svgui
1.9
|
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer. More...
#include <LayerGeometryProvider.h>
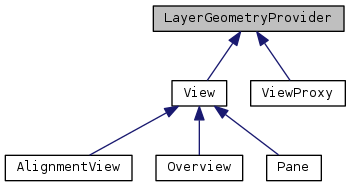
Public Member Functions | |
LayerGeometryProvider () | |
virtual int | getId () const =0 |
Retrieve the id of this object. More... | |
virtual sv_frame_t | getStartFrame () const =0 |
Retrieve the first visible sample frame on the widget. More... | |
virtual sv_frame_t | getCentreFrame () const =0 |
Return the centre frame of the visible widget. More... | |
virtual sv_frame_t | getEndFrame () const =0 |
Retrieve the last visible sample frame on the widget. More... | |
virtual int | getXForFrame (sv_frame_t frame) const =0 |
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative). More... | |
virtual sv_frame_t | getFrameForX (int x) const =0 |
Return the closest frame to the given pixel x-coordinate. More... | |
virtual sv_frame_t | getModelsStartFrame () const =0 |
virtual sv_frame_t | getModelsEndFrame () const =0 |
virtual int | getXForViewX (int viewx) const =0 |
Return the closest pixel x-coordinate corresponding to a given view x-coordinate. More... | |
virtual int | getViewXForX (int x) const =0 |
Return the closest view x-coordinate corresponding to a given pixel x-coordinate. More... | |
virtual double | getYForFrequency (double frequency, double minFreq, double maxFreq, bool logarithmic) const =0 |
Return the (maybe fractional) pixel y-coordinate corresponding to a given frequency, if the frequency range is as specified. More... | |
virtual double | getFrequencyForY (double y, double minFreq, double maxFreq, bool logarithmic) const =0 |
Return the closest frequency to the given (maybe fractional) pixel y-coordinate, if the frequency range is as specified. More... | |
virtual int | getTextLabelYCoord (const Layer *layer, QPainter &) const =0 |
Return a y-coordinate at which text labels for individual items in a layer may be drawn, so as not to overlap with those of other layers. More... | |
virtual bool | getVisibleExtentsForUnit (QString unit, double &min, double &max, bool &log) const =0 |
Return the visible vertical extents for the given unit, if any. More... | |
virtual ZoomLevel | getZoomLevel () const =0 |
Return the zoom level, i.e. More... | |
virtual QRect | getPaintRect () const =0 |
To be called from a layer, to obtain the extent of the surface that the layer is currently painting to. More... | |
virtual QSize | getPaintSize () const |
virtual int | getPaintWidth () const |
virtual int | getPaintHeight () const |
virtual bool | hasLightBackground () const =0 |
virtual QColor | getForeground () const =0 |
virtual QColor | getBackground () const =0 |
virtual ViewManager * | getViewManager () const =0 |
virtual bool | shouldIlluminateLocalFeatures (const Layer *, QPoint &) const =0 |
virtual bool | shouldShowFeatureLabels () const =0 |
virtual void | drawMeasurementRect (QPainter &p, const Layer *, QRect rect, bool focus) const =0 |
virtual void | updatePaintRect (QRect r)=0 |
virtual double | scaleSize (double size) const =0 |
virtual int | scalePixelSize (int size) const =0 |
virtual double | scalePenWidth (double width) const =0 |
virtual QPen | scalePen (QPen pen) const =0 |
virtual View * | getView ()=0 |
virtual const View * | getView () const =0 |
Static Protected Member Functions | |
static int | getNextId () |
Detailed Description
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
The main implementor of this interface is the View class, but other implementations may be used in different circumstances, e.g. as a proxy to handle hi-dpi coordinate mapping.
Note it is expected that some implementations of this may be disposable, created on-the-fly for a single use. Code that receives a LayerGeometryProvider pointer as an argument to something should not, in general, store that pointer as it may be invalidated before the next use. Use getId() to instead obtain a persistent identifier for a LayerGeometryProvider, for example to establish whether the same one is being provided in two separate calls.
Definition at line 45 of file LayerGeometryProvider.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 67 of file LayerGeometryProvider.h.
References getCentreFrame(), getEndFrame(), getFrameForX(), getFrequencyForY(), getId(), getModelsEndFrame(), getModelsStartFrame(), getPaintRect(), getStartFrame(), getTextLabelYCoord(), getViewXForX(), getVisibleExtentsForUnit(), getXForFrame(), getXForViewX(), getYForFrequency(), and getZoomLevel().
