svgui
1.9
|
#include <Colour3DPlotRenderer.h>

Classes | |
struct | Parameters |
struct | RenderResult |
struct | Sources |
Public Member Functions | |
Colour3DPlotRenderer (Sources sources, Parameters parameters) | |
RenderResult | render (const LayerGeometryProvider *v, QPainter &paint, QRect rect) |
Render the requested area using the given painter, obtaining geometry (e.g. More... | |
RenderResult | renderTimeConstrained (const LayerGeometryProvider *v, QPainter &paint, QRect rect) |
Render the requested area using the given painter, obtaining geometry (e.g. More... | |
QRect | getLargestUncachedRect (const LayerGeometryProvider *v) |
Return the area of the largest rectangle within the entire area of the cache that is unavailable in the cache. More... | |
bool | geometryChanged (const LayerGeometryProvider *v) |
Return true if the provider's geometry differs from the cache, or if we are not using a cache. More... | |
bool | willRenderOpaque (const LayerGeometryProvider *v) |
Return true if the rendering will be opaque. More... | |
QColor | getColour (double value) const |
Return the colour corresponding to the given value. More... | |
QRect | findSimilarRegionExtents (QPoint point) const |
Return the enclosing rectangle for the region of similar colour to the given point within the cache. More... | |
Private Types | |
enum | RenderType { DrawBufferPixelResolution, DrawBufferBinResolution, DirectTranslucent } |
Private Member Functions | |
RenderResult | render (const LayerGeometryProvider *v, QPainter &paint, QRect rect, bool timeConstrained) |
MagnitudeRange | renderDirectTranslucent (const LayerGeometryProvider *v, QPainter &paint, QRect rect) |
void | renderToCachePixelResolution (const LayerGeometryProvider *v, int x0, int repaintWidth, bool rightToLeft, bool timeConstrained) |
void | renderToCacheBinResolution (const LayerGeometryProvider *v, int x0, int repaintWidth) |
int | renderDrawBuffer (int w, int h, const std::vector< int > &binforx, const std::vector< double > &binfory, int peakCacheIndex, bool rightToLeft, bool timeConstrained) |
int | renderDrawBufferPeakFrequencies (const LayerGeometryProvider *v, int w, int h, const std::vector< int > &binforx, const std::vector< double > &binfory, bool rightToLeft, bool timeConstrained) |
void | recreateDrawBuffer (int w, int h) |
void | clearDrawBuffer (int w, int h) |
RenderType | decideRenderType (const LayerGeometryProvider *) const |
QImage | scaleDrawBufferImage (QImage source, int targetWidth, int targetHeight) const |
ColumnOp::Column | getColumn (int sx, int minbin, int nbins, std::shared_ptr< DenseThreeDimensionalModel > source) const |
ColumnOp::Column | getColumnRaw (int sx, int minbin, int nbins, std::shared_ptr< DenseThreeDimensionalModel > source) const |
void | getPreferredPeakCache (const LayerGeometryProvider *, int &peakCacheIndex, int &binsPerPeak) const |
void | updateTimings (const RenderTimer &timer, int xPixelCount) |
Private Attributes | |
Sources | m_sources |
Parameters | m_params |
QImage | m_drawBuffer |
std::vector< MagnitudeRange > | m_magRanges |
ScrollableImageCache | m_cache |
ScrollableMagRangeCache | m_magCache |
double | m_secondsPerXPixel |
bool | m_secondsPerXPixelValid |
Detailed Description
Definition at line 49 of file Colour3DPlotRenderer.h.
Member Enumeration Documentation
|
private |
Enumerator | |
---|---|
DrawBufferPixelResolution | |
DrawBufferBinResolution | |
DirectTranslucent |
Definition at line 315 of file Colour3DPlotRenderer.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 119 of file Colour3DPlotRenderer.h.
Member Function Documentation
Colour3DPlotRenderer::RenderResult Colour3DPlotRenderer::render | ( | const LayerGeometryProvider * | v, |
QPainter & | paint, | ||
QRect | rect | ||
) |
Render the requested area using the given painter, obtaining geometry (e.g.
start frame) from the given LayerGeometryProvider.
The whole of the supplied rect will be rendered and the returned QRect will be equal to the supplied QRect. (See renderTimeConstrained for an alternative that may render only part of the rect in cases where obtaining source data is slow and retaining responsiveness is important.)
Note that Colour3DPlotRenderer retains internal cache state related to the size and position of the supplied LayerGeometryProvider. Although it is valid to call render() successively on the same Colour3DPlotRenderer with different LayerGeometryProviders, it will be much faster to use a dedicated Colour3DPlotRenderer for each LayerGeometryProvider.
If the model to render from is not ready, this will throw a std::logic_error exception. The model must be ready and the layer requesting the render must not be dormant in its view, so that the LayerGeometryProvider returns valid results; it is the caller's responsibility to ensure these.
Definition at line 44 of file Colour3DPlotRenderer.cpp.
Referenced by Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
Colour3DPlotRenderer::RenderResult Colour3DPlotRenderer::renderTimeConstrained | ( | const LayerGeometryProvider * | v, |
QPainter & | paint, | ||
QRect | rect | ||
) |
Render the requested area using the given painter, obtaining geometry (e.g.
start frame) from the stored LayerGeometryProvider.
As much of the rect will be rendered as can be managed given internal time constraints (using a RenderTimer object internally). The returned QRect (the rendered field in the RenderResult struct) will contain the area that was rendered. Note that we always render the full requested height, it's only width that is time-constrained.
