svgui
1.9
|
#include <PaneStack.h>
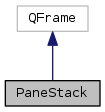
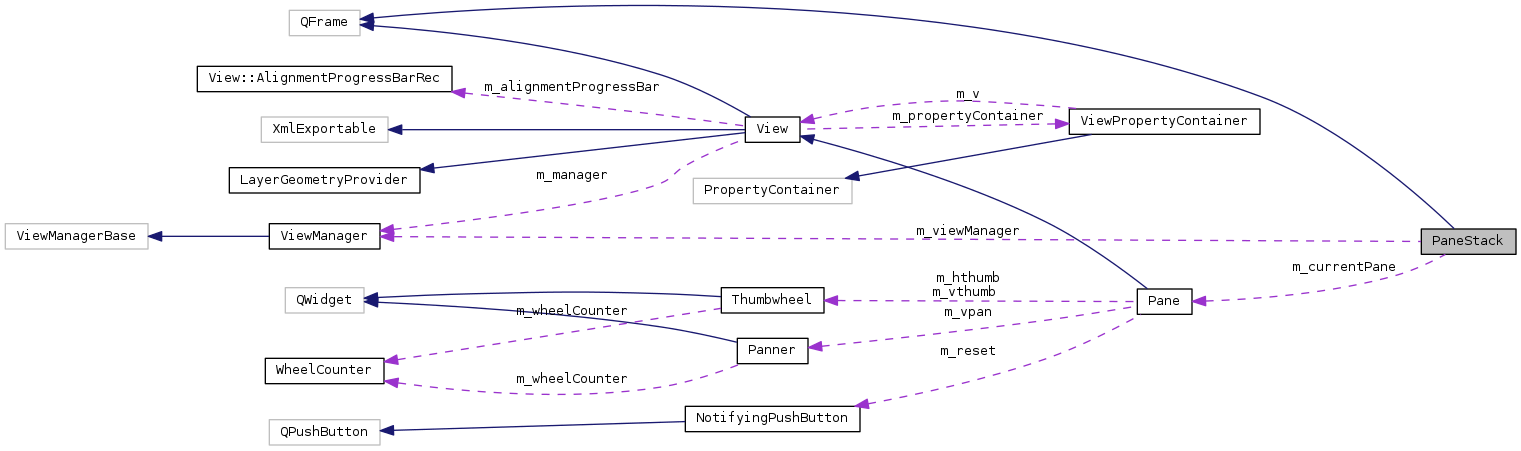
Classes | |
struct | PaneRec |
Public Types | |
enum | Option { Option::Default = 0x0, Option::NoUserResize = 0x1, Option::NoPropertyStacks = 0x2, Option::NoPaneAccessories = 0x4, Option::NoCloseOnFirstPane = 0x8, Option::ShowAlignmentViews = 0x10 } |
These options are for things that must be set on construction, and can't be changed afterwards. More... | |
enum | LayoutStyle { HiddenPropertyStacksLayout = 0, SinglePropertyStackLayout = 1, PropertyStackPerPaneLayout = 2 } |
Runtime-switchable layout style for property stacks. More... | |
typedef int | Options |
Public Slots | |
void | propertyContainerAdded (PropertyContainer *) |
void | propertyContainerRemoved (PropertyContainer *) |
void | propertyContainerSelected (View *client, PropertyContainer *) |
void | propertyContainerContextMenuRequested (View *, PropertyContainer *, QPoint) |
void | viewSelected (View *v) |
void | paneInteractedWith () |
void | rightButtonMenuRequested (QPoint) |
void | paneDropAccepted (QStringList) |
void | paneDropAccepted (QString) |
void | paneDeleteButtonClicked () |
void | indicatorClicked () |
Signals | |
void | currentPaneChanged (Pane *pane) |
void | currentLayerChanged (Pane *pane, Layer *layer) |
void | paneRightButtonMenuRequested (Pane *pane, QPoint position) |
void | panePropertiesRightButtonMenuRequested (Pane *, QPoint) |
void | layerPropertiesRightButtonMenuRequested (Pane *, Layer *, QPoint) |
void | propertyStacksResized (int width) |
void | propertyStacksResized () |
void | contextHelpChanged (const QString &) |
void | paneAdded (Pane *pane) |
void | paneAdded () |
void | paneHidden (Pane *pane) |
void | paneHidden () |
void | paneAboutToBeDeleted (Pane *pane) |
void | paneDeleted () |
void | dropAccepted (Pane *pane, QStringList uriList) |
void | dropAccepted (Pane *pane, QString text) |
void | paneDeleteButtonClicked (Pane *pane) |
void | doubleClickSelectInvoked (sv_frame_t frame) |
Public Member Functions | |
PaneStack (QWidget *parent, ViewManager *viewManager, Options options=0) | |
Pane * | addPane () |
void | deletePane (Pane *pane) |
int | getPaneCount () const |
Pane * | getPane (int n) |
int | getPaneIndex (Pane *pane) |
void | hidePane (Pane *pane) |
void | showPane (Pane *pane) |
int | getHiddenPaneCount () const |
Pane * | getHiddenPane (int n) |
void | setCurrentPane (Pane *pane) |
void | setCurrentLayer (Pane *pane, Layer *layer) |
Pane * | getCurrentPane () |
LayoutStyle | getLayoutStyle () const |
void | setLayoutStyle (LayoutStyle style) |
void | setPropertyStackMinWidth (int mw) |
void | sizePanesEqually () |
Protected Member Functions | |
void | sizePropertyStacks () |
void | showOrHidePaneAccessories () |
void | unlinkAlignmentViews () |
void | relinkAlignmentViews () |
void | resizeEvent (QResizeEvent *) override |
void | adjustAlignmentViewHeights (int forMyHeight) |
Protected Attributes | |
Pane * | m_currentPane |
std::vector< PaneRec > | m_panes |
std::vector< PaneRec > | m_hiddenPanes |
