svgui
1.9
|
ColourMapper.h
Go to the documentation of this file.
Definition: ColourMapper.h:52
Definition: ColourMapper.h:44
Definition: ColourMapper.h:40
Definition: ColourMapper.h:43
Definition: ColourMapper.h:48
static int getColourMapCount()
Return the number of known colour maps.
Definition: ColourMapper.cpp:142
Definition: ColourMapper.h:42
static int getBackwardCompatibilityColourMap(int n)
Older versions of colour-handling code save and reload colour maps by numerical index and can't prope...
Definition: ColourMapper.cpp:232
static QString getColourMapLabel(int n)
Return a human-readable label for the colour map with the given index.
Definition: ColourMapper.cpp:148
Definition: ColourMapper.h:41
Definition: ColourMapper.h:47
Definition: ColourMapper.h:49
QPixmap getExamplePixmap(QSize size) const
Return a pixmap of the given size containing a preview swatch for the colour map. ...
Definition: ColourMapper.cpp:519
bool hasLightBackground() const
Return true if the colour map is intended to be placed over a light background, false otherwise...
Definition: ColourMapper.cpp:488
Definition: ColourMapper.h:54
static int getColourMapById(QString id)
Return the index for the colour map with the given machine-readable id string, or -1 if the id is not...
Definition: ColourMapper.cpp:204
Definition: ColourMapper.h:50
Definition: ColourMapper.h:45
A class for mapping intensity values onto various colour maps.
Definition: ColourMapper.h:27
Definition: ColourMapper.h:46
Definition: ColourMapper.h:53
ColourMapper & operator=(const ColourMapper &)=default
Definition: ColourMapper.h:51
static QString getColourMapId(int n)
Return a machine-readable id string for the colour map with the given index.
Definition: ColourMapper.cpp:177
ColourMapper(int map, bool inverted, double minValue, double maxValue)
Definition: ColourMapper.cpp:124
QColor getContrastingColour() const
Return a colour that contrasts somewhat with the colours in the map, so as to be used for cursors etc...
Definition: ColourMapper.cpp:431
Generated by
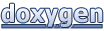