svgui
1.9
|
A cached image for a view that scrolls horizontally, such as a spectrogram. More...
#include <ScrollableImageCache.h>
Public Member Functions | |
ScrollableImageCache () | |
void | invalidate () |
bool | isValid () const |
QSize | getSize () const |
void | resize (QSize newSize) |
Set the size of the cache. More... | |
int | getValidLeft () const |
int | getValidWidth () const |
int | getValidRight () const |
QRect | getValidArea () const |
ZoomLevel | getZoomLevel () const |
void | setZoomLevel (ZoomLevel zoom) |
Set the zoom level. More... | |
sv_frame_t | getStartFrame () const |
void | setStartFrame (sv_frame_t frame) |
Set the start frame. More... | |
const QImage & | getImage () const |
void | scrollTo (const LayerGeometryProvider *v, sv_frame_t newStartFrame) |
Set the new start frame for the cache, according to the geometry of the supplied LayerGeometryProvider, if possible also moving along any existing valid data within the cache so that it continues to be valid for the new start frame. More... | |
void | adjustToTouchValidArea (int &left, int &width, bool &isLeftOfValidArea) const |
Take a left coordinate and width describing a region, and adjust them so that they are contiguous with the cache valid region and so that the union of the adjusted region with the cache valid region contains the supplied region. More... | |
void | drawImage (int left, int width, QImage image, int imageLeft, int imageWidth) |
Draw from an image onto the cache. More... | |
Private Attributes | |
QImage | m_image |
int | m_validLeft |
int | m_validWidth |
sv_frame_t | m_startFrame |
ZoomLevel | m_zoomLevel |
Detailed Description
A cached image for a view that scrolls horizontally, such as a spectrogram.
The cache object holds an image, reports the size of the image (likely the same as the underlying view, but it's the caller's responsibility to set the size appropriately), can scroll the image, and can report and update which contiguous horizontal range of the image is valid.
The only way to update the valid area in a cache is to draw to it using the drawImage call.
Definition at line 37 of file ScrollableImageCache.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 40 of file ScrollableImageCache.h.
Member Function Documentation
|
inline |
Definition at line 46 of file ScrollableImageCache.h.
References m_validWidth.
Referenced by resize(), setStartFrame(), and setZoomLevel().
|
inline |
Definition at line 50 of file ScrollableImageCache.h.
References m_validWidth.
|
inline |
|
inline |
Set the size of the cache.
If the new size differs from the current size, the cache is invalidated.
Definition at line 62 of file ScrollableImageCache.h.
References getSize(), invalidate(), and m_image.
|
inline |
Definition at line 69 of file ScrollableImageCache.h.
References m_validLeft.
|
inline |
Definition at line 73 of file ScrollableImageCache.h.
References m_validWidth.
|
inline |
Definition at line 77 of file ScrollableImageCache.h.
References m_validLeft, and m_validWidth.
|
inline |
Definition at line 81 of file ScrollableImageCache.h.
References m_image, m_validLeft, and m_validWidth.
|
inline |
Definition at line 85 of file ScrollableImageCache.h.
References m_zoomLevel.
|
inline |
Set the zoom level.
If the new zoom level differs from the current one, the cache is invalidated. (Determining whether to invalidate the cache here is the only thing the zoom level is used for.)
Definition at line 95 of file ScrollableImageCache.h.
References invalidate(), and m_zoomLevel.
|
inline |
Definition at line 103 of file ScrollableImageCache.h.
References m_startFrame.
|
inline |
Set the start frame.
If the new start frame differs from the current one, the cache is invalidated. To scroll, i.e. to set the start frame while retaining cache validity where possible, use scrollTo() instead.
Definition at line 113 of file ScrollableImageCache.h.
References invalidate(), and m_startFrame.
|
inline |
Definition at line 120 of file ScrollableImageCache.h.
References adjustToTouchValidArea(), drawImage(), m_image, and scrollTo().
void ScrollableImageCache::scrollTo | ( | const LayerGeometryProvider * | v, |
sv_frame_t | newStartFrame | ||
) |
Set the new start frame for the cache, according to the geometry of the supplied LayerGeometryProvider, if possible also moving along any existing valid data within the cache so that it continues to be valid for the new start frame.
Definition at line 25 of file ScrollableImageCache.cpp.
References LayerGeometryProvider::getXForFrame().
Referenced by getImage().
void ScrollableImageCache::adjustToTouchValidArea | ( | int & | left, |
int & | width, | ||
bool & | isLeftOfValidArea | ||
) | const |
Take a left coordinate and width describing a region, and adjust them so that they are contiguous with the cache valid region and so that the union of the adjusted region with the cache valid region contains the supplied region.
Does not modify anything about the cache, only about the arguments.
Definition at line 114 of file ScrollableImageCache.cpp.
Referenced by getImage().
void ScrollableImageCache::drawImage | ( | int | left, |
int | width, | ||
QImage | image, | ||
int | imageLeft, | ||
int | imageWidth | ||
) |
Draw from an image onto the cache.
The supplied image must have the same height as the cache and the full height is always drawn. The left and width parameters determine the target region of the cache, the imageLeft and imageWidth parameters the source region of the image.
Definition at line 143 of file ScrollableImageCache.cpp.
Referenced by getImage().
Member Data Documentation
|
private |
Definition at line 156 of file ScrollableImageCache.h.
Referenced by getImage(), getSize(), getValidArea(), and resize().
|
private |
Definition at line 157 of file ScrollableImageCache.h.
Referenced by getValidArea(), getValidLeft(), and getValidRight().
|
private |
Definition at line 158 of file ScrollableImageCache.h.
Referenced by getValidArea(), getValidRight(), getValidWidth(), invalidate(), and isValid().
|
private |
Definition at line 159 of file ScrollableImageCache.h.
Referenced by getStartFrame(), and setStartFrame().
|
private |
Definition at line 160 of file ScrollableImageCache.h.
Referenced by getZoomLevel(), and setZoomLevel().
The documentation for this class was generated from the following files:
Generated by
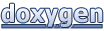