Member Function Documentation
|
inlinestaticprotected |
Definition at line 48 of file LayerGeometryProvider.h.
|
pure virtual |
Retrieve the id of this object.
Implemented in View, and ViewProxy.
Referenced by Colour3DPlotLayer::getRenderer(), SpectrogramLayer::getRenderer(), SliceLayer::getScalePointForX(), SliceLayer::getValueForY(), SliceLayer::getXForScalePoint(), SliceLayer::getYForValue(), LayerGeometryProvider(), SliceLayer::paint(), TimeValueLayer::paint(), SpectrumLayer::paintCrosshairs(), SpectrogramLayer::paintDetailedScale(), Colour3DPlotLayer::paintVerticalScale(), Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
|
pure virtual |
Retrieve the first visible sample frame on the widget.
This is a calculated value based on the centre-frame, widget width and zoom level. The result may be negative.
Implemented in View, and ViewProxy.
Referenced by Colour3DPlotRenderer::geometryChanged(), TimeRulerLayer::getMajorTickUSec(), WaveformLayer::getNormalizeGain(), LayerGeometryProvider(), Layer::paintMeasurementRect(), Colour3DPlotRenderer::render(), TimeRulerLayer::snapToFeatureFrame(), Colour3DPlotExporter::toStringExportRows(), and Layer::updateMeasurePixrects().
|
pure virtual |
Return the centre frame of the visible widget.
This is an exact value that does not depend on the zoom block size. Other frame values (start, end) are calculated from this based on the zoom and other factors.
Implemented in View, and ViewProxy.
Referenced by LayerGeometryProvider(), SliceLayer::paint(), and SpectrumLayer::paint().
|
pure virtual |
Retrieve the last visible sample frame on the widget.
This is a calculated value based on the centre-frame, widget width and zoom level.
Implemented in View, and ViewProxy.
Referenced by TimeInstantLayer::getLocalPoints(), TimeValueLayer::getLocalPoints(), TimeRulerLayer::getMajorTickUSec(), WaveformLayer::getNormalizeGain(), LayerGeometryProvider(), Layer::paintMeasurementRect(), TimeRulerLayer::snapToFeatureFrame(), and Layer::updateMeasurePixrects().
|
pure virtual |
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative).
Implemented in View, and ViewProxy.
Referenced by FlexiNoteLayer::editStart(), BoxLayer::editStart(), RegionLayer::editStart(), NoteLayer::editStart(), TextLayer::getFeatureDescription(), TimeInstantLayer::getFeatureDescription(), FlexiNoteLayer::getFeatureDescription(), BoxLayer::getFeatureDescription(), TimeValueLayer::getFeatureDescription(), RegionLayer::getFeatureDescription(), NoteLayer::getFeatureDescription(), TextLayer::getLocalPoints(), ImageLayer::getLocalPoints(), TimeInstantLayer::getLocalPoints(), TimeValueLayer::getLocalPoints(), TextLayer::getPointToDrag(), FlexiNoteLayer::getRelativeMousePosition(), TimeRulerLayer::getXForUSec(), SpectrogramLayer::illuminateLocalFeatures(), LayerGeometryProvider(), TextLayer::paint(), TimeInstantLayer::paint(), FlexiNoteLayer::paint(), BoxLayer::paint(), SliceLayer::paint(), TimeValueLayer::paint(), ImageLayer::paint(), RegionLayer::paint(), NoteLayer::paint(), WaveformLayer::paintChannel(), Layer::paintMeasurementRect(), Colour3DPlotRenderer::render(), Colour3DPlotRenderer::renderDirectTranslucent(), Colour3DPlotRenderer::renderToCacheBinResolution(), ScrollableMagRangeCache::scrollTo(), ScrollableImageCache::scrollTo(), TextLayer::snapToFeatureFrame(), TimeInstantLayer::snapToFeatureFrame(), TimeRulerLayer::snapToFeatureFrame(), ImageLayer::snapToFeatureFrame(), FlexiNoteLayer::snapToFeatureFrame(), BoxLayer::snapToFeatureFrame(), TimeValueLayer::snapToFeatureFrame(), RegionLayer::snapToFeatureFrame(), NoteLayer::snapToFeatureFrame(), and Layer::updateMeasurePixrects().
|
pure virtual |
Return the closest frame to the given pixel x-coordinate.
