svgui
1.9
|
Colour3DPlotLayer.cpp
Go to the documentation of this file.
792 // SVDEBUG << "Colour3DPlotLayer::setVerticalZoomStep(" <<step <<"): before: miny = " << m_miny << ", maxy = " << m_maxy << endl;
804 // SVDEBUG << "Colour3DPlotLayer::setVerticalZoomStep(" <<step <<"): after: miny = " << m_miny << ", maxy = " << m_maxy << endl;
966 Colour3DPlotLayer::paintVerticalScale(LayerGeometryProvider *v, bool, QPainter &paint, QRect rect) const
1223 SVDEBUG << "Colour3DPlotLayer::paint: model says shouldUseLogValueScale = " << model->shouldUseLogValueScale() << endl;
1228 SVDEBUG << "Colour3DPlotLayer::paint(): m_model is " << m_model << ", zoom level is " << v->getZoomLevel() << ", rect is (" << rect.x() << "," << rect.y() << ") " << rect.width() << "x" << rect.height() << endl;
Definition: ColourScale.h:37
double threshold
Threshold below which every value is mapped to background pixel 0.
Definition: ColourScale.h:59
QString getPropertyValueIconName(const PropertyName &, int value) const override
Definition: Colour3DPlotLayer.cpp:480
virtual bool isLayerDormant(const LayerGeometryProvider *v) const
Return whether the layer is dormant (i.e.
Definition: Layer.cpp:144
bool getDisplayExtents(double &min, double &max) const override
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: Colour3DPlotLayer.cpp:726
int getCurrentVerticalZoomStep() const override
Get the current vertical zoom step.
Definition: Colour3DPlotLayer.cpp:776
virtual bool snapToFeatureFrame(LayerGeometryProvider *, sv_frame_t &, int &resolution, SnapType, int) const
Adjust the given frame to snap to the nearest feature, if possible.
Definition: Layer.h:226
static std::pair< ColumnNormalization, bool > convertToColumnNorm(int value)
Definition: Colour3DPlotLayer.cpp:120
void invalidateRenderers()
Definition: Colour3DPlotLayer.cpp:201
static int convertFromColourScale(ColourScaleType)
Definition: Colour3DPlotLayer.cpp:105
ModelId getExportModel(LayerGeometryProvider *) const override
Return the ID of a model representing the contents of this layer in a form suitable for export to a t...
Definition: Colour3DPlotLayer.cpp:220
virtual QColor getForeground() const =0
const LayerGeometryProvider * provider
Definition: Colour3DPlotExporter.h:31
ColourScale colourScale
A complete ColourScale object by value, used for colour map conversion.
Definition: Colour3DPlotRenderer.h:78
PropertyType getPropertyType(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:329
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Convert the layer's data (though not those of the model it refers to) into XML for file output...
Definition: Layer.cpp:653
virtual ZoomLevel getZoomLevel() const =0
Return the zoom level, i.e.
RenderResult renderTimeConstrained(const LayerGeometryProvider *v, QPainter &paint, QRect rect)
Render the requested area using the given painter, obtaining geometry (e.g.
Definition: Colour3DPlotRenderer.cpp:50
QString getPropertyLabel(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:306
virtual int getIBinForY(const LayerGeometryProvider *v, int y) const
As getBinForY, but rounding to integer values.
Definition: VerticalBinLayer.h:57
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: Colour3DPlotLayer.cpp:1218
void modelReplaced()
void setVerticalZoomStep(int) override
Set the vertical zoom step.
Definition: Colour3DPlotLayer.cpp:787
Definition: ColourMapper.h:40
void setProperty(const PropertyName &, int value) override
Definition: Colour3DPlotLayer.cpp:505
int getCompletion(LayerGeometryProvider *) const override
Return the proportion of background work complete in drawing this view, as a percentage – in most ca...
Definition: Colour3DPlotLayer.cpp:702
Map values within a range onto a set of colours, with a given distribution (linear, log etc) and optional colourmap rotation.
Definition: ColourScale.h:34
virtual void setLayerDormant(const LayerGeometryProvider *v, bool dormant)
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: Layer.cpp:136
sv_samplerate_t getMainModelSampleRate() const
The sample rate of the current main model.
