svgui
1.9
|
Panner.cpp
Go to the documentation of this file.
void mouseDoubleClickEvent(QMouseEvent *e) override
Definition: Panner.cpp:105
void getRectExtents(float &x0, float &y0, float &width, float &height)
Definition: Panner.cpp:217
void setScrollUnit(float unit)
Set the amount the scroll() function or mouse wheel movement makes the panner rectangle move by...
Definition: Panner.cpp:59
static int scalePixelSize(int pixels)
Take a "design pixel" size and scale it for the actual display.
Definition: WidgetScale.h:31
void mouseEntered()
void setRectWidth(float width)
Set the width of the panned rectangle as a fraction (0 -> 1) of that of the whole panner widget...
Definition: Panner.cpp:248
void setRectHeight(float height)
Set the height of the panned rectangle as a fraction (0 -> 1) of that of the whole panner widget...
Definition: Panner.cpp:257
void mouseLeft()
void setRectCentreY(float y)
Set the location of the centre of the panned rectangle on the y axis, as a proportion (0 -> 1) of the...
Definition: Panner.cpp:276
void rectExtentsChanged(float, float, float, float)
Emitted when the panned rectangle is dragged or otherwise moved.
void doubleClicked()
Emitted when the panner is double-clicked (for the "customer" code to pop up a value editing dialog...
void setRectCentreX(float x)
Set the location of the centre of the panned rectangle on the x axis, as a proportion (0 -> 1) of the...
Definition: Panner.cpp:266
void setRectExtents(float x0, float y0, float width, float height)
Set the extents of the panned rectangle within the overall panner widget.
Definition: Panner.cpp:226
void rectCentreMoved(float, float)
Emitted when the rectangle is dragged or otherwise moved (as well as extentsChanged).
Generated by
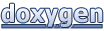