svgui
1.9
|
#include <LayerFactory.h>

Public Types | |
enum | LayerType { Waveform, Spectrogram, TimeRuler, TimeInstants, TimeValues, Notes, FlexiNotes, Regions, Boxes, Text, Image, Colour3DPlot, Spectrum, Slice, MelodicRangeSpectrogram, PeakFrequencySpectrogram, UnknownLayer = 255 } |
typedef std::set< LayerType > | LayerTypeSet |
Public Member Functions | |
virtual | ~LayerFactory () |
LayerTypeSet | getValidLayerTypes (ModelId modelId) |
LayerTypeSet | getValidEmptyLayerTypes () |
Return the set of layer types that an end user should be allowed to create, empty, for subsequent editing. More... | |
LayerType | getLayerType (const Layer *) |
Layer * | createLayer (LayerType type) |
void | setLayerDefaultProperties (LayerType type, Layer *layer) |
Set the default properties of a layer, from the XML string contained in the LayerDefaults settings group for the given layer type. More... | |
void | setLayerProperties (Layer *layer, QString xmlString) |
Set the properties of a layer, from the XML string provided. More... | |
QString | getLayerPresentationName (LayerType type) |
bool | isLayerSliceable (const Layer *) |
void | setModel (Layer *layer, ModelId model) |
std::shared_ptr< Model > | createEmptyModel (LayerType type, ModelId baseModel) |
int | getChannel (Layer *layer) |
void | setChannel (Layer *layer, int channel) |
QString | getLayerIconName (LayerType) |
QString | getLayerTypeName (LayerType) |
LayerType | getLayerTypeForName (QString) |
LayerType | getLayerTypeForClipboardContents (const Clipboard &) |
Static Public Member Functions | |
static LayerFactory * | getInstance () |
Protected Member Functions | |
template<typename LayerClass , typename ModelClass > | |
bool | trySetModel (Layer *layerBase, ModelId modelId) |
Static Protected Attributes | |
static LayerFactory * | m_instance = new LayerFactory |
Detailed Description
Definition at line 27 of file LayerFactory.h.
Member Typedef Documentation
typedef std::set<LayerType> LayerFactory::LayerTypeSet |
Definition at line 60 of file LayerFactory.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Waveform | |
Spectrogram | |
TimeRuler | |
TimeInstants | |
TimeValues | |
Notes | |
FlexiNotes | |
Regions | |
Boxes | |
Text | |
Image | |
Colour3DPlot | |
Spectrum | |
Slice | |
MelodicRangeSpectrogram | |
PeakFrequencySpectrogram | |
UnknownLayer |
Definition at line 30 of file LayerFactory.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 65 of file LayerFactory.cpp.
Member Function Documentation
|
static |
Definition at line 60 of file LayerFactory.cpp.
References m_instance.
Referenced by TimeRulerLayer::getLayerPresentationName(), Layer::getLayerPresentationName(), Layer::getPropertyContainerIconName(), Pane::getTopFlexiNoteLayer(), PropertyStack::repopulate(), Layer::toBriefXml(), Layer::toXml(), and CSVFormatDialog::updateModelLabel().
LayerFactory::LayerTypeSet LayerFactory::getValidLayerTypes | ( | ModelId | modelId | ) |
Definition at line 139 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, Image, MelodicRangeSpectrogram, Notes, PeakFrequencySpectrogram, Regions, Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeValues, and Waveform.
LayerFactory::LayerTypeSet LayerFactory::getValidEmptyLayerTypes | ( | ) |
Return the set of layer types that an end user should be allowed to create, empty, for subsequent editing.
!! and in principle Colour3DPlot – now that's a challenge
Definition at line 202 of file LayerFactory.cpp.
References Boxes, Image, Notes, Regions, Text, TimeInstants, and TimeValues.
LayerFactory::LayerType LayerFactory::getLayerType | ( | const Layer * | layer | ) |
Definition at line 221 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, Image, Notes, Regions, Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeRuler, TimeValues, UnknownLayer, and Waveform.
