svgui
1.9
|
SpectrumLayer.cpp
Go to the documentation of this file.
736 SVDEBUG << "SpectrumLayer::paint: no origin model, or origin model not OK or not ready" << endl;
765 // cerr << "shouldIlluminate = " << shouldIlluminate << ", localPos = " << localPos.x() << "," << localPos.y() << endl;
804 // cerr << "bin = " << bin << ", thresh = " << thresh << ", value = " << fft->getMagnitudeAt(col, bin) << endl;
virtual void setProperty(const PropertyName &, int value) override
Definition: SpectrumLayer.cpp:267
virtual int getXForViewX(int viewx) const =0
Return the closest pixel x-coordinate corresponding to a given view x-coordinate. ...
void paintScale(LayerGeometryProvider *v, const HorizontalScaleProvider *provider, QPainter &paint, QRect r, bool logarithmic)
Definition: HorizontalFrequencyScale.cpp:34
bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: SliceLayer.cpp:1199
virtual bool shouldIlluminateLocalFeatures(const Layer *, QPoint &) const =0
Definition: SliceLayer.h:84
static int scalePixelSize(int pixels)
Take a "design pixel" size and scale it for the actual display.
Definition: ViewManager.cpp:857
virtual void getBiasCurve(BiasCurve &) const override
Definition: SpectrumLayer.cpp:938
virtual double getValueForY(const LayerGeometryProvider *v, double y) const
Definition: SliceLayer.cpp:372
bool hasLightBackground() const override
Return true if the layer currently has a dark colour on a light background, false if it has a light c...
Definition: SliceLayer.cpp:729
PropertyType getPropertyType(const PropertyName &) const override
Definition: SliceLayer.cpp:774
Definition: ColourMapper.h:43
virtual RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SpectrumLayer.cpp:261
Definition: SliceLayer.h:84
virtual QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: SpectrumLayer.cpp:638
QString getPropertyIconName(const PropertyName &) const override
Definition: SliceLayer.cpp:767
virtual double getXForBin(const LayerGeometryProvider *, double bin) const override
Convert a (possibly non-integral) bin into x-coord. May be overridden.
Definition: SpectrumLayer.cpp:432
Definition: PianoScale.h:25
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SliceLayer.cpp:890
virtual double getXForFrequency(const LayerGeometryProvider *, double freq) const override
Definition: SpectrumLayer.cpp:442
virtual void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: SpectrumLayer.cpp:732
Definition: ColourMapper.h:42
Definition: SpectrumLayer.h:31
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
virtual int getHorizontalScaleHeight(LayerGeometryProvider *, QPainter &) const override
Definition: SpectrumLayer.cpp:877
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SliceLayer.cpp:934
void preferenceChanged(PropertyContainer::PropertyName name)
Definition: SpectrumLayer.cpp:366
virtual QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SpectrumLayer.cpp:231
virtual double getFrequencyForX(const LayerGeometryProvider *, double x) const override
Definition: SpectrumLayer.cpp:408
int getHeight(LayerGeometryProvider *v, QPainter &paint)
Definition: HorizontalFrequencyScale.cpp:27
virtual bool getYScaleValue(const LayerGeometryProvider *, int y, double &value, QString &unit) const override
Return the value and unit at the given y coordinate in the given view.
Definition: SpectrumLayer.cpp:465
virtual int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SpectrumLayer.cpp:180
void layerParametersChanged()
Definition: SliceLayer.h:82
Definition: SliceLayer.h:88
bool shouldShowHorizontalValueScale() const
Definition: ViewManager.h:225
double getXForScalePoint(const LayerGeometryProvider *, double p, double pmin, double pmax) const
Convert a point such as a bin number into x-coord, given max & min.
Definition: SliceLayer.cpp:196
virtual double getYForValue(const LayerGeometryProvider *v, double value, double &norm) const
Definition: SliceLayer.cpp:320
virtual bool getYScaleDifference(const LayerGeometryProvider *, int y0, int y1, double &diff, QString &unit) const override
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
Definition: SpectrumLayer.cpp:491
A class for mapping intensity values onto various colour maps.
