svgui
1.9
|
#include <ColourDatabase.h>
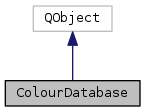
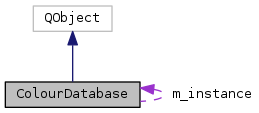
Classes | |
struct | ColourRec |
Public Types | |
enum | WithBackgroundMode { WithAnyBackground, WithDarkBackground, WithLightBackground } |
Signals | |
void | colourDatabaseChanged () |
Public Member Functions | |
int | getColourCount () const |
Return the number of colours in the database. More... | |
QString | getColourName (int c) const |
Return the name of the colour at index c. More... | |
QColor | getColour (int c) const |
Return the colour at index c. More... | |
QColor | getColour (QString name) const |
Return the colour with the given name, if found in the database. More... | |
int | getColourIndex (QString name) const |
Return the index of the colour with the given name, if found in the database. More... | |
int | getColourIndex (QColor c) const |
Return the index of the given colour, if found in the database. More... | |
bool | haveColour (QColor c) const |
Return true if the given colour exists in the database. More... | |
int | getNearbyColourIndex (QColor c, WithBackgroundMode mode=WithAnyBackground) const |
Return the index of the colour in the database that is closest to the given one, by some simple measure. More... | |
int | addColour (QColor c, QString name) |
Add a colour to the database, with the associated name. More... | |
void | removeColour (QString) |
Remove the colour with the given name from the database. More... | |
bool | useDarkBackground (int c) const |
Return true if the colour at index c is marked as using a dark background. More... | |
void | setUseDarkBackground (int c, bool dark) |
Mark the colour at index c as using a dark background. More... | |
QColor | getContrastingColour (int c) const |
Return a colour that contrasts with the one at index c, according to some simple algorithm. More... | |
void | getStringValues (int index, QString &colourName, QString &colourSpec, QString &darkbg) const |
int | putStringValues (QString colourName, QString colourSpec, QString darkbg) |
void | getColourPropertyRange (int *min, int *max) const |
QPixmap | getExamplePixmap (int c, QSize size) const |
Generate a swatch pixmap illustrating the colour at index c. More... | |
Static Public Member Functions | |
static ColourDatabase * | getInstance () |
Protected Types | |
typedef std::vector< ColourRec > | ColourList |
Protected Member Functions | |
ColourDatabase () | |
Protected Attributes | |
ColourList | m_colours |
Static Protected Attributes | |
static ColourDatabase | m_instance |
Detailed Description
Definition at line 26 of file ColourDatabase.h.
Member Typedef Documentation
|
protected |
Definition at line 157 of file ColourDatabase.h.
Member Enumeration Documentation
Enumerator | |
---|---|
WithAnyBackground | |
WithDarkBackground | |
WithLightBackground |
Definition at line 74 of file ColourDatabase.h.
Constructor & Destructor Documentation
|
protected |
Definition at line 32 of file ColourDatabase.cpp.
Member Function Documentation
|
static |
Definition at line 27 of file ColourDatabase.cpp.
References m_instance.
Referenced by ColourComboBox::ColourComboBox(), ColourComboBox::comboActivated(), SingleColourLayer::getBaseQColor(), getColourPropertyRange(), TimeRulerLayer::getDefaultColourHint(), TextLayer::getDefaultColourHint(), TimeInstantLayer::getDefaultColourHint(), RegionLayer::getDefaultColourHint(), NoteLayer::getDefaultColourHint(), SliceLayer::getDefaultColourHint(), FlexiNoteLayer::getDefaultColourHint(), TimeValueLayer::getDefaultColourHint(), SingleColourLayer::getLayerPresentationPixmap(), SingleColourLayer::getPropertyRangeAndValue(), SingleColourLayer::getPropertyValueLabel(), SingleColourLayer::hasLightBackground(), WaveformLayer::paintChannel(), ColourComboBox::rebuild(), SingleColourLayer::setDefaultColourFor(), SingleColourLayer::setProperties(), SingleColourLayer::toXml(), and WaveformLayer::toXml().
int ColourDatabase::getColourCount | ( | ) | const |
Return the number of colours in the database.
Definition at line 37 of file ColourDatabase.cpp.
References m_colours.
Referenced by getColourPropertyRange(), ColourComboBox::rebuild(), and SingleColourLayer::setDefaultColourFor().
QString ColourDatabase::getColourName | ( | int | c | ) | const |
Return the name of the colour at index c.
Definition at line 43 of file ColourDatabase.cpp.
References m_colours.
Referenced by SingleColourLayer::getPropertyValueLabel(), getStringValues(), and ColourComboBox::rebuild().
QColor ColourDatabase::getColour | ( | int | c | ) | const |
Return the colour at index c.
Definition at line 50 of file ColourDatabase.cpp.
References m_colours.
Referenced by SingleColourLayer::getBaseQColor(), getContrastingColour(), getExamplePixmap(), and getStringValues().
QColor ColourDatabase::getColour | ( | QString | name | ) | const |
Return the colour with the given name, if found in the database.
If not found, return Qt::black.
Definition at line 57 of file ColourDatabase.cpp.
References m_colours.
int ColourDatabase::getColourIndex | ( | QString | name | ) | const |
Return the index of the colour with the given name, if found in the database.
