svgui
1.9
|
#include <Pane.h>
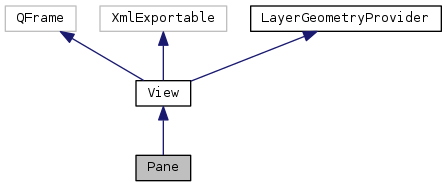
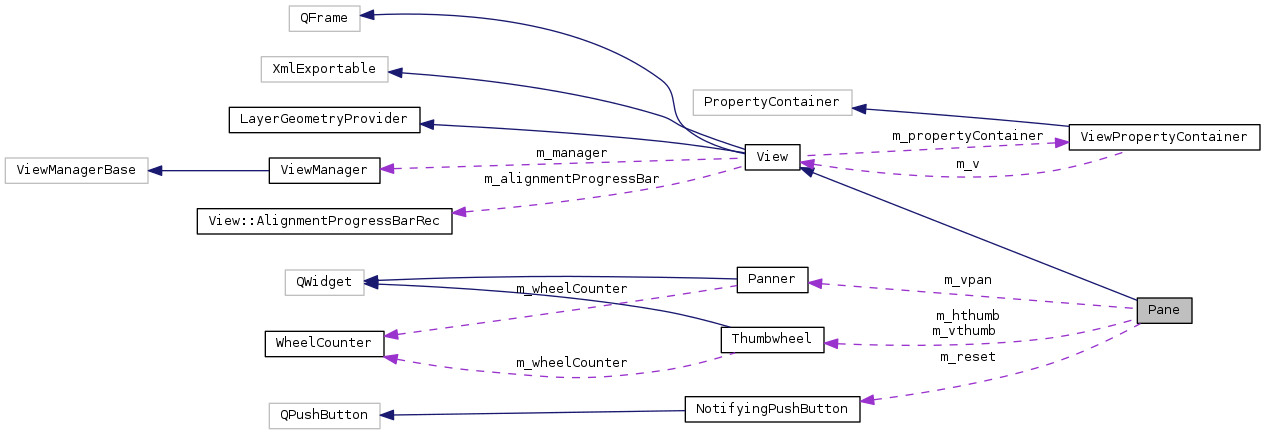
Public Types | |
enum | PaneType { Normal = 0, TonyMain = 1, TonySelection = 2 } |
typedef PropertyContainer::PropertyName | PropertyName |
typedef std::set< ModelId > | ModelSet |
Public Slots | |
virtual void | toolModeChanged () override |
virtual void | zoomWheelsEnabledChanged () override |
virtual void | viewZoomLevelChanged (View *, ZoomLevel, bool locked) override |
virtual void | modelAlignmentCompletionChanged (ModelId) override |
void | horizontalThumbwheelMoved (int value) |
void | verticalThumbwheelMoved (int value) |
void | verticalZoomChanged () |
void | verticalPannerMoved (float x, float y, float w, float h) |
void | verticalPannerContextMenuRequested (const QPoint &) |
void | editVerticalPannerExtents () |
void | resetVerticalPannerExtents () |
virtual void | layerParametersChanged () override |
virtual void | propertyContainerSelected (View *, PropertyContainer *pc) override |
void | zoomToRegion (QRect r) |
void | mouseEnteredWidget () |
void | mouseLeftWidget () |
virtual void | modelChanged (ModelId) |
virtual void | modelChangedWithin (ModelId, sv_frame_t startFrame, sv_frame_t endFrame) |
virtual void | modelCompletionChanged (ModelId) |
virtual void | modelReplaced () |
virtual void | layerParameterRangesChanged () |
virtual void | layerMeasurementRectsChanged () |
virtual void | layerNameChanged () |
virtual void | globalCentreFrameChanged (sv_frame_t) |
virtual void | viewCentreFrameChanged (View *, sv_frame_t) |
virtual void | viewManagerPlaybackFrameChanged (sv_frame_t) |
virtual void | selectionChanged () |
virtual void | overlayModeChanged () |
virtual void | cancelClicked () |
virtual void | progressCheckStalledTimerElapsed () |
Signals | |
void | paneInteractedWith () |
void | rightButtonMenuRequested (QPoint position) |
void | dropAccepted (QStringList uriList) |
void | dropAccepted (QString text) |
void | doubleClickSelectInvoked (sv_frame_t frame) |
void | regionOutlined (QRect rect) |
void | propertyContainerAdded (PropertyContainer *pc) |
void | propertyContainerRemoved (PropertyContainer *pc) |
void | propertyContainerPropertyChanged (PropertyContainer *pc) |
void | propertyContainerPropertyRangeChanged (PropertyContainer *pc) |
void | propertyContainerNameChanged (PropertyContainer *pc) |
void | propertyContainerSelected (PropertyContainer *pc) |
void | propertyChanged (PropertyContainer::PropertyName) |
void | layerModelChanged () |
void | cancelButtonPressed (Layer *) |
void | centreFrameChanged (sv_frame_t frame, bool globalScroll, PlaybackFollowMode followMode) |
void | zoomLevelChanged (ZoomLevel level, bool locked) |
void | contextHelpChanged (const QString &) |
Public Member Functions | |
Pane (QWidget *parent=0) | |
virtual | ~Pane () |
virtual QString | getPropertyContainerIconName () const override |
virtual bool | shouldIlluminateLocalFeatures (const Layer *layer, QPoint &pos) const override |
virtual bool | shouldIlluminateLocalSelection (QPoint &pos, bool &closeToLeft, bool &closeToRight) const override |
void | setCentreLineVisible (bool visible) |
bool | getCentreLineVisible () const |
virtual sv_frame_t | getFirstVisibleFrame () const override |
int | getVerticalScaleWidth () const |
virtual QImage * | renderToNewImage () override |
Render the view contents to a new QImage (which may be wider than the visible View). More... | |
virtual QImage * | renderPartToNewImage (sv_frame_t f0, sv_frame_t f1) override |
Render the view contents between the given frame extents to a new QImage (which may be wider than the visible View). More... | |
virtual QSize | getRenderedImageSize () override |
Calculate and return the size of image that will be generated by renderToNewImage(). More... | |
virtual QSize | getRenderedPartImageSize (sv_frame_t f0, sv_frame_t f1) override |
Calculate and return the size of image that will be generated by renderPartToNewImage(f0, f1). More... | |
virtual void | toXml (QTextStream &stream, QString indent="", QString extraAttributes="") const override |
int | getId () const override |
Retrieve the id of this object. More... | |
sv_frame_t | getStartFrame () const override |
Retrieve the first visible sample frame on the widget. More... | |
void | setStartFrame (sv_frame_t) |
Set the widget pan based on the given first visible frame. More... | |
sv_frame_t | getCentreFrame () const override |
Return the centre frame of the visible widget. More... | |
void | setCentreFrame (sv_frame_t f) |
Set the centre frame of the visible widget. More... | |
sv_frame_t | getEndFrame () const override |
Retrieve the last visible sample frame on the widget. More... | |
int | getXForFrame (sv_frame_t frame) const override |
Return the pixel x-coordinate corresponding to a given sample frame. More... | |
sv_frame_t | getFrameForX (int x) const override |
Return the closest frame to the given pixel x-coordinate. More... | |
int | getXForViewX (int viewx) const override |
Return the closest pixel x-coordinate corresponding to a given view x-coordinate. More... | |
int | getViewXForX (int x) const override |
Return the closest view x-coordinate corresponding to a given pixel x-coordinate. More... | |
double | getYForFrequency (double frequency, double minFreq, double maxFreq, bool logarithmic) const override |
Return the pixel y-coordinate corresponding to a given frequency, if the frequency range is as specified. More... | |
double | getFrequencyForY (double y, double minFreq, double maxFreq, bool logarithmic) const override |
Return the closest frequency to the given pixel y-coordinate, if the frequency range is as specified. More... | |
ZoomLevel | getZoomLevel () const override |
Return the zoom level, i.e. More... | |
virtual void | setZoomLevel (ZoomLevel z) |
Set the zoom level, i.e. More... | |
virtual void | zoom (bool in) |
Zoom in or out. More... | |
virtual void | scroll (bool right, bool lots, bool doEmit=true) |
Scroll left or right by a smallish or largish amount. More... | |
virtual void | addLayer (Layer *v) |
Add a layer to the view. More... | |
virtual void | removeLayer (Layer *v) |
Remove a layer from the view. More... | |
virtual int | getLayerCount () const |
Return the number of layers, regardless of whether visible or dormant, i.e. More... | |
virtual Layer * | getLayer (int n) |
Return the nth layer, counted in stacking order. More... | |
virtual Layer * | getFixedOrderLayer (int n) |
Return the nth layer, counted in the order they were added. More... | |
virtual Layer * | getInteractionLayer () |
Return the layer currently active for tool interaction. More... | |
virtual const Layer * | getInteractionLayer () const |
virtual Layer * | getSelectedLayer () |
Return the layer most recently selected by the user. More... | |
virtual const Layer * | getSelectedLayer () const |
virtual Layer * | getTopLayer () |
Return the "top" layer in the view, whether visible or dormant. More... | |
virtual void | setViewManager (ViewManager *m) |
virtual void | setViewManager (ViewManager *m, sv_frame_t initialFrame) |
ViewManager * | getViewManager () const override |
virtual void | setFollowGlobalPan (bool f) |
virtual bool | getFollowGlobalPan () const |
virtual void | setFollowGlobalZoom (bool f) |
virtual bool | getFollowGlobalZoom () const |
bool | hasLightBackground () const override |
QColor | getForeground () const override |
QColor | getBackground () const override |
void | drawMeasurementRect (QPainter &p, const Layer *, QRect rect, bool focus) const override |
bool | shouldShowFeatureLabels () const override |
virtual void | setPlaybackFollow (PlaybackFollowMode m) |
virtual PlaybackFollowMode | getPlaybackFollow () const |
virtual PropertyContainer::PropertyList | getProperties () const |
virtual QString | getPropertyLabel (const PropertyName &) const |
virtual PropertyContainer::PropertyType | getPropertyType (const PropertyName &) const |
virtual int | getPropertyRangeAndValue (const PropertyName &, int *min, int *max, int *deflt) const |
virtual QString | getPropertyValueLabel (const PropertyName &, int value) const |
virtual void | setProperty (const PropertyName &, int value) |
virtual QString | getPropertyContainerName () const |
virtual int | getPropertyContainerCount () const |
virtual const PropertyContainer * | getPropertyContainer (int i) const |
virtual PropertyContainer * | getPropertyContainer (int i) |
virtual bool | renderToSvgFile (QString filename) |
Render the view contents to a new SVG file. More... | |
virtual bool | renderPartToSvgFile (QString filename, sv_frame_t f0, sv_frame_t f1) |
Render the view contents between the given frame extents to a new SVG file. More... | |
bool | getVisibleExtentsForUnit (QString unit, double &min, double &max, bool &log) const override |
Return the visible vertical extents for the given unit, if any. More... | |
bool | getVisibleExtentsForAnyUnit (double &min, double &max, bool &logarithmic, QString &unit) const |
Return some visible vertical extents and unit. More... | |
int | getTextLabelYCoord (const Layer *layer, QPainter &) const override |
Return a y-coordinate at which text labels for individual items in a layer may be drawn, so as not to overlap with those of other layers. More... | |
virtual sv_frame_t | getLastVisibleFrame () const |
sv_frame_t | getModelsStartFrame () const override |
sv_frame_t | getModelsEndFrame () const override |
QRect | getPaintRect () const override |
To be called from a layer, to obtain the extent of the surface that the layer is currently painting to. More... | |
QSize | getPaintSize () const override |
int | getPaintWidth () const override |
int | getPaintHeight () const override |
double | scaleSize (double size) const override |
int | scalePixelSize (int size) const override |
double | scalePenWidth (double width) const override |
QPen | scalePen (QPen pen) const override |
ModelSet | getModels () |
void | setUseAligningProxy (bool uap) |
!!??? poor name, probably poor api, consider this More... | |
ModelId | getAligningModel () const |
!! More... | |
void | getAligningAndReferenceModels (ModelId &aligning, ModelId &reference) const |
sv_frame_t | alignFromReference (sv_frame_t) const |
sv_frame_t | alignToReference (sv_frame_t) const |
