svgui
1.9
|
ViewProxy.h
Go to the documentation of this file.
double getYForFrequency(double frequency, double minFreq, double maxFreq, bool logarithmic) const override
Return the (maybe fractional) pixel y-coordinate corresponding to a given frequency, if the frequency range is as specified.
Definition: ViewProxy.h:87
sv_frame_t alignToReference(sv_frame_t frame) const
Definition: ViewProxy.h:216
void drawMeasurementRect(QPainter &p, const Layer *layer, QRect rect, bool focus) const override
Definition: ViewProxy.h:158
bool getVisibleExtentsForUnit(QString unit, double &min, double &max, bool &log) const override
Return the visible vertical extents for the given unit, if any.
Definition: View.cpp:197
double getFrequencyForY(double y, double minFreq, double maxFreq, bool logarithmic) const override
Return the closest frequency to the given pixel y-coordinate, if the frequency range is as specified...
Definition: View.cpp:700
int getXForFrame(sv_frame_t frame) const override
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative)...
Definition: ViewProxy.h:64
sv_frame_t getFrameForX(int x) const override
Return the closest frame to the given pixel x-coordinate.
Definition: ViewProxy.h:68
sv_frame_t getCentreFrame() const override
Return the centre frame of the visible widget.
Definition: View.h:93
double scaleSize(double size) const override
Scale up a size in pixels for a hi-dpi display without pixel doubling.
Definition: ViewProxy.h:177
ViewProxy(View *view, int scaleFactor, ModelId alignment)
Create a re-aligning ViewProxy for the given view, mapping using the given scale factor.
Definition: ViewProxy.h:49
sv_frame_t alignFromReference(sv_frame_t frame) const
Definition: ViewProxy.h:224
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
bool shouldIlluminateLocalFeatures(const Layer *layer, QPoint &point) const override
Definition: ViewProxy.h:146
bool shouldIlluminateLocalFeatures(const Layer *, QPoint &) const override
Definition: View.h:282
sv_frame_t getEndFrame() const override
Retrieve the last visible sample frame on the widget.
Definition: ViewProxy.h:61
sv_frame_t getFrameForX(int x) const override
Return the closest frame to the given pixel x-coordinate.
Definition: View.cpp:600
sv_frame_t getCentreFrame() const override
Return the centre frame of the visible widget.
Definition: ViewProxy.h:58
int scalePixelSize(int size) const override
Integer version of scaleSize.
Definition: ViewProxy.h:184
int getTextLabelYCoord(const Layer *layer, QPainter &) const override
Return a y-coordinate at which text labels for individual items in a layer may be drawn...
Definition: View.cpp:367
double getFrequencyForY(double y, double minFreq, double maxFreq, bool logarithmic) const override
Return the closest frequency to the given (maybe fractional) pixel y-coordinate, if the frequency ran...
Definition: ViewProxy.h:93
sv_frame_t getStartFrame() const override
Retrieve the first visible sample frame on the widget.
Definition: ViewProxy.h:55
QRect getPaintRect() const override
To be called from a layer, to obtain the extent of the surface that the layer is currently painting t...
Definition: View.cpp:2219
int getViewXForX(int x) const override
Return the closest view x-coordinate corresponding to a given pixel x-coordinate. ...
Definition: ViewProxy.h:78
ViewProxy(View *view, int scaleFactor)
Create a standard ViewProxy for the given view, mapping using the given scale factor.
Definition: ViewProxy.h:30
QRect getPaintRect() const override
To be called from a layer, to obtain the extent of the surface that the layer is currently painting t...
Definition: ViewProxy.h:117
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
The ViewManager manages properties that may need to be synchronised between separate Views...
Definition: ViewManager.h:78
bool getVisibleExtentsForUnit(QString unit, double &min, double &max, bool &log) const override
Return the visible vertical extents for the given unit, if any.
Definition: ViewProxy.h:101
sv_frame_t getStartFrame() const override
Retrieve the first visible sample frame on the widget.
Definition: View.cpp:445
sv_frame_t getEndFrame() const override
Retrieve the last visible sample frame on the widget.
Definition: View.cpp:451
bool shouldShowFeatureLabels() const override
Definition: ViewProxy.h:154
int getXForViewX(int viewx) const override
Return the closest pixel x-coordinate corresponding to a given view x-coordinate. ...
Definition: ViewProxy.h:75
double getYForFrequency(double frequency, double minFreq, double maxFreq, bool logarithmic) const override
Return the pixel y-coordinate corresponding to a given frequency, if the frequency range is as specif...
Definition: View.cpp:666
sv_frame_t getModelsStartFrame() const override
Definition: ViewProxy.h:81
int getTextLabelYCoord(const Layer *layer, QPainter &paint) const override
Return a y-coordinate at which text labels for individual items in a layer may be drawn...
Definition: ViewProxy.h:98
void drawMeasurementRect(QPainter &p, const Layer *, QRect rect, bool focus) const override
Definition: View.cpp:2767
int getXForFrame(sv_frame_t frame) const override
Return the pixel x-coordinate corresponding to a given sample frame.
Definition: View.cpp:527
Definition: ViewProxy.h:22
double scalePenWidth(double width) const override
Scale up pen width for a hi-dpi display without pixel doubling.
Definition: ViewProxy.h:193
Generated by
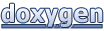