Definition: SingleColourLayer.h:52
virtual void flagBaseColourChanged()
Definition: SingleColourLayer.h:85
Definition: Layer.h:351
PropertyList getProperties() const override
Definition: SingleColourLayer.cpp:60
Definition: SingleColourLayer.h:24
bool m_colourExplicitlySet
Definition: SingleColourLayer.h:93
QPixmap getLayerPresentationPixmap(QSize size) const override
Definition: SingleColourLayer.cpp:47
virtual void setBaseColour(int)
Set the colour used to draw primary items in the layer.
Definition: SingleColourLayer.cpp:220
virtual QColor getForegroundQColor(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:253
virtual ~SingleColourLayer()
Definition: SingleColourLayer.cpp:41
std::vector< QColor > getPartialShades(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:259
virtual QColor getBaseQColor() const
Definition: SingleColourLayer.cpp:241
virtual int getDefaultColourHint(bool, bool &)
Definition: SingleColourLayer.h:86
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SingleColourLayer.cpp:274
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
static ColourRefCount m_colourRefCount
Definition: SingleColourLayer.h:90
QString getPropertyGroupName(const PropertyName &) const override
Definition: SingleColourLayer.cpp:82
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SingleColourLayer.cpp:88
virtual void setDefaultColourFor(LayerGeometryProvider *v)
Definition: SingleColourLayer.cpp:140
PropertyType getPropertyType(const PropertyName &) const override
Definition: SingleColourLayer.cpp:75
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SingleColourLayer.cpp:294
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SingleColourLayer.cpp:113
std::map< int, int > ColourRefCount
Definition: SingleColourLayer.h:89
bool hasLightBackground() const override
Return true if the layer currently has a dark colour on a light background, false if it has a light c...
Definition: SingleColourLayer.cpp:53
virtual QColor getBackgroundQColor(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:247
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SingleColourLayer.cpp:126
void setProperty(const PropertyName &, int value) override
Definition: SingleColourLayer.cpp:132
QString getPropertyLabel(const PropertyName &) const override
Definition: SingleColourLayer.cpp:68
virtual int getBaseColour() const
Retrieve the current primary drawing colour, as a ColourDatabase index value.
Definition: SingleColourLayer.cpp:235
Generated by
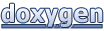