sv_frame_t | getAlignedPlaybackFrame () const |
void | updatePaintRect (QRect r) override |
View * | getView () override |
const View * | getView () const override |
Static Public Member Functions | |
static void | registerShortcuts (KeyReference &kr) |
Protected Types | |
enum | DragMode { UnresolvedDrag, VerticalDrag, HorizontalDrag, FreeDrag } |
typedef std::vector< Layer * > | LayerList |
typedef std::map< Layer *, ProgressBarRec > | ProgressMap |
Protected Slots | |
void | playbackScheduleTimerElapsed () |
Protected Member Functions | |
virtual void | paintEvent (QPaintEvent *e) override |
virtual void | mousePressEvent (QMouseEvent *e) override |
virtual void | mouseReleaseEvent (QMouseEvent *e) override |
virtual void | mouseMoveEvent (QMouseEvent *e) override |
virtual void | mouseDoubleClickEvent (QMouseEvent *e) override |
virtual void | enterEvent (QEvent *e) override |
virtual void | leaveEvent (QEvent *e) override |
virtual void | wheelEvent (QWheelEvent *e) override |
virtual void | resizeEvent (QResizeEvent *e) override |
virtual void | dragEnterEvent (QDragEnterEvent *e) override |
virtual void | dropEvent (QDropEvent *e) override |
void | wheelVertical (int sign, Qt::KeyboardModifiers) |
void | wheelHorizontal (int sign, Qt::KeyboardModifiers) |
void | wheelHorizontalFine (int pixels, Qt::KeyboardModifiers) |
void | drawVerticalScale (QRect r, Layer *, QPainter &) |
void | drawFeatureDescription (Layer *, QPainter &) |
void | drawCentreLine (sv_samplerate_t, QPainter &, bool omitLine) |
void | drawModelTimeExtents (QRect, QPainter &, ModelId) |
void | drawDurationAndRate (QRect, ModelId, sv_samplerate_t, QPainter &) |
void | drawWorkTitle (QRect, QPainter &, ModelId) |
void | drawLayerNames (QRect, QPainter &) |
void | drawEditingSelection (QPainter &) |
void | drawAlignmentStatus (QRect, QPainter &, ModelId, bool down) |
virtual bool | render (QPainter &paint, int x0, sv_frame_t f0, sv_frame_t f1) override |
Selection | getSelectionAt (int x, bool &closeToLeft, bool &closeToRight) const |
bool | editSelectionStart (QMouseEvent *e) |
bool | editSelectionDrag (QMouseEvent *e) |
bool | editSelectionEnd (QMouseEvent *e) |
bool | selectionIsBeingEdited () const |
void | updateHeadsUpDisplay () |
void | updateVerticalPanner () |
bool | canTopLayerMoveVertical () |
bool | setTopLayerDisplayExtents (double displayMin, double displayMax) |
bool | getTopLayerDisplayExtents (double &valueMin, double &valueMax, double &displayMin, double &displayMax, QString *unit=0) |
void | dragTopLayer (QMouseEvent *e) |
void | dragExtendSelection (QMouseEvent *e) |
void | updateContextHelp (const QPoint *pos) |
void | edgeScrollMaybe (int x) |
Layer * | getTopFlexiNoteLayer () |
void | schedulePlaybackFrameMove (sv_frame_t frame) |
DragMode | updateDragMode (DragMode currentMode, QPoint origin, QPoint currentPoint, bool canMoveHorizontal, bool canMoveVertical, bool resistHorizontal, bool resistVertical) |
bool | setCentreFrame (sv_frame_t f, bool doEmit) |
virtual void | drawSelections (QPainter &) |
virtual bool | shouldLabelSelections () const |
virtual void | drawPlayPointer (QPainter &) |
virtual void | setPaintFont (QPainter &paint) |
QSize | scaledSize (const QSize &s, int factor) |
QRect | scaledRect (const QRect &r, int factor) |
sv_samplerate_t | getModelsSampleRate () const |
bool | areLayersScrollable () const |
LayerList | getScrollableBackLayers (bool testChanged, bool &changed) const |
LayerList | getNonScrollableFrontLayers (bool testChanged, bool &changed) const |
Layer * | getScaleProvidingLayerForUnit (QString unit) const |
ZoomLevel | getZoomConstraintLevel (ZoomLevel level, ZoomConstraint::RoundingDirection dir=ZoomConstraint::RoundNearest) const |
int | countZoomLevels () const |
int | getZoomLevelIndex (ZoomLevel level) const |
ZoomLevel | getZoomLevelByIndex (int ix) const |
bool | areLayerColoursSignificant () const |
bool | hasTopLayerTimeXAxis () const |
void | movePlayPointer (sv_frame_t f) |
void | checkProgress (ModelId) |
void | checkAlignmentProgress (ModelId) |
bool | waitForLayersToBeReady () |
int | getProgressBarWidth () const |
int | effectiveDevicePixelRatio () const |
Static Protected Member Functions | |
static int | getNextId () |
Static Protected Attributes | |
static QCursor * | m_measureCursor1 = nullptr |
!! ugh More... | |
static QCursor * | m_measureCursor2 = nullptr |
Detailed Description
Member Typedef Documentation
|
inherited |
|
inherited |
|
protectedinherited |
|
protectedinherited |
Member Enumeration Documentation
enum Pane::PaneType |
|
protected |
Constructor & Destructor Documentation
Pane::Pane | ( | QWidget * | parent = 0 | ) |
Definition at line 73 of file Pane.cpp.
References regionOutlined(), updateHeadsUpDisplay(), and zoomToRegion().
|
virtual |
Definition at line 107 of file Pane.cpp.
References m_lastVerticalPannerContextMenu.
Member Function Documentation
|
inlineoverridevirtual |
Implements View.
Definition at line 43 of file Pane.h.
References setCentreLineVisible(), shouldIlluminateLocalFeatures(), and shouldIlluminateLocalSelection().
|
overridevirtual |
Implements LayerGeometryProvider.
Definition at line 291 of file Pane.cpp.
References View::getInteractionLayer(), ViewManager::getToolModeFor(), m_identifyFeatures, m_identifyPoint, View::m_manager, ViewManager::MeasureMode, ViewManager::shouldIlluminateLocalFeatures(), and shouldIlluminateLocalSelection().
Referenced by getPropertyContainerIconName().
|
overridevirtual |
Reimplemented from View.
Definition at line 315 of file Pane.cpp.
References ViewManager::EditMode, View::getInteractionLayer(), getSelectionAt(), ViewManager::getSelections(), ViewManager::getToolModeFor(), Layer::isLayerEditable(), m_identifyFeatures, m_identifyPoint, View::m_manager, and selectionIsBeingEdited().
Referenced by getPropertyContainerIconName(), and shouldIlluminateLocalFeatures().
void Pane::setCentreLineVisible | ( | bool | visible | ) |
Definition at line 353 of file Pane.cpp.
References m_centreLineVisible.
Referenced by getPropertyContainerIconName().
|
inline |
Definition at line 52 of file Pane.h.
References getFirstVisibleFrame(), getVerticalScaleWidth(), and m_centreLineVisible.
|
overridevirtual |
Reimplemented from View.
Definition at line 1258 of file Pane.cpp.
References View::getFirstVisibleFrame(), View::getFrameForX(), and m_scaleWidth.
Referenced by getCentreLineVisible().
int Pane::getVerticalScaleWidth | ( | ) | const |
Definition at line 532 of file Pane.cpp.
References m_scaleWidth.
Referenced by getCentreLineVisible().
|
inlineoverridevirtual |
Render the view contents to a new QImage (which may be wider than the visible View).
Reimplemented from View.
Definition at line 58 of file Pane.h.
References renderPartToNewImage(), and View::renderToNewImage().
|
overridevirtual |
Render the view contents between the given frame extents to a new QImage (which may be wider than the visible View).
Reimplemented from View.
Definition at line 1200 of file Pane.cpp.
References View::getTopLayer(), Layer::getVerticalScaleWidth(), View::getZoomLevel(), View::m_manager, m_scaleWidth, render(), ViewManager::shouldShowVerticalColourScale(), and ViewManager::shouldShowVerticalScale().
Referenced by renderToNewImage().
|
inlineoverridevirtual |
Calculate and return the size of image that will be generated by renderToNewImage().
Reimplemented from View.
Definition at line 64 of file Pane.h.
References View::getRenderedImageSize(), getRenderedPartImageSize(), registerShortcuts(), and toXml().
|
overridevirtual |
Calculate and return the size of image that will be generated by renderPartToNewImage(f0, f1).
Reimplemented from View.
Definition at line 1239 of file Pane.cpp.
References View::getRenderedPartImageSize(), View::getTopLayer(), Layer::getVerticalScaleWidth(), View::m_manager, ViewManager::shouldShowVerticalColourScale(), and ViewManager::shouldShowVerticalScale().
Referenced by getRenderedImageSize().
|
overridevirtual |
Definition at line 2988 of file Pane.cpp.
References m_centreLineVisible, and View::toXml().
Referenced by getRenderedImageSize().
|
static |
Definition at line 1334 of file Pane.cpp.
References KeyReference::registerAlternativeShortcut(), KeyReference::registerShortcut(), and KeyReference::setCategory().
Referenced by getRenderedImageSize().
|
signal |
Referenced by mousePressEvent(), mouseReleaseEvent(), wheelHorizontalFine(), and wheelVertical().
|
signal |
Referenced by mousePressEvent().
|
signal |
Referenced by dropEvent().
|
signal |
|
signal |
Referenced by mouseDoubleClickEvent().
|
signal |
Referenced by mouseReleaseEvent(), and Pane().
|
overridevirtualslot |
Definition at line 2751 of file Pane.cpp.
References ViewManager::DrawMode, ViewManager::EditMode, ViewManager::EraseMode, ViewManager::getToolModeFor(), View::m_manager, m_measureCursor1, m_measureCursor2, ViewManager::MeasureMode, ViewManager::NavigateMode, ViewManager::NoteEditMode, and ViewManager::SelectMode.
Referenced by mouseReleaseEvent().
|
overridevirtualslot |
Definition at line 2805 of file Pane.cpp.
References updateHeadsUpDisplay().
|
overridevirtualslot |
Definition at line 2812 of file Pane.cpp.
References ViewManager::getZoomWheelsEnabled(), View::m_followZoom, m_hthumb, View::m_manager, updateHeadsUpDisplay(), and View::viewZoomLevelChanged().
|
overridevirtualslot |
Definition at line 933 of file Pane.cpp.
References View::modelAlignmentCompletionChanged().
|
slot |
Definition at line 2515 of file Pane.cpp.
References Thumbwheel::getMaximumValue(), View::getZoomLevelByIndex(), m_hthumb, and View::setZoomLevel().
Referenced by updateHeadsUpDisplay().
|
slot |
Definition at line 2522 of file Pane.cpp.
References View::getLayer(), View::getLayerCount(), Layer::getVerticalZoomSteps(), Layer::setVerticalZoomStep(), updateHeadsUpDisplay(), and updateVerticalPanner().
Referenced by updateHeadsUpDisplay().
|
slot |
Definition at line 2858 of file Pane.cpp.
References Layer::getCurrentVerticalZoomStep(), View::getLayer(), View::getLayerCount(), m_vthumb, and Thumbwheel::setValue().
Referenced by propertyContainerSelected().
|
slot |
Definition at line 2542 of file Pane.cpp.
References getTopLayerDisplayExtents(), and setTopLayerDisplayExtents().
Referenced by updateHeadsUpDisplay().
|
slot |
Definition at line 2555 of file Pane.cpp.