Implemented in View, and ViewProxy.
Referenced by FlexiNoteLayer::addNote(), TextLayer::drawDrag(), TimeInstantLayer::drawDrag(), ImageLayer::drawDrag(), FlexiNoteLayer::drawDrag(), BoxLayer::drawDrag(), TimeValueLayer::drawDrag(), RegionLayer::drawDrag(), NoteLayer::drawDrag(), TextLayer::drawStart(), TimeInstantLayer::drawStart(), ImageLayer::drawStart(), FlexiNoteLayer::drawStart(), BoxLayer::drawStart(), TimeValueLayer::drawStart(), RegionLayer::drawStart(), NoteLayer::drawStart(), TextLayer::editDrag(), ImageLayer::editDrag(), TimeInstantLayer::editDrag(), FlexiNoteLayer::editDrag(), BoxLayer::editDrag(), TimeValueLayer::editDrag(), RegionLayer::editDrag(), NoteLayer::editDrag(), Colour3DPlotLayer::getFeatureDescription(), BoxLayer::getLocalPoint(), TextLayer::getLocalPoints(), TimeInstantLayer::getLocalPoints(), RegionLayer::getLocalPoints(), NoteLayer::getLocalPoints(), FlexiNoteLayer::getLocalPoints(), TimeValueLayer::getLocalPoints(), FlexiNoteLayer::getNoteToEdit(), TextLayer::getPointToDrag(), RegionLayer::getPointToDrag(), NoteLayer::getPointToDrag(), FlexiNoteLayer::getPointToDrag(), WaveformLayer::getSourceFramesForX(), SpectrogramLayer::getXBinRange(), Layer::getXScaleValue(), LayerGeometryProvider(), Layer::measureDrag(), Layer::measureStart(), TimeRulerLayer::paint(), TextLayer::paint(), TimeInstantLayer::paint(), SliceLayer::paint(), FlexiNoteLayer::paint(), BoxLayer::paint(), TimeValueLayer::paint(), ImageLayer::paint(), RegionLayer::paint(), NoteLayer::paint(), WaveformLayer::paintChannel(), SpectrogramLayer::paintCrosshairs(), Colour3DPlotRenderer::renderDirectTranslucent(), Colour3DPlotRenderer::renderToCacheBinResolution(), Colour3DPlotRenderer::renderToCachePixelResolution(), Layer::setMeasureRectFromPixrect(), and FlexiNoteLayer::splitEnd().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by LayerGeometryProvider().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by LayerGeometryProvider(), and TimeValueLayer::paint().
|
pure virtual |
Return the closest pixel x-coordinate corresponding to a given view x-coordinate.
Implemented in View, and ViewProxy.
Referenced by TimeRulerLayer::getMajorTickUSec(), LayerGeometryProvider(), TimeRulerLayer::paint(), SpectrumLayer::paint(), and Colour3DPlotRenderer::render().
|
pure virtual |
Return the closest view x-coordinate corresponding to a given pixel x-coordinate.
Implemented in View, and ViewProxy.
Referenced by LayerGeometryProvider().
|
pure virtual |
Return the (maybe fractional) pixel y-coordinate corresponding to a given frequency, if the frequency range is as specified.
This does not imply any policy about layer frequency ranges, but it might be useful for layers to match theirs up if desired.
Not thread-safe in logarithmic mode. Call only from GUI thread.
Implemented in View, and ViewProxy.
Referenced by SpectrogramLayer::getYForBin(), SpectrogramLayer::getYForFrequency(), LayerGeometryProvider(), PianoScale::paintPianoVertical(), and Colour3DPlotRenderer::renderDrawBufferPeakFrequencies().
|
pure virtual |
Return the closest frequency to the given (maybe fractional) pixel y-coordinate, if the frequency range is as specified.
Not thread-safe in logarithmic mode. Call only from GUI thread.
Implemented in View, and ViewProxy.
Referenced by SpectrogramLayer::getBinForY(), SpectrogramLayer::getFrequencyForY(), and LayerGeometryProvider().
|
pure virtual |
Return a y-coordinate at which text labels for individual items in a layer may be drawn, so as not to overlap with those of other layers.
The returned coordinate will be near the top of the visible widget, but adjusted downward depending on how many other visible layers return true from their implementation of Layer::needsTextLabelHeight().
Implemented in View, and ViewProxy.
