svgui
1.9
|
A class for mapping intensity values onto various colour maps. More...
#include <ColourMapper.h>
Public Types | |
enum | ColourMap { Green, Sunset, WhiteOnBlack, BlackOnWhite, Cherry, Wasp, Ice, FruitSalad, Banded, Highlight, Printer, HighGain, BlueOnBlack, Cividis, Magma } |
Public Member Functions | |
ColourMapper (int map, bool inverted, double minValue, double maxValue) | |
~ColourMapper () | |
ColourMapper (const ColourMapper &)=default | |
ColourMapper & | operator= (const ColourMapper &)=default |
int | getMap () const |
bool | isInverted () const |
double | getMinValue () const |
double | getMaxValue () const |
QColor | map (double value) const |
Map the given value to a colour. More... | |
QColor | getContrastingColour () const |
Return a colour that contrasts somewhat with the colours in the map, so as to be used for cursors etc. More... | |
bool | hasLightBackground () const |
Return true if the colour map is intended to be placed over a light background, false otherwise. More... | |
QPixmap | getExamplePixmap (QSize size) const |
Return a pixmap of the given size containing a preview swatch for the colour map. More... | |
Static Public Member Functions | |
static int | getColourMapCount () |
Return the number of known colour maps. More... | |
static QString | getColourMapLabel (int n) |
Return a human-readable label for the colour map with the given index. More... | |
static QString | getColourMapId (int n) |
Return a machine-readable id string for the colour map with the given index. More... | |
static int | getColourMapById (QString id) |
Return the index for the colour map with the given machine-readable id string, or -1 if the id is not recognised. More... | |
static int | getBackwardCompatibilityColourMap (int n) |
Older versions of colour-handling code save and reload colour maps by numerical index and can't properly handle situations in which the index order changes between releases, or new indices are added. More... | |
Protected Attributes | |
int | m_map |
bool | m_inverted |
double | m_min |
double | m_max |
Detailed Description
A class for mapping intensity values onto various colour maps.
Definition at line 27 of file ColourMapper.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Green | |
Sunset | |
WhiteOnBlack | |
BlackOnWhite | |
Cherry | |
Wasp | |
Ice | |
FruitSalad | |
Banded | |
Highlight | |
Printer | |
HighGain | |
BlueOnBlack | |
Cividis | |
Magma |
Definition at line 39 of file ColourMapper.h.
Constructor & Destructor Documentation
ColourMapper::ColourMapper | ( | int | map, |
bool | inverted, | ||
double | minValue, | ||
double | maxValue | ||
) |
Definition at line 124 of file ColourMapper.cpp.
ColourMapper::~ColourMapper | ( | ) |
Definition at line 137 of file ColourMapper.cpp.
|
default |
Member Function Documentation
|
default |
|
inline |
Definition at line 57 of file ColourMapper.h.
References m_map.
|
inline |
Definition at line 58 of file ColourMapper.h.
References m_inverted.
|
inline |
Definition at line 59 of file ColourMapper.h.
References m_min.
|
inline |
Definition at line 60 of file ColourMapper.h.
References getBackwardCompatibilityColourMap(), getColourMapById(), getColourMapCount(), getColourMapId(), getColourMapLabel(), getContrastingColour(), getExamplePixmap(), hasLightBackground(), m_max, and map().
|
static |
Return the number of known colour maps.
Definition at line 142 of file ColourMapper.cpp.
Referenced by getBackwardCompatibilityColourMap(), getColourMapById(), getColourMapId(), getColourMapLabel(), getContrastingColour(), getMaxValue(), SliceLayer::getPropertyRangeAndValue(), TimeValueLayer::getPropertyRangeAndValue(), RegionLayer::getPropertyRangeAndValue(), Colour3DPlotLayer::getPropertyRangeAndValue(), SpectrogramLayer::getPropertyRangeAndValue(), hasLightBackground(), map(), ColourMapComboBox::rebuild(), SliceLayer::setProperties(), Colour3DPlotLayer::setProperties(), RegionLayer::setProperties(), TimeValueLayer::setProperties(), and SpectrogramLayer::setProperties().
|
static |
Return a human-readable label for the colour map with the given index.
This may have been subject to translation.
Definition at line 148 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, Ice, Magma, map(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by getMaxValue(), SliceLayer::getPropertyValueLabel(), TimeValueLayer::getPropertyValueLabel(), RegionLayer::getPropertyValueLabel(), Colour3DPlotLayer::getPropertyValueLabel(), SpectrogramLayer::getPropertyValueLabel(), and ColourMapComboBox::rebuild().
|
static |
Return a machine-readable id string for the colour map with the given index.