If not found, return -1.
Definition at line 67 of file ColourDatabase.cpp.
References m_colours.
Referenced by TimeRulerLayer::getDefaultColourHint(), TextLayer::getDefaultColourHint(), TimeInstantLayer::getDefaultColourHint(), RegionLayer::getDefaultColourHint(), NoteLayer::getDefaultColourHint(), SliceLayer::getDefaultColourHint(), FlexiNoteLayer::getDefaultColourHint(), TimeValueLayer::getDefaultColourHint(), and putStringValues().
int ColourDatabase::getColourIndex | ( | QColor | c | ) | const |
Return the index of the given colour, if found in the database.
If not found, return -1. Note that it is possible for a colour to appear more than once in the database: names have to be unique in the database, but colours don't. This always returns the first match.
Definition at line 79 of file ColourDatabase.cpp.
References m_colours.
bool ColourDatabase::haveColour | ( | QColor | c | ) | const |
Return true if the given colour exists in the database.
int ColourDatabase::getNearbyColourIndex | ( | QColor | c, |
WithBackgroundMode | mode = WithAnyBackground |
||
) | const |
Return the index of the colour in the database that is closest to the given one, by some simple measure.
This always returns some valid index, unless the database is empty, in which case it returns -1.
Definition at line 91 of file ColourDatabase.cpp.
References m_colours, WithDarkBackground, and WithLightBackground.
int ColourDatabase::addColour | ( | QColor | c, |
QString | name | ||
) |
Add a colour to the database, with the associated name.
Return the index of the colour in the database. Names are unique within the database: if another colour exists already with the given name, its colour value is replaced with the given one. Colours may appear more than once under different names.
Definition at line 200 of file ColourDatabase.cpp.
References ColourDatabase::ColourRec::colour, colourDatabaseChanged(), ColourDatabase::ColourRec::darkbg, m_colours, and ColourDatabase::ColourRec::name.
Referenced by ColourComboBox::comboActivated(), and putStringValues().
void ColourDatabase::removeColour | ( | QString | name | ) |
Remove the colour with the given name from the database.
Definition at line 223 of file ColourDatabase.cpp.
References m_colours.
bool ColourDatabase::useDarkBackground | ( | int | c | ) | const |
Return true if the colour at index c is marked as using a dark background.
Such colours are presumably "bright" ones, but all this reports is whether the colour has been marked with setUseDarkBackground, not any intrinsic property of the colour.
Definition at line 183 of file ColourDatabase.cpp.
References m_colours.
Referenced by getExamplePixmap(), getStringValues(), SingleColourLayer::hasLightBackground(), and SingleColourLayer::setDefaultColourFor().
void ColourDatabase::setUseDarkBackground | ( | int | c, |
bool | dark | ||
) |
Mark the colour at index c as using a dark background.
Generally this should be called for "bright" colours.
Definition at line 190 of file ColourDatabase.cpp.
References colourDatabaseChanged(), and m_colours.
Referenced by ColourComboBox::comboActivated(), and putStringValues().
QColor ColourDatabase::getContrastingColour | ( | int | c | ) | const |
Return a colour that contrasts with the one at index c, according to some simple algorithm.
The returned colour is not necessarily in the database; pass it to getNearbyColourIndex if you need one that is.
Definition at line 149 of file ColourDatabase.cpp.
References getColour().
void ColourDatabase::getStringValues | ( | int | index, |
QString & | colourName, | ||
QString & | colourSpec, | ||
QString & | darkbg | ||
) | const |
Definition at line 235 of file ColourDatabase.cpp.
References getColour(), getColourName(), m_colours, and useDarkBackground().
Referenced by SingleColourLayer::toXml(), and WaveformLayer::toXml().
int ColourDatabase::putStringValues | ( | QString | colourName, |
QString | colourSpec, | ||
QString | darkbg | ||
) |
Definition at line 251 of file ColourDatabase.cpp.
References addColour(), getColourIndex(), and setUseDarkBackground().
Referenced by SingleColourLayer::setProperties().
void ColourDatabase::getColourPropertyRange | ( | int * | min, |
int * | max | ||
) | const |
Definition at line 273 of file ColourDatabase.cpp.
References getColourCount(), and getInstance().
Referenced by SingleColourLayer::getPropertyRangeAndValue().
QPixmap ColourDatabase::getExamplePixmap | ( | int | c, |
QSize | size | ||
) | const |
Generate a swatch pixmap illustrating the colour at index c.
Definition at line 284 of file ColourDatabase.cpp.
References getColour(), and useDarkBackground().
Referenced by SingleColourLayer::getLayerPresentationPixmap(), and ColourComboBox::rebuild().
|
signal |
Referenced by addColour(), and setUseDarkBackground().
Member Data Documentation
|
protected |
Definition at line 158 of file ColourDatabase.h.
Referenced by addColour(), getColour(), getColourCount(), getColourIndex(), getColourName(), getNearbyColourIndex(), getStringValues(), removeColour(), setUseDarkBackground(), and useDarkBackground().
|
staticprotected |
Definition at line 160 of file ColourDatabase.h.
Referenced by getInstance().
The documentation for this class was generated from the following files:
Generated by
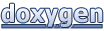