Definition: ColourMapper.h:27
virtual PropertyType getPropertyType(const PropertyName &) const override
Definition: SpectrumLayer.cpp:160
virtual double getFrequencyForBin(double bin) const
Definition: SpectrumLayer.cpp:422
virtual int getPaintHeight() const
Definition: LayerGeometryProvider.h:188
virtual double getBinForX(const LayerGeometryProvider *, double x) const override
Convert an x-coord into (possibly non-integral) bin. May be overridden.
Definition: SpectrumLayer.cpp:398
virtual void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SpectrumLayer.cpp:944
QString getPropertyLabel(const PropertyName &) const override
Definition: SliceLayer.cpp:754
void paintPianoHorizontal(LayerGeometryProvider *v, const HorizontalScaleProvider *p, QPainter &paint, QRect rect)
Definition: PianoScale.cpp:91
virtual bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: SpectrumLayer.cpp:378
double getScalePointForX(const LayerGeometryProvider *, double x, double pmin, double pmax) const
Convert an x-coord into a point such as a bin number, given max & min.
Definition: SliceLayer.cpp:271
virtual void paintCrosshairs(LayerGeometryProvider *, QPainter &, QPoint) const override
Definition: SpectrumLayer.cpp:548
virtual void paintHorizontalScale(LayerGeometryProvider *, QPainter &, int xorigin) const
Definition: SpectrumLayer.cpp:889
virtual ViewManager * getViewManager() const =0
virtual PropertyList getProperties() const override
Definition: SpectrumLayer.cpp:132
void setProperty(const PropertyName &, int value) override
Definition: SliceLayer.cpp:946
Definition: PaintAssistant.h:43
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: SliceLayer.cpp:409
static void drawVisibleText(const LayerGeometryProvider *, QPainter &p, int x, int y, QString text, TextStyle style)
Definition: PaintAssistant.cpp:199
void setProperties(const QXmlAttributes &) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SliceLayer.cpp:1111
void setOversampling(int oversampling)
Definition: SpectrumLayer.cpp:326
virtual QString getFeatureDescriptionAux(LayerGeometryProvider *v, QPoint &, bool includeBinDescription, int &minbin, int &maxbin, int &range) const
Definition: SliceLayer.cpp:101
virtual bool getXScaleValue(const LayerGeometryProvider *v, int x, double &value, QString &unit) const override
Return the value and unit at the given x coordinate in the given view.
Definition: SpectrumLayer.cpp:456
virtual sv_frame_t getCentreFrame() const =0
Return the centre frame of the visible widget.
QColor getContrastingColour() const
Return a colour that contrasts somewhat with the colours in the map, so as to be used for cursors etc...
Definition: ColourMapper.cpp:431
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SliceLayer.cpp:1072
QString getPropertyGroupName(const PropertyName &) const override
Definition: SliceLayer.cpp:788
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SliceLayer.cpp:801
virtual double getBinForFrequency(double freq) const
Definition: SpectrumLayer.cpp:388
virtual void setProperties(const QXmlAttributes &) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SpectrumLayer.cpp:960
virtual int getPaintWidth() const
Definition: LayerGeometryProvider.h:187
int getVerticalScaleWidth(LayerGeometryProvider *v, bool, QPainter &) const override
Definition: SliceLayer.cpp:665
virtual QString getPropertyGroupName(const PropertyName &) const override
Definition: SpectrumLayer.cpp:170
virtual bool getYScaleDifference(const LayerGeometryProvider *v, int y0, int y1, double &diff, QString &unit) const
Return the difference between the values at the given y coordinates in the given view, and the unit of the difference.
Definition: Layer.cpp:173
virtual QString getPropertyLabel(const PropertyName &) const override
Definition: SpectrumLayer.cpp:143
virtual bool getCrosshairExtents(LayerGeometryProvider *, QPainter &, QPoint cursorPos, std::vector< QRect > &extents) const override
Definition: SpectrumLayer.cpp:501
Definition: SliceLayer.h:82
virtual QString getPropertyIconName(const PropertyName &) const override
Definition: SpectrumLayer.cpp:153
Generated by
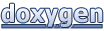