svgui
1.9
|
The CommandHistory class stores a list of executed commands and maintains Undo and Redo actions synchronised with those commands. More...
#include <CommandHistory.h>
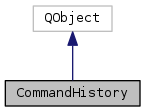
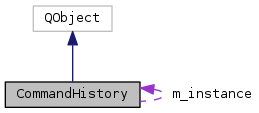
Public Slots | |
virtual void | documentSaved () |
Checkpoint function that should be called when the document is saved. More... | |
void | addExecutedCommand (Command *) |
Add a command to the history that has already been executed, without executing it again. More... | |
void | addCommandAndExecute (Command *) |
Add a command to the history and also execute it. More... | |
void | undo () |
void | redo () |
Signals | |
void | commandExecuted () |
Emitted whenever a command has just been executed or unexecuted, whether by addCommand, undo, or redo. More... | |
void | commandExecuted (Command *) |
Emitted whenever a command has just been executed, whether by addCommand or redo. More... | |
void | commandUnexecuted (Command *) |
Emitted whenever a command has just been unexecuted, whether by addCommand or undo. More... | |
void | documentRestored () |
Emitted when the undo/redo stack has reached the same state at which the documentSaved slot was last called. More... | |
void | activity (QString) |
Emitted when some activity happened (for activity logging). More... | |
Public Member Functions | |
virtual | ~CommandHistory () |
void | clear () |
void | registerMenu (QMenu *menu) |
void | registerToolbar (QToolBar *toolbar) |
void | addCommand (Command *command) |
Add a command to the command history. More... | |
void | addCommand (Command *command, bool execute, bool bundle=false) |
Add a command to the command history. More... | |
int | getUndoLimit () const |
Return the maximum number of items in the undo history. More... | |
void | setUndoLimit (int limit) |
Set the maximum number of items in the undo history. More... | |
int | getRedoLimit () const |
Return the maximum number of items in the redo history. More... | |
void | setRedoLimit (int limit) |
Set the maximum number of items in the redo history. More... | |
int | getMenuLimit () const |
Return the maximum number of items visible in undo and redo menus. More... | |
void | setMenuLimit (int limit) |
Set the maximum number of items in the menus. More... | |
int | getBundleTimeout () const |
Return the time after which a bundle will be closed if nothing is added. More... | |
void | setBundleTimeout (int msec) |
Set the time after which a bundle will be closed if nothing is added. More... | |
void | startCompoundOperation (QString name, bool execute) |
Start recording commands to batch up into a single compound command. More... | |
void | endCompoundOperation () |
Finish recording commands and store the compound command. More... | |
Static Public Member Functions | |
static CommandHistory * | getInstance () |
Protected Types | |
typedef std::stack< Command * > | CommandStack |
Protected Slots | |
void | undoActivated (QAction *) |
void | redoActivated (QAction *) |
void | bundleTimerTimeout () |
Protected Member Functions | |
CommandHistory () | |
void | addToCompound (Command *command, bool execute) |
void | addToBundle (Command *command, bool execute) |
void | closeBundle () |
void | updateActions () |
void | clipCommands () |
void | clipStack (CommandStack &stack, int limit) |
void | clearStack (CommandStack &stack) |
Protected Attributes | |
QAction * | m_undoAction |
QAction * | m_redoAction |
QAction * | m_undoMenuAction |
QAction * | m_redoMenuAction |
QMenu * | m_undoMenu |
QMenu * | m_redoMenu |
std::map< QAction *, int > | m_actionCounts |
CommandStack | m_undoStack |
CommandStack | m_redoStack |
int | m_undoLimit |
int | m_redoLimit |
int | m_menuLimit |
int | m_savedAt |
MacroCommand * | m_currentCompound |
bool | m_executeCompound |
MacroCommand * | m_currentBundle |
bool | m_bundling |
QString | m_currentBundleName |
QTimer * | m_bundleTimer |
int | m_bundleTimeout |
Static Protected Attributes | |
static CommandHistory * | m_instance = nullptr |
Detailed Description
The CommandHistory class stores a list of executed commands and maintains Undo and Redo actions synchronised with those commands.
CommandHistory allows you to associate more than one Undo and Redo menu or toolbar with the same command history, and it keeps them all up-to-date at once. This makes it effective in systems where multiple views may be editing the same data.
Definition at line 52 of file CommandHistory.h.
Member Typedef Documentation
|
protected |
Definition at line 202 of file CommandHistory.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 90 of file CommandHistory.cpp.
References clearStack(), m_redoMenu, m_redoStack, m_savedAt, m_undoMenu, and m_undoStack.
|
protected |
Definition at line 47 of file CommandHistory.cpp.
