svgui
1.9
|
SpectrogramLayer.h
Go to the documentation of this file.
75 void paintVerticalScale(LayerGeometryProvider *v, bool detailed, QPainter &paint, QRect rect) const override;
313 bool getYBinRange(LayerGeometryProvider *v, int y, double &freqBinMin, double &freqBinMax) const;
315 bool getYBinSourceRange(LayerGeometryProvider *v, int y, double &freqMin, double &freqMax) const;
319 bool getXBinSourceRange(LayerGeometryProvider *v, int x, RealTime &timeMin, RealTime &timeMax) const;
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SpectrogramLayer.cpp:512
void setChannel(int)
Specify the channel to use from the source model.
Definition: SpectrogramLayer.cpp:766
void setColourRotation(int)
Specify the colourmap rotation for the colour scale.
Definition: SpectrogramLayer.cpp:947
RangeMapper * getNewVerticalZoomRangeMapper() const override
Create and return a range mapper for vertical zoom step values.
Definition: SpectrogramLayer.cpp:2599
void measureDoubleClick(LayerGeometryProvider *, QMouseEvent *) override
Definition: SpectrogramLayer.cpp:1910
bool getYScaleValue(const LayerGeometryProvider *, int, double &, QString &) const override
Return the value and unit at the given y coordinate in the given view.
Definition: SpectrogramLayer.cpp:1879
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: SpectrogramLayer.h:67
void setNormalizeVisibleArea(bool)
Specify whether to normalize the visible area.
Definition: SpectrogramLayer.cpp:1073
bool isLayerScrollable(const LayerGeometryProvider *) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: SpectrogramLayer.cpp:1115
bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: SpectrogramLayer.cpp:1841
SpectrogramLayer(Configuration=FullRangeDb)
Construct a SpectrogramLayer with default parameters appropriate for the given configuration.
Definition: SpectrogramLayer.cpp:59
PropertyList getProperties() const override
Definition: SpectrogramLayer.cpp:283
double getYForBin(const LayerGeometryProvider *, double bin) const override
!! VerticalBinLayer methods. Note overlap with get*BinRange()
Definition: SpectrogramLayer.cpp:1213
double getFrequencyForY(const LayerGeometryProvider *v, int y) const
Definition: SpectrogramLayer.cpp:1786
void checkCacheSpace(int *suggestedPeakDivisor, bool *createWholeCache) const
Definition: SpectrogramLayer.cpp:1511
SpectrogramLayer represents waveform data (obtained from a DenseTimeValueModel) in spectrogram form...
Definition: SpectrogramLayer.h:51
int getWindowIncrement() const
Definition: SpectrogramLayer.h:323
static std::pair< ColourScaleType, double > convertToColourScale(int value)
Definition: SpectrogramLayer.cpp:202
void illuminateLocalFeatures(LayerGeometryProvider *v, QPainter &painter) const
Definition: SpectrogramLayer.cpp:1732
bool getDisplayExtents(double &min, double &max) const override
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: SpectrogramLayer.cpp:1831
std::map< int, MagnitudeRange > ViewMagMap
Definition: SpectrogramLayer.h:344
void updateMeasureRectYCoords(LayerGeometryProvider *v, const MeasureRect &r) const override
Definition: SpectrogramLayer.cpp:2607
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SpectrogramLayer.cpp:355
bool getXBinSourceRange(LayerGeometryProvider *v, int x, RealTime &timeMin, RealTime &timeMax) const
Definition: SpectrogramLayer.cpp:1278
void paintWithRenderer(LayerGeometryProvider *v, QPainter &paint, QRect rect) const
Definition: SpectrogramLayer.cpp:1652
double getBinForY(const LayerGeometryProvider *, double y) const override
Return the bin number, possibly fractional, at the given y coordinate.
Definition: SpectrogramLayer.cpp:1231
int getColourScaleWidth(QPainter &) const
Definition: SpectrogramLayer.cpp:2167
int getColourRotation() const
Definition: Layer.h:141
bool getYBinRange(LayerGeometryProvider *v, int y, double &freqBinMin, double &freqBinMax) const
Definition: SpectrogramLayer.cpp:1202
void setColourScaleMultiple(double)
Specify multiple factor for colour scale.