References MenuTitle::addTitle(), editVerticalPannerExtents(), getTopLayerDisplayExtents(), m_lastVerticalPannerContextMenu, m_vpan, and resetVerticalPannerExtents().
Referenced by updateHeadsUpDisplay().
|
slot |
Definition at line 2578 of file Pane.cpp.
References RangeInputDialog::getRange(), getTopLayerDisplayExtents(), ViewManager::getZoomWheelsEnabled(), View::m_manager, m_vpan, RangeInputDialog::setRange(), setTopLayerDisplayExtents(), and updateVerticalPanner().
Referenced by updateHeadsUpDisplay(), and verticalPannerContextMenuRequested().
|
slot |
Definition at line 2604 of file Pane.cpp.
References m_vpan, m_vthumb, Thumbwheel::resetToDefault(), and Panner::resetToDefault().
Referenced by verticalPannerContextMenuRequested().
|
overridevirtualslot |
Definition at line 2614 of file Pane.cpp.
References View::layerParametersChanged(), and updateHeadsUpDisplay().
|
overridevirtualslot |
Definition at line 2831 of file Pane.cpp.
References View::getLayer(), View::getLayerCount(), Layer::getNewVerticalZoomRangeMapper(), m_vthumb, View::propertyContainerSelected(), Thumbwheel::setRangeMapper(), updateHeadsUpDisplay(), and verticalZoomChanged().
|
slot |
Definition at line 1933 of file Pane.cpp.
References View::getEndFrame(), View::getFrameForX(), View::getInteractionLayer(), View::getModelsEndFrame(), View::getStartFrame(), View::getZoomConstraintLevel(), Layer::hasTimeXAxis(), View::m_layerStack, Layer::setDisplayExtents(), View::setStartFrame(), View::setZoomLevel(), updateVerticalPanner(), and Layer::zoomToRegion().
Referenced by Pane().
|
slot |
Definition at line 2965 of file Pane.cpp.
References View::contextHelpChanged(), m_hthumb, m_reset, m_vpan, and m_vthumb.
Referenced by updateHeadsUpDisplay().
|
slot |
Definition at line 2982 of file Pane.cpp.
References View::contextHelpChanged().
Referenced by updateHeadsUpDisplay().
|
protectedslot |
Definition at line 1528 of file Pane.cpp.
References View::m_manager, m_playbackFrameMoveScheduled, m_playbackFrameMoveTo, and ViewManager::setPlaybackFrame().
Referenced by schedulePlaybackFrameMove().
|
overrideprotectedvirtual |
!! be nice if this looked a bit more in keeping with the
!! shouldn't use clickPos – needs to use a clicked frame
Definition at line 360 of file Pane.cpp.
References drawAlignmentStatus(), drawCentreLine(), drawDurationAndRate(), drawEditingSelection(), drawFeatureDescription(), drawLayerNames(), drawModelTimeExtents(), drawVerticalScale(), drawWorkTitle(), ViewManager::getAlignMode(), View::getModelsSampleRate(), ViewManager::getToolModeFor(), View::getTopLayer(), View::hasTopLayerTimeXAxis(), ViewManager::isPlaying(), m_centreLineVisible, m_clickedInRange, m_clickPos, m_identifyFeatures, m_identifyPoint, View::m_layerStack, View::m_manager, m_mouseInWidget, m_mousePos, m_navigating, m_scaleWidth, m_shiftPressed, ViewManager::MeasureMode, ViewManager::NavigateMode, View::paintEvent(), Layer::paintMeasurementRects(), selectionIsBeingEdited(), View::setPaintFont(), ViewManager::shouldIlluminateLocalFeatures(), ViewManager::shouldShowCentreLine(), ViewManager::shouldShowDuration(), ViewManager::shouldShowLayerNames(), ViewManager::shouldShowVerticalScale(), and ViewManager::shouldShowWorkTitle().
|
overrideprotectedvirtual |
Definition at line 1371 of file Pane.cpp.
References View::alignToReference(), View::contextHelpChanged(), ViewManager::DrawMode, Layer::drawStart(), ViewManager::EditMode, ViewManager::EraseMode, Layer::eraseStart(), View::getFrameForX(), View::getInteractionLayer(), getSelectionAt(), ViewManager::getToolModeFor(), getTopFlexiNoteLayer(), View::getTopLayer(), getTopLayerDisplayExtents(), View::hasTopLayerTimeXAxis(), Layer::isLayerEditable(), m_altPressed, View::m_centreFrame, m_clickedInRange, m_clickPos, m_ctrlPressed, m_dragCentreFrame, m_dragMode, m_dragStartMinValue, m_editing, m_editingSelection, m_editingSelectionEdge, View::m_followPlay, View::m_manager, m_mousePos, m_navigating, m_releasing, m_resizing, m_selectionStartFrame, m_shiftPressed, ViewManager::MeasureMode, Layer::measureStart(), ViewManager::NavigateMode, ViewManager::NoteEditMode, paneInteractedWith(), PlaybackScrollPage, ViewManager::removeSelection(), rightButtonMenuRequested(), schedulePlaybackFrameMove(), ViewManager::SelectMode, ViewManager::setInProgressSelection(), Layer::SnapLeft, Layer::snapToFeatureFrame(), Layer::splitStart(), and UnresolvedDrag.
|
overrideprotectedvirtual |
Definition at line 1537 of file Pane.cpp.
References ViewManager::addSelection(), ViewManager::clearInProgressSelection(), Layer::drawEnd(), ViewManager::DrawMode, Layer::editEnd(), ViewManager::EditMode, editSelectionEnd(), Layer::eraseEnd(), ViewManager::EraseMode, View::getFrameForX(), View::getId(), ViewManager::getInProgressSelection(), View::getInteractionLayer(), ViewManager::getToolModeFor(), getTopFlexiNoteLayer(), View::getTopLayer(), View::hasTopLayerTimeXAxis(), ViewManager::haveInProgressSelection(), Layer::isLayerEditable(), m_clickedInRange, m_clickPos, m_editing, View::m_manager, m_measureCursor1, m_mousePos, m_navigating, m_releasing, m_shiftPressed, Layer::measureEnd(), ViewManager::MeasureMode, mouseMoveEvent(), ViewManager::NavigateMode, ViewManager::NoteEditMode, paneInteractedWith(), regionOutlined(), ViewManager::SelectMode, ViewManager::setSelection(), Layer::splitEnd(), and toolModeChanged().
|
overrideprotectedvirtual |
!! ew
Definition at line 1667 of file Pane.cpp.
References dragExtendSelection(), dragTopLayer(), Layer::drawDrag(), ViewManager::DrawMode, edgeScrollMaybe(), Layer::editDrag(), ViewManager::EditMode, editSelectionDrag(), editSelectionStart(), Layer::editStart(), Layer::eraseDrag(), ViewManager::EraseMode, View::getInteractionLayer(), getSelectionAt(), ViewManager::getToolModeFor(), getTopFlexiNoteLayer(), View::getTopLayer(), Layer::hasTimeXAxis(), View::hasTopLayerTimeXAxis(), HorizontalDrag, Layer::isLayerEditable(), ViewManager::isPlaying(), m_clickedInRange, m_clickPos, m_dragMode, m_editing, m_identifyFeatures, m_identifyPoint, View::m_manager, m_measureCursor2, m_mousePos, m_navigating, m_playbackFrameMoveScheduled, m_releasing, m_shiftPressed, Layer::measureDrag(), ViewManager::MeasureMode, FlexiNoteLayer::mouseMoveEvent(), ViewManager::NavigateMode, Layer::nearestMeasurementRectChanged(), ViewManager::NoteEditMode, ViewManager::SelectMode, ViewManager::shouldIlluminateLocalFeatures(), UnresolvedDrag, updateContextHelp(), updateDragMode(), and VerticalDrag.
Referenced by mouseReleaseEvent().
|
overrideprotectedvirtual |
Definition at line 2267 of file Pane.cpp.
References Layer::addNote(), ViewManager::clearInProgressSelection(), doubleClickSelectInvoked(), ViewManager::EditMode, Layer::editOpen(), View::getFrameForX(), View::getInteractionLayer(), ViewManager::getOpportunisticEditingEnabled(), ViewManager::getToolModeFor(), View::getTopLayer(), getTopLayerDisplayExtents(), Layer::isLayerEditable(), m_altPressed, m_clickedInRange, m_clickPos, m_ctrlPressed, m_dragCentreFrame, m_dragMode, m_dragStartMinValue, View::m_manager, m_playbackFrameMoveScheduled, m_shiftPressed, Layer::measureDoubleClick(), ViewManager::MeasureMode, ViewManager::NavigateMode, ViewManager::NoteEditMode, ViewManager::SelectMode, View::setCentreFrame(), and UnresolvedDrag.
|
overrideprotectedvirtual |
Definition at line 2341 of file Pane.cpp.
References m_mouseInWidget.
|
overrideprotectedvirtual |
Definition at line 2347 of file Pane.cpp.
References View::contextHelpChanged(), m_identifyFeatures, and m_mouseInWidget.
|
overrideprotectedvirtual |
Definition at line 2363 of file Pane.cpp.
References m_pendingWheelAngle, wheelHorizontal(), wheelHorizontalFine(), and wheelVertical().
|
overrideprotectedvirtual |
Definition at line 2357 of file Pane.cpp.
References updateHeadsUpDisplay().
|
overrideprotectedvirtual |
|
overrideprotectedvirtual |
Definition at line 2642 of file Pane.cpp.
References dropAccepted().
|
protected |
Definition at line 2440 of file Pane.cpp.
References View::getZoomConstraintLevel(), m_vpan, m_vthumb, View::m_zoomLevel, paneInteractedWith(), Thumbwheel::scroll(), Panner::scroll(), and View::setZoomLevel().
Referenced by wheelEvent().
|
protected |
Definition at line 2484 of file Pane.cpp.
References wheelHorizontalFine().
Referenced by wheelEvent().
|
protected |
Definition at line 2492 of file Pane.cpp.
References View::getEndFrame(), View::getModelsEndFrame(), View::getStartFrame(), View::m_centreFrame, View::m_zoomLevel, paneInteractedWith(), and View::setCentreFrame().
Referenced by wheelEvent(), and wheelHorizontal().
|
protected |
Definition at line 539 of file Pane.cpp.
References View::getBackground(), View::getForeground(), View::getId(), View::getLayerCount(), Layer::getLayerPresentationName(), View::getScaleProvidingLayerForUnit(), Layer::getValueExtents(), Layer::getVerticalScaleWidth(), Layer::isLayerDormant(), Layer::isLayerOpaque(), View::m_layerStack, View::m_manager, m_scaleWidth, Layer::paintVerticalScale(), and ViewManager::shouldShowVerticalColourScale().
Referenced by paintEvent().
|
protected |
Definition at line 695 of file Pane.cpp.
References Layer::getFeatureDescription(), View::hasLightBackground(), and m_identifyPoint.
Referenced by paintEvent().
|
protected |
Definition at line 753 of file Pane.cpp.
References PaintAssistant::drawVisibleText(), ViewManager::getMainModelSampleRate(), View::hasLightBackground(), View::m_centreFrame, View::m_layerStack, View::m_manager, PaintAssistant::OutlinedText, Layer::PositionBottom, Layer::PositionMiddle, Layer::PositionTop, View::scalePen(), and ViewManager::shouldShowFrameCount().
Referenced by paintEvent().
|
protected |
Definition at line 827 of file Pane.cpp.
References View::getEndFrame(), View::getStartFrame(), View::getXForFrame(), and View::hasLightBackground().
Referenced by paintEvent().
|
protected |
Definition at line 1120 of file Pane.cpp.
References PaintAssistant::drawVisibleText(), ViewManager::getPlaybackSampleRate(), View::getProgressBarWidth(), View::m_manager, m_scaleWidth, and PaintAssistant::OutlinedText.
Referenced by paintEvent().
|
protected |
Definition at line 940 of file Pane.cpp.