References IconLoader::load(), m_redoAction, m_redoMenu, m_redoMenuAction, m_undoAction, m_undoMenu, m_undoMenuAction, redo(), redoActivated(), undo(), and undoActivated().
Referenced by getBundleTimeout(), and getInstance().
Member Function Documentation
|
static |
Definition at line 101 of file CommandHistory.cpp.
References CommandHistory(), and m_instance.
Referenced by ModelDataTableDialog::addCommand(), NoteLayer::addNoteOff(), FlexiNoteLayer::addNoteOff(), Layer::deleteCurrentMeasureRect(), PropertyBox::editPlayParameters(), Pane::editSelectionEnd(), TextLayer::finish(), TimeInstantLayer::finish(), ImageLayer::finish(), BoxLayer::finish(), RegionLayer::finish(), NoteLayer::finish(), TimeValueLayer::finish(), FlexiNoteLayer::finish(), SpectrogramLayer::measureDoubleClick(), Layer::measureEnd(), ModelDataTableDialog::ModelDataTableDialog(), PropertyBox::playAudibleButtonChanged(), PropertyBox::playGainControlChanged(), PropertyBox::playPanControlChanged(), PropertyBox::propertyControllerChanged(), ViewManager::setSelections(), and PropertyStack::showLayer().
void CommandHistory::clear | ( | ) |
Definition at line 108 of file CommandHistory.cpp.
References clearStack(), closeBundle(), m_redoStack, m_savedAt, m_undoStack, and updateActions().
void CommandHistory::registerMenu | ( | QMenu * | menu | ) |
Definition at line 121 of file CommandHistory.cpp.
References m_redoAction, and m_undoAction.
void CommandHistory::registerToolbar | ( | QToolBar * | toolbar | ) |
Definition at line 128 of file CommandHistory.cpp.
References m_redoMenuAction, and m_undoMenuAction.
Referenced by ModelDataTableDialog::ModelDataTableDialog().
void CommandHistory::addCommand | ( | Command * | command | ) |
Add a command to the command history.
The command will normally be executed before being added; but if a compound operation is in use (see startCompoundOperation below), the execute status of the compound operation will determine whether the command is executed or not.
Definition at line 135 of file CommandHistory.cpp.
References addToCompound(), m_currentCompound, and m_executeCompound.
Referenced by ModelDataTableDialog::addCommand(), addCommandAndExecute(), addExecutedCommand(), NoteLayer::addNoteOff(), FlexiNoteLayer::addNoteOff(), addToBundle(), Layer::deleteCurrentMeasureRect(), PropertyBox::editPlayParameters(), endCompoundOperation(), TextLayer::finish(), TimeInstantLayer::finish(), ImageLayer::finish(), BoxLayer::finish(), RegionLayer::finish(), NoteLayer::finish(), TimeValueLayer::finish(), FlexiNoteLayer::finish(), SpectrogramLayer::measureDoubleClick(), Layer::measureEnd(), PropertyBox::playAudibleButtonChanged(), PropertyBox::playGainControlChanged(), PropertyBox::playPanControlChanged(), PropertyBox::propertyControllerChanged(), ViewManager::setSelections(), and PropertyStack::showLayer().
void CommandHistory::addCommand | ( | Command * | command, |
bool | execute, | ||
bool | bundle = false |
||
) |
Add a command to the command history.
If execute is true, the command will be executed before being added. Otherwise it will be assumed to have been already executed – a command should not be added to the history unless its work has actually been done somehow!
If a compound operation is in use (see startCompoundOperation below), the execute value passed to this method will override the execute status of the compound operation. In this way it's possible to have a compound operation mixing both to-execute and pre-executed commands.
If bundle is true, the command will be a candidate for bundling with any adjacent bundleable commands that have the same name, into a single compound command. This is useful for small commands that may be executed repeatedly altering the same data (e.g. type text, set a parameter) whose number and extent is not known in advance. The bundle parameter will be ignored if a compound operation is already in use.
Definition at line 148 of file CommandHistory.cpp.
References activity(), addToBundle(), addToCompound(), clearStack(), clipCommands(), closeBundle(), commandExecuted(), m_bundling, m_currentBundle, m_currentCompound, m_redoStack, m_savedAt, m_undoStack, and updateActions().
|
inline |
Return the maximum number of items in the undo history.
Definition at line 101 of file CommandHistory.h.
References m_undoLimit, and setUndoLimit().
void CommandHistory::setUndoLimit | ( | int | limit | ) |
Set the maximum number of items in the undo history.
Definition at line 396 of file CommandHistory.cpp.
References clipCommands(), and m_undoLimit.
Referenced by getUndoLimit().
|
inline |
Return the maximum number of items in the redo history.
Definition at line 107 of file CommandHistory.h.