Referenced by TimeRulerLayer::getLayerPresentationName(), Layer::getLayerPresentationName(), and Pane::getTopFlexiNoteLayer().
Definition at line 421 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, getLayerPresentationName(), Image, SpectrogramLayer::MelodicPeaks, SpectrogramLayer::MelodicRange, MelodicRangeSpectrogram, Notes, PeakFrequencySpectrogram, Regions, setLayerDefaultProperties(), Layer::setObjectName(), Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeRuler, TimeValues, UnknownLayer, and Waveform.
Set the default properties of a layer, from the XML string contained in the LayerDefaults settings group for the given layer type.
Leave unchanged any properties not mentioned in the settings.
Definition at line 511 of file LayerFactory.cpp.
References getLayerTypeName(), and setLayerProperties().
Referenced by createLayer().
void LayerFactory::setLayerProperties | ( | Layer * | layer, |
QString | xmlString | ||
) |
Set the properties of a layer, from the XML string provided.
Leave unchanged any properties not mentioned.
Definition at line 524 of file LayerFactory.cpp.
References Layer::setProperties().
Referenced by setLayerDefaultProperties().
QString LayerFactory::getLayerPresentationName | ( | LayerType | type | ) |
Definition at line 70 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, Image, MelodicRangeSpectrogram, Notes, PeakFrequencySpectrogram, Regions, Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeRuler, TimeValues, UnknownLayer, and Waveform.
Referenced by createLayer(), TimeRulerLayer::getLayerPresentationName(), Layer::getLayerPresentationName(), PropertyStack::repopulate(), and CSVFormatDialog::updateModelLabel().
bool LayerFactory::isLayerSliceable | ( | const Layer * | layer | ) |
!! We can create slices of spectrograms, but there's a
Definition at line 106 of file LayerFactory.cpp.
void LayerFactory::setModel | ( | Layer * | layer, |
ModelId | model | ||
) |
Definition at line 317 of file LayerFactory.cpp.
std::shared_ptr< Model > LayerFactory::createEmptyModel | ( | LayerType | type, |
ModelId | baseModel | ||
) |
Definition at line 363 of file LayerFactory.cpp.
References Boxes, FlexiNotes, Image, Notes, Regions, Text, TimeInstants, and TimeValues.
int LayerFactory::getChannel | ( | Layer * | layer | ) |
Definition at line 392 of file LayerFactory.cpp.
void LayerFactory::setChannel | ( | Layer * | layer, |
int | channel | ||
) |
Definition at line 404 of file LayerFactory.cpp.
QString LayerFactory::getLayerIconName | ( | LayerType | type | ) |
Definition at line 241 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, Image, MelodicRangeSpectrogram, Notes, PeakFrequencySpectrogram, Regions, Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeRuler, TimeValues, UnknownLayer, and Waveform.
Referenced by Layer::getPropertyContainerIconName().
QString LayerFactory::getLayerTypeName | ( | LayerType | type | ) |
Definition at line 268 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, Image, MelodicRangeSpectrogram, Notes, PeakFrequencySpectrogram, Regions, Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeRuler, TimeValues, UnknownLayer, and Waveform.
Referenced by setLayerDefaultProperties().
LayerFactory::LayerType LayerFactory::getLayerTypeForName | ( | QString | name | ) |
Definition at line 295 of file LayerFactory.cpp.
References Boxes, Colour3DPlot, FlexiNotes, Image, MelodicRangeSpectrogram, Notes, PeakFrequencySpectrogram, Regions, Slice, Spectrogram, Spectrum, Text, TimeInstants, TimeRuler, TimeValues, UnknownLayer, and Waveform.
LayerFactory::LayerType LayerFactory::getLayerTypeForClipboardContents | ( | const Clipboard & | clip | ) |
Definition at line 570 of file LayerFactory.cpp.
References Notes, Regions, TimeInstants, and TimeValues.
|
inlineprotected |
Definition at line 105 of file LayerFactory.h.
Member Data Documentation
|
staticprotected |
Definition at line 116 of file LayerFactory.h.
Referenced by getInstance().
The documentation for this class was generated from the following files:
Generated by
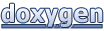