References PaintAssistant::drawVisibleText(), m_scaleWidth, and PaintAssistant::OutlinedText.
Referenced by paintEvent().
|
protected |
Definition at line 975 of file Pane.cpp.
References TextAbbrev::abbreviate(), PaintAssistant::drawVisibleText(), View::getForeground(), ViewManager::getZoomWheelsEnabled(), View::m_layerStack, View::m_manager, PaintAssistant::OutlinedText, and ViewManager::scalePixelSize().
Referenced by paintEvent().
|
protected |
!! duplicating display policy with View::drawSelections
Definition at line 1049 of file Pane.cpp.
References PaintAssistant::drawVisibleText(), View::getForeground(), View::getFrameForX(), View::getModelsSampleRate(), View::getXForFrame(), m_clickPos, m_editingSelection, m_editingSelectionEdge, m_mousePos, and PaintAssistant::OutlinedText.
Referenced by paintEvent().
|
protected |
Definition at line 868 of file Pane.cpp.
References PaintAssistant::drawVisibleText(), m_scaleWidth, and PaintAssistant::OutlinedText.
Referenced by paintEvent().
|
overrideprotectedvirtual |
Reimplemented from View.
Definition at line 1169 of file Pane.cpp.
References View::getBackground(), View::getForeground(), View::getTopLayer(), View::m_manager, m_scaleWidth, Layer::paintVerticalScale(), View::render(), and ViewManager::shouldShowVerticalColourScale().
Referenced by renderPartToNewImage().
|
protected |
Definition at line 1267 of file Pane.cpp.
References ViewManager::getContainingSelection(), View::getFrameForX(), View::getXForFrame(), View::m_manager, and View::scalePixelSize().
Referenced by editSelectionStart(), mouseMoveEvent(), mousePressEvent(), shouldIlluminateLocalSelection(), and updateContextHelp().
|
protected |
Definition at line 2672 of file Pane.cpp.
References ViewManager::EditMode, getSelectionAt(), ViewManager::getToolModeFor(), m_editingSelection, m_editingSelectionEdge, m_identifyFeatures, View::m_manager, and m_mousePos.
Referenced by mouseMoveEvent().
|
protected |
Definition at line 2690 of file Pane.cpp.
References m_editingSelection, and m_mousePos.
Referenced by mouseMoveEvent().
|
protected |
Definition at line 2699 of file Pane.cpp.
References ViewManager::addSelection(), CommandHistory::endCompoundOperation(), View::getFrameForX(), CommandHistory::getInstance(), View::getInteractionLayer(), View::getXForFrame(), m_clickPos, m_editingSelection, m_editingSelectionEdge, View::m_manager, m_mousePos, Layer::moveSelection(), ViewManager::removeSelection(), Layer::resizeSelection(), and CommandHistory::startCompoundOperation().
Referenced by mouseReleaseEvent().
|
protected |
Definition at line 341 of file Pane.cpp.
References View::getFrameForX(), m_clickPos, m_editingSelection, and m_mousePos.
Referenced by paintEvent(), and shouldIlluminateLocalSelection().
|
protected |
Definition at line 113 of file Pane.cpp.
References View::countZoomLevels(), editVerticalPannerExtents(), Layer::getCurrentVerticalZoomStep(), Thumbwheel::getDefaultValue(), View::getLayer(), View::getLayerCount(), Layer::getNewVerticalZoomRangeMapper(), Layer::getVerticalZoomSteps(), View::getZoomLevel(), View::getZoomLevelIndex(), ViewManager::getZoomWheelsEnabled(), horizontalThumbwheelMoved(), m_headsUpDisplay, m_hthumb, View::m_manager, m_reset, m_vpan, m_vthumb, mouseEnteredWidget(), mouseLeftWidget(), ViewManager::scalePixelSize(), Panner::setAlpha(), Thumbwheel::setDefaultValue(), Thumbwheel::setMaximumValue(), Thumbwheel::setMinimumValue(), Thumbwheel::setRangeMapper(), Thumbwheel::setSpeed(), Thumbwheel::setValue(), updateVerticalPanner(), verticalPannerContextMenuRequested(), verticalPannerMoved(), and verticalThumbwheelMoved().
Referenced by layerParametersChanged(), Pane(), propertyContainerSelected(), resizeEvent(), verticalThumbwheelMoved(), viewZoomLevelChanged(), and zoomWheelsEnabledChanged().
|
protected |
Definition at line 256 of file Pane.cpp.
References View::getLayer(), View::getLayerCount(), getTopLayerDisplayExtents(), Layer::getVerticalZoomSteps(), ViewManager::getZoomWheelsEnabled(), View::m_manager, m_vpan, and Panner::setRectExtents().
Referenced by dragTopLayer(), editVerticalPannerExtents(), updateHeadsUpDisplay(), verticalThumbwheelMoved(), and zoomToRegion().
|
protected |
Definition at line 1302 of file Pane.cpp.
References getTopLayerDisplayExtents().
Referenced by dragTopLayer().
|
protected |
Definition at line 1326 of file Pane.cpp.
References View::getTopLayer(), and Layer::setDisplayExtents().
Referenced by dragTopLayer(), editVerticalPannerExtents(), and verticalPannerMoved().
|
protected |
Definition at line 1311 of file Pane.cpp.
References Layer::getDisplayExtents(), View::getTopLayer(), and Layer::getValueExtents().
Referenced by canTopLayerMoveVertical(), dragTopLayer(), editVerticalPannerExtents(), mouseDoubleClickEvent(), mousePressEvent(), updateVerticalPanner(), verticalPannerContextMenuRequested(), and verticalPannerMoved().
|
protected |
Definition at line 2001 of file Pane.cpp.
References canTopLayerMoveVertical(), FreeDrag, View::getFrameForX(), View::getModelsEndFrame(), getTopLayerDisplayExtents(), View::getXForFrame(), HorizontalDrag, ViewManager::isPlaying(), m_altPressed, View::m_centreFrame, m_clickPos, m_dragCentreFrame, m_dragMode, m_dragStartMinValue, View::m_manager, View::setCentreFrame(), setTopLayerDisplayExtents(), UnresolvedDrag, updateDragMode(), updateVerticalPanner(), and VerticalDrag.
Referenced by mouseMoveEvent().
|
protected |
Definition at line 2177 of file Pane.cpp.
References View::alignToReference(), edgeScrollMaybe(), View::getFrameForX(), ViewManager::getInProgressSelection(), View::getInteractionLayer(), View::getModelsEndFrame(), ViewManager::haveInProgressSelection(), m_ctrlPressed, View::m_manager, m_playbackFrameMoveScheduled, m_resizing, m_selectionStartFrame, m_shiftPressed, ViewManager::setInProgressSelection(), Layer::SnapLeft, Layer::SnapRight, and Layer::snapToFeatureFrame().
Referenced by mouseMoveEvent().
|
protected |
!! could call through to a layer function to find out exact meaning
!! could call through to a layer function to find out exact meaning
!! could call through to layer
Definition at line 2873 of file Pane.cpp.
References View::contextHelpChanged(), ViewManager::DrawMode, ViewManager::EditMode, ViewManager::EraseMode, View::getInteractionLayer(), getSelectionAt(), ViewManager::getSelections(), ViewManager::getToolModeFor(), View::hasTopLayerTimeXAxis(), Layer::isLayerEditable(), m_clickedInRange, View::m_manager, ViewManager::NavigateMode, and ViewManager::SelectMode.
Referenced by mouseMoveEvent().
|
protected |
Definition at line 2238 of file Pane.cpp.
References View::getEndFrame(), View::getFrameForX(), View::getStartFrame(), ViewManager::isPlaying(), View::m_centreFrame, View::m_followPlay, View::m_manager, PlaybackScrollContinuous, and View::setCentreFrame().
Referenced by dragExtendSelection(), and mouseMoveEvent().
|
protected |
Definition at line 1359 of file Pane.cpp.
References LayerFactory::FlexiNotes, LayerFactory::getInstance(), LayerFactory::getLayerType(), and View::m_layerStack.
Referenced by mouseMoveEvent(), mousePressEvent(), and mouseReleaseEvent().
|
protected |
Definition at line 1519 of file Pane.cpp.
References m_playbackFrameMoveScheduled, m_playbackFrameMoveTo, and playbackScheduleTimerElapsed().
Referenced by mousePressEvent().
|
protected |
Definition at line 2113 of file Pane.cpp.
References FreeDrag, HorizontalDrag, View::m_manager, ViewManager::scalePixelSize(), UnresolvedDrag, and VerticalDrag.
Referenced by dragTopLayer(), and mouseMoveEvent().
|
inlineoverridevirtualinherited |
Retrieve the id of this object.
Views have their own unique ids, but ViewProxy objects share the id of their View.
Implements LayerGeometryProvider.
Definition at line 72 of file View.h.
References View::getStartFrame(), View::m_id, and View::setStartFrame().
Referenced by AlignmentView::buildMaps(), View::checkAlignmentProgress(), View::checkProgress(), drawVerticalScale(), View::getFrameForX(), ViewProxy::getId(), View::getScaleProvidingLayerForUnit(), View::getVisibleExtentsForUnit(), View::getXForFrame(), View::globalCentreFrameChanged(), AlignmentView::keyFramesChanged(), View::modelAlignmentCompletionChanged(), View::modelChanged(), View::modelChangedWithin(), View::modelCompletionChanged(), View::modelReplaced(), mouseReleaseEvent(), View::movePlayPointer(), AlignmentView::paintEvent(), View::paintEvent(), View::progressCheckStalledTimerElapsed(), View::setCentreFrame(), View::viewManagerPlaybackFrameChanged(), and View::viewZoomLevelChanged().
|
overridevirtualinherited |
Retrieve the first visible sample frame on the widget.
This is a calculated value based on the centre-frame, widget width and zoom level. The result may be negative.
Implements LayerGeometryProvider.
Definition at line 445 of file View.cpp.
References View::getFrameForX().
Referenced by drawModelTimeExtents(), View::drawPlayPointer(), edgeScrollMaybe(), View::getFirstVisibleFrame(), View::getId(), ViewProxy::getStartFrame(), View::modelChangedWithin(), View::movePlayPointer(), Overview::paintEvent(), View::paintEvent(), View::scroll(), wheelHorizontalFine(), and zoomToRegion().
|
inherited |
Set the widget pan based on the given first visible frame.
The frame value may be negative.
Definition at line 457 of file View.cpp.
References View::m_zoomLevel, and View::setCentreFrame().
Referenced by View::getId(), and zoomToRegion().
|
inlineoverridevirtualinherited |
Return the centre frame of the visible widget.
This is an exact value that does not depend on the zoom block size. Other frame values (start, end) are calculated from this based on the zoom and other factors.
Implements LayerGeometryProvider.
Definition at line 93 of file View.h.
References View::m_centreFrame.
Referenced by View::drawPlayPointer(), ViewProxy::getCentreFrame(), View::getFrameForX(), and View::getXForFrame().
|
inlineinherited |
Set the centre frame of the visible widget.
Definition at line 98 of file View.h.
References View::getEndFrame(), View::getFrameForX(), View::getXForFrame(), and View::setCentreFrame().
Referenced by dragTopLayer(), edgeScrollMaybe(), View::globalCentreFrameChanged(), mouseDoubleClickEvent(), View::movePlayPointer(), View::scroll(), View::setCentreFrame(), View::setStartFrame(), View::setViewManager(), and wheelHorizontalFine().
|
protectedinherited |
Definition at line 464 of file View.cpp.
References View::alignToReference(), View::centreFrameChanged(), View::getId(), View::m_centreFrame, View::m_followPan, View::m_followPlay, and View::m_zoomLevel.
|
overridevirtualinherited |
Retrieve the last visible sample frame on the widget.
This is a calculated value based on the centre-frame, widget width and zoom level.
Implements LayerGeometryProvider.
Definition at line 451 of file View.cpp.
References View::getFrameForX().
