svgui
1.9
|
#include <Panner.h>
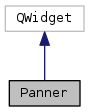
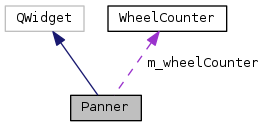
Public Slots | |
void | setRectExtents (float x0, float y0, float width, float height) |
Set the extents of the panned rectangle within the overall panner widget. More... | |
void | setRectWidth (float width) |
Set the width of the panned rectangle as a fraction (0 -> 1) of that of the whole panner widget. More... | |
void | setRectHeight (float height) |
Set the height of the panned rectangle as a fraction (0 -> 1) of that of the whole panner widget. More... | |
void | setRectCentreX (float x) |
Set the location of the centre of the panned rectangle on the x axis, as a proportion (0 -> 1) of the width of the whole panner widget. More... | |
void | setRectCentreY (float y) |
Set the location of the centre of the panned rectangle on the y axis, as a proportion (0 -> 1) of the height of the whole panner widget. More... | |
void | scroll (bool up) |
Move up (if up is true) or down a bit. More... | |
void | scroll (bool up, int n) |
Move up (if up is true) or down a bit. More... | |
void | resetToDefault () |
Signals | |
void | rectExtentsChanged (float, float, float, float) |
Emitted when the panned rectangle is dragged or otherwise moved. More... | |
void | rectCentreMoved (float, float) |
Emitted when the rectangle is dragged or otherwise moved (as well as extentsChanged). More... | |
void | doubleClicked () |
Emitted when the panner is double-clicked (for the "customer" code to pop up a value editing dialog, for example). More... | |
void | mouseEntered () |
void | mouseLeft () |
Public Member Functions | |
Panner (QWidget *parent=0) | |
virtual | ~Panner () |
void | setDefaultRectCentre (float, float) |
void | setThumbColour (QColor colour) |
void | setAlpha (int backgroundAlpha, int thumbAlpha) |
void | setScrollUnit (float unit) |
Set the amount the scroll() function or mouse wheel movement makes the panner rectangle move by. More... | |
void | getRectExtents (float &x0, float &y0, float &width, float &height) |
QSize | sizeHint () const override |
Protected Member Functions | |
void | mousePressEvent (QMouseEvent *e) override |
void | mouseDoubleClickEvent (QMouseEvent *e) override |
void | mouseMoveEvent (QMouseEvent *e) override |
void | mouseReleaseEvent (QMouseEvent *e) override |
void | wheelEvent (QWheelEvent *e) override |
void | paintEvent (QPaintEvent *e) override |
void | enterEvent (QEvent *) override |
void | leaveEvent (QEvent *) override |
void | normalise () |
void | emitAndUpdate () |
float | centreX () const |
float | centreY () const |
Protected Attributes | |
float | m_rectX |
float | m_rectY |
float | m_rectWidth |
float | m_rectHeight |
float | m_scrollUnit |
float | m_defaultCentreX |
float | m_defaultCentreY |
bool | m_defaultsSet |
QColor | m_thumbColour |
int | m_backgroundAlpha |
int | m_thumbAlpha |
bool | m_clicked |
QPoint | m_clickPos |
float | m_dragStartX |
float | m_dragStartY |
WheelCounter | m_wheelCounter |
Detailed Description
Constructor & Destructor Documentation
Panner::Panner | ( | QWidget * | parent = 0 | ) |
Definition at line 28 of file Panner.cpp.
|
virtual |
Definition at line 47 of file Panner.cpp.
Member Function Documentation
void Panner::setDefaultRectCentre | ( | float | cx, |
float | cy | ||
) |
Definition at line 292 of file Panner.cpp.
References m_defaultCentreX, m_defaultCentreY, and m_defaultsSet.
void Panner::setThumbColour | ( | QColor | colour | ) |
void Panner::setAlpha | ( | int | backgroundAlpha, |
int | thumbAlpha | ||
) |
Definition at line 52 of file Panner.cpp.
References m_backgroundAlpha, and m_thumbAlpha.
Referenced by Pane::updateHeadsUpDisplay().
void Panner::setScrollUnit | ( | float | unit | ) |
Set the amount the scroll() function or mouse wheel movement makes the panner rectangle move by.