Referenced by LayerGeometryProvider(), TimeInstantLayer::paint(), TimeValueLayer::paint(), and RegionLayer::paint().
|
pure virtual |
Return the visible vertical extents for the given unit, if any.
That is:
- if at least one non-dormant layer uses the same unit and returns some values from its getDisplayExtents() method, return the extents from the topmost of those
- otherwise, if at least one non-dormant layer uses the same unit, return the union of the value extents of all of those
- otherwise return false
Implemented in View, and ViewProxy.
Referenced by BoxLayer::getScaleExtents(), RegionLayer::getScaleExtents(), NoteLayer::getScaleExtents(), FlexiNoteLayer::getScaleExtents(), TimeValueLayer::getScaleExtents(), LayerGeometryProvider(), and Layer::valueExtentsMatchMine().
|
pure virtual |
Return the zoom level, i.e.
the number of frames per pixel or pixels per frame
Implemented in View, and ViewProxy.
Referenced by Colour3DPlotRenderer::decideRenderType(), Colour3DPlotRenderer::geometryChanged(), WaveformLayer::getFeatureDescription(), TimeRulerLayer::getMajorTickUSec(), Colour3DPlotRenderer::getPreferredPeakCache(), WaveformLayer::getSourceFramesForX(), TimeRulerLayer::getXForUSec(), LayerGeometryProvider(), TimeRulerLayer::paint(), WaveformLayer::paint(), Colour3DPlotLayer::paint(), SpectrogramLayer::paint(), WaveformLayer::paintChannel(), and Colour3DPlotRenderer::render().
|
pure virtual |
To be called from a layer, to obtain the extent of the surface that the layer is currently painting to.
This may be the extent of the view (if 1x display scaling is in effect) or of a larger cached pixmap (if greater display scaling is in effect).
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), getPaintSize(), getPaintWidth(), LayerGeometryProvider(), WaveformLayer::paint(), Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
|
inlinevirtual |
Reimplemented in View, and ViewProxy.
Definition at line 186 of file LayerGeometryProvider.h.
References getPaintRect().
Referenced by Colour3DPlotRenderer::geometryChanged(), and Colour3DPlotRenderer::render().
|
inlinevirtual |
Reimplemented in View, and ViewProxy.
Definition at line 187 of file LayerGeometryProvider.h.
References getPaintRect().
Referenced by SpectrumLayer::getCrosshairExtents(), TextLayer::getLocalPoints(), TimeRulerLayer::getMajorTickUSec(), SliceLayer::getScalePointForX(), SliceLayer::getXForScalePoint(), TimeInstantLayer::paint(), BoxLayer::paint(), TimeValueLayer::paint(), WaveformLayer::paint(), ImageLayer::paint(), RegionLayer::paint(), Colour3DPlotLayer::paint(), SpectrumLayer::paintCrosshairs(), SpectrumLayer::paintHorizontalScale(), Layer::paintMeasurementRect(), and Colour3DPlotRenderer::render().
|
inlinevirtual |
Reimplemented in View, and ViewProxy.
Definition at line 188 of file LayerGeometryProvider.h.
References drawMeasurementRect(), getBackground(), getForeground(), getPaintRect(), getView(), getViewManager(), hasLightBackground(), scalePen(), scalePenWidth(), scalePixelSize(), scaleSize(), shouldIlluminateLocalFeatures(), shouldShowFeatureLabels(), and updatePaintRect().