Referenced by drawModelTimeExtents(), View::drawPlayPointer(), edgeScrollMaybe(), ViewProxy::getEndFrame(), View::getLastVisibleFrame(), View::modelChangedWithin(), View::movePlayPointer(), View::scroll(), View::setCentreFrame(), wheelHorizontalFine(), and zoomToRegion().
|
overridevirtualinherited |
Return the pixel x-coordinate corresponding to a given sample frame.
The frame is permitted to be negative, and the result may be outside the currently visible area. But this should not be called with frame values very far away from the currently visible area, as that could lead to overflow. In that situation an error will be logged and 0 returned.
Implements LayerGeometryProvider.
Definition at line 527 of file View.cpp.
References View::getCentreFrame(), View::getFrameForX(), View::getId(), View::m_centreFrame, and View::m_zoomLevel.
Referenced by dragTopLayer(), drawEditingSelection(), drawModelTimeExtents(), View::drawPlayPointer(), View::drawSelections(), editSelectionEnd(), View::getFrameForX(), getSelectionAt(), ViewProxy::getXForFrame(), View::movePlayPointer(), AlignmentView::paintEvent(), Overview::paintEvent(), View::paintEvent(), View::setCentreFrame(), and Overview::viewManagerPlaybackFrameChanged().
|
overridevirtualinherited |
Return the closest frame to the given pixel x-coordinate.
Implements LayerGeometryProvider.
Definition at line 600 of file View.cpp.
References View::getCentreFrame(), View::getId(), View::getXForFrame(), View::m_centreFrame, and View::m_zoomLevel.
Referenced by dragExtendSelection(), dragTopLayer(), drawEditingSelection(), View::drawSelections(), edgeScrollMaybe(), editSelectionEnd(), View::getEndFrame(), getFirstVisibleFrame(), ViewProxy::getFrameForX(), getSelectionAt(), View::getStartFrame(), View::getXForFrame(), Overview::mouseDoubleClickEvent(), mouseDoubleClickEvent(), Overview::mousePressEvent(), mousePressEvent(), mouseReleaseEvent(), View::movePlayPointer(), Overview::paintEvent(), selectionIsBeingEdited(), View::setCentreFrame(), and zoomToRegion().
|
inlineoverridevirtualinherited |
Return the closest pixel x-coordinate corresponding to a given view x-coordinate.
Default is no scaling, ViewProxy handles scaling case.
Implements LayerGeometryProvider.
|
inlineoverridevirtualinherited |
Return the closest view x-coordinate corresponding to a given pixel x-coordinate.
Default is no scaling, ViewProxy handles scaling case.
Implements LayerGeometryProvider.
Definition at line 134 of file View.h.
References View::addLayer(), View::getFrequencyForY(), View::getYForFrequency(), View::getZoomLevel(), View::removeLayer(), View::scroll(), View::setZoomLevel(), and View::zoom().
|
overridevirtualinherited |
Return the pixel y-coordinate corresponding to a given frequency, if the frequency range is as specified.
This does not imply any policy about layer frequency ranges, but it might be useful for layers to match theirs up if desired.
Not thread-safe in logarithmic mode. Call only from GUI thread.
Implements LayerGeometryProvider.
Definition at line 666 of file View.cpp.
Referenced by View::getViewXForX(), and ViewProxy::getYForFrequency().
|
overridevirtualinherited |
Return the closest frequency to the given pixel y-coordinate, if the frequency range is as specified.
Not thread-safe in logarithmic mode. Call only from GUI thread.
Implements LayerGeometryProvider.
Definition at line 700 of file View.cpp.
Referenced by ViewProxy::getFrequencyForY(), and View::getViewXForX().
|
overridevirtualinherited |
Return the zoom level, i.e.
the number of frames per pixel or pixels per frame
Implements LayerGeometryProvider.
Definition at line 732 of file View.cpp.
References View::m_zoomLevel.
Referenced by View::getRenderedPartImageSize(), View::getViewXForX(), ViewProxy::getZoomLevel(), renderPartToNewImage(), View::renderPartToNewImage(), View::renderPartToSvgFile(), and updateHeadsUpDisplay().
|
virtualinherited |
Set the zoom level, i.e.
the number of frames per pixel or pixels per frame. The centre frame will be unchanged; the start and end frames will change.
!! int dpratio = effectiveDevicePixelRatio();
Definition at line 760 of file View.cpp.
References View::m_followZoom, View::m_zoomLevel, and View::zoomLevelChanged().
Referenced by View::getViewXForX(), horizontalThumbwheelMoved(), View::setViewManager(), View::viewZoomLevelChanged(), wheelVertical(), View::zoom(), and zoomToRegion().
|
virtualinherited |
Zoom in or out.
Definition at line 1879 of file View.cpp.
References View::getZoomConstraintLevel(), View::m_zoomLevel, and View::setZoomLevel().
Referenced by View::getViewXForX().
|
virtualinherited |
Scroll left or right by a smallish or largish amount.
Definition at line 1899 of file View.cpp.
References View::getEndFrame(), View::getModelsEndFrame(), View::getStartFrame(), View::m_centreFrame, and View::setCentreFrame().
Referenced by View::getViewXForX().
|
virtualinherited |
Add a layer to the view.
(Normally this should be handled through some command abstraction instead of using this function directly.)
Definition at line 838 of file View.cpp.
References View::ProgressBarRec::bar, View::ProgressBarRec::cancel, View::cancelClicked(), View::ProgressBarRec::lastStallCheckValue, View::layerMeasurementRectsChanged(), View::layerNameChanged(), View::layerParameterRangesChanged(), View::layerParametersChanged(), View::m_cacheValid, View::m_fixedOrderLayers, View::m_layerStack, View::m_progressBars, View::modelAlignmentCompletionChanged(), View::modelChanged(), View::modelChangedWithin(), View::modelCompletionChanged(), View::modelReplaced(), View::progressCheckStalledTimerElapsed(), View::propertyContainerAdded(), View::scalePixelSize(), SingleColourLayer::setDefaultColourFor(), and View::ProgressBarRec::stallCheckTimer.
Referenced by View::getViewXForX().
|
virtualinherited |
Remove a layer from the view.
Does not delete the layer. (Normally this should be handled through some command abstraction instead of using this function directly.)
Definition at line 905 of file View.cpp.
References View::layerNameChanged(), View::layerParameterRangesChanged(), View::layerParametersChanged(), View::m_cacheValid, View::m_deleting, View::m_fixedOrderLayers, View::m_layerStack, View::m_progressBars, View::modelAlignmentCompletionChanged(), View::modelChanged(), View::modelChangedWithin(), View::modelCompletionChanged(), View::modelReplaced(), and View::propertyContainerRemoved().
Referenced by View::getViewXForX().
|
inlinevirtualinherited |
Return the number of layers, regardless of whether visible or dormant, i.e.
invisible, in this view.
Definition at line 197 of file View.h.
References View::m_layerStack.
Referenced by LayerTreeModel::data(), drawVerticalScale(), FlexiNoteLayer::getAssociatedPitchModel(), View::getInteractionLayer(), View::getModels(), AlignmentView::getSalientModel(), View::getSelectedLayer(), LayerTreeModel::LayerTreeModel(), LayerTreeModel::playParametersAudibilityChanged(), LayerTreeModel::propertyContainerPropertyChanged(), propertyContainerSelected(), ModelMetadataModel::rebuildModelSet(), LayerTreeModel::rowCount(), LayerTreeModel::setData(), updateHeadsUpDisplay(), updateVerticalPanner(), verticalThumbwheelMoved(), and verticalZoomChanged().
|
inlinevirtualinherited |
Return the nth layer, counted in stacking order.
That is, layer 0 is the bottom layer and layer "getLayerCount()-1" is the top one. The returned layer may be visible or it may be dormant, i.e. invisible.
Definition at line 205 of file View.h.
References View::m_layerStack.
Referenced by LayerTreeModel::data(), FlexiNoteLayer::getAssociatedPitchModel(), View::getInteractionLayer(), View::getModels(), AlignmentView::getSalientModel(), View::getSelectedLayer(), LayerTreeModel::LayerTreeModel(), TimeRulerLayer::paint(), LayerTreeModel::playParametersAudibilityChanged(), LayerTreeModel::propertyContainerPropertyChanged(), propertyContainerSelected(), ModelMetadataModel::rebuildModelSet(), LayerTreeModel::setData(), FlexiNoteLayer::setVerticalRangeToNoteRange(), updateHeadsUpDisplay(), updateVerticalPanner(), verticalThumbwheelMoved(), and verticalZoomChanged().
|
inlinevirtualinherited |
Return the nth layer, counted in the order they were added.
Unlike the stacking order used in getLayer(), which changes each time a layer is selected, this ordering remains fixed. The returned layer may be visible or it may be dormant, i.e. invisible.
Definition at line 217 of file View.h.
References View::getInteractionLayer(), View::getSelectedLayer(), and View::m_fixedOrderLayers.
|
virtualinherited |
Return the layer currently active for tool interaction.
This is the topmost non-dormant (i.e. visible) layer in the view. If there are no visible layers in the view, return 0.
Definition at line 960 of file View.cpp.
References View::getLayer(), View::getLayerCount(), View::getSelectedLayer(), Layer::isLayerDormant(), and View::m_layerStack.
Referenced by dragExtendSelection(), editSelectionEnd(), View::getFixedOrderLayer(), View::getInteractionLayer(), mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), shouldIlluminateLocalFeatures(), shouldIlluminateLocalSelection(), updateContextHelp(), and zoomToRegion().
|
virtualinherited |
Definition at line 980 of file View.cpp.
References View::getInteractionLayer().
|
virtualinherited |
Return the layer most recently selected by the user.
This is the layer that any non-tool-driven commands should operate on, in the case where this view is the "current" one.
If the user has selected the view itself more recently than any of the layers on it, this function will return 0, and any non-tool-driven layer commands should be deactivated while this view is current. It will also return 0 if there are no layers in the view.
Note that, unlike getInteractionLayer(), this could return an invisible (dormant) layer.
Definition at line 986 of file View.cpp.
References View::getLayer(), View::getLayerCount(), View::m_haveSelectedLayer, and View::m_layerStack.
Referenced by View::getFixedOrderLayer(), View::getInteractionLayer(), and View::getSelectedLayer().
|
virtualinherited |
Definition at line 996 of file View.cpp.
References View::getSelectedLayer().
|
inlinevirtualinherited |
Return the "top" layer in the view, whether visible or dormant.
This is the same as getLayer(getLayerCount()-1) if there is at least one layer, and 0 otherwise.
For most purposes involving interaction or commands, you probably want either getInteractionLayer() or getSelectedLayer() instead.
Definition at line 258 of file View.h.
References View::m_layerStack, and View::setViewManager().
Referenced by getRenderedPartImageSize(), getTopLayerDisplayExtents(), mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), paintEvent(), render(), renderPartToNewImage(), and setTopLayerDisplayExtents().
|
virtualinherited |
Definition at line 1002 of file View.cpp.
References View::centreFrameChanged(), View::getAlignedPlaybackFrame(), ViewManager::getGlobalCentreFrame(), ViewManager::getGlobalZoom(), ViewManager::getPlaybackFrame(), View::globalCentreFrameChanged(), View::m_followPan, View::m_followPlay, View::m_followZoom, View::m_manager, View::movePlayPointer(), View::overlayModeChanged(), PlaybackIgnore, PlaybackScrollContinuous, PlaybackScrollPage, PlaybackScrollPageWithCentre, View::selectionChanged(), View::setCentreFrame(), View::setZoomLevel(), View::toolModeChanged(), View::viewCentreFrameChanged(), View::viewManagerPlaybackFrameChanged(), View::viewZoomLevelChanged(), View::zoomLevelChanged(), and View::zoomWheelsEnabledChanged().
Referenced by PaneStack::addPane(), View::getTopLayer(), and View::setViewManager().
|
virtualinherited |
Definition at line 1076 of file View.cpp.
