svgui
1.9
|
CommandHistory.h
Go to the documentation of this file.
void addToCompound(Command *command, bool execute)
Definition: CommandHistory.cpp:273
void setBundleTimeout(int msec)
Set the time after which a bundle will be closed if nothing is added.
Definition: CommandHistory.cpp:421
void addExecutedCommand(Command *)
Add a command to the history that has already been executed, without executing it again...
Definition: CommandHistory.cpp:332
void addCommandAndExecute(Command *)
Add a command to the history and also execute it.
Definition: CommandHistory.cpp:338
void startCompoundOperation(QString name, bool execute)
Start recording commands to batch up into a single compound command.
Definition: CommandHistory.cpp:289
void endCompoundOperation()
Finish recording commands and store the compound command.
Definition: CommandHistory.cpp:308
void addCommand(Command *command)
Add a command to the command history.
Definition: CommandHistory.cpp:135
void setUndoLimit(int limit)
Set the maximum number of items in the undo history.
Definition: CommandHistory.cpp:396
void activity(QString)
Emitted when some activity happened (for activity logging).
void setMenuLimit(int limit)
Set the maximum number of items in the menus.
Definition: CommandHistory.cpp:414
void documentRestored()
Emitted when the undo/redo stack has reached the same state at which the documentSaved slot was last ...
void registerToolbar(QToolBar *toolbar)
Definition: CommandHistory.cpp:128
void commandUnexecuted(Command *)
Emitted whenever a command has just been unexecuted, whether by addCommand or undo.
std::map< QAction *, int > m_actionCounts
Definition: CommandHistory.h:200
int getUndoLimit() const
Return the maximum number of items in the undo history.
Definition: CommandHistory.h:101
int getRedoLimit() const
Return the maximum number of items in the redo history.
Definition: CommandHistory.h:107
The CommandHistory class stores a list of executed commands and maintains Undo and Redo actions synch...
Definition: CommandHistory.h:52
int getMenuLimit() const
Return the maximum number of items visible in undo and redo menus.
Definition: CommandHistory.h:113
int getBundleTimeout() const
Return the time after which a bundle will be closed if nothing is added.
Definition: CommandHistory.h:119
void setRedoLimit(int limit)
Set the maximum number of items in the redo history.
Definition: CommandHistory.cpp:405
void commandExecuted()
Emitted whenever a command has just been executed or unexecuted, whether by addCommand, undo, or redo.
void clipStack(CommandStack &stack, int limit)
Definition: CommandHistory.cpp:445
void addToBundle(Command *command, bool execute)
Definition: CommandHistory.cpp:197
virtual void documentSaved()
Checkpoint function that should be called when the document is saved.
Definition: CommandHistory.cpp:427
Generated by
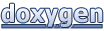