svgui
1.9
|
SliceLayer.h
Go to the documentation of this file.
44 void paintVerticalScale(LayerGeometryProvider *v, bool, QPainter &paint, QRect rect) const override;
Definition: SliceLayer.h:88
bool getDisplayExtents(double &min, double &max) const override
Return the minimum and maximum values within the visible area for the y axis of this layer...
Definition: SliceLayer.cpp:1178
float getGain() const
void paintVerticalScale(LayerGeometryProvider *v, bool, QPainter &paint, QRect rect) const override
Definition: SliceLayer.cpp:684
Definition: SliceLayer.h:170
int getCurrentVerticalZoomStep() const override
Get the current vertical zoom step.
Definition: SliceLayer.cpp:1241
virtual bool hasTimeXAxis() const override
Return true if the X axis on the layer is time proportional to audio frames, false otherwise...
Definition: SliceLayer.h:76
bool getValueExtents(double &min, double &max, bool &logarithmic, QString &unit) const override
Return the minimum and maximum values for the y axis of the model in this layer, as well as whether t...
Definition: SliceLayer.cpp:1161
int getVerticalZoomSteps(int &defaultStep) const override
Get the number of vertical zoom steps available for this layer.
Definition: SliceLayer.cpp:1229
bool setDisplayExtents(double min, double max) override
Set the displayed minimum and maximum values for the y axis to the given range, if supported...
Definition: SliceLayer.cpp:1199
Definition: SingleColourLayer.h:24
Definition: SliceLayer.h:84
Definition: SliceLayer.h:84
virtual double getValueForY(const LayerGeometryProvider *v, double y) const
Definition: SliceLayer.cpp:372
Definition: Layer.h:352
bool hasLightBackground() const override
Return true if the layer currently has a dark colour on a light background, false if it has a light c...
Definition: SliceLayer.cpp:729
PropertyType getPropertyType(const PropertyName &) const override
Definition: SliceLayer.cpp:774
Definition: SliceLayer.h:86
Definition: SliceLayer.h:84
QString getPropertyIconName(const PropertyName &) const override
Definition: SliceLayer.cpp:767
Definition: SliceLayer.h:27
ModelId getModel() const override
Return the ID of the model represented in this layer.
Definition: SliceLayer.h:35
Definition: SliceLayer.h:82
QString getFeatureDescription(LayerGeometryProvider *v, QPoint &) const override
Definition: SliceLayer.cpp:94
QString getPropertyValueLabel(const PropertyName &, int value) const override
Definition: SliceLayer.cpp:890
Definition: SliceLayer.h:86
Definition: SliceLayer.h:86
Interface for classes that provide geometry information (such as size, start frame, and a large number of other properties) about the disposition of a layer.
Definition: LayerGeometryProvider.h:45
RangeMapper * getNewPropertyRangeMapper(const PropertyName &) const override
Definition: SliceLayer.cpp:934
Definition: SliceLayer.h:82
Definition: SliceLayer.h:82
Definition: SliceLayer.h:88
double getXForScalePoint(const LayerGeometryProvider *, double p, double pmin, double pmax) const
Convert a point such as a bin number into x-coord, given max & min.
Definition: SliceLayer.cpp:196
virtual double getYForValue(const LayerGeometryProvider *v, double value, double &norm) const
Definition: SliceLayer.cpp:320
Definition: SliceLayer.h:88
virtual double getBinForX(const LayerGeometryProvider *, double x) const
Convert an x-coord into (possibly non-integral) bin. May be overridden.
Definition: SliceLayer.cpp:265
bool isLayerScrollable(const LayerGeometryProvider *) const override
This should return true if the layer can safely be scrolled automatically by a given view (simply cop...
Definition: SliceLayer.h:80
QString getPropertyLabel(const PropertyName &) const override
Definition: SliceLayer.cpp:754
RangeMapper * getNewVerticalZoomRangeMapper() const override
Create and return a range mapper for vertical zoom step values.
Definition: SliceLayer.cpp:1270
double getScalePointForX(const LayerGeometryProvider *, double x, double pmin, double pmax) const
Convert an x-coord into a point such as a bin number, given max & min.
Definition: SliceLayer.cpp:271
virtual void zoomToRegion(const LayerGeometryProvider *, QRect) override
Update the X and Y axis scales, where appropriate, to focus on the given rectangular region...
Definition: SliceLayer.cpp:1281
int getDefaultColourHint(bool dark, bool &impose) override
Definition: SliceLayer.cpp:1064
void setProperty(const PropertyName &, int value) override
Definition: SliceLayer.cpp:946
bool getNormalize() const
void paint(LayerGeometryProvider *v, QPainter &paint, QRect rect) const override
Paint the given rectangle of this layer onto the given view using the given painter, superimposing it on top of any existing material in that view.
Definition: SliceLayer.cpp:409
void setProperties(const QXmlAttributes &) override
Set the particular properties of a layer (those specific to the subclass) from a set of XML attribute...
Definition: SliceLayer.cpp:1111
virtual QString getFeatureDescriptionAux(LayerGeometryProvider *v, QPoint &, bool includeBinDescription, int &minbin, int &maxbin, int &range) const
Definition: SliceLayer.cpp:101
virtual double getXForBin(const LayerGeometryProvider *, double bin) const
Convert a (possibly non-integral) bin into x-coord. May be overridden.
Definition: SliceLayer.cpp:190
Definition: SliceLayer.h:86
ColourSignificance getLayerColourSignificance() const override
Implements Layer::getLayerColourSignificance()
Definition: SliceLayer.h:46
void toXml(QTextStream &stream, QString indent="", QString extraAttributes="") const override
Definition: SliceLayer.cpp:1072
QString getPropertyGroupName(const PropertyName &) const override
Definition: SliceLayer.cpp:788
int getPropertyRangeAndValue(const PropertyName &, int *min, int *max, int *deflt) const override
Definition: SliceLayer.cpp:801
int getVerticalScaleWidth(LayerGeometryProvider *v, bool, QPainter &) const override
Definition: SliceLayer.cpp:665
void sliceableModelReplaced(ModelId, ModelId)
Definition: SliceLayer.cpp:84
Definition: SliceLayer.h:82
Generated by
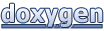