svgui
1.9
|
ImageLayer.h
Go to the documentation of this file.
95 int getVerticalScaleWidth(LayerGeometryProvider *, bool, QPainter &) const override { return 0; }
int getVerticalScaleWidth(LayerGeometryProvider *, bool, QPainter &) const override
Definition: ImageLayer.h:95
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: ImageLayer.cpp:995
bool isLayerScrollable(const LayerGeometryProvider *v) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: ImageLayer.cpp:136
static bool isImageFileSupported(QString url)
Definition: ImageLayer.cpp:609
std::map< const LayerGeometryProvider *, ImageMap > ViewImageMap
Definition: ImageLayer.h:121
Definition: ImageLayer.h:33
void drawEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: ImageLayer.cpp:583
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: ImageLayer.cpp:110
void addCommand(Command *command)
Add a command to the command history.
Definition: CommandHistory.cpp:135
EventVector getLocalPoints(LayerGeometryProvider *v, int x, int y) const
Definition: ImageLayer.cpp:142
bool snapToFeatureFrame(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override
!! too much overlap with TimeValueLayer/TimeInstantLayer/TextLayer
Definition: ImageLayer.cpp:228
void resizeSelection(Selection s, Selection newSize) override
Definition: ImageLayer.cpp:759
bool editOpen(LayerGeometryProvider *, QMouseEvent *) override
Open an editor on the item under the mouse (e.g.
Definition: ImageLayer.cpp:706
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: ImageLayer.cpp:988
QImage getImage(LayerGeometryProvider *v, QString name, QSize maxSize) const
Definition: ImageLayer.cpp:503
std::map< QString, QImage > ImageMap
!! how to reap no-longer-used images?
Definition: ImageLayer.h:120
std::map< QString, FileSource * > FileSourceMap
Definition: ImageLayer.h:122
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: ImageLayer.cpp:260
bool isLayerEditable() const override
This should return true if the layer can be edited by the user.
Definition: ImageLayer.h:85
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
static void checkAddSource(QString img, bool synchronise)
Definition: ImageLayer.cpp:918
void editEnd(LayerGeometryProvider *v, QMouseEvent *) override
Definition: ImageLayer.cpp:691
bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: ImageLayer.cpp:130
void moveSelection(Selection s, sv_frame_t newStartFrame) override
Definition: ImageLayer.cpp:737
PropertyType getPropertyType(const PropertyName &) const override
Definition: ImageLayer.cpp:104
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: ImageLayer.cpp:117
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: ImageLayer.cpp:188
bool paste(LayerGeometryProvider *v, const Clipboard &from, sv_frame_t frameOffset, bool interactive) override
Paste from the given clipboard onto the layer at the given frame offset.
Definition: ImageLayer.cpp:821
Definition: Layer.h:349
void drawDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: ImageLayer.cpp:565
ColourSignificance getLayerColourSignificance() const override
This should return the degree of meaning associated with colour in this layer.
Definition: ImageLayer.h:79
void drawStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: ImageLayer.cpp:540
void drawImage(LayerGeometryProvider *v, QPainter &paint, const Event &p, int x, int nx) const
Definition: ImageLayer.cpp:319
void setProperty(const PropertyName &, int value) override
Definition: ImageLayer.cpp:124
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: ImageLayer.h:67
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
void setLayerDormant(const LayerGeometryProvider *v, bool dormant) override
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: ImageLayer.cpp:466
void copy(LayerGeometryProvider *v, Selection s, Clipboard &to) override
Definition: ImageLayer.cpp:807
int getCompletion(LayerGeometryProvider *) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: ImageLayer.cpp:65
bool getImageOriginalSize(QString name, QSize &size) const
!! how to reap no-longer-used images?
Definition: ImageLayer.cpp:484
void editDrag(LayerGeometryProvider *v, QMouseEvent *) override
Definition: ImageLayer.cpp:669
QString getPropertyLabel(const PropertyName &) const override
Definition: ImageLayer.cpp:98
static QString getLocalFilename(QString img)
Definition: ImageLayer.cpp:891
void checkAddSourceAndConnect(QString img)
Definition: ImageLayer.cpp:906
void editStart(LayerGeometryProvider *v, QMouseEvent *) override
Definition: ImageLayer.cpp:646
virtual bool addImage(sv_frame_t frame, QString url)
Definition: ImageLayer.cpp:624
Generated by
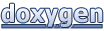