int | m_options |
QSplitter * | m_splitter |
QWidget * | m_autoResizeStack |
QVBoxLayout * | m_autoResizeLayout |
QStackedWidget * | m_propertyStackStack |
ViewManager * | m_viewManager |
int | m_propertyStackMinWidth |
LayoutStyle | m_layoutStyle |
Detailed Description
Definition at line 40 of file PaneStack.h.
Member Typedef Documentation
typedef int PaneStack::Options |
Definition at line 55 of file PaneStack.h.
Member Enumeration Documentation
|
strong |
These options are for things that must be set on construction, and can't be changed afterwards.
Enumerator | |
---|---|
Default | |
NoUserResize | |
NoPropertyStacks | |
NoPaneAccessories | |
NoCloseOnFirstPane | |
ShowAlignmentViews |
Definition at line 47 of file PaneStack.h.
Runtime-switchable layout style for property stacks.
Enumerator | |
---|---|
HiddenPropertyStacksLayout | |
SinglePropertyStackLayout | |
PropertyStackPerPaneLayout |
Definition at line 79 of file PaneStack.h.
Constructor & Destructor Documentation
PaneStack::PaneStack | ( | QWidget * | parent, |
ViewManager * | viewManager, | ||
Options | options = 0 |
||
) |
Definition at line 41 of file PaneStack.cpp.
References HiddenPropertyStacksLayout, m_autoResizeLayout, m_autoResizeStack, m_layoutStyle, m_options, m_propertyStackStack, m_splitter, NoPropertyStacks, and NoUserResize.
Member Function Documentation
Pane * PaneStack::addPane | ( | ) |
Definition at line 88 of file PaneStack.cpp.
References PaneStack::PaneRec::alignmentView, contextHelpChanged(), PaneStack::PaneRec::currentIndicator, doubleClickSelectInvoked(), dropAccepted(), PaneStack::PaneRec::frame, indicatorClicked(), PaneStack::PaneRec::layout, m_autoResizeLayout, m_currentPane, m_layoutStyle, m_options, m_panes, m_propertyStackStack, m_splitter, m_viewManager, NoCloseOnFirstPane, NoPaneAccessories, NoPropertyStacks, NoUserResize, PaneStack::PaneRec::pane, paneAdded(), paneDeleteButtonClicked(), paneDropAccepted(), paneInteractedWith(), propertyContainerAdded(), propertyContainerContextMenuRequested(), propertyContainerRemoved(), propertyContainerSelected(), PaneStack::PaneRec::propertyStack, PropertyStackPerPaneLayout, relinkAlignmentViews(), rightButtonMenuRequested(), ViewManager::scalePixelSize(), setCurrentPane(), View::setViewManager(), ShowAlignmentViews, showOrHidePaneAccessories(), viewSelected(), and PaneStack::PaneRec::xButton.
void PaneStack::deletePane | ( | Pane * | pane | ) |
Definition at line 362 of file PaneStack.cpp.
References m_currentPane, m_hiddenPanes, m_panes, paneAboutToBeDeleted(), paneDeleted(), relinkAlignmentViews(), setCurrentPane(), showOrHidePaneAccessories(), and unlinkAlignmentViews().
int PaneStack::getPaneCount | ( | ) | const |
Definition at line 455 of file PaneStack.cpp.
References m_panes.
Referenced by getPaneIndex(), LayerTreeModel::index(), LayerTreeModel::LayerTreeModel(), ModelMetadataModel::ModelMetadataModel(), LayerTreeModel::playParametersAudibilityChanged(), LayerTreeModel::propertyContainerPropertyChanged(), ModelMetadataModel::rebuildModelSet(), LayerTreeModel::rowCount(), and showOrHidePaneAccessories().