Definition: ViewManager.h:190
int getVerticalScaleWidth(LayerGeometryProvider *v, bool, QPainter &) const override
Definition: Colour3DPlotLayer.cpp:938
bool isLayerScrollable(const LayerGeometryProvider *v) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: Colour3DPlotLayer.cpp:693
virtual sv_frame_t getFrameForX(int x) const =0
Return the closest frame to the given pixel x-coordinate.
bool geometryChanged(const LayerGeometryProvider *v)
Return true if the provider's geometry differs from the cache, or if we are not using a cache...
Definition: Colour3DPlotRenderer.cpp:79
double getYForBin(const LayerGeometryProvider *, double bin) const override
Return the y coordinate at which the given bin "starts" (i.e.
Definition: Colour3DPlotLayer.cpp:823
void handleModelChanged(ModelId)
Definition: Colour3DPlotLayer.cpp:256
Definition: Layer.h:198
static int getColourMapCount()
Return the number of known colour maps.
Definition: ColourMapper.cpp:142
void setInvertVertical(bool i)
Definition: Colour3DPlotLayer.cpp:617
bool getInvertVertical() const
Definition: Colour3DPlotLayer.cpp:644
static int getBackwardCompatibilityColourMap(int n)
Older versions of colour-handling code save and reload colour maps by numerical index and can't prope...
Definition: ColourMapper.cpp:232
void modelChanged(ModelId)
bool snapToFeatureFrame(LayerGeometryProvider *v, sv_frame_t &frame, int &resolution, SnapType snap, int ycoord) const override
Adjust the given frame to snap to the nearest feature, if possible.
Definition: Colour3DPlotLayer.cpp:1252
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
static QString getColourMapLabel(int n)
Return a human-readable label for the colour map with the given index.
Definition: ColourMapper.cpp:148
Definition: Colour3DPlotRenderer.h:49
bool interpolate
Whether to apply smoothing when rendering cells at more than one pixel per cell.
Definition: Colour3DPlotRenderer.h:100
void setGain(float gain)
Set the gain multiplier for sample values in this view.
Definition: Colour3DPlotLayer.cpp:552
bool hasLightBackground() const
Return true if the colour map is intended to be placed over a light background, false otherwise...
Definition: ColourMapper.cpp:488
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: Colour3DPlotLayer.cpp:1280
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: Colour3DPlotLayer.cpp:360
virtual QRect getPaintRect() const =0
To be called from a layer, to obtain the extent of the surface that the layer is currently painting t...
ViewMagMap m_lastRenderedMags
Definition: Colour3DPlotLayer.h:201
static int getColourMapById(QString id)
Return the index for the colour map with the given machine-readable id string, or -1 if the id is not...
Definition: ColourMapper.cpp:204
void layerParametersChanged()
static ColourScaleType convertToColourScale(int value)
Definition: Colour3DPlotLayer.cpp:93
void invalidateMagnitudes()
Definition: Colour3DPlotLayer.cpp:211
RenderResult render(const LayerGeometryProvider *v, QPainter &paint, QRect rect)
Render the requested area using the given painter, obtaining geometry (e.g.
Definition: Colour3DPlotRenderer.cpp:44
bool hasLightBackground() const override
Definition: Colour3DPlotLayer.cpp:662
void setSynchronousPainting(bool synchronous) override
Enable or disable synchronous painting.
Definition: Colour3DPlotLayer.cpp:147
int getVerticalZoomSteps(int &defaultStep) const override
Get the number of vertical zoom steps available for this layer.
Definition: Colour3DPlotLayer.cpp:765
Definition: Layer.h:197
QString getPropertyIconName(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:320
A class for mapping intensity values onto various colour maps.
Definition: ColourMapper.h:27
void setColourScale(ColourScaleType)
Definition: Colour3DPlotLayer.cpp:533
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
int getColourScaleWidth(QPainter &) const
Definition: Colour3DPlotLayer.cpp:930
ColumnNormalization normalization
Type of column normalization.
Definition: Colour3DPlotRenderer.h:81
bool getNormalizeVisibleArea() const
Definition: Colour3DPlotLayer.cpp:611
PropertyList getProperties() const override
Definition: Colour3DPlotLayer.cpp:291
bool invertVertical
Whether to render the whole caboodle upside-down.
Definition: Colour3DPlotRenderer.h:103
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: Colour3DPlotLayer.cpp:865
void paintWithRenderer(LayerGeometryProvider *v, QPainter &paint, QRect rect) const
Definition: Colour3DPlotLayer.cpp:1163
virtual void updatePaintRect(QRect r)=0
const ZoomConstraint * getZoomConstraint() const override
Return a zoom constraint object defining the supported zoom levels for this layer.
