svgui
1.9
|
Colour3DPlotRenderer.h
Go to the documentation of this file.
318 DirectTranslucent
ColourScale colourScale
A complete ColourScale object by value, used for colour map conversion.
Definition: Colour3DPlotRenderer.h:78
bool willRenderOpaque(const LayerGeometryProvider *v)
Return true if the rendering will be opaque.
Definition: Colour3DPlotRenderer.h:228
Map values within a range onto a set of colours, with a given distribution (linear, log etc) and optional colourmap rotation.
Definition: ColourScale.h:34
Definition: RenderTimer.h:20
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
Definition: Colour3DPlotRenderer.h:49
bool interpolate
Whether to apply smoothing when rendering cells at more than one pixel per cell.
Definition: Colour3DPlotRenderer.h:100
Interface for layers in which the Y axis corresponds to bin number rather than scale value...
Definition: VerticalBinLayer.h:28
Colour3DPlotRenderer(Sources sources, Parameters parameters)
Definition: Colour3DPlotRenderer.h:119
bool showDerivative
Whether to show the frame-to-frame difference instead of the actual value.
Definition: Colour3DPlotRenderer.h:107
ColumnNormalization normalization
Type of column normalization.
Definition: Colour3DPlotRenderer.h:81
bool invertVertical
Whether to render the whole caboodle upside-down.
Definition: Colour3DPlotRenderer.h:103
double m_secondsPerXPixel
Definition: Colour3DPlotRenderer.h:282
bool m_secondsPerXPixelValid
Definition: Colour3DPlotRenderer.h:283
A cached image for a view that scrolls horizontally, such as a spectrogram.
Definition: ScrollableImageCache.h:37
QColor getColour(double value) const
Return the colour corresponding to the given value.
Definition: Colour3DPlotRenderer.h:237
A cached set of magnitude range records for a view that scrolls horizontally, such as a spectrogram...
Definition: ScrollableMagRangeCache.h:35
MagnitudeRange range
The magnitude range of the data in the rendered area, after initial scaling (parameters.scaleFactor) and normalisation, for use in displaying colour scale etc.
Definition: Colour3DPlotRenderer.h:143
const VerticalBinLayer * verticalBinLayer
Definition: Colour3DPlotRenderer.h:56
std::vector< ModelId > peakCaches
Definition: Colour3DPlotRenderer.h:59
BinScale binScale
Scale for vertical bin spacing (linear or logarithmic).
Definition: Colour3DPlotRenderer.h:87
ScrollableMagRangeCache m_magCache
Definition: Colour3DPlotRenderer.h:280
std::vector< MagnitudeRange > m_magRanges
Definition: Colour3DPlotRenderer.h:262
Generated by
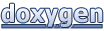