Pane * PaneStack::getPane | ( | int | n | ) |
Definition at line 335 of file PaneStack.cpp.
References m_panes.
Referenced by getPaneIndex(), LayerTreeModel::index(), LayerTreeModel::LayerTreeModel(), ModelMetadataModel::ModelMetadataModel(), LayerTreeModel::playParametersAudibilityChanged(), LayerTreeModel::propertyContainerPropertyChanged(), ModelMetadataModel::rebuildModelSet(), and LayerTreeModel::rowCount().
int PaneStack::getPaneIndex | ( | Pane * | pane | ) |
Definition at line 345 of file PaneStack.cpp.
References getPane(), and getPaneCount().
Referenced by LayerTreeModel::parent().
void PaneStack::hidePane | ( | Pane * | pane | ) |
Definition at line 467 of file PaneStack.cpp.
References m_currentPane, m_hiddenPanes, m_panes, paneHidden(), relinkAlignmentViews(), setCurrentPane(), and showOrHidePaneAccessories().
void PaneStack::showPane | ( | Pane * | pane | ) |
!! update current pane
Definition at line 501 of file PaneStack.cpp.
References m_hiddenPanes, m_panes, relinkAlignmentViews(), and showOrHidePaneAccessories().
int PaneStack::getHiddenPaneCount | ( | ) | const |
Definition at line 461 of file PaneStack.cpp.
References m_hiddenPanes.
Pane * PaneStack::getHiddenPane | ( | int | n | ) |
Definition at line 356 of file PaneStack.cpp.
References m_hiddenPanes.
void PaneStack::setCurrentPane | ( | Pane * | pane | ) |
Definition at line 526 of file PaneStack.cpp.
References currentPaneChanged(), m_currentPane, m_layoutStyle, m_panes, m_propertyStackStack, and PropertyStackPerPaneLayout.
Referenced by addPane(), deletePane(), hidePane(), indicatorClicked(), paneInteractedWith(), propertyContainerSelected(), setCurrentLayer(), and viewSelected().
Definition at line 566 of file PaneStack.cpp.
References PropertyStack::containsContainer(), currentLayerChanged(), PropertyStack::getContainerIndex(), View::getPropertyContainer(), m_currentPane, m_panes, and setCurrentPane().
Pane * PaneStack::getCurrentPane | ( | ) |
Definition at line 598 of file PaneStack.cpp.
References m_currentPane.
|
inline |
Definition at line 85 of file PaneStack.h.
References contextHelpChanged(), currentLayerChanged(), currentPaneChanged(), doubleClickSelectInvoked(), dropAccepted(), indicatorClicked(), layerPropertiesRightButtonMenuRequested(), m_layoutStyle, paneAboutToBeDeleted(), paneAdded(), paneDeleteButtonClicked(), paneDeleted(), paneDropAccepted(), paneHidden(), paneInteractedWith(), panePropertiesRightButtonMenuRequested(), paneRightButtonMenuRequested(), propertyContainerAdded(), propertyContainerContextMenuRequested(), propertyContainerRemoved(), propertyContainerSelected(), propertyStacksResized(), rightButtonMenuRequested(), setLayoutStyle(), setPropertyStackMinWidth(), sizePanesEqually(), and viewSelected().
void PaneStack::setLayoutStyle | ( | LayoutStyle | style | ) |
Definition at line 296 of file PaneStack.cpp.
References HiddenPropertyStacksLayout, m_layoutStyle, m_options, m_panes, m_propertyStackStack, NoPropertyStacks, PropertyStackPerPaneLayout, and SinglePropertyStackLayout.
Referenced by getLayoutStyle().
void PaneStack::setPropertyStackMinWidth | ( | int | mw | ) |
Definition at line 286 of file PaneStack.cpp.
References m_panes, and m_propertyStackMinWidth.
Referenced by getLayoutStyle().
void PaneStack::sizePanesEqually | ( | ) |
Definition at line 753 of file PaneStack.cpp.
References m_options, m_panes, m_splitter, and NoUserResize.
Referenced by getLayoutStyle().
|
signal |
Referenced by getLayoutStyle(), and setCurrentPane().
Referenced by getLayoutStyle(), propertyContainerSelected(), and setCurrentLayer().
|
signal |
Referenced by getLayoutStyle(), and rightButtonMenuRequested().
|
signal |
Referenced by getLayoutStyle(), and propertyContainerContextMenuRequested().