References m_redoLimit, and setRedoLimit().
void CommandHistory::setRedoLimit | ( | int | limit | ) |
Set the maximum number of items in the redo history.
Definition at line 405 of file CommandHistory.cpp.
References clipCommands(), and m_redoLimit.
Referenced by getRedoLimit().
|
inline |
Return the maximum number of items visible in undo and redo menus.
Definition at line 113 of file CommandHistory.h.
References m_menuLimit, and setMenuLimit().
void CommandHistory::setMenuLimit | ( | int | limit | ) |
Set the maximum number of items in the menus.
Definition at line 414 of file CommandHistory.cpp.
References m_menuLimit, and updateActions().
Referenced by getMenuLimit().
|
inline |
Return the time after which a bundle will be closed if nothing is added.
Definition at line 119 of file CommandHistory.h.
References activity(), addCommandAndExecute(), addExecutedCommand(), bundleTimerTimeout(), commandExecuted(), CommandHistory(), commandUnexecuted(), documentRestored(), documentSaved(), endCompoundOperation(), m_bundleTimeout, redo(), redoActivated(), setBundleTimeout(), startCompoundOperation(), undo(), and undoActivated().
void CommandHistory::setBundleTimeout | ( | int | msec | ) |
Set the time after which a bundle will be closed if nothing is added.
Definition at line 421 of file CommandHistory.cpp.
References m_bundleTimeout.
Referenced by getBundleTimeout().
void CommandHistory::startCompoundOperation | ( | QString | name, |
bool | execute | ||
) |
Start recording commands to batch up into a single compound command.
Definition at line 289 of file CommandHistory.cpp.
References closeBundle(), m_currentCompound, and m_executeCompound.
Referenced by Pane::editSelectionEnd(), and getBundleTimeout().
void CommandHistory::endCompoundOperation | ( | ) |
Finish recording commands and store the compound command.
Definition at line 308 of file CommandHistory.cpp.
References addCommand(), and m_currentCompound.
Referenced by Pane::editSelectionEnd(), and getBundleTimeout().
|
virtualslot |
Checkpoint function that should be called when the document is saved.
If the undo/redo stack later returns to the point at which the document was saved, the documentRestored signal will be emitted.
Definition at line 427 of file CommandHistory.cpp.
References closeBundle(), m_savedAt, and m_undoStack.
Referenced by getBundleTimeout().
|
slot |
Add a command to the history that has already been executed, without executing it again.
Equivalent to addCommand(command, false).
Definition at line 332 of file CommandHistory.cpp.
References addCommand().
Referenced by getBundleTimeout().
|
slot |
Add a command to the history and also execute it.
Equivalent to addCommand(command, true).
Definition at line 338 of file CommandHistory.cpp.
References addCommand().
Referenced by getBundleTimeout().
|
slot |
Definition at line 344 of file CommandHistory.cpp.
References activity(), clipCommands(), closeBundle(), commandExecuted(), commandUnexecuted(), documentRestored(), m_redoStack, m_savedAt, m_undoStack, and updateActions().
Referenced by CommandHistory(), getBundleTimeout(), undoActivated(), and updateActions().
|
slot |
Definition at line 370 of file CommandHistory.cpp.
References activity(), closeBundle(), commandExecuted(), documentRestored(), m_redoStack, m_savedAt, m_undoStack, and updateActions().
Referenced by CommandHistory(), getBundleTimeout(), and redoActivated().
|
protectedslot |
Definition at line 488 of file CommandHistory.cpp.
References m_actionCounts, and undo().
Referenced by CommandHistory(), and getBundleTimeout().
|
protectedslot |
Definition at line 497 of file CommandHistory.cpp.
References m_actionCounts, and redo().
Referenced by CommandHistory(), and getBundleTimeout().
|
protectedslot |
Definition at line 263 of file CommandHistory.cpp.
References closeBundle(), and m_currentBundle.
Referenced by addToBundle(), and getBundleTimeout().
|
signal |
Emitted whenever a command has just been executed or unexecuted, whether by addCommand, undo, or redo.
Referenced by addCommand(), addToBundle(), getBundleTimeout(), redo(), and undo().
|
signal |
Emitted whenever a command has just been executed, whether by addCommand or redo.
|
signal |
Emitted whenever a command has just been unexecuted, whether by addCommand or undo.
Referenced by getBundleTimeout(), and undo().
|
signal |
Emitted when the undo/redo stack has reached the same state at which the documentSaved slot was last called.
Referenced by getBundleTimeout(), redo(), and undo().
|
signal |
Emitted when some activity happened (for activity logging).
Referenced by addCommand(), closeBundle(), getBundleTimeout(), redo(), and undo().
|
protected |
Definition at line 273 of file CommandHistory.cpp.
