svgui
1.9
|
ColourMapComboBox.cpp
Go to the documentation of this file.
Very trivial enhancement to QComboBox to make it emit signals when the mouse enters and leaves (for c...
Definition: NotifyingComboBox.h:26
static int getColourMapCount()
Return the number of known colour maps.
Definition: ColourMapper.cpp:142
void colourMapChanged(int index)
static QString getColourMapLabel(int n)
Return a human-readable label for the colour map with the given index.
Definition: ColourMapper.cpp:148
QPixmap getExamplePixmap(QSize size) const
Return a pixmap of the given size containing a preview swatch for the colour map. ...
Definition: ColourMapper.cpp:519
A class for mapping intensity values onto various colour maps.
Definition: ColourMapper.h:27
ColourMapComboBox(bool includeSwatches, QWidget *parent=0)
Definition: ColourMapComboBox.cpp:28
Generated by
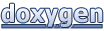