svgui
1.9
|
ScrollableImageCache.h
Go to the documentation of this file.
ZoomLevel getZoomLevel() const
Definition: ScrollableImageCache.h:85
sv_frame_t getStartFrame() const
Definition: ScrollableImageCache.h:103
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
const QImage & getImage() const
Definition: ScrollableImageCache.h:120
ScrollableImageCache()
Definition: ScrollableImageCache.h:40
void drawImage(int left, int width, QImage image, int imageLeft, int imageWidth)
Draw from an image onto the cache.
Definition: ScrollableImageCache.cpp:143
void adjustToTouchValidArea(int &left, int &width, bool &isLeftOfValidArea) const
Take a left coordinate and width describing a region, and adjust them so that they are contiguous wit...
Definition: ScrollableImageCache.cpp:114
void scrollTo(const LayerGeometryProvider *v, sv_frame_t newStartFrame)
Set the new start frame for the cache, according to the geometry of the supplied LayerGeometryProvide...
Definition: ScrollableImageCache.cpp:25
A cached image for a view that scrolls horizontally, such as a spectrogram.
Definition: ScrollableImageCache.h:37
Generated by
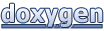