svgui
1.9
|
SingleColourLayer.cpp
Go to the documentation of this file.
143 SVDEBUG << "SingleColourLayer::setDefaultColourFor: m_colourExplicitlySet = " << m_colourExplicitlySet << ", m_defaultColourSet " << m_defaultColourSet << endl;
311 SVDEBUG << "SingleColourLayer::setProperties: changing colour from " << m_colour << " to " << colour << endl;
QString getColourName(int c) const
Return the name of the colour at index c.
Definition: ColourDatabase.cpp:43
virtual void flagBaseColourChanged()
Definition: SingleColourLayer.h:85
virtual QColor getForeground() const =0
virtual QColor getBackground() const =0
PropertyList getProperties() const override
Definition: SingleColourLayer.cpp:60
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Convert the layer's data (though not those of the model it refers to) into XML for file output...
Definition: Layer.cpp:653
bool m_colourExplicitlySet
Definition: SingleColourLayer.h:93
QPixmap getLayerPresentationPixmap(QSize size) const override
Definition: SingleColourLayer.cpp:47
virtual void setBaseColour(int)
Set the colour used to draw primary items in the layer.
Definition: SingleColourLayer.cpp:220
bool useDarkBackground(int c) const
Return true if the colour at index c is marked as using a dark background.
Definition: ColourDatabase.cpp:183
virtual QColor getForegroundQColor(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:253
virtual ~SingleColourLayer()
Definition: SingleColourLayer.cpp:41
void getColourPropertyRange(int *min, int *max) const
Definition: ColourDatabase.cpp:273
int putStringValues(QString colourName, QString colourSpec, QString darkbg)
Definition: ColourDatabase.cpp:251
std::vector< QColor > getPartialShades(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:259
virtual QColor getBaseQColor() const
Definition: SingleColourLayer.cpp:241
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SingleColourLayer.cpp:274
virtual int getDefaultColourHint(bool, bool &)
Definition: SingleColourLayer.h:86
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
int getColourCount() const
Return the number of colours in the database.
Definition: ColourDatabase.cpp:37
static ColourRefCount m_colourRefCount
Definition: SingleColourLayer.h:90
QString getPropertyGroupName(const PropertyName &) const override
Definition: SingleColourLayer.cpp:82
void layerParametersChanged()
void getStringValues(int index, QString &colourName, QString &colourSpec, QString &darkbg) const
Definition: ColourDatabase.cpp:235
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SingleColourLayer.cpp:88
virtual void setDefaultColourFor(LayerGeometryProvider *v)
Definition: SingleColourLayer.cpp:140
PropertyType getPropertyType(const PropertyName &) const override
Definition: SingleColourLayer.cpp:75
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SingleColourLayer.cpp:294
Definition: ColourDatabase.h:26
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SingleColourLayer.cpp:113
std::map< int, int > ColourRefCount
Definition: SingleColourLayer.h:89
bool hasLightBackground() const override
Return true if the layer currently has a dark colour on a light background, false if it has a light c...
Definition: SingleColourLayer.cpp:53
virtual QColor getBackgroundQColor(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:247
virtual bool hasLightBackground() const =0
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SingleColourLayer.cpp:126
void setProperty(const PropertyName &, int value) override
Definition: SingleColourLayer.cpp:132
QString getPropertyLabel(const PropertyName &) const override
Definition: SingleColourLayer.cpp:68
virtual int getBaseColour() const
Retrieve the current primary drawing colour, as a ColourDatabase index value.
Definition: SingleColourLayer.cpp:235
QPixmap getExamplePixmap(int c, QSize size) const
Generate a swatch pixmap illustrating the colour at index c.
Definition: ColourDatabase.cpp:284
Generated by
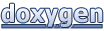