Note that Colour3DPlotRenderer retains internal cache state related to the size and position of the supplied LayerGeometryProvider. Although it is valid to call render() successively on the same Colour3DPlotRenderer with different LayerGeometryProviders, it will be much faster to use a dedicated Colour3DPlotRenderer for each LayerGeometryProvider.
If the model to render from is not ready, this will throw a std::logic_error exception. The model must be ready and the layer requesting the render must not be dormant in its view, so that the LayerGeometryProvider returns valid results; it is the caller's responsibility to ensure these.
Definition at line 50 of file Colour3DPlotRenderer.cpp.
Referenced by Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
QRect Colour3DPlotRenderer::getLargestUncachedRect | ( | const LayerGeometryProvider * | v | ) |
Return the area of the largest rectangle within the entire area of the cache that is unavailable in the cache.
This is only valid in relation to a preceding render() call which is presumed to have set the area, start frame, and zoom level for the cache. It could be used to establish a suitable region for a subsequent paint request (because if an area is not in the cache, it cannot have been rendered since the cache was cleared).
Returns an empty QRect if the cache is entirely valid.
Definition at line 57 of file Colour3DPlotRenderer.cpp.
Referenced by Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
bool Colour3DPlotRenderer::geometryChanged | ( | const LayerGeometryProvider * | v | ) |
Return true if the provider's geometry differs from the cache, or if we are not using a cache.
i.e. if the cache will be regenerated for the next render, or the next render performed from scratch.
Definition at line 79 of file Colour3DPlotRenderer.cpp.
References LayerGeometryProvider::getPaintSize(), LayerGeometryProvider::getStartFrame(), and LayerGeometryProvider::getZoomLevel().
Referenced by Colour3DPlotLayer::paintWithRenderer(), and SpectrogramLayer::paintWithRenderer().
|
inline |
Return true if the rendering will be opaque.
This may be used by the calling layer to determine whether it can scroll directly without regard to any other layers beneath.
Definition at line 228 of file Colour3DPlotRenderer.h.
|
inline |
Return the colour corresponding to the given value.
Definition at line 237 of file Colour3DPlotRenderer.h.
QRect Colour3DPlotRenderer::findSimilarRegionExtents | ( | QPoint | point | ) | const |
Return the enclosing rectangle for the region of similar colour to the given point within the cache.
Return an empty QRect if this is not possible.
- See also
- ImageRegionFinder
Definition at line 1429 of file Colour3DPlotRenderer.cpp.
References ImageRegionFinder::findRegionExtents().
Referenced by SpectrogramLayer::measureDoubleClick().
|
private |
Definition at line 97 of file Colour3DPlotRenderer.cpp.
References LayerGeometryProvider::getPaintSize(), LayerGeometryProvider::getPaintWidth(), LayerGeometryProvider::getStartFrame(), LayerGeometryProvider::getXForFrame(), LayerGeometryProvider::getXForViewX(), LayerGeometryProvider::getZoomLevel(), and PeakFrequencies.
|
private |
Definition at line 467 of file Colour3DPlotRenderer.cpp.
References PaintAssistant::drawVisibleText(), LayerGeometryProvider::getForeground(), LayerGeometryProvider::getFrameForX(), ViewManager::getMainModelSampleRate(), LayerGeometryProvider::getPaintHeight(), LayerGeometryProvider::getViewManager(), LayerGeometryProvider::getXForFrame(), PaintAssistant::OutlinedText, PeakBins, and LayerGeometryProvider::shouldIlluminateLocalFeatures().
|
private |
Definition at line 678 of file Colour3DPlotRenderer.cpp.
References LayerGeometryProvider::getFrameForX(), LayerGeometryProvider::getPaintHeight(), and PeakFrequencies.
|
private |
Definition at line 832 of file Colour3DPlotRenderer.cpp.
References LayerGeometryProvider::getFrameForX(), LayerGeometryProvider::getPaintHeight(), and LayerGeometryProvider::getXForFrame().
|
private |
Definition at line 1006 of file Colour3DPlotRenderer.cpp.
References RenderTimer::FastRender, RenderTimer::NoTimeout, RenderTimer::outOfTime(), and PeakBins.
|
private |
Definition at line 1206 of file Colour3DPlotRenderer.cpp.
References LayerGeometryProvider::getYForFrequency(), Log, RenderTimer::NoTimeout, RenderTimer::outOfTime(), and RenderTimer::SlowRender.
|
private |
Definition at line 1402 of file Colour3DPlotRenderer.cpp.
|
private |
Definition at line 1418 of file Colour3DPlotRenderer.cpp.
|
private |
Definition at line 366 of file Colour3DPlotRenderer.cpp.
References ViewManager::getMainModelSampleRate(), LayerGeometryProvider::getPaintHeight(), LayerGeometryProvider::getViewManager(), LayerGeometryProvider::getZoomLevel(), and PeakFrequencies.
|
private |
Definition at line 760 of file Colour3DPlotRenderer.cpp.
|
private |
Definition at line 406 of file Colour3DPlotRenderer.cpp.
References Phase.
|
private |
Definition at line 442 of file Colour3DPlotRenderer.cpp.
References Phase.
|
private |
Definition at line 629 of file Colour3DPlotRenderer.cpp.
References LayerGeometryProvider::getZoomLevel(), PeakFrequencies, and Phase.
|
private |
Definition at line 1379 of file Colour3DPlotRenderer.cpp.
References RenderTimer::secondsPerItem().
Member Data Documentation
|
private |
Definition at line 249 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 250 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 256 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 262 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 271 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 280 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 282 of file Colour3DPlotRenderer.h.
|
private |
Definition at line 283 of file Colour3DPlotRenderer.h.
The documentation for this class was generated from the following files:
Generated by
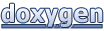