Definition: SpectrogramLayer.cpp:985
bool getXYBinSourceRange(LayerGeometryProvider *v, int x, int y, double &min, double &max, double &phaseMin, double &phaseMax) const
Definition: SpectrogramLayer.cpp:1391
bool m_normalizeVisibleArea
Definition: SpectrogramLayer.h:292
void setNormalization(ColumnNormalization)
Specify the normalization mode for individual columns.
Definition: SpectrogramLayer.cpp:1055
void setThreshold(float threshold)
Set the threshold for sample values to qualify for being shown in the FFT, in voltage units...
Definition: SpectrogramLayer.cpp:879
Definition: Layer.h:353
void invalidateMagnitudes()
Definition: SpectrogramLayer.cpp:1559
void setProperty(const PropertyName &, int value) override
Definition: SpectrogramLayer.cpp:639
void setVerticallyFixed()
Mark the spectrogram layer as having a fixed range in the vertical axis.
Definition: SpectrogramLayer.cpp:139
void setWindowHopLevel(int level)
Definition: SpectrogramLayer.cpp:806
static std::pair< ColumnNormalization, bool > convertToColumnNorm(int value)
Definition: SpectrogramLayer.cpp:229
double getEffectiveMinFrequency() const
Definition: SpectrogramLayer.cpp:1166
bool snapToFeatureFrame(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override
Adjust the given frame to snap to the nearest feature, if possible.
Definition: SpectrogramLayer.cpp:1888
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
VerticalPosition getPreferredFrameCountPosition() const override
Definition: SpectrogramLayer.h:215
int getVerticalScaleWidth(LayerGeometryProvider *v, bool detailed, QPainter &) const override
Definition: SpectrogramLayer.cpp:2177
void setVerticalZoomStep(int) override
Set the vertical zoom step.
Definition: SpectrogramLayer.cpp:2530
ColourScaleType getColourScale() const
Definition: SpectrogramLayer.cpp:979
Definition: Colour3DPlotRenderer.h:49
Interface for layers in which the Y axis corresponds to bin number rather than scale value...
Definition: VerticalBinLayer.h:28
void setMeasureRectYCoord(LayerGeometryProvider *v, MeasureRect &r, bool start, int y) const override
Definition: SpectrogramLayer.cpp:2621
void setOversampling(int oversampling)
Definition: SpectrogramLayer.cpp:822
ModelId getExportModel(LayerGeometryProvider *) const override
Return the ID of a model representing the contents of this layer in a form suitable for export to a t...
Definition: SpectrogramLayer.cpp:147
ColourSignificance getLayerColourSignificance() const override
This should return the degree of meaning associated with colour in this layer.
Definition: SpectrogramLayer.h:221
bool hasLightBackground() const override
Definition: SpectrogramLayer.cpp:1159
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: SpectrogramLayer.cpp:1711
double getYForFrequency(const LayerGeometryProvider *v, double frequency) const
Definition: SpectrogramLayer.cpp:1777
int getWindowHopLevel() const
Definition: SpectrogramLayer.cpp:816
void setSynchronousPainting(bool synchronous) override
Enable or disable synchronous painting.
Definition: SpectrogramLayer.cpp:1568
ColumnNormalization m_normalization
Definition: SpectrogramLayer.h:291
void paintDetailedScale(LayerGeometryProvider *v, QPainter &paint, QRect rect) const
Definition: SpectrogramLayer.cpp:2288
QString getError(LayerGeometryProvider *v) const override
Return an error string if any errors have occurred while loading or processing data for the given vie...
Definition: SpectrogramLayer.cpp:1807
int getVerticalZoomSteps(int &defaultStep) const override
Get the number of vertical zoom steps available for this layer.
Definition: SpectrogramLayer.cpp:2491
bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: SpectrogramLayer.cpp:1815
void preferenceChanged(PropertyContainer::PropertyName name)
Definition: SpectrogramLayer.cpp:742
bool getYBinSourceRange(LayerGeometryProvider *v, int y, double &freqMin, double &freqMax) const
Definition: SpectrogramLayer.cpp:1300
Definition: Layer.h:639
void paintVerticalScale(LayerGeometryProvider *v, bool detailed, QPainter &paint, QRect rect) const override
Definition: SpectrogramLayer.cpp:2199
static int convertFromColourScale(ColourScaleType type, double multiple)
Definition: SpectrogramLayer.cpp:215
const ZoomConstraint * getZoomConstraint() const override
Return a zoom constraint object defining the supported zoom levels for this layer.