References View::setCentreFrame(), and View::setViewManager().
|
inlineoverridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 264 of file View.h.
References View::m_manager, and View::setFollowGlobalPan().
Referenced by ViewProxy::getViewManager().
|
virtualinherited |
Definition at line 1083 of file View.cpp.
References View::m_followPan, View::m_propertyContainer, and View::propertyContainerPropertyChanged().
Referenced by View::getViewManager(), and View::setProperty().
|
inlinevirtualinherited |
Definition at line 267 of file View.h.
References View::m_followPan, and View::setFollowGlobalZoom().
|
virtualinherited |
Definition at line 1090 of file View.cpp.
References View::m_followZoom, View::m_propertyContainer, and View::propertyContainerPropertyChanged().
Referenced by View::getFollowGlobalPan(), and View::setProperty().
|
inlinevirtualinherited |
Definition at line 270 of file View.h.
References View::drawMeasurementRect(), View::getBackground(), View::getForeground(), View::hasLightBackground(), and View::m_followZoom.
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 774 of file View.cpp.
References Layer::ColourAbsent, Layer::ColourDistinguishes, ViewManager::getGlobalDarkBackground(), View::m_layerStack, and View::m_manager.
Referenced by drawCentreLine(), drawFeatureDescription(), View::drawMeasurementRect(), drawModelTimeExtents(), View::getBackground(), View::getFollowGlobalZoom(), View::getForeground(), ViewProxy::hasLightBackground(), and Overview::Overview().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 824 of file View.cpp.
References View::hasLightBackground().
Referenced by drawEditingSelection(), drawLayerNames(), View::drawPlayPointer(), View::drawSelections(), drawVerticalScale(), View::getFollowGlobalZoom(), ViewProxy::getForeground(), View::paintEvent(), render(), and View::render().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 804 of file View.cpp.
References View::hasLightBackground().
Referenced by View::drawPlayPointer(), drawVerticalScale(), ViewProxy::getBackground(), View::getFollowGlobalZoom(), View::paintEvent(), render(), and View::render().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 2767 of file View.cpp.
References PaintAssistant::drawVisibleText(), Layer::getXScaleValue(), Layer::getYScaleDifference(), Layer::getYScaleValue(), View::hasLightBackground(), and PaintAssistant::OutlinedText.
Referenced by ViewProxy::drawMeasurementRect(), and View::getFollowGlobalZoom().
|
inlineoverridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 279 of file View.h.
References View::m_manager, and ViewManager::shouldShowFeatureLabels().
Referenced by ViewProxy::shouldShowFeatureLabels().
|
virtualinherited |
Definition at line 1097 of file View.cpp.
References View::m_followPlay, View::m_propertyContainer, and View::propertyContainerPropertyChanged().
Referenced by Overview::Overview(), View::setProperty(), and View::shouldIlluminateLocalSelection().
|
inlinevirtualinherited |
Definition at line 290 of file View.h.
References View::m_followPlay.
|
virtualinherited |
|
virtualinherited |
|
virtualinherited |
|
virtualinherited |
Definition at line 122 of file View.cpp.
References View::m_followPan, View::m_followPlay, View::m_followZoom, PlaybackIgnore, PlaybackScrollContinuous, PlaybackScrollPage, and PlaybackScrollPageWithCentre.
|
virtualinherited |
|
virtualinherited |
Definition at line 160 of file View.cpp.
References PlaybackIgnore, PlaybackScrollContinuous, PlaybackScrollPageWithCentre, View::setFollowGlobalPan(), View::setFollowGlobalZoom(), and View::setPlaybackFollow().
|
inlinevirtualinherited |
Definition at line 306 of file View.h.
References View::getFirstVisibleFrame(), View::getLastVisibleFrame(), View::getModelsEndFrame(), View::getModelsStartFrame(), View::getPaintRect(), View::getPropertyContainer(), View::getPropertyContainerCount(), View::getPropertyContainerIconName(), View::getRenderedImageSize(), View::getRenderedPartImageSize(), View::getTextLabelYCoord(), View::getVisibleExtentsForAnyUnit(), View::getVisibleExtentsForUnit(), View::renderPartToNewImage(), View::renderPartToSvgFile(), View::renderToNewImage(), View::renderToSvgFile(), and View::toXml().
|
virtualinherited |
Definition at line 177 of file View.cpp.
References View::m_fixedOrderLayers.
Referenced by PropertyStack::containsContainer(), View::getPropertyContainerName(), and PropertyStack::repopulate().
|
virtualinherited |
Definition at line 183 of file View.cpp.
Referenced by PropertyStack::containsContainer(), View::getPropertyContainerName(), PropertyStack::repopulate(), and PaneStack::setCurrentLayer().
|
virtualinherited |
Definition at line 190 of file View.cpp.
References View::m_fixedOrderLayers, and View::m_propertyContainer.
|
virtualinherited |
Render the view contents to a new SVG file.
Definition at line 3201 of file View.cpp.
References View::getModelsEndFrame(), View::getModelsStartFrame(), and View::renderPartToSvgFile().
Referenced by View::getPropertyContainerName().
|
virtualinherited |
Render the view contents between the given frame extents to a new SVG file.
Definition at line 3210 of file View.cpp.
References View::getZoomLevel(), and View::render().
Referenced by View::getPropertyContainerName(), and View::renderToSvgFile().
|
overridevirtualinherited |
Return the visible vertical extents for the given unit, if any.
Overridden from LayerGeometryProvider (see docs there).
Implements LayerGeometryProvider.
Definition at line 197 of file View.cpp.
References Layer::getDisplayExtents(), View::getId(), View::getScaleProvidingLayerForUnit(), Layer::getValueExtents(), and View::m_layerStack.
Referenced by View::getPropertyContainerName(), and ViewProxy::getVisibleExtentsForUnit().
|
inherited |
Return some visible vertical extents and unit.
That is, if at least one non-dormant layer has a non-empty unit and returns some values from its getDisplayExtents() method, return the extents and unit from the topmost of those. Otherwise return false.
Definition at line 323 of file View.cpp.
References Layer::getDisplayExtents(), Layer::getValueExtents(), Layer::isLayerDormant(), and View::m_layerStack.
Referenced by View::getPropertyContainerName().
|
overridevirtualinherited |
Return a y-coordinate at which text labels for individual items in a layer may be drawn, so as not to overlap with those of other layers.
The returned coordinate will be near the top of the visible widget, but adjusted downward depending on how many other visible layers return true from their implementation of Layer::needsTextLabelHeight().
Implements LayerGeometryProvider.
Definition at line 367 of file View.cpp.
References View::m_layerStack, and View::scalePixelSize().
Referenced by View::getPropertyContainerName(), and ViewProxy::getTextLabelYCoord().
|
virtualinherited |
Definition at line 1442 of file View.cpp.
References View::getEndFrame(), and View::getModelsEndFrame().
Referenced by View::getPropertyContainerName().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 1451 of file View.cpp.
References View::m_layerStack.
Referenced by AlignmentView::getDefaultKeyFrames(), View::getFirstVisibleFrame(), View::getModelsEndFrame(), ViewProxy::getModelsStartFrame(), View::getPropertyContainerName(), View::getRenderedImageSize(), Overview::modelChangedWithin(), Overview::paintEvent(), View::renderToNewImage(), and View::renderToSvgFile().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 1475 of file View.cpp.
References View::getModelsStartFrame(), and View::m_layerStack.
Referenced by dragExtendSelection(), dragTopLayer(), AlignmentView::getDefaultKeyFrames(), View::getLastVisibleFrame(), ViewProxy::getModelsEndFrame(), View::getPropertyContainerName(), View::getRenderedImageSize(), Overview::modelChangedWithin(), Overview::mouseMoveEvent(), Overview::paintEvent(), View::renderToNewImage(), View::renderToSvgFile(), View::scroll(), wheelHorizontalFine(), and zoomToRegion().
|
overridevirtualinherited |
To be called from a layer, to obtain the extent of the surface that the layer is currently painting to.
This may be the extent of the view (if 1x display scaling is in effect) or of a larger cached pixmap (if greater display scaling is in effect).
Implements LayerGeometryProvider.
Definition at line 2219 of file View.cpp.
Referenced by View::getPaintHeight(), ViewProxy::getPaintRect(), View::getPaintSize(), View::getPaintWidth(), and View::getPropertyContainerName().
|
inlineoverridevirtualinherited |
Reimplemented from LayerGeometryProvider.
Definition at line 391 of file View.h.
References View::getPaintRect().
|
inlineoverridevirtualinherited |
Reimplemented from LayerGeometryProvider.
Definition at line 392 of file View.h.
References View::getPaintRect().
|
inlineoverridevirtualinherited |
Reimplemented from LayerGeometryProvider.
Definition at line 393 of file View.h.
References View::getPaintRect(), View::scalePen(), View::scalePenWidth(), View::scalePixelSize(), and View::scaleSize().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 1807 of file View.cpp.
Referenced by View::getPaintHeight(), View::scalePenWidth(), View::scalePixelSize(), and ViewProxy::scaleSize().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 1834 of file View.cpp.
References View::scaleSize().
Referenced by View::addLayer(), View::checkProgress(), View::getPaintHeight(), getSelectionAt(), View::getTextLabelYCoord(), and ViewProxy::scalePixelSize().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 1843 of file View.cpp.
References View::scaleSize().
Referenced by View::drawSelections(), View::getPaintHeight(), View::scalePen(), and ViewProxy::scalePenWidth().
|
overridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 1853 of file View.cpp.
References View::scalePenWidth().
Referenced by drawCentreLine(), and View::getPaintHeight().
|
inherited |
Definition at line 1521 of file View.cpp.
References View::getLayer(), View::getLayerCount(), and Layer::getModel().
|
inlineinherited |
!!??? poor name, probably poor api, consider this
Definition at line 404 of file View.h.
References View::alignFromReference(), View::alignToReference(), View::getAlignedPlaybackFrame(), View::getAligningAndReferenceModels(), View::getAligningModel(), and View::m_useAligningProxy.
|
inherited |
!!
Definition at line 1542 of file View.cpp.
References View::getAligningAndReferenceModels().
Referenced by View::alignFromReference(), View::alignToReference(), View::getAlignedPlaybackFrame(), Overview::mousePressEvent(), View::paintEvent(), and View::setUseAligningProxy().
|
inherited |
Definition at line 1550 of file View.cpp.
References ViewManager::getAlignMode(), ViewManager::getPlaybackModel(), View::m_layerStack, and View::m_manager.
Referenced by View::getAligningModel(), and View::setUseAligningProxy().
|
inherited |
Definition at line 1597 of file View.cpp.
References View::getAligningModel(), ViewManager::getAlignMode(), and View::m_manager.
Referenced by Layer::alignFromReference(), AlignmentView::buildMaps(), View::drawSelections(), View::globalCentreFrameChanged(), Overview::paintEvent(), and View::setUseAligningProxy().
|
inherited |
Definition at line 1606 of file View.cpp.
References View::getAligningModel(), ViewManager::getAlignMode(), and View::m_manager.
Referenced by Layer::alignToReference(), AlignmentView::buildMaps(), dragExtendSelection(), Overview::mouseDoubleClickEvent(), Overview::mouseMoveEvent(), mousePressEvent(), Overview::paintEvent(), View::setCentreFrame(), and View::setUseAligningProxy().
|
inherited |
Definition at line 1615 of file View.cpp.
References View::getAligningModel(), ViewManager::getAlignMode(), ViewManager::getPlaybackFrame(), and View::m_manager.
Referenced by Overview::modelReplaced(), View::setUseAligningProxy(), View::setViewManager(), Overview::viewManagerPlaybackFrameChanged(), and View::viewManagerPlaybackFrameChanged().
|
inlineoverridevirtualinherited |
Implements LayerGeometryProvider.
|
inlineoverridevirtualinherited |
Implements LayerGeometryProvider.
|
inlineoverridevirtualinherited |
Implements LayerGeometryProvider.
