svgui
1.9
|
Layer.cpp
Go to the documentation of this file.
160 Layer::getXScaleValue(const LayerGeometryProvider *v, int x, double &value, QString &unit) const
276 // cerr << "sourceFrame = " << sourceFrame << ", referenceFrame = " << referenceFrame << " (have = " << i->hasReferenceFrame() << "), myMappedFrame = " << myMappedFrame << endl;
virtual bool isLayerDormant(const LayerGeometryProvider *v) const
Return whether the layer is dormant (i.e.
Definition: Layer.cpp:144
void modelCompletionChanged(ModelId)
virtual void addMeasurementRect(const QXmlAttributes &)
Add a measurement rectangle from the given XML attributes (presumably taken from a measurement elemen...
Definition: Layer.cpp:333
virtual bool getXScaleValue(const LayerGeometryProvider *v, int x, double &value, QString &unit) const
Return the value and unit at the given x coordinate in the given view.
Definition: Layer.cpp:160
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Convert the layer's data (though not those of the model it refers to) into XML for file output...
Definition: Layer.cpp:653
virtual void updateMeasureRectYCoords(LayerGeometryProvider *v, const MeasureRect &r) const
Definition: Layer.cpp:546
void addCommand(Command *command)
Add a command to the command history.
Definition: CommandHistory.cpp:135
virtual bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const =0
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
virtual void setLayerDormant(const LayerGeometryProvider *v, bool dormant)
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: Layer.cpp:136
virtual sv_frame_t getFrameForX(int x) const =0
Return the closest frame to the given pixel x-coordinate.
Definition: Layer.h:667
ModelId getSourceModel() const
Return the ID of the source model for the model represented in this layer.
Definition: Layer.cpp:68
virtual void paint(LayerGeometryProvider *, QPainter &, QRect) const =0
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
void toXml(QTextStream &stream, QString indent) const
Definition: Layer.cpp:315
virtual void measureDoubleClick(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:444
virtual void measureDrag(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:414
void modelChanged(ModelId)
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
QString getLayerPresentationName(LayerType type)
Definition: LayerFactory.cpp:70
virtual sv_frame_t alignFromReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:198
std::shared_ptr< PlayParameters > getPlayParameters() override
Return the play parameters for this layer, if any.
Definition: Layer.cpp:129
virtual void setMeasureRectYCoord(LayerGeometryProvider *v, MeasureRect &r, bool start, int y) const
Definition: Layer.cpp:554
QString getName() const override
Definition: Layer.cpp:372
virtual QString getLayerPresentationName() const
Definition: Layer.cpp:99
virtual bool getYScaleValue(const LayerGeometryProvider *, int, double &, QString &) const
Return the value and unit at the given y coordinate in the given view.
Definition: Layer.h:550
void layerParametersChanged()
virtual sv_frame_t getStartFrame() const =0
Retrieve the first visible sample frame on the widget.
bool clipboardHasDifferentAlignment(LayerGeometryProvider *v, const Clipboard &clip) const
Definition: Layer.cpp:209
virtual sv_frame_t alignToReference(LayerGeometryProvider *v, sv_frame_t frame) const
Definition: Layer.cpp:187
virtual void measureEnd(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:430
Definition: LayerFactory.h:27
virtual void toBriefXml(QTextStream &stream, QString indent="", QString extraAttributes="") const
Produce XML containing the layer's ID and type.
Definition: Layer.cpp:691
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
Definition: Layer.h:639
bool valueExtentsMatchMine(LayerGeometryProvider *v) const
Definition: Layer.cpp:628
MeasureRectSet::const_iterator findFocusedMeasureRect(QPoint) const
Definition: Layer.cpp:578
Definition: Layer.h:652
void updateMeasurePixrects(LayerGeometryProvider *v) const
Definition: Layer.cpp:507
virtual void drawMeasurementRect(QPainter &p, const Layer *, QRect rect, bool focus) const =0
void showLayer(LayerGeometryProvider *, bool show)
Change the visibility status (dormancy) of the layer in the given view.
Definition: Layer.cpp:153
void modelAlignmentCompletionChanged(ModelId)
virtual bool hasTimeXAxis() const
Return true if the X axis on the layer is time proportional to audio frames, false otherwise...
Definition: Layer.h:475
virtual void setMeasureRectFromPixrect(LayerGeometryProvider *v, MeasureRect &r, QRect pixrect) const
Definition: Layer.cpp:565
virtual ModelId getModel() const =0
Return the ID of the model represented in this layer.
virtual void measureStart(LayerGeometryProvider *, QMouseEvent *)
Definition: Layer.cpp:390
void modelChangedWithin(ModelId, sv_frame_t startFrame, sv_frame_t endFrame)
virtual bool getVisibleExtentsForUnit(QString unit, double &min, double &max, bool &log) const =0
Return the visible vertical extents for the given unit, if any.
virtual sv_frame_t getEndFrame() const =0
Retrieve the last visible sample frame on the widget.
QString getPropertyContainerIconName() const override
Definition: Layer.cpp:80
virtual int getXForFrame(sv_frame_t frame) const =0
Return the pixel x-coordinate corresponding to a given sample frame (which may be negative)...
virtual int getPaintWidth() const
Definition: LayerGeometryProvider.h:187
virtual void paintMeasurementRects(LayerGeometryProvider *, QPainter &, bool showFocus, QPoint focusPoint) const
Definition: Layer.cpp:464
virtual bool getYScaleDifference(const LayerGeometryProvider *v, int y0, int y1, double &diff, QString &unit) const
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
Definition: Layer.cpp:173
virtual bool nearestMeasurementRectChanged(LayerGeometryProvider *, QPoint prev, QPoint now) const
Definition: Layer.cpp:496
void layerNameChanged()
void paintMeasurementRect(LayerGeometryProvider *v, QPainter &paint, const MeasureRect &r, bool focus) const
Definition: Layer.cpp:605
virtual View * getView()=0
Generated by
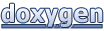