svgui
1.9
|
RenderTimer.h
Go to the documentation of this file.
An operation that the user might accept being slower.
Definition: RenderTimer.h:28
bool outOfTime(double fractionComplete)
Return true if we have run out of time and should suspend rendering and handle user events instead...
Definition: RenderTimer.h:67
std::chrono::time_point< std::chrono::steady_clock > m_start
Definition: RenderTimer.h:104
Definition: RenderTimer.h:20
double secondsPerItem(int itemsRendered) const
Definition: RenderTimer.h:93
A normal rendering operation with normal responsiveness demands.
Definition: RenderTimer.h:25
Generated by
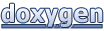