svgui
1.9
|
ColourDatabase.h
Go to the documentation of this file.
QString getColourName(int c) const
Return the name of the colour at index c.
Definition: ColourDatabase.cpp:43
QColor getContrastingColour(int c) const
Return a colour that contrasts with the one at index c, according to some simple algorithm.
Definition: ColourDatabase.cpp:149
void colourDatabaseChanged()
int getNearbyColourIndex(QColor c, WithBackgroundMode mode=WithAnyBackground) const
Return the index of the colour in the database that is closest to the given one, by some simple measu...
Definition: ColourDatabase.cpp:91
void removeColour(QString)
Remove the colour with the given name from the database.
Definition: ColourDatabase.cpp:223
bool useDarkBackground(int c) const
Return true if the colour at index c is marked as using a dark background.
Definition: ColourDatabase.cpp:183
void getColourPropertyRange(int *min, int *max) const
Definition: ColourDatabase.cpp:273
int putStringValues(QString colourName, QString colourSpec, QString darkbg)
Definition: ColourDatabase.cpp:251
void setUseDarkBackground(int c, bool dark)
Mark the colour at index c as using a dark background.
Definition: ColourDatabase.cpp:190
int getColourCount() const
Return the number of colours in the database.
Definition: ColourDatabase.cpp:37
int getColourIndex(QString name) const
Return the index of the colour with the given name, if found in the database.
Definition: ColourDatabase.cpp:67
Definition: ColourDatabase.h:151
void getStringValues(int index, QString &colourName, QString &colourSpec, QString &darkbg) const
Definition: ColourDatabase.cpp:235
bool haveColour(QColor c) const
Return true if the given colour exists in the database.
Definition: ColourDatabase.h:26
int addColour(QColor c, QString name)
Add a colour to the database, with the associated name.
Definition: ColourDatabase.cpp:200
QPixmap getExamplePixmap(int c, QSize size) const
Generate a swatch pixmap illustrating the colour at index c.
Definition: ColourDatabase.cpp:284
Generated by
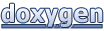