svgui
1.9
|
HorizontalFrequencyScale.cpp
Go to the documentation of this file.
void paintScale(LayerGeometryProvider *v, const HorizontalScaleProvider *provider, QPainter &paint, QRect r, bool logarithmic)
Definition: HorizontalFrequencyScale.cpp:34
virtual double getFrequencyForX(const LayerGeometryProvider *, double x) const =0
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
int getHeight(LayerGeometryProvider *v, QPainter &paint)
Definition: HorizontalFrequencyScale.cpp:27
virtual double getXForFrequency(const LayerGeometryProvider *, double freq) const =0
Interface to be implemented by objects, such as layers or objects they delegate to, in which the X axis corresponds to frequency.
Definition: HorizontalScaleProvider.h:26
Generated by
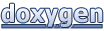