Definition at line 418 of file View.h.
References View::cancelButtonPressed(), View::cancelClicked(), View::centreFrameChanged(), View::contextHelpChanged(), View::globalCentreFrameChanged(), View::layerMeasurementRectsChanged(), View::layerModelChanged(), View::layerNameChanged(), View::layerParameterRangesChanged(), View::layerParametersChanged(), View::modelAlignmentCompletionChanged(), View::modelChanged(), View::modelChangedWithin(), View::modelCompletionChanged(), View::modelReplaced(), View::overlayModeChanged(), View::progressCheckStalledTimerElapsed(), View::propertyChanged(), View::propertyContainerAdded(), View::propertyContainerNameChanged(), View::propertyContainerPropertyChanged(), View::propertyContainerPropertyRangeChanged(), View::propertyContainerRemoved(), View::propertyContainerSelected(), View::selectionChanged(), View::toolModeChanged(), View::View(), View::viewCentreFrameChanged(), View::viewManagerPlaybackFrameChanged(), View::viewZoomLevelChanged(), View::zoomLevelChanged(), and View::zoomWheelsEnabledChanged().
|
signalinherited |
Referenced by View::addLayer(), View::getView(), AlignmentView::setAboveView(), and AlignmentView::setBelowView().
|
signalinherited |
Referenced by View::getView(), and View::removeLayer().
|
signalinherited |
|
signalinherited |
Referenced by View::getView(), and View::layerParameterRangesChanged().
|
signalinherited |
Referenced by View::getView(), and View::layerNameChanged().
|
signalinherited |
Referenced by View::getView(), propertyContainerSelected(), and View::propertyContainerSelected().
|
signalinherited |
Referenced by View::getView().
|
signalinherited |
Referenced by View::getView(), View::modelChanged(), AlignmentView::setAboveView(), and AlignmentView::setBelowView().
|
signalinherited |
Referenced by View::cancelClicked(), and View::getView().
|
signalinherited |
|
signalinherited |
|
signalinherited |
|
virtualslotinherited |
Definition at line 1104 of file View.cpp.
References View::checkProgress(), View::getId(), View::getScrollableBackLayers(), View::layerModelChanged(), and View::m_cacheValid.
Referenced by View::addLayer(), View::getView(), AlignmentView::reconnectModels(), and View::removeLayer().
|
virtualslotinherited |
Definition at line 1137 of file View.cpp.
References View::checkProgress(), View::getEndFrame(), View::getId(), View::getScrollableBackLayers(), View::getStartFrame(), and View::m_cacheValid.
Referenced by View::addLayer(), View::getView(), Overview::modelChangedWithin(), and View::removeLayer().
|
virtualslotinherited |
Definition at line 1184 of file View.cpp.
References View::checkProgress(), and View::getId().
Referenced by View::addLayer(), View::getView(), and View::removeLayer().
|
virtualslotinherited |
Definition at line 1202 of file View.cpp.
References View::getId(), and View::m_cacheValid.
Referenced by View::addLayer(), View::getView(), Overview::modelReplaced(), and View::removeLayer().
|
virtualslotinherited |
Definition at line 1229 of file View.cpp.
References View::propertyContainerPropertyRangeChanged().
Referenced by View::addLayer(), View::getView(), and View::removeLayer().
|
virtualslotinherited |
Definition at line 1236 of file View.cpp.
Referenced by View::addLayer(), and View::getView().
|
virtualslotinherited |
Definition at line 1243 of file View.cpp.
References View::propertyContainerNameChanged().
Referenced by View::addLayer(), View::getView(), and View::removeLayer().
|
virtualslotinherited |
Definition at line 1250 of file View.cpp.
References View::alignFromReference(), View::getId(), View::m_followPan, and View::setCentreFrame().
Referenced by View::getView(), AlignmentView::globalCentreFrameChanged(), and View::setViewManager().
|
virtualslotinherited |
Definition at line 1263 of file View.cpp.
Referenced by View::getView(), View::setViewManager(), and AlignmentView::viewCentreFrameChanged().
|
virtualslotinherited |
Definition at line 1269 of file View.cpp.
References View::getAlignedPlaybackFrame(), View::getId(), View::m_manager, and View::movePlayPointer().
Referenced by View::getView(), and View::setViewManager().
|
virtualslotinherited |
Definition at line 1423 of file View.cpp.
References View::m_cacheValid, and View::m_selectionCached.
Referenced by View::getView(), and View::setViewManager().
|
virtualslotinherited |
Definition at line 432 of file View.cpp.
References View::m_cacheValid.
Referenced by View::getView(), and View::setViewManager().
|
virtualslotinherited |
Definition at line 1925 of file View.cpp.
References View::cancelButtonPressed(), and View::m_progressBars.
Referenced by View::addLayer(), and View::getView().
|
virtualslotinherited |
Definition at line 2146 of file View.cpp.
References View::getId(), and View::m_progressBars.
Referenced by View::addLayer(), and View::getView().
|
protectedvirtualinherited |
Definition at line 2571 of file View.cpp.
References View::alignFromReference(), View::areLayerColoursSignificant(), PaintAssistant::drawVisibleText(), View::getForeground(), View::getFrameForX(), ViewManager::getInProgressSelection(), View::getModelsSampleRate(), ViewManager::getSelections(), View::getXForFrame(), View::hasTopLayerTimeXAxis(), ViewManager::haveInProgressSelection(), View::m_manager, PaintAssistant::OutlinedText, View::scalePenWidth(), View::shouldIlluminateLocalSelection(), View::shouldLabelSelections(), and ViewManager::shouldShowSelectionExtents().
Referenced by View::paintEvent().
|
inlineprotectedvirtualinherited |
Reimplemented in Overview, and AlignmentView.
Definition at line 485 of file View.h.
References View::drawPlayPointer(), View::render(), and View::setPaintFont().
Referenced by View::drawSelections().
|
protectedvirtualinherited |
Definition at line 2733 of file View.cpp.
References View::getBackground(), View::getCentreFrame(), View::getEndFrame(), View::getForeground(), View::getStartFrame(), View::getXForFrame(), ViewManager::isPlaying(), View::m_followPlay, View::m_manager, View::m_playPointerFrame, PlaybackIgnore, PlaybackScrollContinuous, and ViewManager::shouldShowCentreLine().
Referenced by View::paintEvent(), and View::shouldLabelSelections().
|
protectedvirtualinherited |
Definition at line 2190 of file View.cpp.
References View::effectiveDevicePixelRatio().
Referenced by paintEvent(), View::paintEvent(), and View::shouldLabelSelections().
|
inlineprotectedinherited |
Definition at line 490 of file View.h.
Referenced by View::paintEvent().
|
inlineprotectedinherited |
Definition at line 493 of file View.h.
Referenced by View::paintEvent().
|
protectedinherited |
!! Just go for the first, for now. If we were supporting
!! nah, this wants to always return the sr of the main model!
Definition at line 1500 of file View.cpp.
References View::m_layerStack.
Referenced by drawEditingSelection(), View::drawSelections(), and paintEvent().
|
protectedinherited |
Definition at line 1630 of file View.cpp.
References View::m_layerStack.
|
protectedinherited |
Definition at line 1640 of file View.cpp.
References View::m_lastScrollableBackLayers, and View::m_layerStack.
Referenced by View::modelChanged(), View::modelChangedWithin(), and View::paintEvent().
|
protectedinherited |
Definition at line 1676 of file View.cpp.
References View::m_lastNonScrollableBackLayers, and View::m_layerStack.
Referenced by View::paintEvent().
|
protectedinherited |
Definition at line 246 of file View.cpp.
References Layer::getDisplayExtents(), View::getId(), Layer::getLayerPresentationName(), Layer::getValueExtents(), Layer::isLayerDormant(), and View::m_layerStack.
Referenced by drawVerticalScale(), and View::getVisibleExtentsForUnit().
|
protectedinherited |
Definition at line 1708 of file View.cpp.
References View::m_layerStack.
Referenced by View::countZoomLevels(), View::getZoomLevelByIndex(), View::getZoomLevelIndex(), Overview::modelChangedWithin(), Overview::paintEvent(), View::paintEvent(), wheelVertical(), View::zoom(), and zoomToRegion().
|
protectedinherited |
Definition at line 1738 of file View.cpp.
References View::getZoomConstraintLevel().
Referenced by updateHeadsUpDisplay().
|
protectedinherited |
Definition at line 1782 of file View.cpp.
References View::getZoomConstraintLevel().
Referenced by updateHeadsUpDisplay().
|
protectedinherited |
Definition at line 1757 of file View.cpp.
References View::getZoomConstraintLevel().
Referenced by horizontalThumbwheelMoved().
|
protectedinherited |
Definition at line 1859 of file View.cpp.
References Layer::ColourHasMeaningfulValue, and View::m_layerStack.
Referenced by View::drawSelections().
|
protectedinherited |
Definition at line 1870 of file View.cpp.
References View::m_layerStack.
Referenced by View::drawSelections(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), paintEvent(), and updateContextHelp().
|
protectedinherited |
Definition at line 1289 of file View.cpp.
References View::getEndFrame(), View::getFrameForX(), View::getId(), ViewManager::getPlaySelectionMode(), ViewManager::getSelections(), View::getStartFrame(), View::getXForFrame(), ViewManager::isPlaying(), View::m_followPlay, View::m_followPlayIsDetached, View::m_manager, View::m_playPointerFrame, PlaybackIgnore, PlaybackScrollContinuous, PlaybackScrollPage, PlaybackScrollPageWithCentre, and View::setCentreFrame().
Referenced by View::setViewManager(), and View::viewManagerPlaybackFrameChanged().
|
protectedinherited |
Definition at line 1946 of file View.cpp.
References View::AlignmentProgressBarRec::bar, View::getId(), View::m_alignmentProgressBar, View::m_lastError, View::m_progressBars, View::m_showProgress, and View::scalePixelSize().
Referenced by View::modelChanged(), View::modelChangedWithin(), and View::modelCompletionChanged().
|
protectedinherited |
Definition at line 2080 of file View.cpp.
References View::AlignmentProgressBarRec::alignedModel, View::AlignmentProgressBarRec::bar, View::getId(), View::m_alignmentProgressBar, and View::m_showProgress.
Referenced by View::modelAlignmentCompletionChanged().
|
protectedinherited |
Definition at line 3006 of file View.cpp.
References View::m_layerStack.
Referenced by View::render(), and View::renderToNewImage().
|
protectedinherited |
Definition at line 2173 of file View.cpp.
References View::AlignmentProgressBarRec::bar, View::m_alignmentProgressBar, and View::m_progressBars.
Referenced by drawDurationAndRate().
|
protectedinherited |
Definition at line 741 of file View.cpp.
Referenced by View::paintEvent(), and View::setPaintFont().
|
inlinestaticprotectedinherited |
Definition at line 48 of file LayerGeometryProvider.h.
Member Data Documentation
|
protected |
Definition at line 171 of file Pane.h.
Referenced by editSelectionStart(), leaveEvent(), mouseMoveEvent(), paintEvent(), shouldIlluminateLocalFeatures(), and shouldIlluminateLocalSelection().
|
protected |
Definition at line 172 of file Pane.h.
Referenced by drawFeatureDescription(), mouseMoveEvent(), paintEvent(), shouldIlluminateLocalFeatures(), and shouldIlluminateLocalSelection().
|
protected |
Definition at line 173 of file Pane.h.
Referenced by dragTopLayer(), drawEditingSelection(), editSelectionEnd(), mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), paintEvent(), and selectionIsBeingEdited().
|
protected |
Definition at line 174 of file Pane.h.
Referenced by drawEditingSelection(), editSelectionDrag(), editSelectionEnd(), editSelectionStart(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), paintEvent(), and selectionIsBeingEdited().
|
protected |
Definition at line 175 of file Pane.h.
Referenced by mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), paintEvent(), and updateContextHelp().
|
protected |
Definition at line 176 of file Pane.h.