References m_currentCompound.
Referenced by addCommand().
|
protected |
Definition at line 197 of file CommandHistory.cpp.
References addCommand(), bundleTimerTimeout(), closeBundle(), commandExecuted(), m_bundleTimeout, m_bundleTimer, m_bundling, m_currentBundle, m_currentBundleName, and updateActions().
Referenced by addCommand().
|
protected |
Definition at line 250 of file CommandHistory.cpp.
References activity(), m_currentBundle, and m_currentBundleName.
Referenced by addCommand(), addToBundle(), bundleTimerTimeout(), clear(), documentSaved(), redo(), startCompoundOperation(), and undo().
|
protected |
Definition at line 506 of file CommandHistory.cpp.
References m_actionCounts, m_menuLimit, m_redoAction, m_redoMenu, m_redoMenuAction, m_redoStack, m_undoAction, m_undoMenu, m_undoMenuAction, m_undoStack, and undo().
Referenced by addCommand(), addToBundle(), clear(), redo(), setMenuLimit(), and undo().
|
protected |
Definition at line 434 of file CommandHistory.cpp.
References clipStack(), m_redoLimit, m_redoStack, m_savedAt, m_undoLimit, and m_undoStack.
Referenced by addCommand(), setRedoLimit(), setUndoLimit(), and undo().
|
protected |
Definition at line 445 of file CommandHistory.cpp.
References clearStack(), and m_undoLimit.
Referenced by clipCommands().
|
protected |
Definition at line 472 of file CommandHistory.cpp.
Referenced by addCommand(), clear(), clipStack(), and ~CommandHistory().
Member Data Documentation
|
staticprotected |
Definition at line 191 of file CommandHistory.h.
Referenced by getInstance().
|
protected |
Definition at line 193 of file CommandHistory.h.
Referenced by CommandHistory(), registerMenu(), and updateActions().
|
protected |
Definition at line 194 of file CommandHistory.h.
Referenced by CommandHistory(), registerMenu(), and updateActions().
|
protected |
Definition at line 195 of file CommandHistory.h.
Referenced by CommandHistory(), registerToolbar(), and updateActions().
|
protected |
Definition at line 196 of file CommandHistory.h.
Referenced by CommandHistory(), registerToolbar(), and updateActions().
|
protected |
Definition at line 197 of file CommandHistory.h.
Referenced by CommandHistory(), updateActions(), and ~CommandHistory().
|
protected |
Definition at line 198 of file CommandHistory.h.
Referenced by CommandHistory(), updateActions(), and ~CommandHistory().
|
protected |
Definition at line 200 of file CommandHistory.h.
Referenced by redoActivated(), undoActivated(), and updateActions().
|
protected |
Definition at line 203 of file CommandHistory.h.
Referenced by addCommand(), clear(), clipCommands(), documentSaved(), redo(), undo(), updateActions(), and ~CommandHistory().
|
protected |
Definition at line 204 of file CommandHistory.h.
Referenced by addCommand(), clear(), clipCommands(), redo(), undo(), updateActions(), and ~CommandHistory().
|
protected |
Definition at line 206 of file CommandHistory.h.
Referenced by clipCommands(), clipStack(), getUndoLimit(), and setUndoLimit().
|
protected |
Definition at line 207 of file CommandHistory.h.
Referenced by clipCommands(), getRedoLimit(), and setRedoLimit().
|
protected |
Definition at line 208 of file CommandHistory.h.
Referenced by getMenuLimit(), setMenuLimit(), and updateActions().
|
protected |
Definition at line 209 of file CommandHistory.h.
Referenced by addCommand(), clear(), clipCommands(), documentSaved(), redo(), undo(), and ~CommandHistory().
|
protected |
Definition at line 211 of file CommandHistory.h.
Referenced by addCommand(), addToCompound(), endCompoundOperation(), and startCompoundOperation().
|
protected |
Definition at line 212 of file CommandHistory.h.
Referenced by addCommand(), and startCompoundOperation().
|
protected |
Definition at line 215 of file CommandHistory.h.
Referenced by addCommand(), addToBundle(), bundleTimerTimeout(), and closeBundle().
|
protected |
Definition at line 216 of file CommandHistory.h.
Referenced by addCommand(), and addToBundle().
|
protected |
Definition at line 217 of file CommandHistory.h.
Referenced by addToBundle(), and closeBundle().
|
protected |
Definition at line 218 of file CommandHistory.h.
Referenced by addToBundle().
|
protected |
Definition at line 219 of file CommandHistory.h.
Referenced by addToBundle(), getBundleTimeout(), and setBundleTimeout().
The documentation for this class was generated from the following files:
Generated by
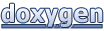