The default value of 0 means to select a value automatically based on the dimensions of the panner rectangle.
Definition at line 59 of file Panner.cpp.
References m_scrollUnit.
void Panner::getRectExtents | ( | float & | x0, |
float & | y0, | ||
float & | width, | ||
float & | height | ||
) |
Definition at line 217 of file Panner.cpp.
References m_rectHeight, m_rectWidth, m_rectX, and m_rectY.
|
override |
Definition at line 286 of file Panner.cpp.
|
signal |
Emitted when the panned rectangle is dragged or otherwise moved.
Arguments are x0, y0, width and height of the rectangle in the range 0 -> 1 as proportions of the width and height of the whole widget.
Referenced by emitAndUpdate(), and mouseMoveEvent().
|
signal |
Emitted when the rectangle is dragged or otherwise moved (as well as extentsChanged).
Arguments are the centre coordinates of the rectangle in the range 0 -> 1 as proportions of the width and height of the whole widget.
Referenced by emitAndUpdate(), and mouseMoveEvent().
|
signal |
Emitted when the panner is double-clicked (for the "customer" code to pop up a value editing dialog, for example).
Referenced by mouseDoubleClickEvent().
|
signal |
Referenced by enterEvent().
|
signal |
Referenced by leaveEvent().
|
slot |
Set the extents of the panned rectangle within the overall panner widget.
Coordinates are in the range 0 -> 1 in both axes, with 0 at the top in the y axis.
Definition at line 226 of file Panner.cpp.
References emitAndUpdate(), m_rectHeight, m_rectWidth, m_rectX, m_rectY, and normalise().
Referenced by Pane::updateVerticalPanner().
|
slot |
Set the width of the panned rectangle as a fraction (0 -> 1) of that of the whole panner widget.
Definition at line 248 of file Panner.cpp.
References emitAndUpdate(), m_rectWidth, and normalise().
|
slot |
Set the height of the panned rectangle as a fraction (0 -> 1) of that of the whole panner widget.
Definition at line 257 of file Panner.cpp.
References emitAndUpdate(), m_rectHeight, and normalise().
|
slot |
Set the location of the centre of the panned rectangle on the x axis, as a proportion (0 -> 1) of the width of the whole panner widget.
Definition at line 266 of file Panner.cpp.
References emitAndUpdate(), m_rectWidth, m_rectX, and normalise().
|
slot |
Set the location of the centre of the panned rectangle on the y axis, as a proportion (0 -> 1) of the height of the whole panner widget.
Definition at line 276 of file Panner.cpp.
References emitAndUpdate(), m_rectHeight, m_rectY, and normalise().
|
slot |
Move up (if up is true) or down a bit.
This is basically the same action as rolling the mouse wheel one notch.
Definition at line 65 of file Panner.cpp.
Referenced by wheelEvent(), and Pane::wheelVertical().
|
slot |
Move up (if up is true) or down a bit.
This is basically the same action as rolling the mouse wheel n notches.
Definition at line 71 of file Panner.cpp.
References emitAndUpdate(), m_rectHeight, m_rectY, m_scrollUnit, and normalise().
|
slot |
Definition at line 300 of file Panner.cpp.
References emitAndUpdate(), m_defaultCentreX, m_defaultCentreY, m_rectHeight, m_rectWidth, m_rectX, m_rectY, and normalise().
Referenced by mousePressEvent(), and Pane::resetVerticalPannerExtents().
|
overrideprotected |
Definition at line 90 of file Panner.cpp.
References m_clicked, m_clickPos, m_dragStartX, m_dragStartY, m_rectX, m_rectY, and resetToDefault().
|
overrideprotected |
Definition at line 105 of file Panner.cpp.
References doubleClicked().
|
overrideprotected |
Definition at line 115 of file Panner.cpp.
References centreX(), centreY(), m_clicked, m_clickPos, m_dragStartX, m_dragStartY, m_rectHeight, m_rectWidth, m_rectX, m_rectY, normalise(), rectCentreMoved(), and rectExtentsChanged().
Referenced by mouseReleaseEvent().
|
overrideprotected |
Definition at line 132 of file Panner.cpp.
References m_clicked, and mouseMoveEvent().
|
overrideprotected |
Definition at line 141 of file Panner.cpp.