Referenced by Colour3DPlotRenderer::decideRenderType(), ImageLayer::drawImage(), Colour3DPlotLayer::getBinForY(), SpectrumLayer::getCrosshairExtents(), SpectrogramLayer::getCrosshairExtents(), TextLayer::getHeightForY(), TextLayer::getLocalPoints(), BoxLayer::getValueForY(), RegionLayer::getValueForY(), NoteLayer::getValueForY(), FlexiNoteLayer::getValueForY(), TimeValueLayer::getValueForY(), WaveformLayer::getValueForY(), SpectrogramLayer::getYBinRange(), Colour3DPlotLayer::getYForBin(), TextLayer::getYForHeight(), BoxLayer::getYForValue(), RegionLayer::getYForValue(), NoteLayer::getYForValue(), FlexiNoteLayer::getYForValue(), TimeValueLayer::getYForValue(), WaveformLayer::getYForValue(), TextLayer::paint(), TimeRulerLayer::paint(), TimeInstantLayer::paint(), FlexiNoteLayer::paint(), SliceLayer::paint(), TimeValueLayer::paint(), WaveformLayer::paint(), ImageLayer::paint(), RegionLayer::paint(), SpectrumLayer::paint(), WaveformLayer::paintChannel(), SpectrumLayer::paintCrosshairs(), SpectrogramLayer::paintCrosshairs(), SpectrumLayer::paintHorizontalScale(), LinearColourScale::paintVertical(), LogNumericalScale::paintVertical(), LogColourScale::paintVertical(), LinearNumericalScale::paintVertical(), FlexiNoteLayer::paintVerticalScale(), TimeValueLayer::paintVerticalScale(), SliceLayer::paintVerticalScale(), NoteLayer::paintVerticalScale(), SpectrogramLayer::paintVerticalScale(), Colour3DPlotRenderer::renderDirectTranslucent(), Colour3DPlotRenderer::renderToCacheBinResolution(), Colour3DPlotRenderer::renderToCachePixelResolution(), Layer::setMeasureRectYCoord(), RegionLayer::spacingIndexToY(), Layer::updateMeasureRectYCoords(), and RegionLayer::yToSpacingIndex().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), WaveformLayer::paintChannel(), WaveformLayer::paintChannelScaleGuides(), and SingleColourLayer::setDefaultColourFor().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by PaintAssistant::drawVisibleText(), SingleColourLayer::getForegroundQColor(), getPaintHeight(), SpectrogramLayer::illuminateLocalFeatures(), TextLayer::paint(), FlexiNoteLayer::paint(), ImageLayer::paint(), RegionLayer::paint(), NoteLayer::paint(), SpectrogramLayer::paintDetailedScale(), Colour3DPlotLayer::paintVerticalScale(), and Colour3DPlotRenderer::renderDirectTranslucent().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by PaintAssistant::drawVisibleText(), SingleColourLayer::getBackgroundQColor(), getPaintHeight(), TextLayer::paint(), SliceLayer::paint(), and ImageLayer::paint().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by Colour3DPlotRenderer::decideRenderType(), Colour3DPlotLayer::getFeatureDescription(), getPaintHeight(), TimeRulerLayer::paint(), SliceLayer::paint(), WaveformLayer::paintChannelScaleGuides(), SpectrumLayer::paintHorizontalScale(), and Colour3DPlotRenderer::renderDirectTranslucent().
|
pure virtual |
Implemented in View, ViewProxy, and Pane.
Referenced by getPaintHeight(), SpectrogramLayer::illuminateLocalFeatures(), TextLayer::isLayerScrollable(), TimeInstantLayer::isLayerScrollable(), BoxLayer::isLayerScrollable(), NoteLayer::isLayerScrollable(), RegionLayer::isLayerScrollable(), FlexiNoteLayer::isLayerScrollable(), TimeValueLayer::isLayerScrollable(), TextLayer::paint(), TimeInstantLayer::paint(), BoxLayer::paint(), FlexiNoteLayer::paint(), TimeValueLayer::paint(), RegionLayer::paint(), NoteLayer::paint(), SpectrumLayer::paint(), and Colour3DPlotRenderer::renderDirectTranslucent().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), and TimeValueLayer::paint().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), and Layer::paintMeasurementRect().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by TimeInstantLayer::getLocalPoints(), TimeValueLayer::getLocalPoints(), getPaintHeight(), and WaveformLayer::paintChannel().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), BoxLayer::paint(), and RegionLayer::paint().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by getPaintHeight(), SliceLayer::paint(), and TimeValueLayer::paint().
|
pure virtual |
Implemented in View, and ViewProxy.
Referenced by Layer::alignFromReference(), Layer::alignToReference(), TextLayer::drawEnd(), TextLayer::editOpen(), FlexiNoteLayer::getAssociatedPitchModel(), getPaintHeight(), FlexiNoteLayer::mouseMoveEvent(), TimeRulerLayer::paint(), TimeInstantLayer::paint(), RegionLayer::paint(), TextLayer::paste(), ImageLayer::paste(), TimeInstantLayer::paste(), BoxLayer::paste(), TimeValueLayer::paste(), RegionLayer::paste(), FlexiNoteLayer::paste(), NoteLayer::paste(), and FlexiNoteLayer::setVerticalRangeToNoteRange().
|
pure virtual |
The documentation for this class was generated from the following file:
Generated by
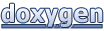