Definition: SpectrogramLayer.h:66
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SpectrogramLayer.cpp:626
void paintDetailedScalePhase(LayerGeometryProvider *v, QPainter &paint, QRect rect) const
Definition: SpectrogramLayer.cpp:2387
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SpectrogramLayer.cpp:2634
QString getPropertyIconName(const PropertyName &) const override
Definition: SpectrogramLayer.cpp:322
QString getPropertyGroupName(const PropertyName &) const override
Definition: SpectrogramLayer.cpp:338
void setProperties(const QXmlAttributes &attributes) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SpectrogramLayer.cpp:2703
BinDisplay getBinDisplay() const
Definition: SpectrogramLayer.cpp:1049
void paintCrosshairs(LayerGeometryProvider *, QPainter &, QPoint) const override
Definition: SpectrogramLayer.cpp:1969
double getEffectiveMaxFrequency() const
Definition: SpectrogramLayer.cpp:1184
Colour3DPlotRenderer * getRenderer(LayerGeometryProvider *) const
Definition: SpectrogramLayer.cpp:1574
View is the base class of widgets that display one or more overlaid views of data against a horizonta...
Definition: View.h:55
std::map< int, Colour3DPlotRenderer * > ViewRendererMap
Definition: SpectrogramLayer.h:349
QString getPropertyValueIconName(const PropertyName &, int value) const override
Definition: SpectrogramLayer.cpp:610
ColumnNormalization getNormalization() const
Definition: SpectrogramLayer.cpp:1067
void setLayerDormant(const LayerGeometryProvider *v, bool dormant) override
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: SpectrogramLayer.cpp:1091
bool isLayerOpaque() const override
This should return true if the layer completely obscures any underlying layers.
Definition: SpectrogramLayer.h:219
void setGain(float gain)
Set the gain multiplier for sample values in this view.
Definition: SpectrogramLayer.cpp:858
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: SpectrogramLayer.cpp:2054
void setColourScale(ColourScaleType)
Specify the scale for sample levels.
Definition: SpectrogramLayer.cpp:967
QString getPropertyLabel(const PropertyName &) const override
Definition: SpectrogramLayer.cpp:303
int getCompletion(LayerGeometryProvider *v) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: SpectrogramLayer.cpp:1795
ModelId getSliceableModel() const override
Definition: SpectrogramLayer.cpp:1553
double m_colourScaleMultiple
Definition: SpectrogramLayer.h:285
double getColourScaleMultiple() const
Definition: SpectrogramLayer.cpp:997
bool getAdjustedYBinSourceRange(LayerGeometryProvider *v, int x, int y, double &freqMin, double &freqMax, double &adjFreqMin, double &adjFreqMax) const
Definition: SpectrogramLayer.cpp:1322
void setWindowType(WindowType type)
Definition: SpectrogramLayer.cpp:838
bool getXBinRange(LayerGeometryProvider *v, int x, double &windowMin, double &windowMax) const
Definition: SpectrogramLayer.cpp:1251
int getCurrentVerticalZoomStep() const override
Get the current vertical zoom step.
Definition: SpectrogramLayer.cpp:2515
bool getCrosshairExtents(LayerGeometryProvider *, QPainter &, QPoint cursorPos, std::vector< QRect > &extents) const override
Definition: SpectrogramLayer.cpp:1925
bool getNormalizeVisibleArea() const
Definition: SpectrogramLayer.cpp:1085
void setBinDisplay(BinDisplay)
Specify the processing of frequency bins for the y axis.
Definition: SpectrogramLayer.cpp:1038
WindowType getWindowType() const
Definition: SpectrogramLayer.cpp:852
static int convertFromColumnNorm(ColumnNormalization norm, bool visible)
Definition: SpectrogramLayer.cpp:241
PropertyType getPropertyType(const PropertyName &) const override
Definition: SpectrogramLayer.cpp:328
Generated by
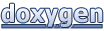