References WheelCounter::count(), m_wheelCounter, and scroll().
|
overrideprotected |
Definition at line 160 of file Panner.cpp.
References m_backgroundAlpha, m_rectHeight, m_rectWidth, m_rectX, m_rectY, m_thumbAlpha, m_thumbColour, and WidgetScale::scalePixelSize().
|
overrideprotected |
Definition at line 148 of file Panner.cpp.
References mouseEntered().
|
overrideprotected |
Definition at line 154 of file Panner.cpp.
References mouseLeft().
|
protected |
Definition at line 192 of file Panner.cpp.
References centreX(), centreY(), m_defaultCentreX, m_defaultCentreY, m_defaultsSet, m_rectHeight, m_rectWidth, m_rectX, and m_rectY.
Referenced by mouseMoveEvent(), resetToDefault(), scroll(), setRectCentreX(), setRectCentreY(), setRectExtents(), setRectHeight(), and setRectWidth().
|
protected |
Definition at line 209 of file Panner.cpp.
References centreX(), centreY(), m_rectHeight, m_rectWidth, m_rectX, m_rectY, rectCentreMoved(), and rectExtentsChanged().
Referenced by resetToDefault(), scroll(), setRectCentreX(), setRectCentreY(), setRectExtents(), setRectHeight(), and setRectWidth().
|
inlineprotected |
Definition at line 149 of file Panner.h.
Referenced by emitAndUpdate(), mouseMoveEvent(), and normalise().
|
inlineprotected |
Definition at line 150 of file Panner.h.
Referenced by emitAndUpdate(), mouseMoveEvent(), and normalise().
Member Data Documentation
|
protected |
Definition at line 135 of file Panner.h.
Referenced by emitAndUpdate(), getRectExtents(), mouseMoveEvent(), mousePressEvent(), normalise(), paintEvent(), resetToDefault(), setRectCentreX(), and setRectExtents().
|
protected |
Definition at line 136 of file Panner.h.
Referenced by emitAndUpdate(), getRectExtents(), mouseMoveEvent(), mousePressEvent(), normalise(), paintEvent(), resetToDefault(), scroll(), setRectCentreY(), and setRectExtents().
|
protected |
Definition at line 137 of file Panner.h.
Referenced by emitAndUpdate(), getRectExtents(), mouseMoveEvent(), normalise(), paintEvent(), resetToDefault(), setRectCentreX(), setRectExtents(), and setRectWidth().
|
protected |
Definition at line 138 of file Panner.h.
Referenced by emitAndUpdate(), getRectExtents(), mouseMoveEvent(), normalise(), paintEvent(), resetToDefault(), scroll(), setRectCentreY(), setRectExtents(), and setRectHeight().
|
protected |
Definition at line 139 of file Panner.h.
Referenced by scroll(), and setScrollUnit().
|
protected |
Definition at line 141 of file Panner.h.
Referenced by normalise(), resetToDefault(), and setDefaultRectCentre().
|
protected |
Definition at line 142 of file Panner.h.
Referenced by normalise(), resetToDefault(), and setDefaultRectCentre().
|
protected |
Definition at line 143 of file Panner.h.
Referenced by normalise(), and setDefaultRectCentre().
|
protected |
Definition at line 145 of file Panner.h.
Referenced by paintEvent().
|
protected |
Definition at line 146 of file Panner.h.
Referenced by paintEvent(), and setAlpha().
|
protected |
Definition at line 147 of file Panner.h.
Referenced by paintEvent(), and setAlpha().
|
protected |
Definition at line 152 of file Panner.h.
Referenced by mouseMoveEvent(), mousePressEvent(), and mouseReleaseEvent().
|
protected |
Definition at line 153 of file Panner.h.
Referenced by mouseMoveEvent(), and mousePressEvent().
|
protected |
Definition at line 154 of file Panner.h.
Referenced by mouseMoveEvent(), and mousePressEvent().
|
protected |
Definition at line 155 of file Panner.h.
Referenced by mouseMoveEvent(), and mousePressEvent().
|
protected |
Definition at line 157 of file Panner.h.
Referenced by wheelEvent().
The documentation for this class was generated from the following files:
Generated by
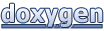