Referenced by getLayoutStyle(), and propertyContainerContextMenuRequested().
|
signal |
|
signal |
Referenced by getLayoutStyle(), and sizePropertyStacks().
|
signal |
Referenced by addPane(), and getLayoutStyle().
|
signal |
|
signal |
Referenced by addPane(), and getLayoutStyle().
|
signal |
|
signal |
Referenced by getLayoutStyle(), and hidePane().
|
signal |
Referenced by deletePane(), and getLayoutStyle().
|
signal |
Referenced by deletePane(), and getLayoutStyle().
|
signal |
Referenced by addPane(), getLayoutStyle(), and paneDropAccepted().
|
signal |
|
signal |
|
signal |
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 604 of file PaneStack.cpp.
References sizePropertyStacks().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 610 of file PaneStack.cpp.
References sizePropertyStacks().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 616 of file PaneStack.cpp.
References PropertyStack::containsContainer(), currentLayerChanged(), PropertyStack::getClient(), m_currentPane, m_panes, and setCurrentPane().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 637 of file PaneStack.cpp.
References layerPropertiesRightButtonMenuRequested(), and panePropertiesRightButtonMenuRequested().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 654 of file PaneStack.cpp.
References setCurrentPane().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 661 of file PaneStack.cpp.
References setCurrentPane().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 669 of file PaneStack.cpp.
References paneRightButtonMenuRequested().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 715 of file PaneStack.cpp.
References dropAccepted().
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 722 of file PaneStack.cpp.
References dropAccepted().
|
slot |
Definition at line 729 of file PaneStack.cpp.
References m_panes.
Referenced by addPane(), and getLayoutStyle().
|
slot |
Definition at line 740 of file PaneStack.cpp.
References m_panes, and setCurrentPane().
Referenced by addPane(), and getLayoutStyle().
|
protected |
Definition at line 677 of file PaneStack.cpp.
References m_panes, m_propertyStackMinWidth, m_propertyStackStack, and propertyStacksResized().
Referenced by propertyContainerAdded(), and propertyContainerRemoved().
|
protected |
Definition at line 433 of file PaneStack.cpp.
References getPaneCount(), m_options, m_panes, NoCloseOnFirstPane, and NoPaneAccessories.
Referenced by addPane(), deletePane(), hidePane(), and showPane().
|
protected |
|
protected |
Definition at line 213 of file PaneStack.cpp.
References adjustAlignmentViewHeights(), m_options, m_panes, and ShowAlignmentViews.
Referenced by addPane(), deletePane(), hidePane(), and showPane().
|
overrideprotected |
Definition at line 247 of file PaneStack.cpp.
References adjustAlignmentViewHeights().
|
protected |
Definition at line 253 of file PaneStack.cpp.
References m_options, m_panes, NoUserResize, ViewManager::scalePixelSize(), and ShowAlignmentViews.
Referenced by relinkAlignmentViews(), and resizeEvent().
Member Data Documentation
|
protected |
Definition at line 131 of file PaneStack.h.
Referenced by addPane(), deletePane(), getCurrentPane(), hidePane(), propertyContainerSelected(), setCurrentLayer(), and setCurrentPane().
|
protected |
Definition at line 144 of file PaneStack.h.
Referenced by addPane(), adjustAlignmentViewHeights(), deletePane(), getPane(), getPaneCount(), hidePane(), indicatorClicked(), paneDeleteButtonClicked(), propertyContainerSelected(), relinkAlignmentViews(), setCurrentLayer(), setCurrentPane(), setLayoutStyle(), setPropertyStackMinWidth(), showOrHidePaneAccessories(), showPane(), sizePanesEqually(), sizePropertyStacks(), and unlinkAlignmentViews().
|
protected |
Definition at line 145 of file PaneStack.h.
Referenced by deletePane(), getHiddenPane(), getHiddenPaneCount(), hidePane(), and showPane().
|
protected |
Definition at line 147 of file PaneStack.h.
Referenced by addPane(), adjustAlignmentViewHeights(), PaneStack(), relinkAlignmentViews(), setLayoutStyle(), showOrHidePaneAccessories(), and sizePanesEqually().
|
protected |
Definition at line 148 of file PaneStack.h.
Referenced by addPane(), PaneStack(), and sizePanesEqually().
|
protected |
Definition at line 149 of file PaneStack.h.
Referenced by PaneStack().
|
protected |
Definition at line 150 of file PaneStack.h.
Referenced by addPane(), and PaneStack().
|
protected |
Definition at line 152 of file PaneStack.h.
Referenced by addPane(), PaneStack(), setCurrentPane(), setLayoutStyle(), and sizePropertyStacks().
|
protected |
Definition at line 154 of file PaneStack.h.
Referenced by addPane().
|
protected |
Definition at line 155 of file PaneStack.h.
Referenced by setPropertyStackMinWidth(), and sizePropertyStacks().
|
protected |
Definition at line 166 of file PaneStack.h.
Referenced by addPane(), getLayoutStyle(), PaneStack(), setCurrentPane(), and setLayoutStyle().
The documentation for this class was generated from the following files:
Generated by
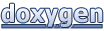