svgui
1.9
|
TimeInstantLayer.cpp
Go to the documentation of this file.
814 tr("The instants you are pasting came from a layer with different source material from this one. Do you want to re-align them in time, to match the source material for this layer?"),
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: TimeInstantLayer.cpp:129
virtual bool snapToFeatureFrame(LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType, int) const
Adjust the given frame to snap to the nearest feature, if possible.
Definition: Layer.h:226
QString getLabelPreceding(sv_frame_t) const override
Definition: TimeInstantLayer.cpp:223
void drawStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:504
PropertyList getProperties() const override
Definition: SingleColourLayer.cpp:60
virtual bool shouldIlluminateLocalFeatures(const Layer *, QPoint &) const =0
Definition: SingleColourLayer.h:24
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: TimeInstantLayer.h:67
void editStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:610
void modelReplaced()
QString getPropertyLabel(const PropertyName &) const override
Definition: TimeInstantLayer.cpp:93
void drawEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:545
Definition: ItemEditDialog.h:34
bool needsTextLabelHeight() const override
True if this layer will need to place text labels when it is painted.
Definition: TimeInstantLayer.cpp:161
virtual QColor getForegroundQColor(LayerGeometryProvider *v) const
Definition: SingleColourLayer.cpp:253
bool paste(LayerGeometryProvider *v, const Clipboard &from, sv_frame_t frameOffset, bool interactive) override
Paste from the given clipboard onto the layer at the given frame offset.
Definition: TimeInstantLayer.cpp:800
void eraseEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:591
virtual sv_frame_t getFrameForX(int x) const =0
Return the closest frame to the given pixel x-coordinate.
void eraseDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:586
void resizeSelection(Selection s, Selection newSize) override
Definition: TimeInstantLayer.cpp:738
void setProperty(const PropertyName &, int value) override
Definition: TimeInstantLayer.cpp:143
Definition: Layer.h:198
PropertyType getPropertyType(const PropertyName &) const override
Definition: TimeInstantLayer.cpp:100
Definition: ItemEditDialog.h:28
void editDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:633
virtual double scaleSize(double size) const =0
virtual QColor getBaseQColor() const
Definition: SingleColourLayer.cpp:241
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SingleColourLayer.cpp:274
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
int getCompletion(LayerGeometryProvider *) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: TimeInstantLayer.cpp:56
void copy(LayerGeometryProvider *v, Selection s, Clipboard &to) override
Definition: TimeInstantLayer.cpp:786
int getColourIndex(QString name) const
Return the index of the colour with the given name, if found in the database.
Definition: ColourDatabase.cpp:67
virtual sv_frame_t alignFromReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:198
void setPlotStyle(PlotStyle style)
Definition: TimeInstantLayer.cpp:153
void layerParametersChanged()
Definition: ItemEditDialog.h:37
bool isLayerScrollable(const LayerGeometryProvider *v) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: TimeInstantLayer.cpp:169
PropertyList getProperties() const override
Definition: TimeInstantLayer.cpp:85
bool clipboardHasDifferentAlignment(LayerGeometryProvider *v, const Clipboard &clip) const
Definition: Layer.cpp:209
ChangeEventsCommand * m_editingCommand
Definition: TimeInstantLayer.h:124
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SingleColourLayer.cpp:88
virtual sv_frame_t alignToReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:187
EventVector getLocalPoints(LayerGeometryProvider *v, int) const
Definition: TimeInstantLayer.cpp:176
void deleteSelection(Selection s) override
Definition: TimeInstantLayer.cpp:767
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
void drawDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:527
PropertyType getPropertyType(const PropertyName &) const override
Definition: SingleColourLayer.cpp:75
void moveSelection(Selection s, sv_frame_t newStartFrame) override
Definition: TimeInstantLayer.cpp:716
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SingleColourLayer.cpp:294
Definition: Layer.h:196
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: TimeInstantLayer.cpp:317
int getDefaultColourHint(bool dark, bool &impose) override
Definition: TimeInstantLayer.cpp:869
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: TimeInstantLayer.cpp:107
void eraseStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:567
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: TimeInstantLayer.cpp:887
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SingleColourLayer.cpp:113
bool snapToFeatureFrame(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override
Adjust the given frame to snap to the nearest feature, if possible.
Definition: TimeInstantLayer.cpp:278
Definition: PaintAssistant.h:43
virtual int getTextLabelYCoord(const Layer *layer, QPainter &) const =0
Return a y-coordinate at which text labels for individual items in a layer may be drawn...
static void drawVisibleText(const LayerGeometryProvider *, QPainter &p, int x, int y, QString text, TextStyle style)
Definition: PaintAssistant.cpp:199
virtual sv_frame_t getEndFrame() const =0
Retrieve the last visible sample frame on the widget.
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: TimeInstantLayer.cpp:241
void setProperty(const PropertyName &, int value) override
Definition: SingleColourLayer.cpp:132
virtual int getXForFrame(sv_frame_t frame) const =0
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative)...
virtual int getPaintWidth() const
Definition: LayerGeometryProvider.h:187
bool editOpen(LayerGeometryProvider *, QMouseEvent *) override
Open an editor on the item under the mouse (e.g.
Definition: TimeInstantLayer.cpp:680
QString getPropertyLabel(const PropertyName &) const override
Definition: SingleColourLayer.cpp:68
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: TimeInstantLayer.cpp:877
virtual View * getView()=0
void editEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: TimeInstantLayer.cpp:656
Generated by
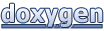