Definition: Colour3DPlotLayer.cpp:85
Definition: Layer.h:196
void setNormalizeVisibleArea(bool)
Specify whether to normalize the visible area.
Definition: Colour3DPlotLayer.cpp:599
virtual ViewManager * getViewManager() const =0
virtual int getIYForBin(const LayerGeometryProvider *v, int bin) const
As getYForBin, but rounding to integer values.
Definition: VerticalBinLayer.h:42
void setProperties(const QXmlAttributes &) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: Colour3DPlotLayer.cpp:1332
void invalidatePeakCache()
Definition: Colour3DPlotLayer.cpp:188
QRect getLargestUncachedRect(const LayerGeometryProvider *v)
Return the area of the largest rectangle within the entire area of the cache that is unavailable in t...
Definition: Colour3DPlotRenderer.cpp:57
bool m_normalizeVisibleArea
Definition: Colour3DPlotLayer.h:174
const int m_peakCacheDivisor
Definition: Colour3DPlotLayer.h:193
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: Colour3DPlotLayer.cpp:444
static int convertFromColumnNorm(ColumnNormalization norm, bool visible)
Definition: Colour3DPlotLayer.cpp:132
bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: Colour3DPlotLayer.cpp:746
Definition: PaintAssistant.h:43
const VerticalBinLayer * verticalBinLayer
Definition: Colour3DPlotExporter.h:28
static QString getColourMapId(int n)
Return a machine-readable id string for the colour map with the given index.
Definition: ColourMapper.cpp:177
void paintVerticalScale(LayerGeometryProvider *v, bool, QPainter &paint, QRect rect) const override
Definition: Colour3DPlotLayer.cpp:966
static void drawVisibleText(const LayerGeometryProvider *, QPainter &p, int x, int y, QString text, TextStyle style)
Definition: PaintAssistant.cpp:199
MagnitudeRange range
The magnitude range of the data in the rendered area, after initial scaling (parameters.scaleFactor) and normalisation, for use in displaying colour scale etc.
Definition: Colour3DPlotRenderer.h:143
void modelChangedWithin(ModelId, sv_frame_t startFrame, sv_frame_t endFrame)
ColumnNormalization getNormalization() const
Definition: Colour3DPlotLayer.cpp:593
RangeMapper * getNewVerticalZoomRangeMapper() const override
Create and return a range mapper for vertical zoom step values.
Definition: Colour3DPlotLayer.cpp:810
Colour3DPlotRenderer * getRenderer(const LayerGeometryProvider *) const
Definition: Colour3DPlotLayer.cpp:1090
void setLayerDormant(const LayerGeometryProvider *v, bool dormant) override
Indicate that a layer is not currently visible in the given view and is not expected to become visibl...
Definition: Colour3DPlotLayer.cpp:669
const VerticalBinLayer * verticalBinLayer
Definition: Colour3DPlotRenderer.h:56
bool getYScaleValue(const LayerGeometryProvider *, int, double &, QString &) const override
Return the value and unit at the given y coordinate in the given view.
Definition: Colour3DPlotLayer.cpp:758
void handleModelChangedWithin(ModelId, sv_frame_t, sv_frame_t)
Definition: Colour3DPlotLayer.cpp:273
std::vector< ModelId > peakCaches
Definition: Colour3DPlotRenderer.h:59
BinScale binScale
Scale for vertical bin spacing (linear or logarithmic).
Definition: Colour3DPlotRenderer.h:87
virtual int getPaintWidth() const
Definition: LayerGeometryProvider.h:187
void setNormalization(ColumnNormalization)
Specify the normalization mode for individual columns.
Definition: Colour3DPlotLayer.cpp:582
bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: Colour3DPlotLayer.cpp:710
double getBinForY(const LayerGeometryProvider *, double y) const override
Return the bin number, possibly fractional, at the given y coordinate.
Definition: Colour3DPlotLayer.cpp:842
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:496
ColumnNormalization m_normalization
Definition: Colour3DPlotLayer.h:173
QString getPropertyGroupName(const PropertyName &) const override
Definition: Colour3DPlotLayer.cpp:340
Generated by
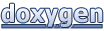