svgui
1.9
|
LayerTree.cpp
Go to the documentation of this file.
virtual bool isLayerDormant(const LayerGeometryProvider *v) const
Return whether the layer is dormant (i.e.
Definition: Layer.cpp:144
Definition: PaneStack.h:40
QModelIndex index(int row, int column, const QModelIndex &parent=QModelIndex()) const override
Definition: LayerTree.cpp:522
QVariant data(const QModelIndex &index, int role) const override
Definition: LayerTree.cpp:165
void propertyContainerRemoved(PropertyContainer *)
Definition: LayerTree.cpp:332
Qt::ItemFlags flags(const QModelIndex &index) const override
Definition: LayerTree.cpp:213
virtual int getLayerCount() const
Return the number of layers, regardless of whether visible or dormant, i.e.
Definition: View.h:197
ModelMetadataModel(PaneStack *stack, bool waveModelsOnly, QObject *parent=0)
Definition: LayerTree.cpp:30
Qt::ItemFlags flags(const QModelIndex &index) const override
Definition: LayerTree.cpp:491
QModelIndex parent(const QModelIndex &index) const override
Definition: LayerTree.cpp:246
void propertyContainerRemoved(PropertyContainer *)
Definition: LayerTree.cpp:143
LayerTreeModel(PaneStack *stack, QObject *parent=0)
Definition: LayerTree.cpp:266
void propertyContainerSelected(PropertyContainer *)
Definition: LayerTree.cpp:338
void propertyContainerPropertyChanged(PropertyContainer *)
Definition: LayerTree.cpp:155
void propertyContainerAdded(PropertyContainer *)
Definition: LayerTree.cpp:136
std::shared_ptr< PlayParameters > getPlayParameters() override
Return the play parameters for this layer, if any.
Definition: Layer.cpp:129
void propertyContainerPropertyChanged(PropertyContainer *)
Definition: LayerTree.cpp:350
QModelIndex index(int row, int column, const QModelIndex &parent=QModelIndex()) const override
Definition: LayerTree.cpp:235
void propertyContainerAdded(PropertyContainer *)
Definition: LayerTree.cpp:326
int columnCount(const QModelIndex &parent=QModelIndex()) const override
Definition: LayerTree.cpp:259
int rowCount(const QModelIndex &parent=QModelIndex()) const override
Definition: LayerTree.cpp:566
void propertyContainerSelected(PropertyContainer *)
Definition: LayerTree.cpp:150
virtual Layer * getLayer(int n)
Return the nth layer, counted in stacking order.
Definition: View.h:205
QModelIndex parent(const QModelIndex &index) const override
Definition: LayerTree.cpp:547
void playParametersAudibilityChanged(bool)
Definition: LayerTree.cpp:160
QVariant headerData(int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const override
Definition: LayerTree.cpp:507
void showLayer(LayerGeometryProvider *, bool show)
Change the visibility status (dormancy) of the layer in the given view.
Definition: Layer.cpp:153
QVariant headerData(int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const override
Definition: LayerTree.cpp:220
void playParametersAudibilityChanged(bool)
Definition: LayerTree.cpp:367
virtual ModelId getModel() const =0
Return the ID of the model represented in this layer.
bool setData(const QModelIndex &index, const QVariant &value, int role) override
Definition: LayerTree.cpp:207
QString getPropertyContainerIconName() const override
Definition: Layer.cpp:80
bool setData(const QModelIndex &index, const QVariant &value, int role) override
Definition: LayerTree.cpp:457
int rowCount(const QModelIndex &parent=QModelIndex()) const override
Definition: LayerTree.cpp:252
int columnCount(const QModelIndex &parent=QModelIndex()) const override
Definition: LayerTree.cpp:582
QVariant data(const QModelIndex &index, int role) const override
Definition: LayerTree.cpp:395
Generated by
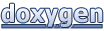