This is not translated and is intended for use in file I/O.
Definition at line 177 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, Ice, Magma, map(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by getMaxValue(), SliceLayer::toXml(), RegionLayer::toXml(), TimeValueLayer::toXml(), Colour3DPlotLayer::toXml(), and SpectrogramLayer::toXml().
|
static |
Return the index for the colour map with the given machine-readable id string, or -1 if the id is not recognised.
Definition at line 204 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, Ice, Magma, map(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by getMaxValue(), SliceLayer::setProperties(), Colour3DPlotLayer::setProperties(), RegionLayer::setProperties(), TimeValueLayer::setProperties(), and SpectrogramLayer::setProperties().
|
static |
Older versions of colour-handling code save and reload colour maps by numerical index and can't properly handle situations in which the index order changes between releases, or new indices are added.
So when we save a colour map by id, we should also save a compatibility value that can be re-read by such code. This value is an index into the series of colours used by pre-3.2 SV code, namely (Default/Green, Sunset, WhiteOnBlack, BlackOnWhite, RedOnBlue, YellowOnBlack, BlueOnBlack, FruitSalad, Banded, Highlight, Printer, HighGain). It should represent the closest equivalent to the current colour scheme available in that set. This function returns that index.
Definition at line 232 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, Ice, Magma, map(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by getMaxValue(), SliceLayer::toXml(), RegionLayer::toXml(), TimeValueLayer::toXml(), Colour3DPlotLayer::toXml(), and SpectrogramLayer::toXml().
QColor ColourMapper::map | ( | double | value | ) | const |
Map the given value to a colour.
The value will be clamped to the range minValue to maxValue (where both are drawn from the constructor arguments).
Definition at line 265 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, ice, Ice, m_inverted, m_map, m_max, m_min, Magma, mapDiscrete(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by getBackwardCompatibilityColourMap(), ColourScale::getColourForPixel(), RegionLayer::getColourForValue(), TimeValueLayer::getColourForValue(), getColourMapById(), getColourMapId(), getColourMapLabel(), getContrastingColour(), getExamplePixmap(), getMaxValue(), hasLightBackground(), SliceLayer::paint(), and SpectrumLayer::paint().
QColor ColourMapper::getContrastingColour | ( | ) | const |
Return a colour that contrasts somewhat with the colours in the map, so as to be used for cursors etc.
Definition at line 431 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, Ice, m_map, Magma, map(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by getMaxValue(), SpectrogramLayer::getRenderer(), and SpectrumLayer::paintCrosshairs().
bool ColourMapper::hasLightBackground | ( | ) | const |
Return true if the colour map is intended to be placed over a light background, false otherwise.
This is typically true if the colours corresponding to higher values are darker than those corresponding to lower values.
Definition at line 488 of file ColourMapper.cpp.
References Banded, BlackOnWhite, BlueOnBlack, Cherry, Cividis, FruitSalad, getColourMapCount(), Green, HighGain, Highlight, Ice, m_map, Magma, map(), Printer, Sunset, Wasp, and WhiteOnBlack.
Referenced by ColourScale::getColourForPixel(), getMaxValue(), SliceLayer::hasLightBackground(), SpectrogramLayer::hasLightBackground(), and Colour3DPlotLayer::hasLightBackground().
QPixmap ColourMapper::getExamplePixmap | ( | QSize | size | ) | const |
Return a pixmap of the given size containing a preview swatch for the colour map.
Definition at line 519 of file ColourMapper.cpp.
References m_max, m_min, and map().
Referenced by getMaxValue(), and ColourMapComboBox::rebuild().
Member Data Documentation
|
protected |
Definition at line 129 of file ColourMapper.h.
Referenced by getContrastingColour(), getMap(), hasLightBackground(), and map().
|
protected |
Definition at line 130 of file ColourMapper.h.
Referenced by isInverted(), and map().
|
protected |
Definition at line 131 of file ColourMapper.h.
Referenced by ColourMapper(), getExamplePixmap(), getMinValue(), and map().
|
protected |
Definition at line 132 of file ColourMapper.h.
Referenced by ColourMapper(), getExamplePixmap(), getMaxValue(), and map().
The documentation for this class was generated from the following files:
Generated by
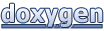