Referenced by dragExtendSelection(), mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), and paintEvent().
|
protected |
Definition at line 177 of file Pane.h.
Referenced by dragExtendSelection(), mouseDoubleClickEvent(), and mousePressEvent().
|
protected |
Definition at line 178 of file Pane.h.
Referenced by dragTopLayer(), mouseDoubleClickEvent(), and mousePressEvent().
|
protected |
Definition at line 180 of file Pane.h.
Referenced by mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), and paintEvent().
|
protected |
Definition at line 181 of file Pane.h.
Referenced by dragExtendSelection(), and mousePressEvent().
|
protected |
Definition at line 182 of file Pane.h.
Referenced by mouseMoveEvent(), mousePressEvent(), and mouseReleaseEvent().
|
protected |
Definition at line 183 of file Pane.h.
Referenced by mouseMoveEvent(), mousePressEvent(), and mouseReleaseEvent().
|
protected |
Definition at line 184 of file Pane.h.
Referenced by dragTopLayer(), mouseDoubleClickEvent(), and mousePressEvent().
|
protected |
Definition at line 185 of file Pane.h.
Referenced by dragTopLayer(), mouseDoubleClickEvent(), and mousePressEvent().
|
protected |
Definition at line 186 of file Pane.h.
Referenced by getCentreLineVisible(), paintEvent(), setCentreLineVisible(), and toXml().
|
protected |
Definition at line 187 of file Pane.h.
Referenced by dragExtendSelection(), and mousePressEvent().
|
protected |
Definition at line 188 of file Pane.h.
Referenced by drawEditingSelection(), editSelectionDrag(), editSelectionEnd(), editSelectionStart(), mousePressEvent(), and selectionIsBeingEdited().
|
protected |
Definition at line 189 of file Pane.h.
Referenced by drawEditingSelection(), editSelectionEnd(), editSelectionStart(), and mousePressEvent().
|
mutableprotected |
Definition at line 190 of file Pane.h.
Referenced by drawAlignmentStatus(), drawDurationAndRate(), drawVerticalScale(), drawWorkTitle(), getFirstVisibleFrame(), getVerticalScaleWidth(), paintEvent(), render(), and renderPartToNewImage().
|
protected |
Definition at line 192 of file Pane.h.
Referenced by wheelEvent().
|
protected |
Definition at line 200 of file Pane.h.
Referenced by dragTopLayer(), mouseDoubleClickEvent(), mouseMoveEvent(), and mousePressEvent().
|
protected |
Definition at line 210 of file Pane.h.
Referenced by updateHeadsUpDisplay().
|
protected |
Definition at line 211 of file Pane.h.
Referenced by editVerticalPannerExtents(), mouseEnteredWidget(), resetVerticalPannerExtents(), updateHeadsUpDisplay(), updateVerticalPanner(), verticalPannerContextMenuRequested(), and wheelVertical().
|
protected |
Definition at line 212 of file Pane.h.
Referenced by horizontalThumbwheelMoved(), mouseEnteredWidget(), updateHeadsUpDisplay(), and viewZoomLevelChanged().
|
protected |
Definition at line 213 of file Pane.h.
Referenced by mouseEnteredWidget(), propertyContainerSelected(), resetVerticalPannerExtents(), updateHeadsUpDisplay(), verticalZoomChanged(), and wheelVertical().
|
protected |
Definition at line 214 of file Pane.h.
Referenced by mouseEnteredWidget(), and updateHeadsUpDisplay().
|
protected |
Definition at line 215 of file Pane.h.
Referenced by verticalPannerContextMenuRequested(), and ~Pane().
|
protected |
Definition at line 217 of file Pane.h.
Referenced by enterEvent(), leaveEvent(), and paintEvent().
|
protected |
Definition at line 219 of file Pane.h.
Referenced by dragExtendSelection(), mouseDoubleClickEvent(), mouseMoveEvent(), playbackScheduleTimerElapsed(), and schedulePlaybackFrameMove().
|
protected |
Definition at line 220 of file Pane.h.
Referenced by playbackScheduleTimerElapsed(), and schedulePlaybackFrameMove().
|
staticprotected |
!! ugh
!! for HUD – pull out into a separate class
Definition at line 222 of file Pane.h.
Referenced by mouseReleaseEvent(), and toolModeChanged().
|
staticprotected |
Definition at line 223 of file Pane.h.
Referenced by mouseMoveEvent(), and toolModeChanged().
|
protectedinherited |
Definition at line 481 of file View.h.
Referenced by View::getId().
|
protectedinherited |
Definition at line 540 of file View.h.
Referenced by dragTopLayer(), drawCentreLine(), edgeScrollMaybe(), View::getCentreFrame(), View::getFrameForX(), View::getXForFrame(), Overview::mouseMoveEvent(), mousePressEvent(), Overview::paintEvent(), View::paintEvent(), View::render(), View::scroll(), View::setCentreFrame(), View::toXml(), AlignmentView::viewCentreFrameChanged(), and wheelHorizontalFine().
|
protectedinherited |
Definition at line 541 of file View.h.
Referenced by View::getFrameForX(), View::getXForFrame(), View::getZoomLevel(), Overview::modelChangedWithin(), Overview::mouseMoveEvent(), Overview::paintEvent(), View::paintEvent(), View::render(), View::setCentreFrame(), View::setStartFrame(), View::setZoomLevel(), View::toXml(), AlignmentView::viewAboveZoomLevelChanged(), wheelHorizontalFine(), wheelVertical(), and View::zoom().
|
protectedinherited |
Definition at line 542 of file View.h.
Referenced by View::getFollowGlobalPan(), View::getPropertyRangeAndValue(), View::globalCentreFrameChanged(), Overview::Overview(), View::setCentreFrame(), View::setFollowGlobalPan(), View::setViewManager(), and View::toXml().
|
protectedinherited |
Definition at line 543 of file View.h.
Referenced by View::getFollowGlobalZoom(), View::getPropertyRangeAndValue(), Overview::Overview(), Overview::paintEvent(), View::setFollowGlobalZoom(), View::setViewManager(), View::setZoomLevel(), View::toXml(), viewZoomLevelChanged(), and View::viewZoomLevelChanged().
|
protectedinherited |
Definition at line 544 of file View.h.
Referenced by View::drawPlayPointer(), edgeScrollMaybe(), View::getPlaybackFollow(), View::getPropertyRangeAndValue(), Overview::mouseMoveEvent(), mousePressEvent(), View::movePlayPointer(), View::setCentreFrame(), View::setPlaybackFollow(), View::setViewManager(), and View::toXml().
|
protectedinherited |
Definition at line 545 of file View.h.
Referenced by View::movePlayPointer().
|
protectedinherited |
Definition at line 546 of file View.h.
Referenced by View::drawPlayPointer(), Overview::modelReplaced(), View::movePlayPointer(), and Overview::viewManagerPlaybackFrameChanged().
|
protectedinherited |
Definition at line 548 of file View.h.
Referenced by View::checkAlignmentProgress(), and View::checkProgress().
|
protectedinherited |
Definition at line 550 of file View.h.
Referenced by View::paintEvent(), and View::~View().
|
protectedinherited |
Definition at line 551 of file View.h.
Referenced by View::paintEvent(), and View::~View().
|
protectedinherited |
Definition at line 552 of file View.h.
Referenced by View::addLayer(), View::layerParametersChanged(), View::modelChanged(), View::modelChangedWithin(), View::modelReplaced(), View::overlayModeChanged(), View::paintEvent(), View::propertyContainerSelected(), View::removeLayer(), and View::selectionChanged().
|
protectedinherited |
Definition at line 553 of file View.h.
Referenced by View::paintEvent().
|
protectedinherited |
Definition at line 554 of file View.h.
Referenced by View::paintEvent().
|
protectedinherited |
Definition at line 555 of file View.h.
Referenced by View::selectionChanged().
|
protectedinherited |
Definition at line 557 of file View.h.
Referenced by View::removeLayer(), and View::~View().
|
protectedinherited |
Definition at line 559 of file View.h.
Referenced by View::addLayer(), View::areLayerColoursSignificant(), View::areLayersScrollable(), drawCentreLine(), drawLayerNames(), drawVerticalScale(), View::getAligningAndReferenceModels(), View::getInteractionLayer(), View::getLayer(), View::getLayerCount(), View::getModelsEndFrame(), View::getModelsSampleRate(), View::getModelsStartFrame(), View::getNonScrollableFrontLayers(), View::getScaleProvidingLayerForUnit(), View::getScrollableBackLayers(), View::getSelectedLayer(), View::getTextLabelYCoord(), getTopFlexiNoteLayer(), View::getTopLayer(), View::getVisibleExtentsForAnyUnit(), View::getVisibleExtentsForUnit(), View::getZoomConstraintLevel(), View::hasLightBackground(), View::hasTopLayerTimeXAxis(), Overview::modelChangedWithin(), paintEvent(), View::paintEvent(), View::propertyContainerSelected(), View::removeLayer(), View::render(), View::waitForLayersToBeReady(), and zoomToRegion().
|
protectedinherited |
Definition at line 560 of file View.h.
Referenced by View::addLayer(), View::getFixedOrderLayer(), View::getPropertyContainer(), View::getPropertyContainerCount(), View::removeLayer(), and View::toXml().
|
protectedinherited |
Definition at line 561 of file View.h.
Referenced by View::getSelectedLayer(), and View::propertyContainerSelected().
|
protectedinherited |
Definition at line 563 of file View.h.
Referenced by View::paintEvent(), and View::setUseAligningProxy().
|
protectedinherited |
Definition at line 565 of file View.h.
Referenced by View::checkProgress().
|
mutableprotectedinherited |
Definition at line 568 of file View.h.
Referenced by View::getScrollableBackLayers().
|
mutableprotectedinherited |
Definition at line 569 of file View.h.
Referenced by View::getNonScrollableFrontLayers().
|
protectedinherited |
Definition at line 578 of file View.h.
Referenced by View::addLayer(), View::cancelClicked(), View::checkProgress(), View::getProgressBarWidth(), View::progressCheckStalledTimerElapsed(), and View::removeLayer().
|
protectedinherited |
Definition at line 584 of file View.h.
Referenced by View::checkAlignmentProgress(), View::checkProgress(), and View::getProgressBarWidth().
|
protectedinherited |
Definition at line 586 of file View.h.
Referenced by View::alignFromReference(), View::alignToReference(), dragExtendSelection(), dragTopLayer(), drawCentreLine(), drawDurationAndRate(), drawLayerNames(), View::drawPlayPointer(), View::drawSelections(), drawVerticalScale(), edgeScrollMaybe(), editSelectionEnd(), editSelectionStart(), editVerticalPannerExtents(), View::getAlignedPlaybackFrame(), View::getAligningAndReferenceModels(), AlignmentView::getDefaultKeyFrames(), getRenderedPartImageSize(), getSelectionAt(), View::getViewManager(), View::hasLightBackground(), mouseDoubleClickEvent(), mouseMoveEvent(), mousePressEvent(), mouseReleaseEvent(), View::movePlayPointer(), AlignmentView::paintEvent(), paintEvent(), playbackScheduleTimerElapsed(), render(), renderPartToNewImage(), View::setViewManager(), shouldIlluminateLocalFeatures(), shouldIlluminateLocalSelection(), View::shouldShowFeatureLabels(), toolModeChanged(), updateContextHelp(), updateDragMode(), updateHeadsUpDisplay(), updateVerticalPanner(), View::View(), View::viewManagerPlaybackFrameChanged(), and viewZoomLevelChanged().
|
protectedinherited |
Definition at line 587 of file View.h.
Referenced by View::getPropertyContainer(), View::propertyContainerSelected(), View::setFollowGlobalPan(), View::setFollowGlobalZoom(), View::setPlaybackFollow(), View::View(), and View::~View().
The documentation for this class was generated from the following files:
Generated by
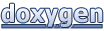